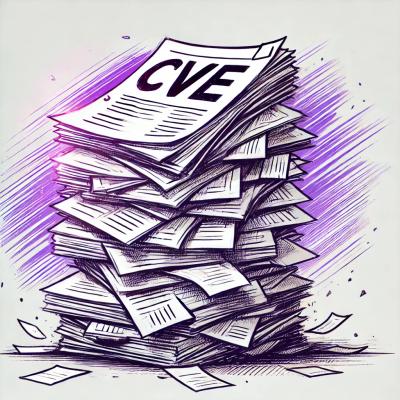
Security News
Node.js EOL Versions CVE Dubbed the "Worst CVE of the Year" by Security Experts
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
PubNub is a real-time communication platform that allows developers to build applications with real-time messaging, presence, and data synchronization capabilities. It is commonly used for chat applications, live updates, IoT device communication, and more.
Real-time Messaging
This feature allows you to send real-time messages to a specific channel. The code sample demonstrates how to publish a message to a channel using PubNub.
const PubNub = require('pubnub');
const pubnub = new PubNub({
publishKey: 'your-publish-key',
subscribeKey: 'your-subscribe-key'
});
pubnub.publish({
channel: 'my_channel',
message: { text: 'Hello, World!' }
}, (status, response) => {
if (status.error) {
console.log('Publish failed: ', status);
} else {
console.log('Message published with timetoken', response.timetoken);
}
});
Real-time Subscription
This feature allows you to subscribe to a channel and receive real-time messages. The code sample demonstrates how to listen for new messages on a subscribed channel.
const PubNub = require('pubnub');
const pubnub = new PubNub({
publishKey: 'your-publish-key',
subscribeKey: 'your-subscribe-key'
});
pubnub.addListener({
message: function(event) {
console.log('New message received: ', event.message);
}
});
pubnub.subscribe({
channels: ['my_channel']
});
Presence Detection
This feature allows you to detect the presence of users in a channel. The code sample demonstrates how to listen for presence events such as join, leave, and timeout.
const PubNub = require('pubnub');
const pubnub = new PubNub({
publishKey: 'your-publish-key',
subscribeKey: 'your-subscribe-key'
});
pubnub.addListener({
presence: function(event) {
console.log('Presence event: ', event);
}
});
pubnub.subscribe({
channels: ['my_channel'],
withPresence: true
});
History
This feature allows you to retrieve the message history of a channel. The code sample demonstrates how to fetch the last 10 messages from a channel's history.
const PubNub = require('pubnub');
const pubnub = new PubNub({
publishKey: 'your-publish-key',
subscribeKey: 'your-subscribe-key'
});
pubnub.history({
channel: 'my_channel',
count: 10
}, (status, response) => {
if (status.error) {
console.log('History retrieval failed: ', status);
} else {
console.log('Message history: ', response.messages);
}
});
Socket.IO is a library that enables real-time, bidirectional, and event-based communication between web clients and servers. It is commonly used for chat applications, live updates, and real-time analytics. Compared to PubNub, Socket.IO is more focused on web sockets and may require more server-side setup.
Pusher is a hosted service that makes it easy to add real-time data and functionality to web and mobile applications. It offers features like real-time messaging, presence, and webhooks. Pusher is similar to PubNub in terms of functionality but is a hosted service with a different pricing model.
Ably is a real-time messaging service that provides pub/sub messaging, presence, and data synchronization. It is designed for high scalability and reliability. Ably offers similar features to PubNub but emphasizes performance and global data distribution.
PubNub for JavaScript Docs have been moved to: http://www.pubnub.com/docs/javascript/javascript-sdk.html
FAQs
Publish & Subscribe Real-time Messaging with PubNub
The npm package pubnub receives a total of 129,987 weekly downloads. As such, pubnub popularity was classified as popular.
We found that pubnub demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 7 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.