What is qrcode-terminal?
The qrcode-terminal package allows you to generate QR codes directly in your terminal. This is particularly useful for CLI applications and development tools where displaying a QR code in a graphical user interface is not feasible. The QR codes generated can encode URLs, text, or other data, and can be scanned using a smartphone or any other QR code reader.
What are qrcode-terminal's main functionalities?
Generate QR Code in Terminal
This feature allows you to generate a QR code in the terminal. The example provided generates a QR code for the URL 'https://www.example.com'. The option 'small: true' makes the QR code compact.
const qrcode = require('qrcode-terminal');
qrcode.generate('https://www.example.com', { small: true }, function (qrcode) {
console.log(qrcode);
});
Other packages similar to qrcode-terminal
qrcode
The 'qrcode' package is a more versatile library for generating QR codes. It supports generating QR codes in various formats including image files, which makes it suitable for both server-side and client-side applications. Unlike qrcode-terminal, which is limited to terminal output, qrcode can be used in a broader range of applications.
qr-image
This package allows you to generate QR codes as PNG, SVG, EPS, or PDF files. It is similar to qrcode-terminal in that it encodes data into QR codes, but it offers more output formats, making it more flexible for different use cases where file outputs are required rather than terminal display.
QRCode Terminal Edition 
Going where no QRCode has gone before.
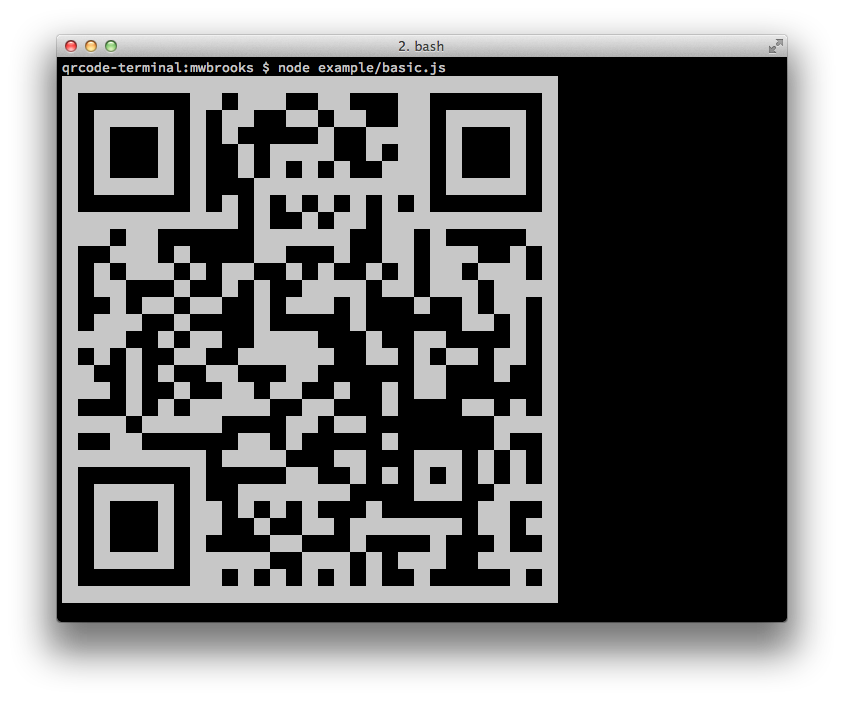
Node Library
Install
Can be installed with:
$ npm install qrcode-terminal
and used:
var qrcode = require('qrcode-terminal');
Usage
To display some data to the terminal just call:
qrcode.generate('This will be a QRCode, eh!');
You can even specify the error level (default is 'L'):
qrcode.setErrorLevel('Q');
qrcode.generate('This will be a QRCode with error level Q!');
If you don't want to display to the terminal but just want to string you can provide a callback:
qrcode.generate('http://github.com', function (qrcode) {
console.log(qrcode);
});
If you want to display small output, provide opts
with small
:
qrcode.generate('This will be a small QRCode, eh!', {small: true});
qrcode.generate('This will be a small QRCode, eh!', {small: true}, function (qrcode) {
console.log(qrcode)
});
Command-Line
Install
$ npm install -g qrcode-terminal
Usage
$ qrcode-terminal --help
$ qrcode-terminal 'http://github.com'
$ echo 'http://github.com' | qrcode-terminal
Support
Server-side
node-qrcode is a popular server-side QRCode generator that
renders to a canvas
object.
Developing
To setup the development envrionment run npm install
To run tests run npm test
Contributers
Gord Tanner <gtanner@gmail.com>
Micheal Brooks <michael@michaelbrooks.ca>