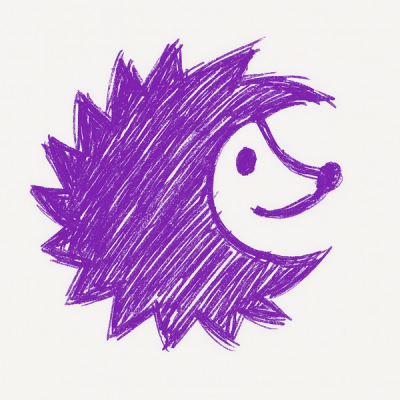
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
posthtml-fetch
Advanced tools
This plugin allows you to fetch remote content and display it in your HTML.
Input:
<fetch url="https://jsonplaceholder.typicode.com/users/1">
{{ response.name }}'s username is {{ response.username }}
</fetch>
Output:
Leanne Graham's username is Bret
$ npm i posthtml posthtml-fetch
const posthtml = require('posthtml')
const pf = require('posthtml-fetch')
posthtml()
.use(pf())
.process('<fetch url="https://example.test">{{ response }}</fetch>')
.then(result => console.log(result.html))
// response body
The response body will be available under the response
local variable.
The plugin supports json
and text
responses.
Only the response body is returned.
The plugin uses posthtml-expressions
, so you can use any of its tags to work with the response
.
For example, you can iterate over items in a JSON response:
<fetch url="https://jsonplaceholder.typicode.com/users">
<each loop="user in response">
{{ user.name }}
</each>
</fetch>
You can configure the plugin with the following options.
tags
Default: ['fetch', 'remote']
Array of supported tag names.
Only tags from this array will be processed by the plugin.
Example:
const posthtml = require('posthtml')
const pf = require('posthtml-fetch')
posthtml()
.use(pf({
tags: ['get']
}))
.process('<get url="https://example.test">{{ response }}</get>')
.then(result => console.log(result.html))
attribute
Default: 'url'
String representing attribute name containing the URL to fetch.
Example:
const posthtml = require('posthtml')
const pf = require('posthtml-fetch')
posthtml()
.use(pf({
attribute: 'from'
}))
.process('<fetch from="https://example.test">{{ response }}</fetch>')
.then(result => {
console.log(result.html)
// => ...interpolated response from https://example.test
})
got
The plugin uses got
to fetch data. You can pass options directly to it, inside the got
object.
Example:
const posthtml = require('posthtml')
const pf = require('posthtml-fetch')
posthtml()
.use(pf({
got: {
// pass options to got...
}
}))
.process('<fetch url="https://example.test">{{ response }}</fetch>')
.then(result => {
console.log(result.html)
// => ...interpolated response from https://example.test
})
preserveTag
Allows you to leave an item. Default value false
.
Example:
const posthtml = require('posthtml')
const pf = require('posthtml-fetch')
posthtml()
.use(pf({
preserveTag: true
}))
.process('<fetch url="https://example.test">{{ response }}</fetch>')
.then(result => {
console.log(result.html)
// => <fetch url="https://example.test">...interpolated response from https://example.test</fetch>
})
after/before
List of plugins that will be called after/before receiving and processing locals
Example:
const posthtml = require('posthtml')
const pf = require('posthtml-fetch')
posthtml()
.use(pf({
plugins: {
after(tree) {
// Your plugin implementation
},
before: [
tree => {
// Your plugin implementation
},
tree => {
// Your plugin implementation
}
]
}
}))
.process('<fetch url="https://example.test">{{ response }}</fetch>')
.then(result => {
console.log(result.html)
// => ...interpolated response from https://example.test
})
FAQs
PostHTML plugin for fetching and displaying remote or local content.
The npm package posthtml-fetch receives a total of 19,520 weekly downloads. As such, posthtml-fetch popularity was classified as popular.
We found that posthtml-fetch demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.