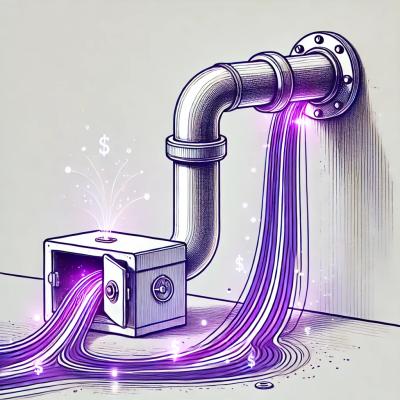
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
queue-typescript
Advanced tools
Simple Typescript Queue with generics type templating and support for iterator and iterable protocols.
This queue uses the linked-list-typescript as the underlying datastructure.
See Also:
npm:
npm install --save queue-typescript
yarn:
yarn add queue-typescript
install dev dependencies. There are no production dependencies.
yarn
npm install
build using the options in tsconfig.json
yarn|npm run build
run all package tests
yarn|npm run test
see the test coverage report
yarn|npm run coverage
yarn|npm run coverage:report
Importing:
import { Queue } from 'queue-typescript';
const { Queue } = require('queue-typescript')
Create an empty queue by omitting any arguments during instantiation.
let queue = new Queue<number>()
Create a new queue and initialize it with values. Values will be added from front to back. i.e. the first argument will be at the front of the queue and the last argument will be at the back of the queue.
Specify the type using the typescript templating to enable type-checking of all values going into and out of the queue.
let items: number[] = [4, 5, 6, 7];
let queue = new Queue<number>(...items);
let items: string[] = ['one', 'two', 'three', 'four'];
let queue = new Queue<string>(...items);
Typescript will check if the values match the type given to the template when initializing the new queue.
let items: = ['one', 'two', 'three', 4];
let queue = new Queue<string>(...items); // arguments are not all strings
Create a new queue using custom types or classes. All values are retained as references and not copies so removed values can be compared using strict comparison.
class Foo {
private val:number;
constructor(val: number) {
this.val = val;
}
get bar(): number { return this.val }
}
let foo1 = new Foo(1);
let foo2 = new Foo(2);
let foo3 = new Foo(3);
let fooQueue = new Queue<Foo>(foo1, foo2, foo3)
fooQueue.front.bar // => 1
let val = queue.dequeue()
val // => foo1
Specify any
to allow the queue to take values of any type.
let queue = new Queue<any>(4, 'hello' { hello: 'world' })
queue.length // => 3
queue.front // => 4
The queue supports both iterator and iterable protocols allowing it to be used
with the for...of
and ...spread
operators and with deconstruction.
for...of
:
let items: number[] = [4, 5, 6];
let queue = new Queue<number>(...items);
for (let item of queue) {
console.log(item)
}
//4
//5
//6
...spread
:
let items: number[] = [4, 5, 6];
let queue = new Queue<number>(...items);
function manyArgs(...args) {
for (let i in args) {
console.log(args[i])
}
}
manyArgs(...queue);
//4
//5
//6
deconstruction
:
let items: number[] = [4, 5, 6, 7];
let queue = new Queue<number>(...items);
let [a, b, c] = queue;
//a => 4
//b => 5
//c => 6
Peek at the front of the queue. This will not remove the value from the queue.
let items: number[] = [4, 5, 6, 7];
let queue = new Queue<number>(...items);
queue.front // => 4
Query the length of the queue. An empty queue will return 0.
let items: number[] = [4, 5, 6, 7];
let queue = new Queue<number>(...items);
queue.length // => 4
Enqueue an item at the back of the queue. The new item will replace the previous last item.
let items: number[] = [4, 5, 6, 7];
let queue = new Queue<number>(...items);
queue.length // => 4
queue.enqueue(8)
queue.length // => 5
Removes the item from the front of the queue and returns the item.
let items: number[] = [4, 5, 6, 7];
let queue = new Queue<number>(...items);
queue.length // => 4
let val = queue.dequeue()
queue.length // => 3
queue.front // => 5
val // => 4
This method simply returns [...this]
.
Converts the queue into an array and returns the array representation. This method does not mutate the queue in any way.
Objects are not copied, so all non-primitive items in the array are still referencing the queue items.
let items: number[] = [4, 5, 6, 7];
let queue = new Queue<number>(...items);
let result = queue.toArray()
result // => [4, 5, 6, 7]
FAQs
Simple Typescript Queue with generics type support
The npm package queue-typescript receives a total of 7,613 weekly downloads. As such, queue-typescript popularity was classified as popular.
We found that queue-typescript demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.