popup-prompt
Advanced tools
Comparing version 1.1.0 to 1.1.5
@@ -7,2 +7,9 @@ # Changelog | ||
### `v1.1.5` | ||
- Added `componentListBox` method to the `Popup` class. This is used to allow the user to select an available option. | ||
- Added new `showCredentials` function. This asks the user for their username and password of any account (which you can specifiy). _Note: The username and password will be returned, so you will have to validate it._ | ||
- Fixed a few minor bugs and code format. | ||
- Resolved the issue where icons and images would not show up correctly ([#5](https://github.com/arnavthorat78/Popup-Prompt/issues/5)). | ||
### `v1.1.0` | ||
@@ -9,0 +16,0 @@ |
@@ -164,2 +164,50 @@ // MIT License | ||
/** | ||
* Show a credential prompt to the user. On Windows, this includes a picture of keys as the header, along with the username and password input. | ||
* | ||
* This method returns an object, with the username, password, and error. If an error occured, then username and password are `null`, otherwise, if everything went okay, error is `null`. | ||
* | ||
* Please note that the password is stored in plain text. It is your responsibility to make the string secure. | ||
* | ||
* Example: | ||
* | ||
* ```js | ||
* popup | ||
* .showCredentials( | ||
* "Popup Prompt Login", | ||
* "Please enter your credentials to login to Popup Prompt.", | ||
* "", | ||
* "popup-prompt" | ||
* ) | ||
* .then((cred) => { | ||
* console.log(cred); | ||
* }) | ||
* .catch((err) => { | ||
* console.log(err); | ||
* }); | ||
* ``` | ||
* | ||
* If the user does not cancel the authentication, then the returned value should be an object. The popup will look as below (on Windows 10). | ||
* | ||
* 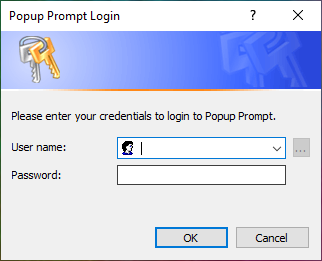 | ||
* | ||
* That's all! | ||
* | ||
* @param title The title to display on the top of the popup. | ||
* @param message The message to display to the user, telling them what the credential prompt is for. | ||
* @param username The default username to show in the username field. | ||
* @param targetName The name that will show behind the username. This shows the target of the user. | ||
* @returns A `Promise`, which contains an object, having the `username`, `password`, and `error` parameters. | ||
*/ | ||
export const showCredentials: ( | ||
title: string, | ||
message: string, | ||
username?: string, | ||
targetName?: string | ||
) => Promise<{ | ||
username: string | null; | ||
password: string | null; | ||
error: string | null; | ||
}>; | ||
////////////// | ||
@@ -339,2 +387,24 @@ // Classes // | ||
): this; | ||
/** | ||
* Create a list box, which is a list of items for the user to select from. | ||
* | ||
* _Note: This method does not open the popup/window. To do this, run `openPopup`._ | ||
* | ||
* @param name A name, which is the list's variable name. **Beware that if you pass a value that exists, there could be errors.** | ||
* @param items An array of strings, which are the items to display. | ||
* @param location The location. This is an array of two numbers. These numbers determine the location from the top-left corner of the popup text, from the top-left of the popup window. For example, if the numbers are `[10, 10]`, then `10` is the width, and `10` is the height. | ||
* @param size The size. This is an array of two numbers. These numbers determine the size of the list box. | ||
* @param height The height. This is the displayed height. | ||
* @param listen This parameter specifies if the end result should be the selected value. Default value is `false`. | ||
* @param windowName The name of the window. **It is not recommended to change this value**, since if you do change it, then you will have to pass it in almost every other method. Therefore, it is easier to leave it alone (the default value is `window`). | ||
*/ | ||
componentListBox( | ||
name: string, | ||
items: string[], | ||
location: [number, number], | ||
size: [number, number], | ||
height: number, | ||
listen?: boolean, | ||
windowName?: string | ||
): this; | ||
@@ -341,0 +411,0 @@ /** |
@@ -30,2 +30,3 @@ // MIT License | ||
const showPrompt = require("./lib/functions/showPrompt").showPrompt; | ||
const showCredentials = require("./lib/functions/showCredentials").showCredentials; | ||
@@ -40,3 +41,4 @@ // Getting classes | ||
showPrompt, | ||
showCredentials, | ||
Popup, | ||
}; |
@@ -79,3 +79,3 @@ // MIT License | ||
#getUserDir(relativePath) { | ||
const userDir = path.dirname(__dirname); | ||
const userDir = process.cwd(); | ||
const fullPath = path.resolve(userDir, relativePath); | ||
@@ -95,3 +95,3 @@ | ||
let code = `\n | ||
[System.Windows.Forms.Application]::EnableVisualStyles(); | ||
[System.Windows.Forms.Application]::EnableVisualStyles() | ||
$${name} = New-Object System.Windows.Forms.Form | ||
@@ -138,3 +138,3 @@ $${name}.Text = "${title}" | ||
code += `\n$${name}.Add_Click({ | ||
Set-Content .\\popup.txt $${name}.DialogResult; | ||
Set-Content .\\popup.txt $${name}.DialogResult | ||
})`; | ||
@@ -155,3 +155,3 @@ } | ||
code += `\n$${name}.add_KeyUp({ | ||
Set-Content .\\popup.txt $${name}.Text; | ||
Set-Content .\\popup.txt $${name}.Text | ||
})`; | ||
@@ -168,11 +168,11 @@ } | ||
let code = `\n | ||
$${fileName} = (Get-Item "${this.#getUserDir(path)}"); | ||
$${imgName} = [System.Drawing.Image]::Fromfile($${fileName}); | ||
$${fileName} = (Get-Item "${this.#getUserDir(path)}") | ||
$${imgName} = [System.Drawing.Image]::Fromfile($${fileName}) | ||
$${name} = New-Object Windows.Forms.PictureBox; | ||
$${name}.Width = $${imgName}.Size.Width; | ||
$${name}.Height = $${imgName}.Size.Height; | ||
$${name}.Location = New-Object System.Drawing.Point(${location[0]}, ${location[1]}); | ||
$${name}.Image = $${imgName}; | ||
$${windowName}.Controls.Add($${name}); | ||
$${name} = New-Object Windows.Forms.PictureBox | ||
$${name}.Width = $${imgName}.Size.Width | ||
$${name}.Height = $${imgName}.Size.Height | ||
$${name}.Location = New-Object System.Drawing.Point(${location[0]}, ${location[1]}) | ||
$${name}.Image = $${imgName} | ||
$${windowName}.Controls.Add($${name}) | ||
`; | ||
@@ -183,3 +183,26 @@ | ||
} | ||
componentListBox(name, items, location, size, height, listen = false, windowName = "window") { | ||
let list = ""; | ||
items.forEach((item) => { | ||
list += `[void] $${name}.Items.Add("${item}")\n`; | ||
}); | ||
let code = `\n | ||
$${name} = New-Object System.Windows.Forms.ListBox | ||
$${name}.Location = New-Object System.Drawing.Point(${location[0]}, ${location[1]}) | ||
$${name}.Size = New-Object System.Drawing.Size(${size[0]}, ${size[1]}) | ||
$${name}.Height = ${height} | ||
${list} | ||
$${windowName}.Controls.Add($${name})`; | ||
if (listen) { | ||
code += `\n$${name}.add_SelectedIndexChanged({ | ||
Set-Content .\\popup.txt $${name}.SelectedItem | ||
})`; | ||
} | ||
this.#code.components += code; | ||
return this; | ||
} | ||
renderWindow(topmost = false, name = "window") { | ||
@@ -186,0 +209,0 @@ const addTypes = `Add-Type -AssemblyName System.Windows.Forms |
@@ -46,4 +46,10 @@ // MIT License | ||
}, | ||
showCredentials: { | ||
title: [], | ||
message: [], | ||
username: [], | ||
targetName: [], | ||
}, | ||
}; | ||
module.exports = { AVAILABLE_VALUES }; |
@@ -23,4 +23,4 @@ // MIT License | ||
const VERSION = "1.1.0"; | ||
const VERSION = "1.1.5"; | ||
module.exports = { VERSION }; |
{ | ||
"name": "popup-prompt", | ||
"version": "1.1.0", | ||
"version": "1.1.5", | ||
"description": "Show popup and prompt windows to your users.", | ||
@@ -19,2 +19,3 @@ "license": "MIT", | ||
"prompt", | ||
"credentials", | ||
"npm", | ||
@@ -21,0 +22,0 @@ "powershell", |
121
README.md
@@ -30,2 +30,3 @@ # Popup-Prompt | ||
- [Method: `showPrompt`](#method-showprompt) | ||
- [Method: `showCredentials`](#method-showcredentials) | ||
- [Class: `Popup`](#class-popup) | ||
@@ -37,2 +38,3 @@ - [`createWindow`](#createwindow) | ||
- [`componentImage`](#componentimage) | ||
- [`componentListBox`](#componentlistbox) | ||
- [`renderWindow`](#renderwindow) | ||
@@ -87,9 +89,10 @@ - [`openPopup`](#openpopup) | ||
| Name & Parameters | Type | Description | | ||
| -------------------------------------------------------------------- | -------- | ---------------------------------------------------- | | ||
| `VERSION` | Constant | Show the current version of the package. | | ||
| `AVAILABLE_VALUES` | Constant | Show the values that can be passed into the methods. | | ||
| `showMessageBox(title, message[, type][, picture][, defaultOption])` | Method | Show a customizable message popup window. | | ||
| `showPrompt(title, message[, defaultValue])` | Method | Show a customizable prompt popup window. | | ||
| `Prompt()` | Class | Make a fully customizable popup window. | | ||
| Name & Parameters | Type | Description | | ||
| -------------------------------------------------------------------- | -------- | -------------------------------------------------------------- | | ||
| `VERSION` | Constant | Show the current version of the package. | | ||
| `AVAILABLE_VALUES` | Constant | Show the values that can be passed into the methods. | | ||
| `showMessageBox(title, message[, type][, picture][, defaultOption])` | Method | Show a message popup window. | | ||
| `showPrompt(title, message[, defaultValue])` | Method | Show a prompt popup window, in which a user can enter text in. | | ||
| `showCredentials(title, message[, username][, targetName])` | Method | Show a credential popup window. | | ||
| `Popup()` | Class | Make a fully customizable popup window. | | ||
@@ -102,9 +105,8 @@ ## Versions (Legacy and Coming) | ||
Version **1.1.0** included... | ||
Version **1.1.5** included... | ||
- Added `componentImage` method to the `Popup` class. This can be used to add any type of image. | ||
- Added relative path support to icons and images for the `Popup` class. | ||
- Added support for non-resizable `Popup` windows. | ||
- Added discriptive errors. | ||
- Added real-life example in `README`. | ||
- Added `componentListBox` method to the `Popup` class. This is used to allow the user to select an available option. | ||
- Added new `showCredentials` function. This asks the user for their username and password of any account (which you can specifiy). _Note: The username and password will be returned, so you will have to validate it._ | ||
- Fixed a few minor bugs and code format. | ||
- Resolved the issue where icons and images would not show up correctly ([#5](https://github.com/arnavthorat78/Popup-Prompt/issues/5)). | ||
@@ -115,11 +117,10 @@ ### Coming Version | ||
Version **1.1.5** is set to include... | ||
Version **1.2.0** is set to include... | ||
- Added `componentListBox` method to the `Popup` class. This is used to allow the user to select an available option. | ||
- Added new `showCredentials` function. This asks the user for their username and password of any account (which you can specifiy). _Note: The username and password will be returned, so you will have to validate it._ | ||
- Fixed a few minor bugs and code format. | ||
- Added a new methods in the `Popup` class called `componentCalendar`. This displays a calendar for the user to select a date from. | ||
- Fixed a few bugs. | ||
## Usage | ||
There are currently two methods, two constants, and one class available. They will be shown below. | ||
There are currently three methods, two constants, and one class available. They will be shown below. | ||
@@ -168,3 +169,9 @@ ### Constant: `VERSION` | ||
}, | ||
showPrompt: { title: [], message: [], defaultValue: [] } | ||
showPrompt: { title: [], message: [], defaultValue: [] }, | ||
showCredentials: { | ||
title: [], | ||
message: [], | ||
username: [], | ||
targetName: [], | ||
}, | ||
} | ||
@@ -312,2 +319,54 @@ ``` | ||
### Method: `showCredentials` | ||
``` | ||
showCredentials(title, message[, username][, targetName]) | ||
``` | ||
The method creates a credential popup, which asks the user for their credentials. This includes the title, the message, and a default username and/or target name. | ||
_Note: When you run this method, you will notice a PowerShell file appear in your current working directory. This is essential for the credential to display. Also, when the user enters their text, a text file will appear with the text entered. This is for the PowerShell file to communicate with NodeJS._ | ||
This method returns a `Promise`, which contains an object with credential and error information. _Note: If the user presses the Cancel button, presses the **X** (close) button, or an error occured, then the returned value will have `username` and `password` be `null`._ | ||
Below is an example of how to use the method. | ||
```js | ||
popup | ||
.showCredentials( | ||
"Popup Prompt Login", | ||
"Please enter your credentials to login to Popup Prompt.", | ||
"", | ||
"popup-prompt" | ||
) | ||
.then((cred) => { | ||
console.log(cred); | ||
}) | ||
.catch((err) => { | ||
console.log(err); | ||
}); | ||
``` | ||
When you run the code, a PowerShell file will appear in your current working directory, and then, a credential popup, similar to the one below (depending on your OS) will show up. | ||
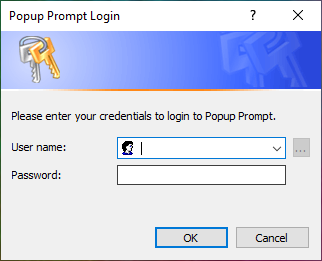 | ||
Once the user clicks the _OK_ button, the _Cancel_ button, or the _Close_ button, a text file will appear in your current working directory, and then, in the terminal/command prompt, an object will appear. The data may varie, depending on how the user reacted. | ||
``` | ||
{ username: 'popup-prompt\\popup', password: 'password', error: null } | ||
``` | ||
Now, let's go over what happens. | ||
The first parameter is the title to display on the top of the popup. | ||
The second parameter is the message to display to the user, telling them what the credential prompt is for. | ||
The third parameter is the default username to show in the username field. | ||
The fourth parameter is the name that will show behind the username. This shows the target of the user. | ||
And that's how easy it is to display a credential popup to your user! | ||
### Class: `Popup` | ||
@@ -542,2 +601,26 @@ | ||
#### `componentListBox` | ||
``` | ||
componentListBox(name, items, location, size, height[, listen][, windowName]) | ||
``` | ||
Create a list box, which is a list of items for the user to select from. | ||
_Note: This method does not open the popup/window. To do this, run `openPopup`._ | ||
The first parameter is the name, which is the list's variable name. **Beware that if you pass a value that exists, there could be errors.** | ||
The second parameter is the array of strings, which are the items to display. | ||
The third parameter is the location. This is an array of two numbers. These numbers determine the location from the top-left corner of the popup text, from the top-left of the popup window. For example, if the numbers are `[10, 10]`, then `10` is the width, and `10` is the height. | ||
The fourth parameter is the size. This is an array of two numbers. These numbers determine the size of the list box. | ||
The fifth parameter is the height. This is the displayed height. | ||
The sixth parameter specifies if the end result should be the selected value. Default value is `false`. | ||
The seventh parameter is the name of the window. **It is not recommended to change this value**, since if you do change it, then you will have to pass it in almost every other method. Therefore, it is easier to leave it alone (the default value is `window`). | ||
#### `renderWindow` | ||
@@ -544,0 +627,0 @@ |
89276
17
974
864
4