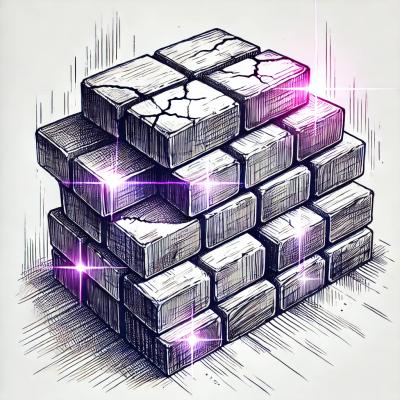
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
promised-models
Advanced tools
$npm install --save promised-models
var Model = require('promises-models'),
FashionModel = new Model.inherit({
attributes: {
name: Model.attributeTypes.String
}
}),
model = new FashionModel({
name: 'Kate'
});
model.get('name'); // 'Kate'
Model.inherit(properties, [classPorperties])
Creates you own model class by extending Model
. You can define attributes, instance/class method and properties. Inheritance is built over inherit.
var CountedModels = Model.inherit({
__constructor: function () {
this.__base.apply(this, arguments); //super
this.attributes.index.set(this.__self._count); //static properties
this.__self._count ++;
},
getIndex: function () {
return this.get('index');
}
}, {
_count: 0,
getCount: function () {
return this._count;
}
});
Model.attributeTypes
Namespace for predefined types of attributes. Supported types:
String
Number
Boolean
List
— for storing arraysModel
— for nested modelsModelsList
— for nested collectionsObject
— serializable objectsYou can extend default attribute types or create your own
var DateAttribute = Model.attributeTypes.Number.inherit({
//..
}),
FashionModel = Model.inherit({
attributes: {
name: Model.attributeTypes.String,
birthDate: DateAttribute
}
});
Note: models.attributes
will be replaced in constructor with attribute instances.
var model = new FashionModel();
model.attributes.birthDate instanceof DateAttribute; //true
model.set(attributeName, value)
Set current value of attribute.
var model = new FashionModel();
model.set('name', 'Kate');
model.attributes.name.set('Kate');
model.set({
name: 'Kate',
birthDate: new Date(1974, 1, 16)
});
Note: setting null
is equivalent to call .unset()
model.get(attributeName)
Get current value of attribute.
var model = new FashionModel({
name: 'Kate',
birthDate: new Date(1974, 1, 16)
})
model.get('name'); //Kate
model.attributes.name.get(); //Kate
model.get('some'); //throws error as unknown attribute
model.toJSON()
Return shallow copy of model data.
Note: You can create internal attributes, which wouldn't be included to returned object.
var FashionModel = new Model.inherit({
attributes: {
name: Model.attributeTypes.String.inherit({
internal: true;
}),
sename: Model.attributeTypes.String.inherit({
internal: true;
}),
fullName: Model.attributeTypes.String
}
}),
model = new FashionModel({
name: 'Kate',
sename: 'Moss',
fullName: 'Kate Moss'
});
model.toJSON(); // {fullName: 'Kate Moss'}
model.get('name'); // Kate
model.isChanged([branch])
Has model changed since init or last commit/save/fetch.
var FashionModel = Model.inherit({
attributes: {
name: Model.attributeTypes.String,
weight: Model.attributeTypes.Number.inherit({
default: 50
})
}
}),
model = new FashionModel({
name: 'Kate',
weight: 55
});
model.isChanged(); //false
model.set('weight', 56);
model.isChanged(); //true
model.commit([branch])
Cache current model state
var model = new FashionModel();
model.set({
name: 'Kate',
weight: 55
});
model.isChanged();//true
model.commit();
model.isChanged();//false
model.revert([branch])
Revert model state to last cashed one
var model = new FashionModel({
name: 'Kate',
weight: 55
});
model.set('weight', 56);
model.revert();
model.get('weight'); //55
model.isChanged(); //false
Note: You can create your own cache by passing branch param.
var RENDERED = 'RENDERED';
model.on('change', function () {
if (model.isChanged(RENDERED)) {
View.render();
model.commit(RENDERED);
}
});
model.on([attributes], events, cb, [ctx])
Add event handler for one or multiple model events.
List of events:
change
– some of attributes have been changedchange:attributeName
– attributeName
have been changeddestruct
– model was destructedcalculate
– async calculations startedmodel.on('change', this.changeHandler, this)
.on('change:weight change:name', this.changeHandler, this);
model.un([attributes], events, cb, [ctx])
Unsubscribe event handler from events.
//subscribe
model.on('weight name', 'change', this.changeHandler, this);
//unsubscribe
model.un('change:weight change:name', this.changeHandler, this);
model.destruct()
Remove all events handlers from model and removes model from collections
model.isSet(attributeName)
Returns true
if attribute was set via constructor or set
var model = new FashionModel();
model.isSet('name'); //false
model.set('name', 'Kate');
model.isSet('name'); //true
model.unset(attributeName)
Set attribute to default value and model.isSet() === 'false'
var model = new FashionModel();
model.set('name', 'Kate');
model.unset('name');
model.isSet('name'); //false
model.get('name'); //empty string (default value)
model.validate()
Validate model attributes.
var FashionModel = Model.inherit({
attributes: {
name: Model.attributeTypes.String.inherit({
validate: function () {
return $.get('/validateName', {
name: this.get()
}).then(function () {
return true; //valid
}, function () {
return false; //invalid
});
}
})
}
}),
model = new FashionModel();
model.validate().fail(function (err) {
if (err instanceof Model.ValidationError) {
console.log('Invalid attributes:' + err.attributes.join());
} else {
return err;
}
}).done();
model.ready()
Fulfils when all calculations over model finished.
var FashionModel = Model.inherit({
attributes: {
name: Model.attributeTypes.String,
ratingIndex: Model.attributeTypes.Number.inherit({
calculate: function () {
return $.get('/rating', {
annualFee: this.model.get('annualFee')
});
}
}),
annualFee: Model.attributeTypes.Number
}
}),
model = new FashionModel();
model.set('annualFee', 1000000);
model.ready().then(function () {
model.get('ratingIndex');
}).done();
model.fetch()
Fetch data associated with model from storage.
var FashionModel = Model.inherit({
attributes: {
name: Model.attributeTypes.String
},
storage: Model.Storage.inherit({
find: function (model) {
return $.get('/models', {
id: model.id
});
}
})
}),
model = new FashionModel(id);
model.fetch().then(function () {
model.get('name');
}).done();
model.save()
var FashionModel = Model.inherit({
attributes: {
name: Model.attributeTypes.String,
weight: Model.attributeTypes.Number
},
storage: Model.Storage.inherit({
insert: function (model) {
return $.post('/models', model.toJSON()).then(function (result) {
return result.id;
});
},
update: function (model) {
return $.put('/models', model.toJSON());
}
})
}),
model = new FashionModel();
model.set({
name: 'Kate',
weight: 55
});
model.save().then(function () { //create
model.id; //storage id
model.set('weight', 56);
return model.save(); //update
}).done()
model.remove()
Removes model from storage.
model.isNew()
model.isReady()
model.trigger(event)
model.calculate()
model.CHANGE_BRANCH
model.CALCULATIONS_BRANCH
These methods provided for advanced model extending. Consult source for details.
Model.Storage
Abstract class for model storage
var FashionModel = Model.inherit({
attributes: {
//..
},
storage: Model.Storage.inherit({
//..
})
});
Model.storage
Storage class
var SuperModel = FashionModel.inherit({
storage: FashionModel.storage.inherit({ //extend storage from FashionModel
//..
})
});
Model.Attribute
Base class for model attribute
var CustomAttribute = Model.attribute.inherit({
//..
})
Model.attributes
Model class attributes
var SuperModel = FashionModel.inherit({
attributes: {
name: FashionModel.attributes.name,
weight: FashionModel.attributes.weight.inherit({
default: 50
})
}
});
Array like object returned for fields types List
and ModelsList
var Podium = Model.inherit({
attributes: {
models: Model.attributeTypes.ModelsList(FashionModel)
}
}),
podium = new Podium(data),
list = podium.get('models'), //instanceof List
model = list.get(0); //instanceof Model
List inerits Array mutating methods: pop
, push
, reverse
, shift
, sort
, splice
, unshift
podium.get('models').push(new FashionModel());
list.get(index)
Get list item by index
podium.get('models').get(0);// instanceof Model
list.length()
Returns length of list
list.toArray()
Returns shallow copy of Array, wich stores List items
podium.get('models').forEach(function (model) {
model; // instanceof Model
});
Model.ValidationError
Error class for validation fail report
$ npm test
FAQs
promise based, typed attributes, nested models and collections
The npm package promised-models receives a total of 1 weekly downloads. As such, promised-models popularity was classified as not popular.
We found that promised-models demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.