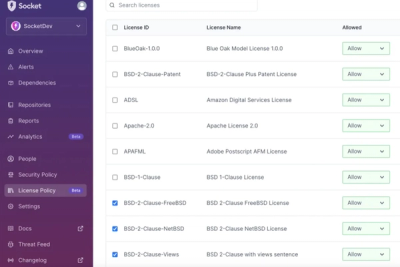
Product
Introducing License Enforcement in Socket
Ensure open-source compliance with Socket’s License Enforcement Beta. Set up your License Policy and secure your software!
prettier-plugin-embed
Advanced tools
A configurable Prettier plugin to format embedded languages in js/ts files.
A Configurable Prettier Plugin to Format Embedded Languages in js
/ts
Files.
npm i -D prettier-plugin-embed
This Prettier plugin (namely prettier-plugin-embed
) provides a configurable solution for formatting embedded languages in the form of template literals within JavaScript or TypeScript files.
Although Prettier has introduced the embedded-language-formatting
option for formatting embedded languages, it only supports two modes: auto
and off
. Therefore it doesn't allow for individual formatting adjustments for specific languages, nor does it support customizing the languages that require formatting and identifiers for identification. These limitations restrict the usability of this feature. For more detailed discussions, refer to: https://github.com/prettier/prettier/issues/4424 and https://github.com/prettier/prettier/issues/5588.
By leveraging Prettier's plugin system, this plugin overrides the default embed
function of the estree
printer, so varieties of new languages can be hooked in through this function. Check this file to get an idea of how this is accomplished.
Support for Additional Languages: Extend the embedded language formatting capability to include languages such as XML, SQL, PHP, and more.
Dual Identification Modes: Identify embedded languages by tags identifier`...`
or comments /* identifier */ `...`
preceding the template literals.
Customizable Language Identifiers: Customize the identifiers used for identifying the embedded languages.
Formatting Opt-out Mechanism: Offer the capability to deactivate formatting for certain identifiers, including the default ones (html
, css
...) supported by the embedded-language-formatting
option.
Configurable Formatting Style: Provide additional options to tailor the formatting style for embedded languages.
Strongly Typed API: Benefit from comprehensive type support for configuring this plugin's options when employing the Prettier API in TypeScript.
Easy Integration: Integrate with the existing Prettier setup seamlessly, requiring minimal configuration to get started.
npm i -D prettier-plugin-embed
This is a Prettier plugin, which follows the standard usage pattern of many other Prettier plugins:
Via --plugin
:
prettier --write main.ts --plugin=prettier-plugin-embed
Via the plugins
options:
await prettier.format(code, {
filepath: "main.ts",
plugins: ["prettier-plugin-embed"],
});
{
"plugins": ["prettier-plugin-embed"]
}
To use this plugin, embedded-language-formatting
option must be set to auto
(which is the default setting as of now), because this option serves as the main switch for activating embedded language formatting.
This plugin does not aim to implement parsers or printers to support every newly added embedded language. Instead, ideally, it makes use of existing Prettier plugins for those languages and only adds formatting support when they are embedded in template literals.
Therefore, to enable formatting for a specific embedded language, the corresponding Prettier plugin for that language must also be loaded. For example, if you wish to format embedded XML code, you will need to load both this plugin and @prettier/plugin-xml
. To find out which other plugins are required when using this plugin, please refer to the Language-Specific Options section below.
Embedded languages to be formatted are required to be enclosed in the template literals, and are identified by the preceding tags identifier`...`
or block comments /* identifier */ `...`
. This plugin comes pre-configured with a built-in set of identifiers for identifying various embedded languages. For instance, using identifiers like xml
or svg
will trigger automatic formatting for the embedded XML code. You can specify an alternative list of identifiers using the embeddedXmlIdentifiers
option. The naming convention for these options follows the pattern of embedded<Language>Identifiers
for other languages as well. Further details on these options and how to configure them are also available in the Language-Specific Options section.
To exclude certain identifiers from being identified, including the default ones supported by the embedded-language-formatting
option, add them to the list of the embeddedNoopIdentifiers
option. Any matching identifiers listed in this option will take precedence over other embedded<Language>Identifiers
options, effectively disabling their formatting.
Option | Default | Description |
---|---|---|
embeddedNoopIdentifiers | [] | Tag or comment identifiers that prevent their subsequent template literals from being identified as embedded languages and from being formatted |
This doesn't require other plugins and can override the native embedded language formatting. It serves as a way to turn off embedded language formatting for specific language identifiers.
Option | Default | Description |
---|---|---|
embeddedCssIdentifiers | [...] | Tag or comment identifiers that make their subsequent template literals be identified as CSS code |
Formatting embedded CSS code doesn't require other plugins and uses the parsers and printers provided by Prettier natively. This can override the native embedded language formatting for CSS code.
Option | Default | Description |
---|---|---|
embeddedEsIdentifiers | [...] | Tag or comment identifiers that make their subsequent template literals be identified as ECMAScript (JavaScript) code |
embeddedEsParser | "babel" | The parser used to parse the ECMASCript (JavaScript) code |
Formatting embedded ECMAScript code doesn't require other plugins and uses the parsers and printers provided by Prettier natively. This can override the native embedded language formatting for ECMAScript code.
Option | Default | Description |
---|---|---|
embeddedHtmlIdentifiers | [...] | Tag or comment identifiers that make their subsequent template literals be identified as ECMAScript (JavaScript) code |
Formatting embedded HTML code doesn't require other plugins and uses the parsers and printers provided by Prettier natively. This can override the native embedded language formatting for HTML code.
Option | Default | Description |
---|---|---|
embeddedMarkdownIdentifiers | [...] | Tag or comment identifiers that make their subsequent template literals be identified as Markdown code |
Formatting embedded Markdown code doesn't require other plugins and uses the parsers and printers provided by Prettier natively. This can override the native embedded language formatting for Markdown code.
Option | Default | Description |
---|---|---|
embeddedPhpIdentifiers | [...] | Tag or comment identifiers that make their subsequent template literals be identified as PHP code |
Formatting embedded PHP code requires @prettier/plugin-php
to be loaded as well. And options supported by @prettier/plugin-php
can therefore be used to further control the formatting behavior.
Option | Default | Description |
---|---|---|
embeddedRubyIdentifiers | [...] | Tag or comment identifiers that make their subsequent template literals be identified as Ruby code |
Formatting embedded Ruby code requires @prettier/plugin-ruby
to be loaded and its dependencies to be installed as well. And options supported by @prettier/plugin-php
can therefore be used to further control the formatting behavior.
Option | Default | Description |
---|---|---|
embeddedSqlIdentifiers | [...] | Tag or comment identifiers that make their subsequent template literals be identified as SQL code |
Formatting embedded SQL code requires prettier-plugin-sql
to be loaded as well. And options supported by prettier-plugin-sql
can therefore be used to further control the formatting behavior.
Note that prettier-plugin-sql
supports many different SQL dialects, and there's a one-to-one correspondence between the dialects and the identifiers. To support custom identifiers for a specific dialect, put the identifiers after that specific dialect in the identifier list in option embeddedSql
.
Option | Default | Description |
---|---|---|
embeddedTsIdentifiers | [...] | Tag or comment identifiers that make their subsequent template literals be identified as TypeScript code |
embeddedTsParser | "typescript" | The parser used to parse the TypeScript code |
Formatting embedded TypeScript code doesn't require other plugins and uses the parsers and printers provided by Prettier natively. This can override the native embedded language formatting for TypeScript code.
Option | Default | Description |
---|---|---|
embeddedXmlIdentifiers | [...] | Tag or comment identifiers that make their subsequent template literals be identified as XML code |
Formatting embedded XML code requires @prettier/plugin-xml
to be loaded as well. And options supported by @prettier/plugin-xml
can therefore be used to further control the formatting behavior.
Option | Default | Description |
---|---|---|
noEmbeddedIdentificationByComment | [] | Turns off /* identifier */ `...` comment-based language identification for the specified identifiers |
noEmbeddedIdentificationByTag | [] | Turns off identifier`...` tag-based language identification for the specified identifiers |
preserveEmbeddedExteriorWhitespaces | [] | Preserves leading and trailing whitespaces in the formatting results for the specified identifiers |
noEmbeddedMultiLineIndentation | [] | Turns off auto indentation in the formatting results when they are formatted to span multi lines for the specified identifiers |
TBD.
Bug fixes, new language support and tests are welcome. Please have a look at the project structure before getting started. Feel free to leave questions or suggestions.
MIT
FAQs
A configurable Prettier plugin to format embedded languages in JS/TS files.
The npm package prettier-plugin-embed receives a total of 12,139 weekly downloads. As such, prettier-plugin-embed popularity was classified as popular.
We found that prettier-plugin-embed demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Ensure open-source compliance with Socket’s License Enforcement Beta. Set up your License Policy and secure your software!
Product
We're launching a new set of license analysis and compliance features for analyzing, managing, and complying with licenses across a range of supported languages and ecosystems.
Product
We're excited to introduce Socket Optimize, a powerful CLI command to secure open source dependencies with tested, optimized package overrides.