What is prettyjson?
The prettyjson npm package is a utility for formatting JSON data in a human-readable way. It provides a simple and customizable way to print JSON objects with colors and indentation, making it easier to debug and visualize JSON data.
What are prettyjson's main functionalities?
Basic JSON Formatting
This feature allows you to format a JSON object into a more readable string with indentation and colors. The example code demonstrates how to use prettyjson to render a simple JSON object.
const prettyjson = require('prettyjson');
const data = { name: 'John', age: 30, city: 'New York' };
console.log(prettyjson.render(data));
Customizing Colors
This feature allows you to customize the colors used for keys and values in the formatted JSON output. The example code shows how to set custom colors for keys and string values.
const prettyjson = require('prettyjson');
const data = { name: 'John', age: 30, city: 'New York' };
const options = { keysColor: 'red', stringColor: 'blue' };
console.log(prettyjson.render(data, options));
Rendering Arrays
This feature supports rendering arrays of JSON objects in a readable format. The example code demonstrates how to use prettyjson to render an array of JSON objects.
const prettyjson = require('prettyjson');
const data = [ { name: 'John', age: 30 }, { name: 'Jane', age: 25 } ];
console.log(prettyjson.render(data));
Other packages similar to prettyjson
json-stringify-pretty-compact
The json-stringify-pretty-compact package provides a way to stringify JSON data with a focus on compactness and readability. It offers a different approach to formatting compared to prettyjson, focusing on minimizing the output size while maintaining readability.
json-colorizer
The json-colorizer package is another tool for formatting JSON data with colors. It offers similar functionality to prettyjson but with different customization options and a focus on colorizing the JSON output.
prettyjson 
Package for formatting JSON data in a coloured YAML-style, perfect for CLI output
How to install
The easiest way is by installing it from the npm
repository:
$ npm install prettyjson
If you'd prefer to install the latest master version of prettyjson
, you can clone the GitHub source repository
and then install it using npm
:
$ git clone "https://github.com/rafeca/prettyjson.git"
$ npm install -g prettyjson/
How to use it
It's pretty easy to use it... you just have to include it in your script and call the render()
method:
var prettyjson = require('prettyjson');
var data = {
username: 'rafeca',
url: 'https://github.com/rafeca',
twitter_account: 'https://twitter.com/rafeca',
projects: ['prettyprint', 'connfu']
};
console.log(prettyjson.render(data));
And will output:
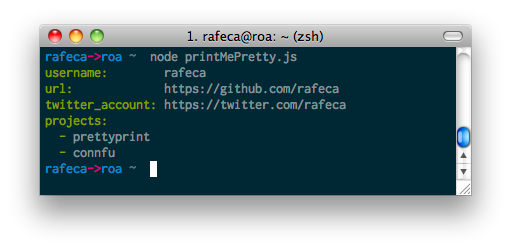
You can also configure the colors of the hash keys and array dashes
(using colors.js colors syntax):
var prettyjson = require('prettyjson');
var data = {
username: 'rafeca',
url: 'https://github.com/rafeca',
twitter_account: 'https://twitter.com/rafeca',
projects: ['prettyprint', 'connfu']
};
console.log(prettyjson.render(data, {
keysColor: 'rainbow',
dashColor: 'magenta'
}));
Will output something like:
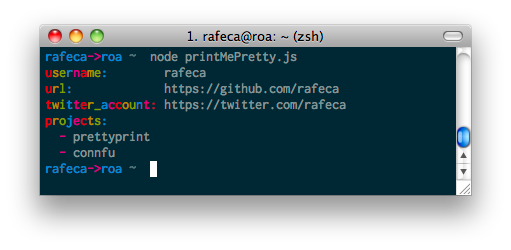
Annotated source
You can check the annotated source for more information about how it works
Running Tests
To run the test suite first invoke the following command within the repo, installing the development dependencies:
$ npm install --dev
then run the tests:
$ npm test
Contributors
- Rafael de Oleza <rafeca at gmail dot com>
- Francisco Perez Lopez <francis at tid dot es>
License
(The MIT License)
Copyright (c) 2011 Rafael de Oleza <rafeca@gmail.com>
Permission is hereby granted, free of charge, to any person obtaining
a copy of this software and associated documentation files (the
'Software'), to deal in the Software without restriction, including
without limitation the rights to use, copy, modify, merge, publish,
distribute, sublicense, and/or sell copies of the Software, and to
permit persons to whom the Software is furnished to do so, subject to
the following conditions:
The above copyright notice and this permission notice shall be
included in all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED 'AS IS', WITHOUT WARRANTY OF ANY KIND,
EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT.
IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY
CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT,
TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE
SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.