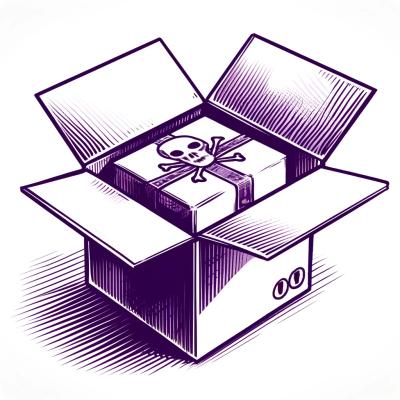
Security News
Research
Data Theft Repackaged: A Case Study in Malicious Wrapper Packages on npm
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
The pg-int8 npm package is designed to handle 64-bit integers (int8) in PostgreSQL. It provides functionality to parse and serialize 64-bit integers, which are not natively supported in JavaScript due to its limitation of 53-bit integer precision.
Parsing 64-bit Integers
This feature allows you to parse a string representation of a 64-bit integer into a BigInt in JavaScript. This is useful when dealing with PostgreSQL int8 values.
const pgInt8 = require('pg-int8');
const parsedValue = pgInt8.parse('9223372036854775807');
console.log(parsedValue); // 9223372036854775807n
Serializing 64-bit Integers
This feature allows you to serialize a BigInt in JavaScript back into a string representation of a 64-bit integer. This is useful when you need to store or transmit int8 values to PostgreSQL.
const pgInt8 = require('pg-int8');
const serializedValue = pgInt8.serialize(9223372036854775807n);
console.log(serializedValue); // '9223372036854775807'
The big-integer package provides arbitrary-precision arithmetic in JavaScript. It allows you to handle integers larger than the JavaScript Number type can natively support. While it is more general-purpose compared to pg-int8, it can be used to handle 64-bit integers as well.
The bignumber.js package is another library for arbitrary-precision arithmetic. It is similar to big-integer but also supports floating-point numbers. It can be used to handle 64-bit integers, but it is more versatile and can handle a wider range of numeric operations.
64-bit big-endian signed integer-to-string conversion designed for pg.
const readInt8 = require('pg-int8');
readInt8(Buffer.from([0, 1, 2, 3, 4, 5, 6, 7]))
// '283686952306183'
FAQs
64-bit big-endian signed integer-to-string conversion
The npm package pg-int8 receives a total of 6,647,384 weekly downloads. As such, pg-int8 popularity was classified as popular.
We found that pg-int8 demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
Research
Security News
Attackers used a malicious npm package typosquatting a popular ESLint plugin to steal sensitive data, execute commands, and exploit developer systems.
Security News
The Ultralytics' PyPI Package was compromised four times in one weekend through GitHub Actions cache poisoning and failure to rotate previously compromised API tokens.