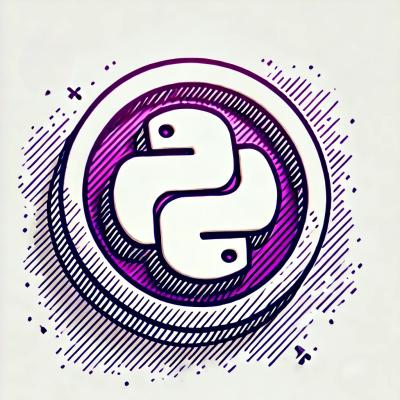
Security News
PyPI Introduces Digital Attestations to Strengthen Python Package Security
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
proxy-middleware
Advanced tools
The proxy-middleware npm package is a simple middleware for connecting to a proxy server. It is typically used in development environments to forward requests to another server, often to avoid cross-origin issues or to simulate a production environment.
Basic Proxy Setup
This code sets up a basic proxy server that forwards requests from '/api' to 'http://example.com'. It uses the 'connect' package to create a server and the 'proxy-middleware' package to handle the proxying.
const connect = require('connect');
const http = require('http');
const proxy = require('proxy-middleware');
const url = require('url');
const app = connect();
const proxyOptions = url.parse('http://example.com');
proxyOptions.route = '/api';
app.use(proxy(proxyOptions));
http.createServer(app).listen(3000);
Custom Headers
This code demonstrates how to add custom headers to the proxied requests. The 'headers' option in 'proxyOptions' allows you to specify any headers you want to include in the request.
const connect = require('connect');
const http = require('http');
const proxy = require('proxy-middleware');
const url = require('url');
const app = connect();
const proxyOptions = url.parse('http://example.com');
proxyOptions.route = '/api';
proxyOptions.headers = { 'X-Special-Proxy-Header': 'foobar' };
app.use(proxy(proxyOptions));
http.createServer(app).listen(3000);
Path Rewriting
This code shows how to rewrite the path of the proxied request. The 'rewrite' option in 'proxyOptions' allows you to specify a mapping of paths to be rewritten.
const connect = require('connect');
const http = require('http');
const proxy = require('proxy-middleware');
const url = require('url');
const app = connect();
const proxyOptions = url.parse('http://example.com');
proxyOptions.route = '/api';
proxyOptions.rewrite = { '^/api': '/new-api' };
app.use(proxy(proxyOptions));
http.createServer(app).listen(3000);
The 'http-proxy-middleware' package is a more feature-rich alternative to 'proxy-middleware'. It provides additional functionalities such as context matching, path rewriting, and more advanced proxying options. It is also actively maintained and widely used in the community.
The 'node-http-proxy' package is a powerful HTTP proxy library for Node.js. It offers a wide range of features including WebSocket proxying, load balancing, and more. It is more complex than 'proxy-middleware' but provides greater flexibility and control over proxying behavior.
The 'express-http-proxy' package is designed specifically for use with Express.js. It provides a simple way to proxy requests in an Express application, with support for custom headers, path rewriting, and more. It is a good choice if you are already using Express.js in your project.
var connect = require('connect');
var url = require('url');
var proxy = require('proxy-middleware');
var app = connect();
app.use('/api', proxy(url.parse('https://example.com/endpoint')));
// now requests to '/api/x/y/z' are proxied to 'https://example.com/endpoint/x/y/z'
//same as example above but also uses a short hand string only parameter
app.use('/api-string-only', proxy('https://example.com/endpoint'));
proxyMiddleware(options)
options
allows any options that are permitted on the http
or https
request options.
Other options:
route
: you can pass the route for connect middleware within the options, as well.via
: by default no via header is added. If you pass true
for this option the local hostname will be used for the via header. You can also pass a string for this option in which case that will be used for the via header.cookieRewrite
: this option can be used to support cookies via the proxy by rewriting the cookie domain to that of the proxy server. By default cookie domains are not rewritten. The cookieRewrite
option works as the via
option - if you pass true
the local hostname will be used, and if you pass a string that will be used as the rewritten cookie domain.preserveHost
: When enabled, this option will pass the Host: line from the incoming request to the proxied host. Default: false
.var proxyOptions = url.parse('https://example.com/endpoint');
proxyOptions.route = '/api';
var middleWares = [proxy(proxyOptions) /*, ...*/];
// Grunt connect uses this method
connect(middleWares);
FAQs
http(s) proxy as connect middleware
The npm package proxy-middleware receives a total of 168,557 weekly downloads. As such, proxy-middleware popularity was classified as popular.
We found that proxy-middleware demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.