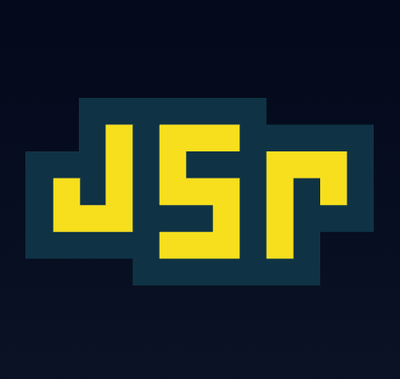
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
prosemirror-markdown
Advanced tools
The prosemirror-markdown package provides tools to convert between ProseMirror's document model and Markdown. It allows you to parse Markdown into a ProseMirror document and serialize a ProseMirror document back into Markdown.
Parsing Markdown to ProseMirror Document
This feature allows you to parse a Markdown string into a ProseMirror document. The code sample demonstrates how to use the defaultMarkdownParser to convert a Markdown string into a ProseMirror document.
const { schema } = require('prosemirror-schema-basic');
const { defaultMarkdownParser } = require('prosemirror-markdown');
const { DOMParser } = require('prosemirror-model');
const markdownText = '# Hello World\nThis is a paragraph.';
const doc = defaultMarkdownParser.parse(markdownText);
console.log(doc.toJSON());
Serializing ProseMirror Document to Markdown
This feature allows you to serialize a ProseMirror document back into a Markdown string. The code sample demonstrates how to use the defaultMarkdownSerializer to convert a ProseMirror document into a Markdown string.
const { schema } = require('prosemirror-schema-basic');
const { defaultMarkdownSerializer } = require('prosemirror-markdown');
const { Node } = require('prosemirror-model');
const doc = Node.fromJSON(schema, {
type: 'doc',
content: [
{ type: 'heading', attrs: { level: 1 }, content: [{ type: 'text', text: 'Hello World' }] },
{ type: 'paragraph', content: [{ type: 'text', text: 'This is a paragraph.' }] }
]
});
const markdownText = defaultMarkdownSerializer.serialize(doc);
console.log(markdownText);
markdown-it is a Markdown parser that can be extended with plugins to support custom syntax. Unlike prosemirror-markdown, it does not directly integrate with ProseMirror but can be used to parse and render Markdown independently.
remark is a Markdown processor powered by plugins. It can parse and transform Markdown and can be extended to support various syntaxes. While it does not directly integrate with ProseMirror, it offers a flexible way to handle Markdown processing.
showdown is a bidirectional Markdown to HTML converter written in JavaScript. It focuses on converting Markdown to HTML and vice versa, but does not provide direct integration with ProseMirror's document model.
[ WEBSITE | ISSUES | FORUM | GITTER ]
This is a (non-core) module for ProseMirror. ProseMirror is a well-behaved rich semantic content editor based on contentEditable, with support for collaborative editing and custom document schemas.
This module implements a ProseMirror schema that corresponds to the document schema used by CommonMark, and a parser and serializer to convert between ProseMirror documents in that schema and CommonMark/Markdown text.
This code is released under an MIT license. There's a forum for general discussion and support requests, and the Github bug tracker is the place to report issues.
We aim to be an inclusive, welcoming community. To make that explicit, we have a code of conduct that applies to communication around the project.
schema: Schema<"doc" | "paragraph" | "blockquote" | "horizontal_rule" | "heading" | "code_block" | "ordered_list" | "bullet_list" | "list_item" | "text" | "image" | "hard_break", "em" | "strong" | "link" | "code">
Document schema for the data model used by CommonMark.
class
MarkdownParserA configuration of a Markdown parser. Such a parser uses markdown-it to tokenize a file, and then runs the custom rules it is given over the tokens to create a ProseMirror document tree.
new MarkdownParser(schema: Schema, tokenizer: any, tokens: Object<ParseSpec>)
Create a parser with the given configuration. You can configure
the markdown-it parser to parse the dialect you want, and provide
a description of the ProseMirror entities those tokens map to in
the tokens
object, which maps token names to descriptions of
what to do with them. Such a description is an object, and may
have the following properties:
schema: Schema
The parser's document schema.
tokenizer: any
This parser's markdown-it tokenizer.
tokens: Object<ParseSpec>
The value of the tokens
object used to construct this
parser. Can be useful to copy and modify to base other parsers
on.
parse(text: string) → any
Parse a string as CommonMark markup, and create a ProseMirror document as prescribed by this parser's rules.
interface
ParseSpecObject type used to specify how Markdown tokens should be parsed.
node?: string
This token maps to a single node, whose type can be looked up
in the schema under the given name. Exactly one of node
,
block
, or mark
must be set.
block?: string
This token (unless noCloseToken
is true) comes in _open
and _close
variants (which are appended to the base token
name provides a the object property), and wraps a block of
content. The block should be wrapped in a node of the type
named to by the property's value. If the token does not have
_open
or _close
, use the noCloseToken
option.
mark?: string
This token (again, unless noCloseToken
is true) also comes
in _open
and _close
variants, but should add a mark
(named by the value) to its content, rather than wrapping it
in a node.
attrs?: Attrs
Attributes for the node or mark. When getAttrs
is provided,
it takes precedence.
getAttrs?: fn(token: any, tokenStream: any[], index: number) → Attrs
A function used to compute the attributes for the node or mark that takes a markdown-it token and returns an attribute object.
noCloseToken?: boolean
Indicates that the markdown-it
token has
no _open
or _close
for the nodes. This defaults to true
for code_inline
, code_block
and fence
.
ignore?: boolean
When true, ignore content for the matched token.
defaultMarkdownParser: MarkdownParser
A parser parsing unextended CommonMark, without inline HTML, and producing a document in the basic schema.
class
MarkdownSerializerA specification for serializing a ProseMirror document as Markdown/CommonMark text.
new MarkdownSerializer(nodes: Object<fn(state: MarkdownSerializerState, node: Node, parent: Node, index: number)>, marks: Object<Object>, options?: Object = {})
Construct a serializer with the given configuration. The nodes
object should map node names in a given schema to function that
take a serializer state and such a node, and serialize the node.
options
escapeExtraCharacters?: RegExp
Extra characters can be added for escaping. This is passed directly to String.replace(), and the matching characters are preceded by a backslash.
nodes: Object<fn(state: MarkdownSerializerState, node: Node, parent: Node, index: number)>
The node serializer functions for this serializer.
marks: Object<Object>
The mark serializer info.
options: Object
escapeExtraCharacters?: RegExp
Extra characters can be added for escaping. This is passed directly to String.replace(), and the matching characters are preceded by a backslash.
serialize(content: Node, options?: Object = {}) → string
Serialize the content of the given node to CommonMark.
options
tightLists?: boolean
Whether to render lists in a tight style. This can be overridden on a node level by specifying a tight attribute on the node. Defaults to false.
class
MarkdownSerializerStateThis is an object used to track state and expose
methods related to markdown serialization. Instances are passed to
node and mark serialization methods (see toMarkdown
).
options: {tightLists?: boolean, escapeExtraCharacters?: RegExp}
The options passed to the serializer.
wrapBlock(delim: string, firstDelim: string, node: Node, f: fn())
Render a block, prefixing each line with delim
, and the first
line in firstDelim
. node
should be the node that is closed at
the end of the block, and f
is a function that renders the
content of the block.
ensureNewLine()
Ensure the current content ends with a newline.
write(content?: string)
Prepare the state for writing output (closing closed paragraphs, adding delimiters, and so on), and then optionally add content (unescaped) to the output.
closeBlock(node: Node)
Close the block for the given node.
text(text: string, escape?: boolean = true)
Add the given text to the document. When escape is not false
,
it will be escaped.
render(node: Node, parent: Node, index: number)
Render the given node as a block.
renderContent(parent: Node)
Render the contents of parent
as block nodes.
renderInline(parent: Node)
Render the contents of parent
as inline content.
renderList(node: Node, delim: string, firstDelim: fn(index: number) → string)
Render a node's content as a list. delim
should be the extra
indentation added to all lines except the first in an item,
firstDelim
is a function going from an item index to a
delimiter for the first line of the item.
esc(str: string, startOfLine?: boolean = false) → string
Escape the given string so that it can safely appear in Markdown
content. If startOfLine
is true, also escape characters that
have special meaning only at the start of the line.
repeat(str: string, n: number) → string
Repeat the given string n
times.
markString(mark: Mark, open: boolean, parent: Node, index: number) → string
Get the markdown string for a given opening or closing mark.
getEnclosingWhitespace(text: string) → {leading?: string, trailing?: string}
Get leading and trailing whitespace from a string. Values of leading or trailing property of the return object will be undefined if there is no match.
defaultMarkdownSerializer: MarkdownSerializer
A serializer for the basic schema.
1.13.1 (2024-09-26)
Fix a type error caused by use of an older markdown-it type package.
FAQs
ProseMirror Markdown integration
The npm package prosemirror-markdown receives a total of 0 weekly downloads. As such, prosemirror-markdown popularity was classified as not popular.
We found that prosemirror-markdown demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.