prettyjson

Package for formatting JSON data in a coloured YAML-style, perfect for CLI output.
How to install
Just install it via NPM:
$ npm install -g prettyjson
This will install prettyjson
globally, so it will be added automatically
to your PATH
.
Using it (from the CLI)
This package installs a command line interface to render JSON data in a more
convenient way. You can use the CLI in three different ways:
Decode a JSON file: If you want to see the contents of a JSON file, just pass
it as the first argument to the CLI:
$ prettyjson package.json
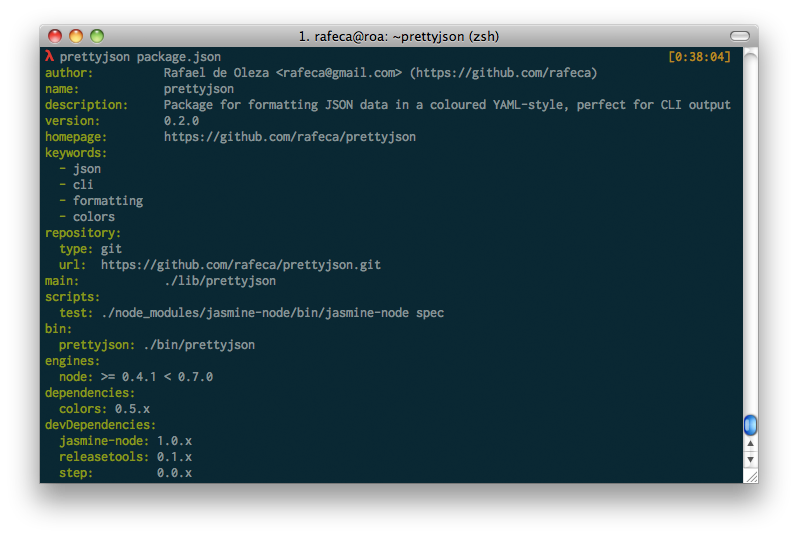
Decode the stdin: You can also pipe the result of a command (for example an
HTTP request) to the CLI to see the JSON result in a clearer way:
$ curl https://api.github.com/users/rafeca | prettyjson
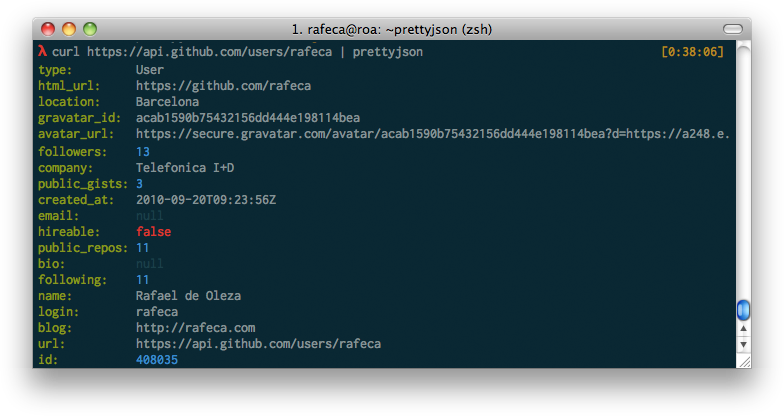
Decode random strings: if you call the CLI with no arguments, you'll get a
prompt where you can past JSON strings and they'll be automatically displayed in a clearer way:

Command line options
It's possible to customize the output through some command line options:
$ prettyjson --string=red --multiline_string=cyan --keys=blue --dash=yellow --number=green package.json
$ prettyjson --nocolor=1 package.json
$ prettyjson --indent=4 package.json
$ prettyjson --inline-arrays=1 package.json
Deprecation Notice: The old configuration through environment variables is
deprecated and it will be removed in the next major version (1.0.0).
Using it (from Node.js)
It's pretty easy to use it. You just have to include it in your script and call
the render()
method:
var prettyjson = require('prettyjson');
var data = {
username: 'rafeca',
url: 'https://github.com/rafeca',
twitter_account: 'https://twitter.com/rafeca',
projects: ['prettyprint', 'connfu']
};
var options = {
noColor: true
};
console.log(prettyjson.render(data, options));
And will output:
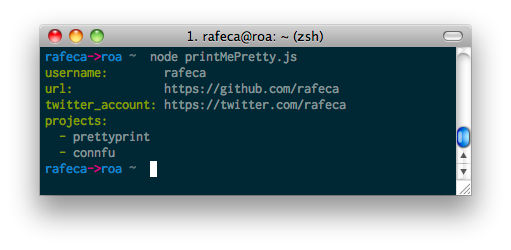
You can also configure the colors of the hash keys and array dashes
(using colors.js colors syntax):
var prettyjson = require('prettyjson');
var data = {
username: 'rafeca',
url: 'https://github.com/rafeca',
twitter_account: 'https://twitter.com/rafeca',
projects: ['prettyprint', 'connfu']
};
console.log(prettyjson.render(data, {
keysColor: 'rainbow',
dashColor: 'magenta',
stringColor: 'white',
multilineStringColor: 'cyan'
}));
Will output something like:

Running Tests
To run the test suite first invoke the following command within the repo,
installing the development dependencies:
$ npm install
then run the tests:
$ npm test
On windows, you can run the tests with:
C:\git\prettyjson> npm run-script testwin