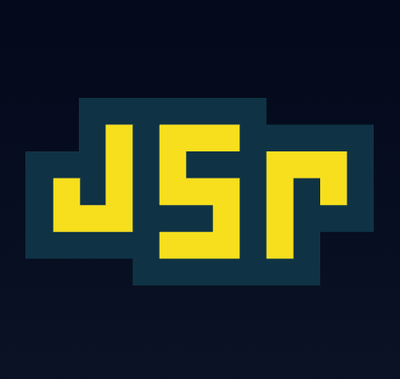
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
promise-ws
Advanced tools
A promise based WebSocket implementation for Node.js. Built on top of ws
import { Server, Client } from 'promise-ws';
(async function main() {
const port = 3000;
const server = await Server.create({ port });
server.reply('say', async (data) => {
console.log('data'); /* 'hello' */
return 'world';
});
const url = `ws://127.0.0.1:${port}`;
await Client.autoReconnect(url, async (client) => {
const response = await client.request('say', 'hello');
console.log(response); /* 'world' */
});
}());
$ npm install promise-ws
options
<Object>: All options will be passed to WebSocket.Server(opsions), except for clientTracking
, which will always be true
.server
<Promise<Server>>Create a WebSocket server
Server extends EventEmitter, which forwards these events from WebSocket.Server:
name
<String>: The name of the eventresponse
<Function>: The callback function. Should return a promiseAdd a reply function. Will be called when client calls request()
. The response function arguments are the same with client.request(name, ...arguments)
arguments. The returning value in response would be reply to the issuer client request function.
Alias for server#onReply(name, response)
Alias for server#onReply(name, response)
name
<String>: The name of the eventresponse
<Function>: The callback functionRemove a reply function.
name
<String>: The name of the eventGet reply function count by name.
name
<String>: The name of the event...args
<Any>: The request argumentsresponseArray
<Promise[<Any>]/>: Response array by clients repliedRequest to all clients and wait for reply.
wss
<WebSocketServer>Get <WebSocket.Server> instance.
Stops the server.
The connected clients.
address
<String>: The address/URL to which to connectoptions
<Object>onClose
<Function>: The callback function when client closedclient
<Promise<Client>>Create a WebSocket client.
import { Client } from 'promise-ws';
(async function main() {
const client = await Client.create('ws://127.0.0.1:3000', {
onClose() { console.error('server closed'); }
});
/* do something... */
}());
address
<String>: The address/URL to which to connectwaitUntil
<Function>: The main function to handle client. Should return a promiseCreate a WebSocket client and pass to the first argumet of waitUntil
function.
The waitUntil
function will keep running until one of these reasons:
waitUntil
function returns a value. The value will be returned to Client.connect()
as a promisewaitUntil
function throws an error. This will throw a rejectionimport { Client } from 'promise-ws';
(async function main() {
try {
const url = 'ws://127.0.0.1:3000';
const res = await Client.connect(url, async (client) => {
/* do something... */
return 'chris';
});
console.log('res:', res); /* res: chris */
}
catch (err) {
if (err.message === 'CLOSE') { console.error('server closed'); }
else { console.error(err); }
}
}());
address
<String>: The address/URL to which to connectwaitUntil
<Function>: The main function to handle client. Should return a promisedelay
<Number>: Delay time before reconnect in ms. Defaults to 1000
Like Client.connect()
, but if server closed, it will never throw error, and it will try to reconnect to the server after delay.
import { Client } from 'promise-ws';
(async function main() {
try {
const url = 'ws://127.0.0.1:3000';
const res = await Client.autoReconnect(url, async (client) => {
/* do something... */
return 'chris';
});
console.log('res:', res); /* res: chris */
}
catch (err) {
console.error(err);
}
}());
Client extends EventEmitter, which forwards these events from WebSocket.Client:
name
<String>: The name of the eventresponse
<Function>: The callback function. Should return a promiseAdd a reply function. Will be called when server calls request()
. The response function arguments are the same with server.request(name, ...arguments)
arguments. The returning value in response would be reply to the server request function.
Alias for client#onReply(name, response)
Alias for client#onReply(name, response)
name
<String>: The name of the eventresponse
<Function>: The callback functionRemove a reply function.
name
<String>: The name of the eventGet reply function count by name.
name
<String>: The name of the event...args
<Any>: The request argumentsresponseData
<Promise<Any>/>: Response data by server repliedRequest to all clients and wait for reply.
ws
<WebSocketClient>Get <WebSocket.Client> instance.
Stops the client.
MIT
FAQs
A Promise-Based WebSocket implementation for Node.js
The npm package promise-ws receives a total of 6,606 weekly downloads. As such, promise-ws popularity was classified as popular.
We found that promise-ws demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.