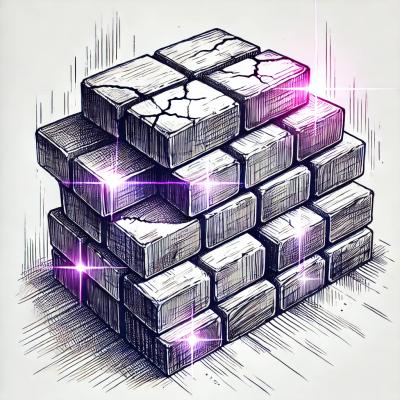
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
npm install quoridor
Generates a new game.
import { createNewGame, getUnicodeRepresentation } from 'quoridor';
const game = createNewGame();
console.log(getUnicodeRepresentation(game));
// ┌───╫───╫───╫───╫───╫───╫───╫───╫───╫───╫───┐
// │ ║ ║ ║ ║ ║ ║ ║ ║ ║ ║ │
// │ ┌───┬───┬───┬───┬───┬───┬───┬───┬───┐ │
// │ 9 │ │ │ │ │ 2 │ │ │ │ │ │
// │ ├───┼───┼───┼───┼───┼───┼───┼───┼───┤ │
// │ 8 │ │ │ │ │ │ │ │ │ │ │
// │ ├───┼───┼───┼───┼───┼───┼───┼───┼───┤ │
// │ 7 │ │ │ │ │ │ │ │ │ │ │
// │ ├───┼───┼───┼───┼───┼───┼───┼───┼───┤ │
// │ 6 │ │ │ │ │ │ │ │ │ │ │
// │ ├───┼───┼───┼───┼───┼───┼───┼───┼───┤ │
// │ 5 │ │ │ │ │ │ │ │ │ │ │
// │ ├───┼───┼───┼───┼───┼───┼───┼───┼───┤ │
// │ 4 │ │ │ │ │ │ │ │ │ │ │
// │ ├───┼───┼───┼───┼───┼───┼───┼───┼───┤ │
// │ 3 │ │ │ │ │ │ │ │ │ │ │
// │ ├───┼───┼───┼───┼───┼───┼───┼───┼───┤ │
// │ 2 │ │ │ │ │ │ │ │ │ │ │
// │ ├───┼───┼───┼───┼───┼───┼───┼───┼───┤ │
// │ 1 │ │ │ │ │ 1 │ │ │ │ │ │
// │ └───┴───┴───┴───┴───┴───┴───┴───┴───┘ │
// │ ║ A ║ B ║ C ║ D ║ E ║ F ║ G ║ H ║ I ║ │
// └───╫───╫───╫───╫───╫───╫───╫───╫───╫───╫───┘
Generates a game from an array of moves. Does not verify that the moves are valid.
import { createGameFromMoves, getUnicodeRepresentation } from 'quoridor';
const game = createGameFromMoves(['e2', 'e8', 'd7v']);
console.log(getUnicodeRepresentation(game));
// ┌───╫───╫───╫───╫───╫───╫───╫───╫───╫───╫───┐
// │ ║ ║ ║ ║ ║ ║ ║ ║ ║ ║ │
// │ ┌───┬───┬───┬───┬───┬───┬───┬───┬───┐ │
// │ 9 │ │ │ │ │ │ │ │ │ │ │
// │ ├───┼───┼───┼───┼───┼───┼───┼───┼───┤ │
// │ 8 │ │ │ │ │ │ │ │ │ │ │
// │ ├───┼───┼───┼───┼───┼───┼───┼───┼───┤ │
// │ 7 │ │ │ │ ║ 2 │ │ │ │ │ │
// │ ├───┼───┼───┼───╫───┼───┼───┼───┼───┤ │
// │ 6 │ │ │ │ ║ │ │ │ │ │ │
// │ ├───┼───┼───┼───┼───┼───┼───┼───┼───┤ │
// │ 5 │ │ │ │ │ │ │ │ │ │ │
// │ ├───┼───┼───┼───┼───┼───┼───┼───┼───┤ │
// │ 4 │ │ │ │ │ │ │ │ │ │ │
// │ ├───┼───┼───┼───┼───┼───┼───┼───┼───┤ │
// │ 3 │ │ │ │ │ 1 │ │ │ │ │ │
// │ ├───┼───┼───┼───┼───┼───┼───┼───┼───┤ │
// │ 2 │ │ │ │ │ │ │ │ │ │ │
// │ ├───┼───┼───┼───┼───┼───┼───┼───┼───┤ │
// │ 1 │ │ │ │ │ │ │ │ │ │ │
// │ └───┴───┴───┴───┴───┴───┴───┴───┴───┘ │
// │ ║ A ║ B ║ C ║ D ║ E ║ F ║ G ║ H ║ I │
// └───╫───╫───╫───╫───╫───╫───╫───╫───╫───────┘
Returns a string representation of the board game state using Unicode box-drawing characters.
import { createNewGame, getUnicodeRepresentation } from 'quoridor';
const unicodeRepresentation = getUnicodeRepresentation(createNewGame())
console.log(unicodeRepresentation);
// ┌───╫───╫───╫───╫───╫───╫───╫───╫───╫───╫───┐
// │ ║ ║ ║ ║ ║ ║ ║ ║ ║ ║ │
// │ ┌───┬───┬───┬───┬───┬───┬───┬───┬───┐ │
// │ 9 │ │ │ │ │ 2 │ │ │ │ │ │
// │ ├───┼───┼───┼───┼───┼───┼───┼───┼───┤ │
// │ 8 │ │ │ │ │ │ │ │ │ │ │
// │ ├───┼───┼───┼───┼───┼───┼───┼───┼───┤ │
// │ 7 │ │ │ │ │ │ │ │ │ │ │
// │ ├───┼───┼───┼───┼───┼───┼───┼───┼───┤ │
// │ 6 │ │ │ │ │ │ │ │ │ │ │
// │ ├───┼───┼───┼───┼───┼───┼───┼───┼───┤ │
// │ 5 │ │ │ │ │ │ │ │ │ │ │
// │ ├───┼───┼───┼───┼───┼───┼───┼───┼───┤ │
// │ 4 │ │ │ │ │ │ │ │ │ │ │
// │ ├───┼───┼───┼───┼───┼───┼───┼───┼───┤ │
// │ 3 │ │ │ │ │ │ │ │ │ │ │
// │ ├───┼───┼───┼───┼───┼───┼───┼───┼───┤ │
// │ 2 │ │ │ │ │ │ │ │ │ │ │
// │ ├───┼───┼───┼───┼───┼───┼───┼───┼───┤ │
// │ 1 │ │ │ │ │ 1 │ │ │ │ │ │
// │ └───┴───┴───┴───┴───┴───┴───┴───┴───┘ │
// │ ║ A ║ B ║ C ║ D ║ E ║ F ║ G ║ H ║ I ║ │
// └───╫───╫───╫───╫───╫───╫───╫───╫───╫───╫───┘
Checks if a move is valid.
import { createGameFromMoves, isMoveValid } from 'quoridor';
const game = createGameFromMoves(['e2', 'e8', 'd7v']);
const move = 'd7';
const moveIsValid = isMoveValid(game, move);
console.log(moveIsValid);
// false
Checks if a string is a move. This can be convenient when using TypeScript since it can be used as a type guard to safely cast a string to a Move.
import { isMove } from 'quoridor';
const game = createGameFromMoves(['e2', 'e8', 'd7v']);
const move = 'd7';
const moveIsValid = isMove(game, move);
console.log(isMove('a1'));
// true
console.log(isMove('humbug'));
// false
console.log(isMove('i9'))
// false
Returns a new game with the most recent move undone. If no moves have been made yet, an identical game is returned.
import { createGameFromMoves, undo, getUnicodeRepresentation } from 'quoridor';
const game = createGameFromMoves(['e2', 'e8', 'd7v']);
const gameWithUndoneMove = undo(game);
console.log(getUnicodeRepresentation(game));
// ┌───╫───╫───╫───╫───╫───╫───╫───╫───╫───╫───┐
// │ ║ ║ ║ ║ ║ ║ ║ ║ ║ ║ │
// │ ┌───┬───┬───┬───┬───┬───┬───┬───┬───┐ │
// │ 9 │ │ │ │ │ │ │ │ │ │ │
// │ ├───┼───┼───┼───┼───┼───┼───┼───┼───┤ │
// │ 8 │ │ │ │ │ │ │ │ │ │ │
// │ ├───┼───┼───┼───┼───┼───┼───┼───┼───┤ │
// │ 7 │ │ │ │ ║ 2 │ │ │ │ │ │
// │ ├───┼───┼───┼───╫───┼───┼───┼───┼───┤ │
// │ 6 │ │ │ │ ║ │ │ │ │ │ │
// │ ├───┼───┼───┼───┼───┼───┼───┼───┼───┤ │
// │ 5 │ │ │ │ │ │ │ │ │ │ │
// │ ├───┼───┼───┼───┼───┼───┼───┼───┼───┤ │
// │ 4 │ │ │ │ │ │ │ │ │ │ │
// │ ├───┼───┼───┼───┼───┼───┼───┼───┼───┤ │
// │ 3 │ │ │ │ │ 1 │ │ │ │ │ │
// │ ├───┼───┼───┼───┼───┼───┼───┼───┼───┤ │
// │ 2 │ │ │ │ │ │ │ │ │ │ │
// │ ├───┼───┼───┼───┼───┼───┼───┼───┼───┤ │
// │ 1 │ │ │ │ │ │ │ │ │ │ │
// │ └───┴───┴───┴───┴───┴───┴───┴───┴───┘ │
// │ ║ A ║ B ║ C ║ D ║ E ║ F ║ G ║ H ║ I │
// └───╫───╫───╫───╫───╫───╫───╫───╫───╫───────┘
console.log(getUnicodeRepresentation(gameWithUndoneMove));
// ┌───╫───╫───╫───╫───╫───╫───╫───╫───╫───╫───┐
// │ ║ ║ ║ ║ ║ ║ ║ ║ ║ ║ │
// │ ┌───┬───┬───┬───┬───┬───┬───┬───┬───┐ │
// │ 9 │ │ │ │ │ │ │ │ │ │ │
// │ ├───┼───┼───┼───┼───┼───┼───┼───┼───┤ │
// │ 8 │ │ │ │ │ │ │ │ │ │ │
// │ ├───┼───┼───┼───┼───┼───┼───┼───┼───┤ │
// │ 7 │ │ │ │ │ 2 │ │ │ │ │ │
// │ ├───┼───┼───┼───┼───┼───┼───┼───┼───┤ │
// │ 6 │ │ │ │ │ │ │ │ │ │ │
// │ ├───┼───┼───┼───┼───┼───┼───┼───┼───┤ │
// │ 5 │ │ │ │ │ │ │ │ │ │ │
// │ ├───┼───┼───┼───┼───┼───┼───┼───┼───┤ │
// │ 4 │ │ │ │ │ │ │ │ │ │ │
// │ ├───┼───┼───┼───┼───┼───┼───┼───┼───┤ │
// │ 3 │ │ │ │ │ 1 │ │ │ │ │ │
// │ ├───┼───┼───┼───┼───┼───┼───┼───┼───┤ │
// │ 2 │ │ │ │ │ │ │ │ │ │ │
// │ ├───┼───┼───┼───┼───┼───┼───┼───┼───┤ │
// │ 1 │ │ │ │ │ │ │ │ │ │ │
// │ └───┴───┴───┴───┴───┴───┴───┴───┴───┘ │
// │ ║ A ║ B ║ C ║ D ║ E ║ F ║ G ║ H ║ I ║ │
// └───╫───╫───╫───╫───╫───╫───╫───╫───╫───╫───┘
Returns a new game with the most recently undone move redone. If no moves have been undone yet, an identical game is returned.
import { createGameFromMoves, redo, undo, getUnicodeRepresentation } from 'quoridor';
const game = createGameFromMoves(['e2', 'e8', 'd7v']);
const gameWithUndoneMove = undo(game);
const gameWithUndoneMoveRedone = redo(gameWithUndoneMove);
console.log(getUnicodeRepresentation(game));
// ┌───╫───╫───╫───╫───╫───╫───╫───╫───╫───╫───┐
// │ ║ ║ ║ ║ ║ ║ ║ ║ ║ ║ │
// │ ┌───┬───┬───┬───┬───┬───┬───┬───┬───┐ │
// │ 9 │ │ │ │ │ │ │ │ │ │ │
// │ ├───┼───┼───┼───┼───┼───┼───┼───┼───┤ │
// │ 8 │ │ │ │ │ │ │ │ │ │ │
// │ ├───┼───┼───┼───┼───┼───┼───┼───┼───┤ │
// │ 7 │ │ │ │ ║ 2 │ │ │ │ │ │
// │ ├───┼───┼───┼───╫───┼───┼───┼───┼───┤ │
// │ 6 │ │ │ │ ║ │ │ │ │ │ │
// │ ├───┼───┼───┼───┼───┼───┼───┼───┼───┤ │
// │ 5 │ │ │ │ │ │ │ │ │ │ │
// │ ├───┼───┼───┼───┼───┼───┼───┼───┼───┤ │
// │ 4 │ │ │ │ │ │ │ │ │ │ │
// │ ├───┼───┼───┼───┼───┼───┼───┼───┼───┤ │
// │ 3 │ │ │ │ │ 1 │ │ │ │ │ │
// │ ├───┼───┼───┼───┼───┼───┼───┼───┼───┤ │
// │ 2 │ │ │ │ │ │ │ │ │ │ │
// │ ├───┼───┼───┼───┼───┼───┼───┼───┼───┤ │
// │ 1 │ │ │ │ │ │ │ │ │ │ │
// │ └───┴───┴───┴───┴───┴───┴───┴───┴───┘ │
// │ ║ A ║ B ║ C ║ D ║ E ║ F ║ G ║ H ║ I │
// └───╫───╫───╫───╫───╫───╫───╫───╫───╫───────┘
console.log(getUnicodeRepresentation(gameWithUndoneMove));
// ┌───╫───╫───╫───╫───╫───╫───╫───╫───╫───╫───┐
// │ ║ ║ ║ ║ ║ ║ ║ ║ ║ ║ │
// │ ┌───┬───┬───┬───┬───┬───┬───┬───┬───┐ │
// │ 9 │ │ │ │ │ │ │ │ │ │ │
// │ ├───┼───┼───┼───┼───┼───┼───┼───┼───┤ │
// │ 8 │ │ │ │ │ │ │ │ │ │ │
// │ ├───┼───┼───┼───┼───┼───┼───┼───┼───┤ │
// │ 7 │ │ │ │ │ 2 │ │ │ │ │ │
// │ ├───┼───┼───┼───┼───┼───┼───┼───┼───┤ │
// │ 6 │ │ │ │ │ │ │ │ │ │ │
// │ ├───┼───┼───┼───┼───┼───┼───┼───┼───┤ │
// │ 5 │ │ │ │ │ │ │ │ │ │ │
// │ ├───┼───┼───┼───┼───┼───┼───┼───┼───┤ │
// │ 4 │ │ │ │ │ │ │ │ │ │ │
// │ ├───┼───┼───┼───┼───┼───┼───┼───┼───┤ │
// │ 3 │ │ │ │ │ 1 │ │ │ │ │ │
// │ ├───┼───┼───┼───┼───┼───┼───┼───┼───┤ │
// │ 2 │ │ │ │ │ │ │ │ │ │ │
// │ ├───┼───┼───┼───┼───┼───┼───┼───┼───┤ │
// │ 1 │ │ │ │ │ │ │ │ │ │ │
// │ └───┴───┴───┴───┴───┴───┴───┴───┴───┘ │
// │ ║ A ║ B ║ C ║ D ║ E ║ F ║ G ║ H ║ I ║ │
// └───╫───╫───╫───╫───╫───╫───╫───╫───╫───╫───┘
console.log(getUnicodeRepresentation(gameWithUndoneMoveRedone));
// ┌───╫───╫───╫───╫───╫───╫───╫───╫───╫───╫───┐
// │ ║ ║ ║ ║ ║ ║ ║ ║ ║ ║ │
// │ ┌───┬───┬───┬───┬───┬───┬───┬───┬───┐ │
// │ 9 │ │ │ │ │ │ │ │ │ │ │
// │ ├───┼───┼───┼───┼───┼───┼───┼───┼───┤ │
// │ 8 │ │ │ │ │ │ │ │ │ │ │
// │ ├───┼───┼───┼───┼───┼───┼───┼───┼───┤ │
// │ 7 │ │ │ │ ║ 2 │ │ │ │ │ │
// │ ├───┼───┼───┼───╫───┼───┼───┼───┼───┤ │
// │ 6 │ │ │ │ ║ │ │ │ │ │ │
// │ ├───┼───┼───┼───┼───┼───┼───┼───┼───┤ │
// │ 5 │ │ │ │ │ │ │ │ │ │ │
// │ ├───┼───┼───┼───┼───┼───┼───┼───┼───┤ │
// │ 4 │ │ │ │ │ │ │ │ │ │ │
// │ ├───┼───┼───┼───┼───┼───┼───┼───┼───┤ │
// │ 3 │ │ │ │ │ 1 │ │ │ │ │ │
// │ ├───┼───┼───┼───┼───┼───┼───┼───┼───┤ │
// │ 2 │ │ │ │ │ │ │ │ │ │ │
// │ ├───┼───┼───┼───┼───┼───┼───┼───┼───┤ │
// │ 1 │ │ │ │ │ │ │ │ │ │ │
// │ └───┴───┴───┴───┴───┴───┴───┴───┴───┘ │
// │ ║ A ║ B ║ C ║ D ║ E ║ F ║ G ║ H ║ I │
// └───╫───╫───╫───╫───╫───╫───╫───╫───╫───────┘
Check that linting, formatting, build and tests pass
npm run lint
npm run format
npm run build
npm test
Bump version
npm version [major | minor | patch]
Publish to NPM
npm publish
FAQs
A JavaScipt Quoridor library for move validation etc.
The npm package quoridor receives a total of 8 weekly downloads. As such, quoridor popularity was classified as not popular.
We found that quoridor demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.