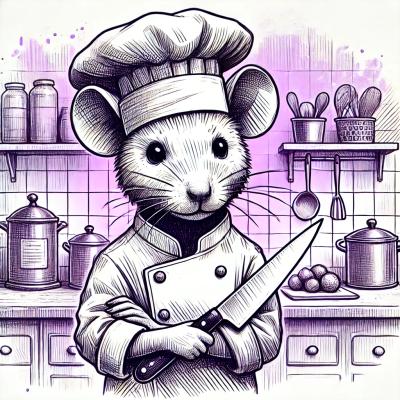
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Powerful promise utilities for any JS environment - because who uses callbacks in 2018?
npm i promiso
Promise
defined globally (try
es6-promise polyfill)const promiso = require('promiso');
// Double a number after 1 second.
const slowDouble = (number) => {
return new Promise((resolve) => {
setTimeout(() => resolve(number * 2), 1000);
});
};
const numbers = [1, 2, 3, 4];
promiso.mapLimit(numbers, 2, slowDouble)
.then((doubled) => {
// 4 items limited to 2 concurrent executions, so this should fire after about 2 seconds.
console.log(doubled); // [2, 4, 6, 8]
});
async
/await
const promiso = require('promiso');
// Helper function for async/await.
const sleep = (time) => new Promise((resolve) => setTimeout(resolve, time));
// Triple a number after 1 second.
const slowTriple = async (number) => {
await sleep(1000);
return number * 3;
};
const numbers = [1, 2, 3, 4, 5, 6];
const main = async () => {
const tripled = await promiso.mapLimit(numbers, 3, slowTriple);
// 6 items limited to 3 concurrent executions, so this should complete after about 2 seconds.
console.log(tripled); // [3, 6, 9, 12, 15, 18]
};
main();
Promiso is transpiled from TypeScript with type definitions, so it should work out of the box with TypeScript :-)
Actual docs coming soon :-)
See the documentation for the original async library, but Promiso offers these improvements:
The following functions are available (with support planned for every function available in async):
FAQs
Powerful promise utilities for any JS environment
We found that promiso demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.