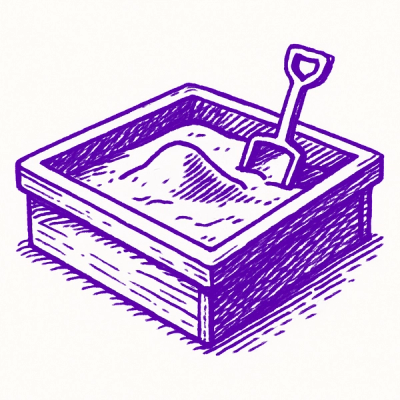
Research
/Security News
Critical Vulnerability in NestJS Devtools: Localhost RCE via Sandbox Escape
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
probe-image-size
Advanced tools
Get image size without full download (JPG, GIF, PNG, WebP, BMP, TIFF, PSD)
The probe-image-size npm package is used to quickly and efficiently determine the dimensions and type of an image by reading only a small portion of the file. This can be particularly useful for applications that need to handle images but want to avoid loading entire files into memory.
Get Image Dimensions
This feature allows you to get the dimensions of an image by reading a stream of the image file. The code sample demonstrates how to use the probe-image-size package to read an image file from the filesystem and log its dimensions.
const probe = require('probe-image-size');
const fs = require('fs');
async function getImageDimensions(filePath) {
const stream = fs.createReadStream(filePath);
const result = await probe(stream);
console.log(result);
}
getImageDimensions('path/to/image.jpg');
Get Image Type
This feature allows you to determine the type of an image (e.g., JPEG, PNG, GIF) by reading a stream of the image file. The code sample demonstrates how to use the probe-image-size package to read an image file from the filesystem and log its type.
const probe = require('probe-image-size');
const fs = require('fs');
async function getImageType(filePath) {
const stream = fs.createReadStream(filePath);
const result = await probe(stream);
console.log(result.type);
}
getImageType('path/to/image.jpg');
Get Image Dimensions from URL
This feature allows you to get the dimensions of an image from a URL by fetching the image and reading its stream. The code sample demonstrates how to use the probe-image-size package to fetch an image from a URL and log its dimensions.
const probe = require('probe-image-size');
const fetch = require('node-fetch');
async function getImageDimensionsFromURL(url) {
const response = await fetch(url);
const result = await probe(response.body);
console.log(result);
}
getImageDimensionsFromURL('https://example.com/image.jpg');
The image-size package is another popular library for determining the dimensions of an image. Unlike probe-image-size, image-size reads the entire file into memory, which can be less efficient for large images. However, it supports a wide range of image formats and is straightforward to use.
Sharp is a high-performance image processing library that can also be used to get image metadata, including dimensions. While it offers more extensive image manipulation capabilities compared to probe-image-size, it is also more complex and has a larger footprint.
Jimp is a JavaScript image processing library that can read image dimensions and perform various image manipulations. It is written entirely in JavaScript and does not require native dependencies, making it easier to install and use in some environments. However, it may not be as performant as probe-image-size for simply probing image dimensions.
Get image size without full download. Supported image types: JPG, GIF, PNG, WebP, BMP, TIFF, SVG, PSD, ICO, AVIF, HEIC, HEIF.
Key features:
npm install probe-image-size
const probe = require('probe-image-size');
// Get by URL
let result = await probe('http://example.com/image.jpg');
console.log(result); // =>
/*
{
width: xx,
height: yy,
type: 'jpg',
mime: 'image/jpeg',
wUnits: 'px',
hUnits: 'px',
url: 'http://example.com/image.jpg'
}
*/
// By URL with options
let result = await probe('http://example.com/image.jpg', { rejectUnauthorized: false });
console.log(result);
// From the stream
let result = await probe(require('fs').createReadStream('image.jpg'));
console.log(result);
// From a Buffer (sync)
let data = require('fs').readFileSync('image.jpg');
console.log(probe.sync(data));
Note:
stream.js
/ http.js
/ sync.js
directly.http.js
dependencies, you can create your own wrapper
for stream.js
.src
can be of this types:
options
- HTTP only. See needle
documentation, and customized defaults.keepOpen
(Boolean) - stream only. Keep stream open after parser finishes
(input stream will be closed by default)result
(Promise) contains:
{
width: XX,
height: YY,
length: ZZ, // byte length of the file (if available, HTTP only)
type: ..., // image 'type' (usual file name extention)
mime: ..., // mime type
wUnits: 'px', // width units type ('px' by default, can be different for SVG)
hUnits: 'px', // height units type ('px' by default, can be different for SVG)
url: ..., // HTTP only, last url for the image in chain of redirects
// (if no redirects, same as src)
// optional, image orientation (from Exif), number from 1 to 8;
// you may wish to swap width and height if orientation is >= 5
orientation: X,
// optional, full list of sizes for ICO (always) and AVIF (if multiple images)
variants: [ { width, height }, ... ] | undefined
}
Width and height in the output object represent image size before any transformations (orientation, cropping) are applied. Orientation is returned separately, which you may wish to apply afterwards depending on browser support (browsers only support JPEG orientation for now). See known issues for details.
Returned errors can be extended with 2 fields:
code
- equals to ECONTENT
if the library failed to parse the file;status
- equals to a HTTP status code if it receives a non-200 response.Sync version can eat arrays, typed arrays and buffers. On success it returns the same result as async version. On fail it returns null.
Note. Formats like JPEG & TIFF can store size anywhere (far from the head). That usually does not happens, but if you need guarantees - always provide full file content to sync methods. We strongly recommend to use async version as memory-friendly.
You can support this project via Tidelift subscription.
[7.2.3] - 2022-01-27
Error
extend in FF, #71.FAQs
Get image size without full download (JPG, GIF, PNG, WebP, BMP, TIFF, PSD)
The npm package probe-image-size receives a total of 687,888 weekly downloads. As such, probe-image-size popularity was classified as popular.
We found that probe-image-size demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
Product
Customize license detection with Socket’s new license overlays: gain control, reduce noise, and handle edge cases with precision.
Product
Socket now supports Rust and Cargo, offering package search for all users and experimental SBOM generation for enterprise projects.