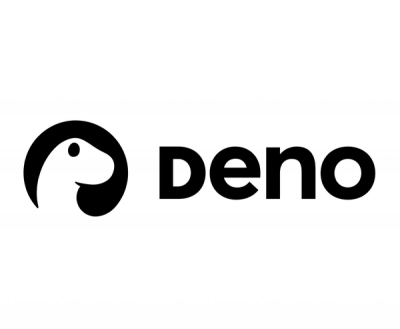
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
npm install qiwi-shop
Qiwi shop rest api module (qiwi.com)
const QiwiShop = require("qiwi-shop");
const express = require("express");
const bodyParser = require("body-parser");
const app = express();
app.use(bodyParser.json());
const projectId = "0000001"; // shop id
const apiId = "000000001"; // api id
const apiPassword = "api"; // api password
const notificationPassword = "notification"; // notification password
const inFiveDays = new Date().getTime() + 1000 * 60 * 60 * 24 * 5;
const qiwi = new QiwiShop(projectId, apiId, apiPassword, notificationPassword);
// this action is optional
qiwi.beforeCreateBill = function (billId, data) {
// you can save some information to db before the request e.t.c.
// you can return a promise
}
app.post('/payments/bill/create/', (req, res, next) => {
qiwi.createBill({
user: 'tel:+7910100100',
amount: '10',
ccy: 'RUB',
comment: 'recharge',
lifetime: new Date(inFiveDays).toISOString(),
pay_source: 'qw',
prv_name: 'example.com'
}).then((result) => {
if(!result || !result.response || result.response.result_code != 0) {
throw new Error('Qiwi bill creation fail');
}
// redirect to payment (optional)
res.redirect(qiwi.getPaymentUrl({
shop: qiwi.projectId,
transaction: result.response.bill.bill_id
}));
});
});
// notification handler
const successHandler = (data, callback) => {
// data === req.body
// save payment info or something else to db e.t.c
// callback() or return promise
};
const errorHandler = (err, meta) => {
// you can save something to a file, db e.t.c.
// the operation must be synchronous or in the background
};
let authenticationBySignature = false; // false == basic authentication
app.post('payments/notification/', qiwi.notify(successHandler, errorHandler, authenticationBySignature));
You can write a custom notification handler, but the library version includes a data/authentication validation and automatically send all headers in the necessary format
you can find all arguments in your qiwi shop account
returns qiwi bill creation url
will be called before the bill creation request, but after the hash creation
returns promise, create a bill, data options must comply with documentation
.user and .amount is required
returns a promise, get a bill status
returns a promise, cancel a bill
returns a promise, refunds a bill, data options must comply with documentation
returns a promise, gets a bill refund status
checks a notification by a basic authentication
checks a notification by a signature
creates xml string for the response
qiwi notification handler, it is "connect" middleware
FAQs
Qiwi shop rest api module (qiwi.com)
The npm package qiwi-shop receives a total of 2 weekly downloads. As such, qiwi-shop popularity was classified as not popular.
We found that qiwi-shop demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.