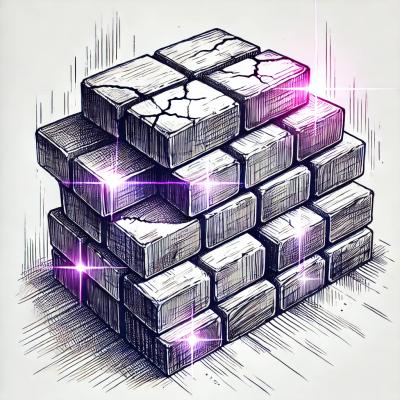
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
quiq-chat
Advanced tools
A high-level JavaScript Library to handle the communication with Quiq Messaging APIs when building a web chat app
A high-level JavaScript Library to handle the communication with Quiq Messaging APIs when building a web chat app
Install quiq-chat
with
npm install --save quiq-chat
or
yarn add quiq-chat
This library is built for modern browsers, and expects access to ES6 prototype methods. If you need to target older browsers, you'll need to include a polyfill. We recommend babel-polyfill:
Install with
npm install --save babel-polyfill
or
yarn add babel-polyfill
Then include at the top of your module like so:
import 'babel-polyfill';
Note that this will modify the prototypes of global objects such as Array and Object, as well as add a Promise prototype.
The quiq-chat library exports a QuiqChatClient, which is a singleton. This means that you can import it as many times as you want, in as many modules as you want, and always get back the same instance of the client.
Import it like so:
import QuiqChatClient from 'quiq-chat';
QuiqChatClient
exposes a fluent API for client setup, allowing you to chain calls together.
First, we call initialize(host, contactPoint)
. We then register our own handler functions to respond to different chat events. Finally, we call start()
.
The start
method returns a promise that resolves once the client is fully initialized and ready to send and receive messages.
Below we show a simple setup, with handler functions for new transcript elements and agent typing events.
QuiqChatClient
.initialize('tenant.goquiq.com', 'default')
.onTranscriptChange(messages => {
// Will log out messages and events (such as user registration and transcript email)
messages.forEach(msg => console.log(msg)
})
.onAgentTyping(isTyping => {
if (isTyping) {
console.log("The agent started typing!")
} else {
console.log("The agent stopped typing!")
}
})
// Connect that chat client
.start()
.then(() => {
console.log("The chat client is connected and ready to send and receive messages");
});
Begins the chat session, allowing the client to send and receive messages. Should be called immediately after initializing and registering event handlers. The returned Promise is resolved once the session is active and everything is ready to go.
Ends the chat session. The client will no longer receive messages.
Register your event handling functions prior calling QuiqChatClient.start()
. All of these methods can be chained together.
Called whenever new messages are received. transcriptItems
is an array containing full transcript (messages and events) of the current chat.
Called whenever the support agent starts or stops typing
Called whenever there is a non-retryable error or an error that has exceeded the maximum number of retries from the API.
Called whenever any error from the API has been resolved
Called when a text or attachment message could not be delivered to the agent.
Called when Register event is received through a websocket message
Called when the end users previous session has expired and has begun a new session. This is a good spot to have the UI reset itself to an initial state
Called when the isAgentAssigned value changes.
Called when the estimate wait time calculation changes.
Called when chat is trying to reconnect with Quiq's servers (reconnecting === true
), or has finished reconnecting (reconnecting === false
). This can be used for showing a "we're trying to reconnect you" message or similar.
Called when quiq-chat gets in a fatal state and page holding webchat needs to be refreshed.
Called whenever Quiq-related data stored in the browser's localStorage changes.
Context is data that is set by the chat client and is available throughout the Quiq system as part of the conversation. It can be viewed by agents, included in webhooks and leveraged in queuing rules.
Replaces the entire context. This new context will be sent to Quiq with every subsequent message.
Performs a shallow merge of the current context with the provided updates. The resulting context will be sent to Quiq with every subsequent message.
Retrieve all messages and events for the current chat. If cache
is set to true, a hit to the API is not made, and only the messages currently in memory are returned.
Send a text message from the customer. The first message sent from the client will initialize (start) a conversation.
Send an attachment message containing a File from the customer. The type of this file must conform to the allowed file types set in your configuration. The method also accepts a progressCallback
function which will be fired during upload of the file with values between 0 and 100, denoting percentage uploaded. Upon completion of upload, this method returns a string containing the id
of the new message.
Returns whether the end user has triggered a registration event. This happens when the sendRegistration
API is called, and the server has confirmed the registration was valid.
Returns the unique identifier for this session. If the user is not logged in, returns undefined
.
Creates a session for the current user, if one does not already exist. Returns the unique identifier (handle) for the new or existing session.
Fetches whether or not there are agents available for the contact point the webchat is connected to. The value of this call is cached for 10 seconds.
Sends a message to Quiq Messaging that the end user is typing and what they've typed in the message field
Returns whether the end user's chat has been taken up by an agent. This returns true when the agent sends their first message.
Returns the estimate wait time in milliseconds. This is the amount of time we estimate it will take for the user's chat to be assigned to an agent. If this is undefined or null, then no ETA is currently available.
Email a transcript of the current conversation to the specified e-mail. If an agent has not yet responded to the conversation, a 400 will be returned.
Sets which levels of log statements will be output to the console. Pass in silent
to disable logging entirely.
Utility function to tell the client if quiq-chat has the capability to set its required data in a persistent way.
Utility function to return if the end-user is using a browser supported by Quiq.
Returns whether the end-user has performed a meaningful action, such as submitting the Welcome Form, or sending a message to the agent.
Returns the last state of chat's visibility. Can be used to re-open webchat on page turns if the user had chat previously open. Defaults to false if user has taken no actions.
Returns all Quiq-related data stored locally in the browser's localStorage. Includes any custom data set using the setCustomPersistentData()
method.
Stores a key/value pair in persistent storage (available between refreshes and browser closes). Can be retrieved using the getPersistentData()
method.
TextMessage | AttachmentMessage;
{
authorType: 'Customer' | 'User',
text: string,
id: string,
timestamp: number,
type: 'Text',
}
{
id: string,
timestamp: number,
type: 'Attachment',
authorType: 'Customer' | 'User',
url: string,
contentType: string,
}
{
authorType?: 'Customer' | 'User',
id: string,
timestamp: number,
type: 'Join' | 'Leave' | 'Register' | 'SendTranscript' | 'End' | 'Spam',
}
{
code?: number,
message?: string,
status?: number,
}
'Join' | 'Leave';
{
email: string,
originUrl: string,
timezone?: string,
};
{
id: string,
timestamp: number,
type: string,
};
{
href?: string, // The URL of the page from which the customer is currently chatting. For example, agents can use this value to know which product the customer is viewing.
intent?: string, // Indicates the "purpose" of a conversation, can be used in the Quiq queuing system to control where the chat conversation is routed.
data?: Object, // Arbitrary, serialiozable data that can be accessed in bots, webhokos and queing rules.
};
QuiqChat works with any browser that supports Local Storage, and CORS requests. The isSupportedBrowser
utility function can be used to determine if the end-user is using a browser supported by Quiq. The following browsers and versions are supported:
FAQs
The quiq-chat library has been officially deprecated. Contact Quiq for more information.
The npm package quiq-chat receives a total of 24 weekly downloads. As such, quiq-chat popularity was classified as not popular.
We found that quiq-chat demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.