What is phin?
The phin npm package is a lightweight HTTP client designed for simplicity and minimalism. It is used for making HTTP requests from Node.js environments. It supports promises and can handle various types of requests such as GET, POST, and more.
What are phin's main functionalities?
Simple HTTP GET requests
This code sample demonstrates how to perform a simple HTTP GET request to a specified URL using phin.
const phin = require('phin')
phin('https://example.com', (err, res) => {
if (err) throw err
console.log(res.body)
})
HTTP POST requests with JSON
This code sample shows how to perform an HTTP POST request with JSON data using phin.
const phin = require('phin')
const options = {
url: 'https://example.com/post',
method: 'POST',
headers: {'Content-Type': 'application/json'},
data: { key: 'value' }
}
phin(options, (err, res) => {
if (err) throw err
console.log(res.body)
})
Promisified HTTP requests
This code sample illustrates how to use phin with promises to make asynchronous HTTP requests.
const phin = require('phin').promisified
async function makeRequest() {
try {
const res = await phin('https://example.com')
console.log(res.body)
} catch (err) {
console.error(err)
}
}
makeRequest()
Other packages similar to phin
axios
Axios is a popular HTTP client for the browser and Node.js. It supports promises by default and has a wide range of features including interceptors, automatic transforms for JSON data, and client-side protection against XSRF. It is more feature-rich compared to phin but also larger in size.
got
Got is another HTTP request library for Node.js that is designed to be more user-friendly and powerful than the built-in http module. It supports streams, advanced timeout handling, and instances with custom defaults. It is more comprehensive than phin but also more complex.
node-fetch
node-fetch is a light-weight module that brings the Fetch API to Node.js. It aims to provide a consistent API with the browser's fetch function. It is similar to phin in terms of simplicity but follows the Fetch API standards.
superagent
Superagent is a small progressive client-side HTTP request library. It has a fluent API that allows chaining of methods to configure requests, and it can be extended with plugins. It is more feature-rich than phin and has a different API design focused on chaining methods.
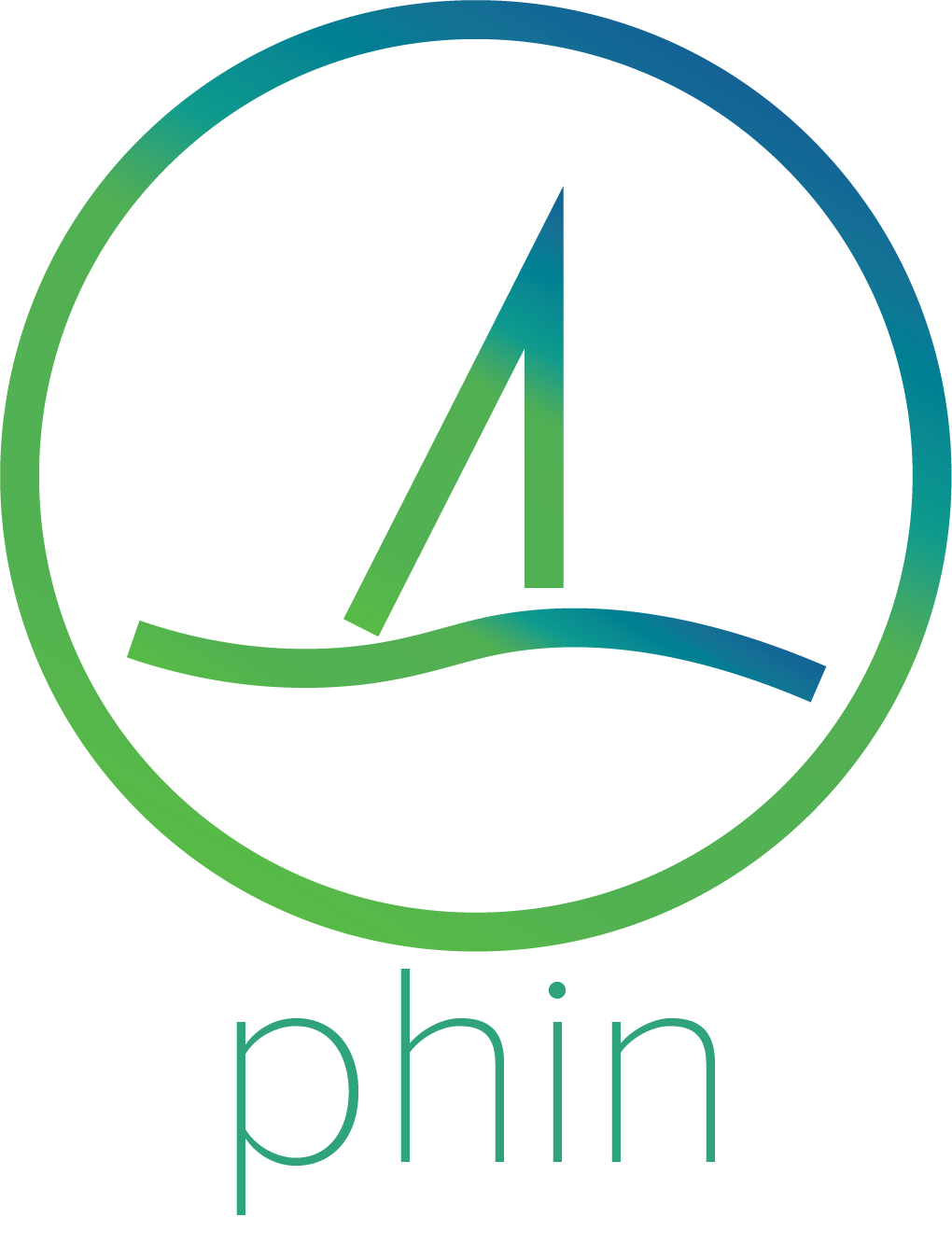
The ultra-lightweight Node.js HTTP client
Full documentation | GitHub | NPM
Simple Usage
const p = require('phin')
const res = await p('https://ethanent.me')
console.log(res.body)
Note that the above should be in an async context! phin also provides an unpromisified version of the library.
Install
npm install phin
Why phin?
phin is trusted by some really important projects. The hundreds of contributors at Less, for example, depend on phin as part of their development process.
Also, phin is super lightweight. Like 99.8% smaller than request lightweight. To compare to other libraries, see phin vs. the Competition.
Quick Demos
Simple POST:
await p({
url: 'https://ethanent.me',
method: 'POST',
data: {
hey: 'hi'
}
})
Unpromisified Usage
const p = require('phin').unpromisified
p('https://ethanent.me', (err, res) => {
if (!err) console.log(res.body)
})
Simple parsing of JSON:
const res = await p({
'url': 'https://ethanent.me/name',
'parse': 'json'
})
console.log(res.body.first)
Default Options
const ppostjson = p.defaults({
'method': 'POST',
'parse': 'json',
'timeout': 2000
})
const res = await ppostjson('https://ethanent.me/somejson')
Custom Core HTTP Options
phin allows you to set core HTTP options.
await p({
'url': 'https://ethanent.me/name',
'core': {
'agent': myAgent
}
})
Full Documentation
There's a lot more which can be done with the phin library.
See the phin documentation.
phin vs. the Competition
phin is a very lightweight library, yet it contains all of the common HTTP client features included in competing libraries!
Here's a size comparison table:
Package | Size |
---|
request |  |
superagent |  |
isomorphic-fetch |  |
axios |  |
got |  |
r2 |  |
node-fetch |  |
snekfetch |  |
phin |  |