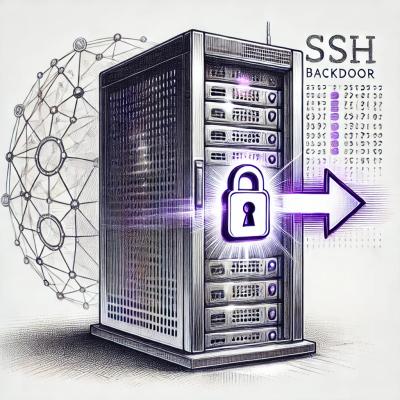
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
QReader is a JavaScript library that provides an easy-to-use QR code scanner using a video element. It allows you to integrate QR code scanning functionality into web applications with minimal setup.
Note! This library is in a very early development stage, and may contain bugs.
You can install QReader via npm:
npm install qreader
import QReader from 'qreader';
// Assuming you have a <video> element in your HTML
const videoElement = document.createElement('video');
document.body.appendChild(videoElement);
const qreader = new QReader(videoElement, onScanCallback);
function onScanCallback(data) {
console.log('QR Code scanned:', data);
// Handle the scanned QR code data here
}
// Start scanning
qreader.resumeScanning();
// Pause scanning
// qreader.pauseScanning();
// Destroy instance when done
// qreader.destroyInstance();
import QReader from 'qreader';
const qreader = new QReader(videoElement, onScan, options?);
Method | Description |
---|---|
pauseScanning() | Pauses QR code scanning. |
resumeScanning() | Resumes QR code scanning. |
destroyInstance() | Stops the QR code scanner and cleans up resources. |
Property | Type | Description |
---|---|---|
latestScan | string | null | Contains the latest scanned QR code data, or null if no QR code has been scanned yet. |
isCameraActive | boolean | Indicates whether the camera is actively scanning for QR codes. |
isScanningPaused | boolean | Indicates whether the scanning process is currently paused. |
FAQs
QR Code Scanner written in Typescript
We found that qreader demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.