What is prettier-plugin-java?
The prettier-plugin-java npm package is a plugin for Prettier that formats Java code. It ensures that Java code adheres to a consistent style, making it easier to read and maintain.
What are prettier-plugin-java's main functionalities?
Code Formatting
This feature automatically formats Java code to follow a consistent style. For example, it ensures proper indentation, spacing, and line breaks.
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
Consistent Style
This feature enforces a consistent coding style across the entire codebase, making it easier to read and maintain. It handles things like method spacing, bracket placement, and more.
public class Example {
private int value;
public int getValue() {
return value;
}
public void setValue(int value) {
this.value = value;
}
}
Integration with Prettier
This feature allows the plugin to be easily integrated with Prettier, enabling seamless formatting of Java code alongside other languages supported by Prettier.
{
"prettier": {
"plugins": ["prettier-plugin-java"]
}
}
Other packages similar to prettier-plugin-java
google-java-format
google-java-format is a program that reformats Java source code to comply with Google Java Style. It is similar to prettier-plugin-java in that it enforces a consistent style, but it specifically adheres to Google's style guide.
spotless
Spotless is a general-purpose code formatter that supports multiple languages, including Java. It can be configured to use different formatters, such as google-java-format or prettier-plugin-java, providing flexibility in formatting rules.

prettier-plugin-java
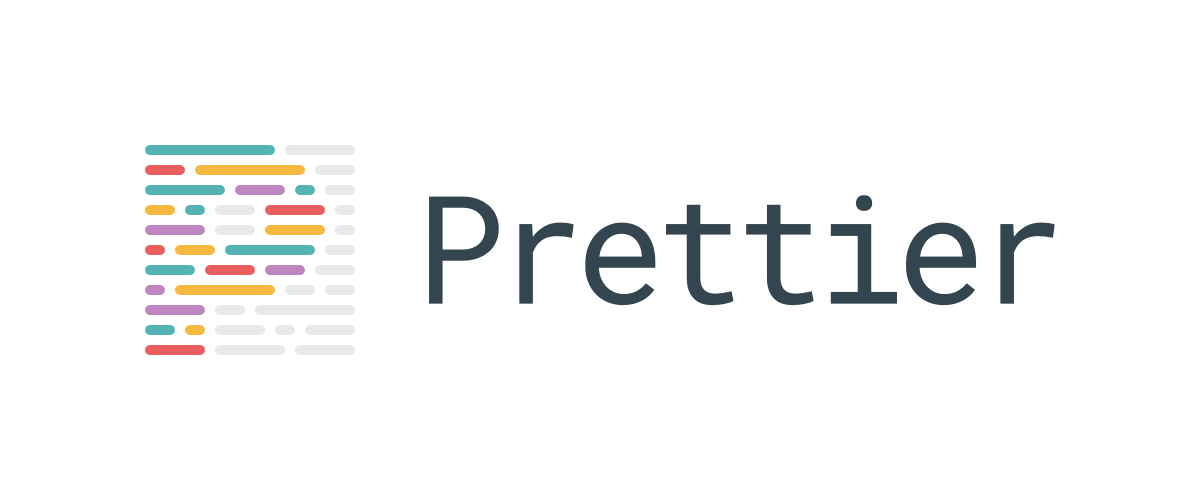
Prettier is an opinionated code formatter which forces a certain coding style. It makes the code consistent through an entire project.
This plugin allows the support of Java on Prettier.
The plugin implementation is pretty straightforward as it uses java-parser (thanks to Chevrotain) visitor to traverse the Concrete Syntax Tree and apply the format processing on each node (it uses Prettier API).
Installation
Pre-requirements
Since the plugin is meant to be used with Prettier, you need to install it:
npm install --save-dev --save-exact prettier
or
yarn add prettier --dev --exact
Install plugin
npm install prettier-plugin-java --save-dev
or
yarn add prettier-plugin-java --dev
CLI
If you installed Prettier globally and want to format java code via the CLI, run the following command:
npm install -g prettier-plugin-java
The plugin will be automatically loaded, check here for more.
Usage
CLI
prettier --write MyJavaFile.java
If the plugin is not automatically loaded:
prettier --write MyJavaFile.java --plugin=./node_modules/prettier-plugin-java
API
const prettier = require("prettier");
const javaText = `
public class HelloWorldExample{
public static void main(String args[]){
System.out.println("Hello World !");
}
}
`;
const formattedText = prettier.format(javaText, {
parser: "java",
tabWidth: 2
});
Example of formatted code
Input
public class HelloWorld {
public static void main(String[] args) {System.out.println("Hello World!");;;;;}
@Override
public String toString() {
return "Hello World";
}
public int sum(int argument1,int argument2,int argument3,int argument4,int argument5
) {
return argument1+argument2+ argument3 +argument4 + argument5;
}
}
Output
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello World!");
}
@Override
public String toString() {
return "Hello World";
}
public int sum(
int argument1,
int argument2,
int argument3,
int argument4,
int argument5
) {
return argument1 + argument2 + argument3 + argument4 + argument5;
}
}
Options
We added a custom option entrypoint
in order to run prettier on code snippet.
Usage
prettier --write MyJava.java --entrypoint compilationUnit
Here is the exhaustive list of all entrypoints.
Example
MyJavaCode.java content:
public void myfunction() {
mymethod.is().very().very().very().very().very().very().very().very().very().very().very().very().very().very().big();
}
Run:
prettier --write MyJavaCode.java --entrypoint classBodyDeclaration
Result:
public void myfunction() {
mymethod
.is()
.very()
.very()
.very()
.very()
.very()
.very()
.very()
.very()
.very()
.very()
.very()
.very()
.very()
.very()
.big();
}
v2.0.0
Breaking changes
- Drop support of Node.js 12
Enhancements
- Support pattern matching guards (Issue #535 closed with #559)
// Input
class T {
void test(Buyer other) {
return switch (other) {
case null -> true;
case Buyer b && this.bestPrice > b.bestPrice -> true;
case Buyer b && this.bestPrice > b.bestPrice -> {
return true;
}
case (Buyer b && this.bestPrice > b.bestPrice) -> true;
case Buyer b &&
this.bestPrice > b.bestPrice &&
this.bestPrice > b.bestPrice &&
this.bestPrice > b.bestPrice &&
this.bestPrice > b.bestPrice -> true;
case Buyer b &&
this.bestPrice > b.bestPrice &&
this.bestPrice > b.bestPrice &&
this.bestPrice > b.bestPrice &&
this.bestPrice > b.bestPrice -> {
return true;
}
case (
Buyer b &&
this.bestPrice > b.bestPrice &&
this.bestPrice > b.bestPrice &&
this.bestPrice > b.bestPrice &&
this.bestPrice > b.bestPrice
) -> true;
case (
Buyer b &&
this.bestPrice > b.bestPrice &&
this.bestPrice > b.bestPrice &&
this.bestPrice > b.bestPrice &&
this.bestPrice > b.bestPrice
) -> {
return true;
}
default -> false;
};
}
}
// Output
class T {
void test(Buyer other) {
return switch (other) {
case null -> true;
case Buyer b && this.bestPrice > b.bestPrice -> true;
case Buyer b && this.bestPrice > b.bestPrice -> {
return true;
}
case (Buyer b && this.bestPrice > b.bestPrice) -> true;
case Buyer b &&
this.bestPrice > b.bestPrice &&
this.bestPrice > b.bestPrice &&
this.bestPrice > b.bestPrice &&
this.bestPrice > b.bestPrice -> true;
case Buyer b &&
this.bestPrice > b.bestPrice &&
this.bestPrice > b.bestPrice &&
this.bestPrice > b.bestPrice &&
this.bestPrice > b.bestPrice -> {
return true;
}
case (
Buyer b &&
this.bestPrice > b.bestPrice &&
this.bestPrice > b.bestPrice &&
this.bestPrice > b.bestPrice &&
this.bestPrice > b.bestPrice
) -> true;
case (
Buyer b &&
this.bestPrice > b.bestPrice &&
this.bestPrice > b.bestPrice &&
this.bestPrice > b.bestPrice &&
this.bestPrice > b.bestPrice
) -> {
return true;
}
default -> false;
};
}
}
Fixes
- Fix parsing of escaped spaces in strings (Issue #541 closed with #543)
public class Test {
public static final String REGEX = "^\s$";
}
- Fix unwanted space in "exports"-statement in module-info.java (Issue #550 closed with #551)
Thanks to @BjornJaspers for the fix
// Input
open module org.myorg.module {
requires some.required.module;
exports org.myorg.module.exportpackage1;
exports org.myorg.module.exportpackage2;
}
// Prettier 1.6.2
open module org.myorg.module {
requires some.required.module;
exports org.myorg.module.exportpackage1;
exports org.myorg.module.exportpackage2;
}
// Prettier 1.6.3
open module org.myorg.module {
requires some.required.module;
exports org.myorg.module.exportpackage1;
exports org.myorg.module.exportpackage2;
}
Misc
- doc: add VSCode Java import order configuration (#546)
Thanks to @derkoe for the contribution