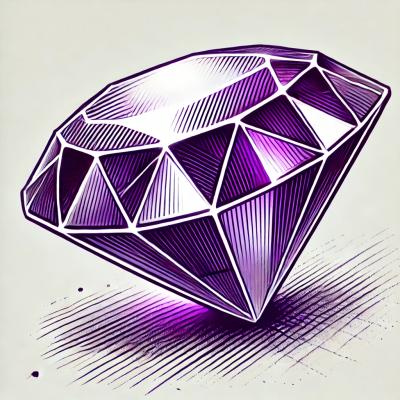
Security News
Highlights from the 2024 Rails Community Survey
A record 2,709 developers participated in the 2024 Ruby on Rails Community Survey, revealing key tools, practices, and trends shaping the Rails ecosystem.
promise-toolbox
Advanced tools
Essential utils for promises.
Features:
Installation of the npm package:
> npm install --save promise-toolbox
Make your async functions cancellable.
import { cancellable } from 'promise-toolbox'
const asyncFunction = cancellable(async function (cancellation, a, b) {
cancellation.catch(() => {
// do stuff regarding the cancellation request.
})
// do other stuff.
})
const promise = asyncFunction('foo', 'bar')
promise.cancel()
If the function is a method of a class or an object, you can use
cancellable
as a decorator:
class MyClass {
@cancellable
async asyncMethod (cancellation, a, b) {
cancellation.catch(() => {
// do stuff regarding the cancellation request.
})
// do other stuff.
}
}
Discouraged but sometimes necessary way to create a promise.
import { defer } from 'promise-toolbox'
const { promise, resolve } = defer()
promise.then(value => {
console.log(value)
})
resolve(3)
Easiest and most efficient way to promisify a function call.
import { fromCallback } from 'promise-toolbox'
fromCallback(cb => fs.readFile('foo.txt', cb))
.then(content => {
console.log(content)
})
import { isPromise } from 'promise-toolbox'
if (isPromise(foo())) {
console.log('foo() returns a promise')
}
Easiest and most efficient way to wait for a fixed amount of promises.
import { join } from 'promise-toolbox'
join(getPictures(), getComments(), getTweets(), (pictures, comments, tweets) => {
console.log(`in total: ${pictures.length + comments.length + tweets.length}`)
})
This function can be used as if they were methods, i.e. by passing the promise (or promises) as the context.
This is extremely easy using ES2016's bind syntax.
const promises = [
Promise.resolve('foo'),
Promise.resolve('bar')
]
promises::all().then(values => {
console.log(values)
})
// → [ 'foo', 'bar' ]
If you are still an older version of ECMAScript, fear not: simply pass
the promise (or promises) as the first argument of the .call()
method:
var promises = [
Promise.resolve('foo'),
Promise.resolve('bar')
]
all.call(promises).then(function (values) {
console.log(values)
})
// → [ 'foo', 'bar' ]
Waits for all promises of a collection to be resolved.
Contrary to the standard
Promise.all()
, this function works also with objects.
import { all } from 'promise-toolbox'
console.log([
Promise.resolve('foo'),
Promise.resolve('bar')
]::all())
// → ['foo', 'bar']
console.log({
foo: Promise.resolve('foo'),
bar: Promise.resolve('bar')
}::all())
// → {
// foo: 'foo',
// bar: 'bar'
// }
Register a node-style callback on this promise.
import { asCallback } from 'promise-toolbox'
// This function can be used either with node-style callbacks or with
// promises.
function getDataFor (input, callback) {
return dataFromDataBase(input)::asCallback(callback)
}
Delays the resolution of a promise by
ms
milliseconds.Note: the rejection is not delayed.
console.log(await Promise.resolve('500ms passed')::delay(500))
// → 500 ms passed
Also works with a value:
console.log(await delay.call('500ms passed', 500))
// → 500 ms passed
Iterates in order over a collection of promises waiting for each of them to be resolved.
[
Promise.resolve('foo'),
Promise.resolve('bar'),
]::forEach(value => {
console.log(value)
})
// →
// foo
// bar
Execute a handler regardless of the promise fate. Similar to the
finally
block in synchronous codes.The resolution value or rejection reason of the initial promise if forwarded.
import { lastly } from 'promise-toolbox'
function ajaxGetAsync (url) {
return new Promise((resolve, reject) => {
const xhr = new XMLHttpRequest
xhr.addEventListener('error', reject)
xhr.addEventListener('load', resolve)
xhr.open('GET', url)
xhr.send(null)
})::lastly(() => {
$('#ajax-loader-animation').hide()
})
}
Creates async functions taking node-style callbacks, create new ones returning promises.
import fs from 'fs'
import { promisify, promisifyAll } from 'promise-toolbox'
// Promisify a single function.
//
// If possible, the function name is kept and the new length is set.
const readFile = fs.readFile::promisify()
// Or all functions (own or inherited) exposed on a object.
const fsPromise = fs::promisifyAll()
readFile(__filename).then(content => console.log(content))
fsPromise.readFileAsync(__filename).then(content => console.log(content))
Returns a promise which resolves to an objects which reflects the resolution of this promise.
import { reflect } from 'promise-toolbox'
const inspection = await promise::reflect()
if (inspection.isFulfilled()) {
console.log(inspection.value())
} else {
console.error(inspection.reason())
}
Waits for
count
promises in a collection to be resolved.
import { some } from 'promise-toolbox'
const [ first, seconds ] = await [
ping('ns1.example.org'),
ping('ns2.example.org'),
ping('ns3.example.org'),
ping('ns4.example.org')
]::some(2)
Automatically rejects a promise if it is still pending after
ms
milliseconds.
await doLongOperation()::timeout(100)
> npm install
The sources files are watched and automatically recompiled on changes.
> npm run dev
> npm run test-dev
Contributions are very welcomed, either on the documentation or on the code.
You may:
ISC © Julien Fontanet
FAQs
Essential utils for promises
The npm package promise-toolbox receives a total of 65,834 weekly downloads. As such, promise-toolbox popularity was classified as popular.
We found that promise-toolbox demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
A record 2,709 developers participated in the 2024 Ruby on Rails Community Survey, revealing key tools, practices, and trends shaping the Rails ecosystem.
Security News
In 2023, data breaches surged 78% from zero-day and supply chain attacks, but developers are still buried under alerts that are unable to prevent these threats.
Security News
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.