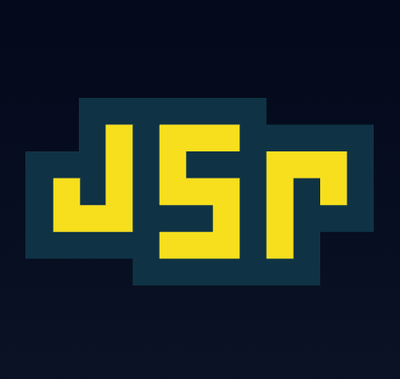
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Prisma is an open-source database toolkit. It includes a JavaScript/TypeScript ORM for Node.js, migrations and a modern GUI to view and edit the data in your database. You can use Prisma in new projects or add it to an existing one.
Prisma is an open-source database toolkit that includes an ORM (Object-Relational Mapper), migrations, and a query builder for Node.js and TypeScript. It simplifies database access, ensures type safety, and can be used to build GraphQL and REST APIs.
ORM (Object-Relational Mapping)
Prisma's ORM allows you to interact with your database through an object-oriented model. The code sample demonstrates how to fetch all users from the database using Prisma's ORM.
const { PrismaClient } = require('@prisma/client');
const prisma = new PrismaClient();
async function main() {
const allUsers = await prisma.user.findMany();
console.log(allUsers);
}
main()
.catch(e => {
throw e
})
.finally(async () => {
await prisma.$disconnect()
});
Migrations
Prisma Migrate generates and runs SQL migrations based on your Prisma schema. The code sample shows how to create and apply a new migration called 'init'.
npx prisma migrate dev --name init
Type Safety
Prisma ensures type safety by generating TypeScript types based on your database schema. The code sample demonstrates fetching a user with a specific ID and the result is typed as 'User'.
const user: User = await prisma.user.findUnique({ where: { id: 1 } });
Data Modeling
Prisma uses a schema file to model your database. The code sample shows a Prisma schema definition for a User model with an auto-incrementing ID, name, and unique email.
model User {
id Int @id @default(autoincrement())
name String
email String @unique
}
Query Builder
Prisma's query builder allows you to construct complex queries with ease. The code sample demonstrates ordering users by their name in ascending order.
const sortedUsers = await prisma.user.findMany({
orderBy: {
name: 'asc',
},
});
Sequelize is a promise-based Node.js ORM for Postgres, MySQL, MariaDB, SQLite, and Microsoft SQL Server. It features solid transaction support, relations, eager and lazy loading, read replication, and more. Compared to Prisma, Sequelize has been around for longer and has a more traditional API, but it might not offer the same level of type safety and simplicity in schema management.
TypeORM is an ORM that can run in Node.js, Browser, Cordova, PhoneGap, Ionic, React Native, NativeScript, and Electron platforms and can be used with TypeScript and JavaScript. TypeORM is highly influenced by other ORMs, such as Hibernate, Doctrine, and Entity Framework. It provides a similar level of type safety as Prisma but with a different approach to defining models and running migrations.
Knex.js is a SQL query builder for Postgres, MSSQL, MySQL, MariaDB, SQLite3, Oracle, and Amazon Redshift, designed to be flexible, portable, and fun to use. It does not include an ORM by default, but it can be paired with objection.js for ORM functionality. Knex is more of a query builder than a full-fledged ORM and lacks the type safety and model generation features of Prisma.
Prisma is a next-generation ORM that consists of these tools:
Prisma Client can be used in any Node.js or TypeScript backend application (including serverless applications and microservices). This can be a REST API, a GraphQL API a gRPC API, or anything else that needs a database.
The fastest way to get started with Prisma is by following the Quickstart (5 min).
The Quickstart is based on a preconfigured SQLite database. You can also get started with your own database (PostgreSQL and MySQL) by following one of these guides:
Prisma has a large and supportive community of enthusiastic application developers. You can join us on Discord and here on GitHub.
If you have a security issue to report, please contact us at security@prisma.io.
You can ask questions and initiate discussions about Prisma-related topics in the prisma
repository on GitHub.
If you see an error message or run into an issue, please make sure to create a bug report! You can find best practices for creating bug reports (like including additional debugging output) in the docs.
If Prisma currently doesn't have a certain feature, be sure to check out the roadmap to see if this is already planned for the future.
If the feature on the roadmap is linked to a GitHub issue, please make sure to leave a +1 on the issue and ideally a comment with your thoughts about the feature!
Refer to our contribution guidelines and Code of Conduct for contributors.
FAQs
Prisma is an open-source database toolkit. It includes a JavaScript/TypeScript ORM for Node.js, migrations and a modern GUI to view and edit the data in your database. You can use Prisma in new projects or add it to an existing one.
The npm package prisma receives a total of 1,982,649 weekly downloads. As such, prisma popularity was classified as popular.
We found that prisma demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 6 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.