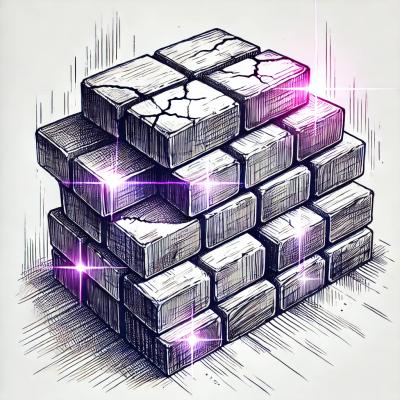
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
promisechain
Advanced tools
Promise provides chain mechanism with then
function.
But its writing is a little annoying.
This library enable more lazy way.
var p = Promise(function(resolve, reject){
setTimeout(function(){
resolve([1,2,3,4,5]);
}, 10);
})
Promisechain.chainable(p)
.map(function(v){
return v*2;
})
.reduce(function(acc, v) {
return acc + v;
}, 0)
.filter(function(v) {
return v > 20;
})
.recovery(99)
.pipe(function(v) {
return {result :v};
})
.then(function(v){
console.log(v);
});
// => {result:30}
Use bower.
bower isntall promisechain
<script src="./bower_components/promisechain/dist/promisechain_bundle.js"></script>
Promisechain
will be installed to global.
Or use require
style with browserify.
Use npm.
npm isntall promisechain
var chainable = require("promisechain").chainable;
Promisechain.chainable(p Promise)
Add chain-able methods to p
.
All chain-able methods return chain-able promise.
filter(predicate Function)
Filter value with provided predicate.
If predicate is not function
, ===
operator will be used as predicate.
chainable(p)
.filter(function(v) {
return v > 20;
});
.then(
function(v){console.log("This block will be called when v > 20");},
function(){console.log("This block will be called when v <= 20");}
);
chainable(p)
.filter("HELLO");
.then(
function(v){console.log("This block will be called when v === 'HELLO'");},
function(){console.log("This block will be called when v !== 'HELLO'");}
);
Notice
Unlike underscore
's' or lodash
's filter
, this filter
method does not iterate value.
Just apply predicator to value itself.
If you want filter element of array, use pipe
instead.
chainable(Promise.resolve([1,2,3,4,5]))
.pipe(function(arr){
return _.filter(arr, function(v){return v > 2;});
})
.then(function(){
console.log(v); // => [3,4,5]
});
pipe(func Function)
Pipe values to next step.
function double(v){ return v*2;}
chainable(Promise.resolve(2))
.pipe(double);
.pipe(double);
.then(
function(v){console.log(v);} // => 8
);
recovery(value Any)
Recovery promise chain when before steps are rejected.
chainable(Promise.resolve(2))
.filter(function(){return v > 10;});
.recovery(100);
.then(
function(v){console.log(v);} // => 100,
function(){console.log("This block will never be called");}
);
These methods are alias to underscore
's basic collection utility.
Apply underscore
's method with arguments, then return chain-able promise.
What it is not listed here, You can use with pipe
method.
Noteice
If invalid parameter is passed to underscore
's method,
Unless it throw Error
, promise will not be rejected.
Generally it will return empty array []
. So promise chain will not be rejected but resolved with []
;
map
An alias of underscore
's' map
.
function double(v){ return v*2;}
chainable(Promise.resolve([1,2,3,4,5]))
.map(double);
.then(
function(v){console.log(v);} // => [2,4,6,8,10],
);
reduce
An alias of underscore
's' reduce
.
function sum(acc, v){ return v + acc;}
chainable(Promise.resolve([1,2,3,4,5]))
.reduce(sum, 0)
.then(
function(v){console.log(v);} // => 15,
);
first
, head
and take
An alias of underscore
's' first
.
chainable(Promise.resolve([1,2,3,4,5]))
.first()
.then(
function(v){console.log(v);} // => 1,
);
initial
An alias of underscore
's' initial
.
chainable(Promise.resolve([1,2,3,4,5]))
.initial()
.then(
function(v){console.log(v);} // => [1,2,3,4],
);
last
An alias of underscore
's' last
.
chainable(Promise.resolve([1,2,3,4,5]))
.last()
.then(
function(v){console.log(v);} // => 5,
);
rest
, tail
, drop
An alias of underscore
's' rest
.
chainable(Promise.resolve([1,2,3,4,5]))
.rest()
.then(
function(v){console.log(v);} // => [2,3,4,5]
);
keys
An alias of underscore
's' keys
.
chainable(Promise.resolve({
"key1": "val1",
"key2": "val2"
}))
.keys()
.then(
function(v){console.log(v);} // => ["key1", "key2"]
);
values
An alias of underscore
's' values
.
chainable(Promise.resolve({
"key1": "val1",
"key2": "val2"
}))
.values()
.then(
function(v){console.log(v);} // => ["val1", "val2"]
);
values
An alias of underscore
's' values
.
chainable(Promise.resolve({
"key1": "val1",
"key2": "val2"
}))
.pairs()
.then(
function(v){console.log(v);} // => [["key1","val1"], ["key2","val2"]
);
Install Node.js and NPM.
git clone git://github.com/georegeosddev/promisechain.git
cd promisechain
npm install
npm run-script build
MIT
FAQs
Chainable promise
The npm package promisechain receives a total of 1 weekly downloads. As such, promisechain popularity was classified as not popular.
We found that promisechain demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.