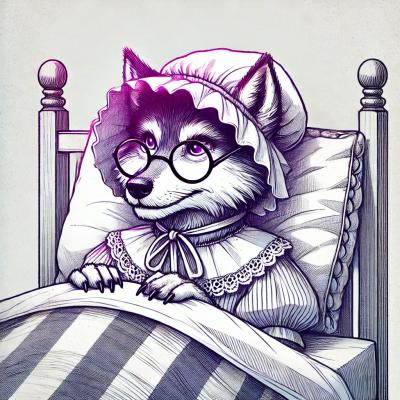
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
The printf npm package is a JavaScript implementation of the C-style printf function, which allows for formatted string output. It supports various format specifiers and can be used to format strings, numbers, and other data types in a structured way.
String Formatting
This feature allows you to format strings by embedding format specifiers within the string. In this example, '%s' is replaced by the string 'world'.
const printf = require('printf');
console.log(printf('Hello, %s!', 'world'));
Number Formatting
This feature allows you to format numbers. In this example, '%d' is replaced by the number 42.
const printf = require('printf');
console.log(printf('The number is: %d', 42));
Floating Point Formatting
This feature allows you to format floating-point numbers to a specified precision. In this example, '%.2f' formats the number 3.14159 to two decimal places.
const printf = require('printf');
console.log(printf('Pi is approximately %.2f', 3.14159));
Hexadecimal Formatting
This feature allows you to format numbers as hexadecimal. In this example, '%x' formats the number 255 as 'ff'.
const printf = require('printf');
console.log(printf('Hexadecimal: %x', 255));
The sprintf-js package provides similar functionality to printf, offering a JavaScript implementation of the sprintf function. It supports a wide range of format specifiers and is highly configurable. Compared to printf, sprintf-js offers more extensive documentation and additional features like named arguments.
The util package is a core Node.js module that includes the util.format function, which provides similar string formatting capabilities. While not as feature-rich as printf or sprintf-js, util.format is built into Node.js and does not require an additional dependency.
_ _ __ (_) | | / _| _ __ _ __ _ _ __ | |_| |_ | '_ \| '__| | '_ \| __| _| | |_) | | | | | | | |_| | | .__/|_| |_|_| |_|\__|_| | | |_|
A complete implementation of the printf
C functions family
for Node.JS, written in pure JavaScript.
The code is strongly inspired by the one available in the Dojo Toolkit.
Bonus! You get extra features, like the %O
converter (which inspect
s
the argument). See Extra Features below.
Via NPM:
$ npm install printf
Use it like you would in C (printf
/sprintf
):
var printf = require('printf');
var result = printf(format, args...);
It can also output the result for you, as fprintf
:
var printf = require('printf');
printf(write_stream, format, args...);
(space)assert.eql(' -42', printf('% 5d', -42));
+
(plus)assert.eql(' +42', printf('%+5d', 42));
0
(zero)assert.eql('00042', printf('%05d', 42));
-
(minus)assert.eql('42 ', printf('%-5d', 42));
assert.eql('42.90', printf('%.2f', 42.8952));
assert.eql('042.90', printf('%06.2f', 42.8952));
assert.eql('\x7f', printf('%c', 0x7f));
assert.eql('a', printf('%c', 'a'));
assert.eql('"', printf('%c', 34));
assert.eql('10%', printf('%d%%', 10));
assert.eql('+hello+', printf('+%s+', 'hello'));
assert.eql('$', printf('%c', 36));
The %O
converter will call util.inspect(...)
at the argument:
assert.eql("Debug: { hello: 'Node', repeat: false }",
printf('Debug: %O', {hello: 'Node', "repeat": false})
);
assert.eql("Test: { hello: 'Node' }",
printf('%2$s: %1$O', {"hello": 'Node'}, 'Test')
);
Important: it's a capital "O", not a zero!
Specifying a precision lets you control the depth up to which the object is formatted:
assert.eql("Debug: { depth0: { depth1_: 0, depth1: [Object] } }",
printf('Debug: %.1O', {depth0: {depth1: {depth2: {depth3: true}}, depth1_: 0}})
);
You can use the alternative form flag together with %O
to disable representation of non-enumerable properties (useful for arrays):
assert.eql("With non-enumerable properties: [ 1, 2, 3, 4, 5, [length]: 5 ]",
printf('With non-enumerable properties: %O', [1, 2, 3, 4, 5])
);
assert.eql("Without non-enumerable properties: [ 1, 2, 3, 4, 5 ]",
printf('Without non-enumerable properties: %#O', [1, 2, 3, 4, 5])
);
In addition to the old-fashioned n$
,
you can use hashes and property names!
assert.eql('Hot Pockets',
printf('%(temperature)s %(crevace)ss', {
temperature: 'Hot',
crevace: 'Pocket'
})
);
assert.eql('Hot Pockets',
printf('%2$s %1$ss', 'Pocket', 'Hot')
);
Lenght and precision can now be variable:
assert.eql(' foo', printf('%*s', 'foo', 4));
assert.eql(' 3.14', printf('%*.*f', 3.14159265, 10, 2));
assert.eql('000003.142', printf('%0*.*f', 3.14159265, 10, 3));
assert.eql('3.1416 ', printf('%-*.*f', 3.14159265, 10, 4));
Tests are written in CoffeeScript executed with Mocha. To use it, simple run npm install
, it will install
Mocha and its dependencies in your project's node_modules
directory followed by npm test
.
To run the tests:
npm install
npm test
The test suite is run online with Travis against the versions 0.9, 0.10 and 0.11 of Node.js.
Version 0.2.4
FAQs
Full implementation of the `printf` family in pure JS.
We found that printf demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.