Pressure.js

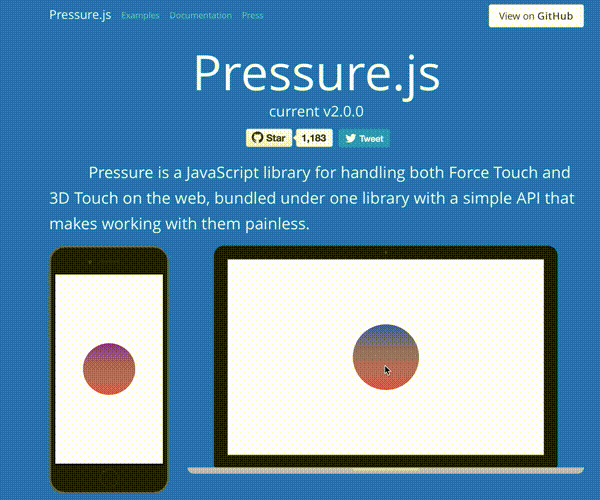
Pressure is a JavaScript library for handling both Force Touch and 3D Touch on the web, bundled under one library with a simple API that makes working with them painless.
Head over to the documentation for installation instructions, supported devices, and more details on pressure.js.
Install
Download pressure.min.js or pressure.js files from GitHub or install with npm or bower
npm
npm install pressure --save
bower
bower install pressure --save
Setup
Use pressure in the global space:
Pressure.set('#id-name', {
change: function(force){
this.innerHTML = force;
}
});
OR use it with browserify or CommonJS like setups:
var Pressure = require('pressure');
Pressure.set('#id-name', {
change: function(force){
this.innerHTML = force;
}
});
Usage
NOTE: the "this" keyword in each of the callback methods will be the element itself that has force applied to it
Pressure.set('#element', {
start: function(event){
},
end: function(){
},
startDeepPress: function(event){
},
endDeepPress: function(){
},
change: function(force, event){
},
unsupported: function(){
}
});
jQuery Usage
NOTE: the "this" keyword in each of the callback methods will be the element itself that has force applied to it
$('#element').pressure({
start: function(event){
},
end: function(){
},
startDeepPress: function(event){
},
endDeepPress: function(){
},
change: function(force, event){
},
unsupported: function(){
}
});
Options
With Pressure, the third paramater is an optional object of options that can be passed in.
Polyfill Support
Using the "polyfill" keyword, you can disable polyfill support for the element. The polyfill is enabled by default and is useful if the device or browser does not support pressure, it will fall back to using time. For example instead of force from 0 to 1, it counts up from 0 to 1 over the course of one second, as long as you are holding the element. Try some of the examples on the main page on a devices that does not support pressure and see for yourself how it works.
Pressure.set('#example', {
change: function(force, event){
this.innerHTML = force;
},
unsupported: function(){
alert("Oh no, this device does not support pressure.");
}
}, {polyfill: false});
Polyfill Speed Up
If you are using the polyfill (on by default), you can see the "polyfillSpeedUp" speed to determine how fast the polyfill takes to go from 0 to 1. The value is an integer in milliseconds and the default is 1000 (1 second).
Pressure.set('#example', {
change: function(force, event){
this.innerHTML = force;
}
}, {polyfillSpeedUp: 5000});
Polyfill Speed Down
If you are using the polyfill (on by default), you can see the "polyfillSpeedDown" speed to determine how fast the polyfill takes to go from 1 to 0 when you let go. The value is an integer in milliseconds and the default is 0 (aka off).
Pressure.set('#example', {
change: function(force, event){
this.innerHTML = force;
}
}, {polyfillSpeedDown: 2000});
Only run on Force Touch trackpads (mouse)
Set the option only to the type you want it to run on 'mouse', 'touch', or 'pointer'. The names are the types of events that pressure will respond to.
Pressure.set('#example',{
change: function(force, event){
console.log(force);
},
}, {only: 'mouse'});
Only run on 3D Touch (touch)
Pressure.set('#example',{
change: function(force, event){
console.log(force);
},
}, {only: 'touch'});
Only run on Pointer Supported Devices (pointer)
Pressure.set('#example',{
change: function(force, event){
console.log(force);
},
}, {only: 'pointer'});
Change the preventSelect option
The preventDefault option in "true" by default and it prevents the default actions that happen on 3D "peel and pop" actions and the Force "define word" actions as well as other defaults. To allow the defaults to run set preventDefault to "false"
Pressure.set('#example',{
change: function(force, event){
console.log(force);
},
}, {preventSelect: false});
Helpers
Config
You can use Pressure.config()
to set default configurations for site wide setup. All of the configurations are the same as the options listed above.
Heads Up: If you have a config set, you can always overide the config on individual Pressure elements by passing in any of the options listed above to a specific Pressure block.
When using the jQuery Pressure library, use $.pressureConfig()
rather than Pressure.map()
Pressure.config({
polyfill: true,
polyfillSpeedUp: 1000,
polyfillSpeedDown: 0,
preventDefault: true,
only: null
});
Map
You can use Pressure.map()
to map a value from one range of values to another. It takes 5 params: Pressure.map(inputValue, inputValueMin, inputValueMax, mapToMin, mapToMax);
Here is a good write up on how this works in the Processing framework: Map Function.
When using the jQuery Pressure library, use $.pressureMap()
rather than Pressure.map()
Pressure.set('#element', {
change: function(force, event){
this.style.width = Pressure.map(force, 0, 1, 100, 200);
}
});