What is primereact?
PrimeReact is a comprehensive collection of rich UI components for React. It provides a wide range of components that are designed to be highly customizable and easy to use, making it suitable for building modern, responsive web applications.
What are primereact's main functionalities?
DataTable
The DataTable component is used to display data in a tabular format. It supports features like sorting, filtering, and pagination.
import React from 'react';
import { DataTable } from 'primereact/datatable';
import { Column } from 'primereact/column';
const MyDataTable = () => {
const data = [
{ id: 1, name: 'John', age: 30 },
{ id: 2, name: 'Jane', age: 25 }
];
return (
<DataTable value={data}>
<Column field="id" header="ID" />
<Column field="name" header="Name" />
<Column field="age" header="Age" />
</DataTable>
);
};
export default MyDataTable;
Dialog
The Dialog component is used to display content in a modal window. It can be customized with headers, footers, and various other options.
import React, { useState } from 'react';
import { Dialog } from 'primereact/dialog';
import { Button } from 'primereact/button';
const MyDialog = () => {
const [visible, setVisible] = useState(false);
return (
<div>
<Button label="Show" icon="pi pi-external-link" onClick={() => setVisible(true)} />
<Dialog header="Header" visible={visible} style={{ width: '50vw' }} onHide={() => setVisible(false)}>
<p>Content</p>
</Dialog>
</div>
);
};
export default MyDialog;
Chart
The Chart component is used to create various types of charts, such as bar, line, and pie charts. It is built on top of the popular Chart.js library.
import React from 'react';
import { Chart } from 'primereact/chart';
const MyChart = () => {
const data = {
labels: ['January', 'February', 'March', 'April', 'May'],
datasets: [
{
label: 'My First dataset',
backgroundColor: '#42A5F5',
borderColor: '#1E88E5',
data: [65, 59, 80, 81, 56]
}
]
};
return <Chart type="bar" data={data} />;
};
export default MyChart;
Other packages similar to primereact
material-ui
Material-UI is a popular React UI framework that implements Google's Material Design. It offers a wide range of components and customization options. Compared to PrimeReact, Material-UI has a more modern design aesthetic and is widely adopted in the React community.
ant-design
Ant Design is a comprehensive UI framework for React that provides a large set of high-quality components. It is known for its robust design system and extensive documentation. Ant Design is similar to PrimeReact in terms of the breadth of components offered but has a different design philosophy.
semantic-ui-react
Semantic UI React is the official React integration for Semantic UI. It provides a set of components that are easy to use and customize. While it offers fewer components compared to PrimeReact, it focuses on simplicity and ease of use.

PrimeReact
PrimeReact is a rich set of open source UI Components for React.
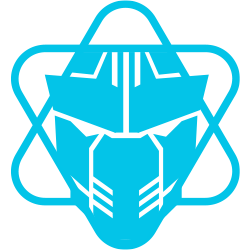
See PrimeReact homepage for live showcase and documentation.
Download
PrimeReact is available at npm, if you have an existing application run the following command to download it to your project.
npm install primereact --save
Import
import {Accordion,AccordionTab} from 'primereact/components/accordion/Accordion';
Dependencies
Majority of PrimeReact components (95%) are native and there are some exceptions having 3rd party dependencies such as Google Maps for GMap.
In addition, components require font-awesome for icons, classNames package to manage style classes and react-transition-group for animations.
dependencies: {
"react": "^16.0.0",
"react-dom": "^16.0.0",
"react-transition-group": "^2.2.1",
"font-awesome": "^4.7.0",
"classnames": "^2.2.5"
}
Styles
The css dependencies are as follows, note that you may change the theme with another one of your choice.
primereact/resources/themes/omega/theme.css
primereact/resources/primereact.min.css
primereact.min.css is a bundle that contains styles of all components, if you require a style of a specific component import the css from the folder of the component along with the common.css.
primereact/resources/themes/omega/theme.css
primereact/components/common/common.css
primereact/components/autocomplete/AutoComplete.css
If you are using a bundler such as webpack with a css loader you may also import them to your main application component, an example from create-react-app would be.
import 'primereact/resources/primereact.min.css';
import 'primereact/resources/themes/omega/theme.css';
import 'font-awesome/css/font-awesome.css';
QuickStart
An example application based on create-react-app is available at github.
TypeScript
Typescript
Typescript is fully supported as type definition files are provided in the npm package of PrimeReact. A sample typescript-primereact application is available as well at github.