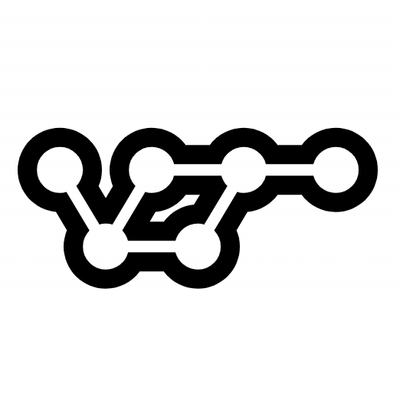
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
phantom-worker
Advanced tools
A PhantomJS worker. Execute the Single Page Application then return html.
git clone https://github.com/kelp404/phantom-worker.git
cd phantom-worker
npm install
pm2 start process.json
const device = require('device');
const phantomWorker = require('phantom-worker');
exports.baseView = function (req, res, next) {
if (device(req.headers['user-agent']).type === 'bot') {
// Render the html for bots.
phantomWorker.render({
url: req.protocol + '://' + req.get('host') + req.originalUrl,
isRemoveCompletedJob: true
}).then(function(content){
res.send(content);
});
return;
}
};
You also can create the task by yourself via Kue.
const device = require('device');
const kue = require('kue');
const queue = kue.createQueue({
redis: {
host: 'localhost',
port: 6379,
db: 2
}
});
exports.baseView = function (req, res, next) {
if (device(req.headers['user-agent']).type === 'bot') {
// Render the html for bots.
const job = queue.create('phantom-worker', {
url: req.protocol + '://' + req.get('host') + req.originalUrl
});
job.on('complete', function (content) {
job.remove();
res.send(content);
});
job.save(function(error) {
if (error) {
next(new Error(error));
}
});
return;
}
};
phantom-worker use config.
You can add your config for your NODE_ENV
.
/config/production.js
module.exports = {
phantomWorker: {
name: 'phantom-worker',
isCleanAllScriptTags: true, // Remove all <script> in the html when it is true.
concurrentQuantity: 2, // How many PhantomJS work in the same time?
contentCacheTTL: 86400, // 24 hrs = 86400s
contentCacheRedisPrefix: 'c:',
phantomArguments: [
'--load-images=no',
'--disk-cache=yes',
'--disk-cache-path=/tmp/phantom-worker'
],
readContentAfter: 0, // minute seconds. If it is 0 checkContentScript will bee executed.
checkContentInterval: 200, // minute seconds
checkContentRetryTimes: 50,
checkContentScript: "function(){return document.getElementsByClassName('nprogress-busy').length <= 0}",
redis: {
host: 'localhost',
port: 6379,
password: '',
db: 2
}
}
};
If your web use NProgress. You can use this script to check the client site render is done.
function(){
return document.getElementsByClassName('nprogress-busy').length <= 0;
}
FAQs
A PhantomJS worker. Execute the Single Page Application then return html.
The npm package phantom-worker receives a total of 1 weekly downloads. As such, phantom-worker popularity was classified as not popular.
We found that phantom-worker demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.