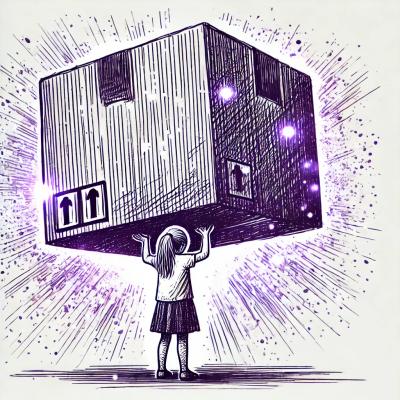
Security News
The Unpaid Backbone of Open Source: Solo Maintainers Face Increasing Security Demands
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.
Rambda is a lightweight and fast utility library that provides a variety of functions for functional programming in JavaScript. It is a smaller and faster alternative to Ramda, offering a similar API but with a focus on performance and simplicity.
Currying
Currying is a technique of evaluating functions with multiple arguments, one at a time. Rambda's `curry` function allows you to transform a function so that it can be called with fewer arguments than it expects, returning a new function that takes the remaining arguments.
const R = require('rambda');
const add = R.curry((a, b) => a + b);
const add5 = add(5);
console.log(add5(3)); // 8
Composition
Function composition is the process of combining two or more functions to produce a new function. Rambda's `compose` function allows you to create a pipeline of functions that are executed from right to left.
const R = require('rambda');
const add = (a, b) => a + b;
const multiply = (a, b) => a * b;
const addAndMultiply = R.compose(R.multiply(2), R.add(3));
console.log(addAndMultiply(4)); // 14
Cloning
Cloning is the process of creating a deep copy of an object. Rambda's `clone` function allows you to create a deep copy of an object, ensuring that changes to the new object do not affect the original object.
const R = require('rambda');
const obj = {a: 1, b: 2};
const clonedObj = R.clone(obj);
console.log(clonedObj); // {a: 1, b: 2}
Filtering
Filtering is the process of selecting a subset of items from a collection based on a predicate function. Rambda's `filter` function allows you to filter elements in an array or object based on a provided predicate.
const R = require('rambda');
const isEven = n => n % 2 === 0;
const numbers = [1, 2, 3, 4, 5, 6];
const evenNumbers = R.filter(isEven, numbers);
console.log(evenNumbers); // [2, 4, 6]
Mapping
Mapping is the process of transforming each item in a collection using a provided function. Rambda's `map` function allows you to apply a function to each element in an array or object, returning a new array or object with the transformed elements.
const R = require('rambda');
const double = n => n * 2;
const numbers = [1, 2, 3, 4, 5];
const doubledNumbers = R.map(double, numbers);
console.log(doubledNumbers); // [2, 4, 6, 8, 10]
Lodash is a popular utility library that provides a wide range of functions for common programming tasks. It is more feature-rich compared to Rambda but also larger in size. Lodash focuses on performance and ease of use, offering a comprehensive set of tools for working with arrays, objects, strings, and more.
Underscore is another utility library that provides a variety of functional programming helpers. It is similar to Lodash but with a smaller footprint and fewer features. Underscore offers a core set of functions for working with collections, arrays, objects, and functions, making it a good choice for projects that need a lightweight utility library.
Ramda is a functional programming library for JavaScript that emphasizes immutability and pure functions. It offers a similar API to Rambda but with a larger set of functions and a focus on functional programming principles. Ramda is more feature-rich but also larger in size compared to Rambda.
Rambda
is smaller and faster alternative to the popular functional programming library Ramda. - Documentation
import { compose, map, filter } from 'rambda'
const result = compose(
map(x => x * 2),
filter(x => x > 2)
)([1, 2, 3, 4])
// => [6, 8]
You can test this example in Rambda's REPL
TypeScript definitions are included in the library, in comparison to Ramda, where you need to additionally install @types/ramda
.
Still, you need to be aware that functional programming features in TypeScript
are in development, which means that using R.compose/R.pipe can be problematic.
Important - Rambda version 7.1.0
(or higher) requires TypeScript version 4.3.3
(or higher).
Ramda
uses a lot of internals, which hides a lot of logic. Reading the full source code of a method can be challenging.
If the project is written in Javascript, then go to source definition
action will lead you to actual implementation of the method.
You can use immutable version of Rambda definitions, which is linted with ESLint functional/prefer-readonly-type
plugin.
import {add} from 'rambda/immutable'
Latest version of Ramba available for Deno
users is 3 years old. This is not the case with Rambda as most of recent releases are available for Deno
users.
Also, Rambda
provides you with included TS definitions:
// Deno extension(https://marketplace.visualstudio.com/items?itemName=denoland.vscode-deno)
// is installed and initialized
import * as R from "https://deno.land/x/rambda/mod.ts";
import * as Ramda from "https://deno.land/x/ramda/mod.ts";
R.add(1)('foo') // => will trigger warning in VSCode as it should
Ramda.add(1)('foo') // => will not trigger warning in VSCode
R.path
, R.paths
, R.assocPath
and R.lensPath
Standard usage of R.path
is R.path(['a', 'b'], {a: {b: 1} })
.
In Rambda you have the choice to use dot notation(which is arguably more readable):
R.path('a.b', {a: {b: 1} })
R.pick
and R.omit
Similar to dot notation, but the separator is comma(,
) instead of dot(.
).
R.pick('a,b', {a: 1 , b: 2, c: 3} })
// No space allowed between properties
Rambda is generally more performant than Ramda
as the benchmarks can prove that.
One of the main issues with Ramda
is the slow process of releasing new versions. This is not the case with Rambda as releases are made on regular basis.
into
invert
invertObj
invoker
keysIn
lift
liftN
mapAccum
mapAccumRight
memoizeWith
mergeDeepWith
mergeDeepWithKey
mergeWithKey
nAry
nthArg
o
otherwise
pair
partialRight
pathSatisfies
pipeWith
project
promap
reduceRight
reduceWhile
reduced
remove
scan
sequence
splitWhenever
symmetricDifferenceWith
andThen
toPairsIn
unary
uncurryN
unfold
unionWith
until
useWith
valuesIn
xprod
thunkify
default
Most of above methods are in progress to be added to Rambda. The following methods are not going to be added:
__ - placeholder method allows user to further customize the method call. While, it seems useful initially, the price is too high in terms of complexity for TypeScript definitions. If it is not easy exressable in TypeScript, it is not worth it as Rambda is a TypeScript first library.
construct - Using classes is not very functional programming oriented.
constructN - same as above
transduce - currently is out of focus
traverse - same as above
yarn add rambda
For UMD usage either use ./dist/rambda.umd.js
or the following CDN link:
https://unpkg.com/rambda@CURRENT_VERSION/dist/rambda.umd.js
import {add} from "https://deno.land/x/rambda/mod.ts";
Rambda's type detects async functions and unresolved Promises
. The returned values are 'Async'
and 'Promise'
.
Rambda's type handles NaN input, in which case it returns NaN
.
Rambda's forEach can iterate over objects not only arrays.
Rambda's map, filter, partition when they iterate over objects, they pass property and input object as predicate's argument.
Rambda's filter returns empty array with bad input(null
or undefined
), while Ramda throws.
Ramda's clamp work with strings, while Rambda's method work only with numbers.
Ramda's indexOf/lastIndexOf work with strings and lists, while Rambda's method work only with lists as iterable input.
Error handling, when wrong inputs are provided, may not be the same. This difference will be better documented once all brute force tests are completed.
TypeScript definitions between rambda
and @types/ramda
may vary.
If you need more Ramda methods in Rambda, you may either submit a
PR
or check the extended version of Rambda - Rambdax. In case of the former, you may want to consult with Rambda contribution guidelines.
There are methods which are benchmarked only with Ramda
and Rambda
(i.e. no Lodash
).
Note that some of these methods, are called with and without curring. This is done in order to give more detailed performance feedback.
The benchmarks results are produced from latest versions of Rambda, Lodash(4.17.21) and Ramda(0.29.1).
method | Rambda | Ramda | Lodash |
---|---|---|---|
add | 🚀 Fastest | 21.52% slower | 82.15% slower |
adjust | 8.48% slower | 🚀 Fastest | 🔳 |
all | 🚀 Fastest | 7.18% slower | 🔳 |
allPass | 🚀 Fastest | 88.25% slower | 🔳 |
allPass | 🚀 Fastest | 98.56% slower | 🔳 |
and | 🚀 Fastest | 89.09% slower | 🔳 |
any | 🚀 Fastest | 92.87% slower | 45.82% slower |
anyPass | 🚀 Fastest | 98.25% slower | 🔳 |
append | 🚀 Fastest | 2.07% slower | 🔳 |
applySpec | 🚀 Fastest | 80.43% slower | 🔳 |
assoc | 72.32% slower | 60.08% slower | 🚀 Fastest |
clone | 🚀 Fastest | 91.86% slower | 86.48% slower |
compose | 6.07% slower | 16.89% slower | 🚀 Fastest |
converge | 78.63% slower | 🚀 Fastest | 🔳 |
curry | 🚀 Fastest | 28.86% slower | 🔳 |
curryN | 🚀 Fastest | 41.05% slower | 🔳 |
defaultTo | 🚀 Fastest | 48.91% slower | 🔳 |
drop | 🚀 Fastest | 82.35% slower | 🔳 |
dropLast | 🚀 Fastest | 86.74% slower | 🔳 |
equals | 58.37% slower | 96.73% slower | 🚀 Fastest |
filter | 6.7% slower | 72.03% slower | 🚀 Fastest |
find | 🚀 Fastest | 85.14% slower | 42.65% slower |
findIndex | 🚀 Fastest | 86.48% slower | 72.27% slower |
flatten | 🚀 Fastest | 85.68% slower | 3.57% slower |
ifElse | 🚀 Fastest | 58.56% slower | 🔳 |
includes | 🚀 Fastest | 81.64% slower | 🔳 |
indexOf | 🚀 Fastest | 80.17% slower | 🔳 |
indexOf | 🚀 Fastest | 82.2% slower | 🔳 |
init | 🚀 Fastest | 92.24% slower | 13.3% slower |
is | 🚀 Fastest | 57.69% slower | 🔳 |
isEmpty | 🚀 Fastest | 97.14% slower | 54.99% slower |
last | 🚀 Fastest | 93.43% slower | 5.28% slower |
lastIndexOf | 🚀 Fastest | 85.19% slower | 🔳 |
map | 🚀 Fastest | 86.6% slower | 11.73% slower |
match | 🚀 Fastest | 44.83% slower | 🔳 |
merge | 🚀 Fastest | 12.21% slower | 55.76% slower |
none | 🚀 Fastest | 96.48% slower | 🔳 |
objOf | 🚀 Fastest | 38.05% slower | 🔳 |
omit | 🚀 Fastest | 69.95% slower | 97.34% slower |
over | 🚀 Fastest | 56.23% slower | 🔳 |
path | 37.81% slower | 77.81% slower | 🚀 Fastest |
pick | 🚀 Fastest | 19.07% slower | 80.2% slower |
pipe | 🚀 Fastest | 0.11% slower | 🔳 |
prop | 🚀 Fastest | 87.95% slower | 🔳 |
propEq | 🚀 Fastest | 91.92% slower | 🔳 |
range | 🚀 Fastest | 61.8% slower | 57.44% slower |
reduce | 60.48% slower | 77.1% slower | 🚀 Fastest |
repeat | 48.57% slower | 68.98% slower | 🚀 Fastest |
replace | 33.45% slower | 33.99% slower | 🚀 Fastest |
set | 🚀 Fastest | 50.35% slower | 🔳 |
sort | 🚀 Fastest | 40.23% slower | 🔳 |
sortBy | 🚀 Fastest | 25.29% slower | 56.88% slower |
split | 🚀 Fastest | 55.37% slower | 17.64% slower |
splitEvery | 🚀 Fastest | 71.98% slower | 🔳 |
take | 🚀 Fastest | 91.96% slower | 4.72% slower |
takeLast | 🚀 Fastest | 93.39% slower | 19.22% slower |
test | 🚀 Fastest | 82.34% slower | 🔳 |
type | 🚀 Fastest | 48.6% slower | 🔳 |
uniq | 🚀 Fastest | 84.9% slower | 🔳 |
uniqBy | 51.93% slower | 🚀 Fastest | 🔳 |
uniqWith | 8.29% slower | 🚀 Fastest | 🔳 |
uniqWith | 14.23% slower | 🚀 Fastest | 🔳 |
update | 🚀 Fastest | 52.35% slower | 🔳 |
view | 🚀 Fastest | 76.15% slower | 🔳 |
Walmart Canada reported by w-b-dev
It adds a
and b
.
Try this R.add example in Rambda REPL
Try this R.addIndex example in Rambda REPL
Same as R.addIndex
, but it will passed indexes are decreasing, instead of increasing.
adjust<T>(index: number, replaceFn: (x: T) => T, list: T[]): T[]
It replaces index
in array list
with the result of replaceFn(list[i])
.
Try this R.adjust example in Rambda REPL
adjust<T>(index: number, replaceFn: (x: T) => T, list: T[]): T[];
adjust<T>(index: number, replaceFn: (x: T) => T): (list: T[]) => T[];
import { cloneList } from './_internals/cloneList.js'
import { curry } from './curry.js'
function adjustFn(
index, replaceFn, list
){
const actualIndex = index < 0 ? list.length + index : index
if (index >= list.length || actualIndex < 0) return list
const clone = cloneList(list)
clone[ actualIndex ] = replaceFn(clone[ actualIndex ])
return clone
}
export const adjust = curry(adjustFn)
import { add } from './add.js'
import { adjust } from './adjust.js'
import { pipe } from './pipe.js'
const list = [ 0, 1, 2 ]
const expected = [ 0, 11, 2 ]
test('happy', () => {})
test('happy', () => {
expect(adjust(
1, add(10), list
)).toEqual(expected)
})
test('with curring type 1 1 1', () => {
expect(adjust(1)(add(10))(list)).toEqual(expected)
})
test('with curring type 1 2', () => {
expect(adjust(1)(add(10), list)).toEqual(expected)
})
test('with curring type 2 1', () => {
expect(adjust(1, add(10))(list)).toEqual(expected)
})
test('with negative index', () => {
expect(adjust(
-2, add(10), list
)).toEqual(expected)
})
test('when index is out of bounds', () => {
const list = [ 0, 1, 2, 3 ]
expect(adjust(
4, add(1), list
)).toEqual(list)
expect(adjust(
-5, add(1), list
)).toEqual(list)
})
all<T>(predicate: (x: T) => boolean, list: T[]): boolean
It returns true
, if all members of array list
returns true
, when applied as argument to predicate
function.
Try this R.all example in Rambda REPL
all<T>(predicate: (x: T) => boolean, list: T[]): boolean;
all<T>(predicate: (x: T) => boolean): (list: T[]) => boolean;
export function all(predicate, list){
if (arguments.length === 1) return _list => all(predicate, _list)
for (let i = 0; i < list.length; i++){
if (!predicate(list[ i ])) return false
}
return true
}
import { all } from './all.js'
const list = [ 0, 1, 2, 3, 4 ]
test('when true', () => {
const fn = x => x > -1
expect(all(fn)(list)).toBeTrue()
})
test('when false', () => {
const fn = x => x > 2
expect(all(fn, list)).toBeFalse()
})
import {all} from 'rambda'
describe('all', () => {
it('happy', () => {
const result = all(
x => {
x // $ExpectType number
return x > 0
},
[1, 2, 3]
)
result // $ExpectType boolean
})
it('curried needs a type', () => {
const result = all<number>(x => {
x // $ExpectType number
return x > 0
})([1, 2, 3])
result // $ExpectType boolean
})
})
allPass<T>(predicates: ((x: T) => boolean)[]): (input: T) => boolean
It returns true
, if all functions of predicates
return true
, when input
is their argument.
Try this R.allPass example in Rambda REPL
allPass<T>(predicates: ((x: T) => boolean)[]): (input: T) => boolean;
allPass<T>(predicates: ((...inputs: T[]) => boolean)[]): (...inputs: T[]) => boolean;
export function allPass(predicates){
return (...input) => {
let counter = 0
while (counter < predicates.length){
if (!predicates[ counter ](...input)){
return false
}
counter++
}
return true
}
}
import { allPass } from './allPass.js'
test('happy', () => {
const rules = [ x => typeof x === 'number', x => x > 10, x => x * 7 < 100 ]
expect(allPass(rules)(11)).toBeTrue()
expect(allPass(rules)(undefined)).toBeFalse()
})
test('when returns true', () => {
const conditionArr = [ val => val.a === 1, val => val.b === 2 ]
expect(allPass(conditionArr)({
a : 1,
b : 2,
})).toBeTrue()
})
test('when returns false', () => {
const conditionArr = [ val => val.a === 1, val => val.b === 3 ]
expect(allPass(conditionArr)({
a : 1,
b : 2,
})).toBeFalse()
})
test('works with multiple inputs', () => {
const fn = function (
w, x, y, z
){
return w + x === y + z
}
expect(allPass([ fn ])(
3, 3, 3, 3
)).toBeTrue()
})
import {allPass, filter} from 'rambda'
describe('allPass', () => {
it('happy', () => {
const x = allPass<number>([
y => {
y // $ExpectType number
return typeof y === 'number'
},
y => {
return y > 0
},
])(11)
x // $ExpectType boolean
})
it('issue #642', () => {
const isGreater = (num: number) => num > 5
const pred = allPass([isGreater])
const xs = [0, 1, 2, 3]
const filtered1 = filter(pred)(xs)
filtered1 // $ExpectType number[]
const filtered2 = xs.filter(pred)
filtered2 // $ExpectType number[]
})
it('issue #604', () => {
const plusEq = function(w: number, x: number, y: number, z: number) {
return w + x === y + z
}
const result = allPass([plusEq])(3, 3, 3, 3)
result // $ExpectType boolean
})
})
It returns function that always returns x
.
Try this R.always example in Rambda REPL
Logical AND
Try this R.and example in Rambda REPL
any<T>(predicate: (x: T) => boolean, list: T[]): boolean
It returns true
, if at least one member of list
returns true, when passed to a predicate
function.
Try this R.any example in Rambda REPL
any<T>(predicate: (x: T) => boolean, list: T[]): boolean;
any<T>(predicate: (x: T) => boolean): (list: T[]) => boolean;
export function any(predicate, list){
if (arguments.length === 1) return _list => any(predicate, _list)
let counter = 0
while (counter < list.length){
if (predicate(list[ counter ], counter)){
return true
}
counter++
}
return false
}
import { any } from './any.js'
const list = [ 1, 2, 3 ]
test('happy', () => {
expect(any(x => x < 0, list)).toBeFalse()
})
test('with curry', () => {
expect(any(x => x > 2)(list)).toBeTrue()
})
import {any} from 'rambda'
describe('R.any', () => {
it('happy', () => {
const result = any(
x => {
x // $ExpectType number
return x > 2
},
[1, 2, 3]
)
result // $ExpectType boolean
})
it('when curried needs a type', () => {
const result = any<number>(x => {
x // $ExpectType number
return x > 2
})([1, 2, 3])
result // $ExpectType boolean
})
})
anyPass<T>(predicates: ((x: T) => boolean)[]): (input: T) => boolean
It accepts list of predicates
and returns a function. This function with its input
will return true
, if any of predicates
returns true
for this input
.
Try this R.anyPass example in Rambda REPL
anyPass<T>(predicates: ((x: T) => boolean)[]): (input: T) => boolean;
anyPass<T>(predicates: ((...inputs: T[]) => boolean)[]): (...inputs: T[]) => boolean;
export function anyPass(predicates){
return (...input) => {
let counter = 0
while (counter < predicates.length){
if (predicates[ counter ](...input)){
return true
}
counter++
}
return false
}
}
import { anyPass } from './anyPass.js'
test('happy', () => {
const rules = [ x => typeof x === 'string', x => x > 10 ]
const predicate = anyPass(rules)
expect(predicate('foo')).toBeTrue()
expect(predicate(6)).toBeFalse()
})
test('happy', () => {
const rules = [ x => typeof x === 'string', x => x > 10 ]
expect(anyPass(rules)(11)).toBeTrue()
expect(anyPass(rules)(undefined)).toBeFalse()
})
const obj = {
a : 1,
b : 2,
}
test('when returns true', () => {
const conditionArr = [ val => val.a === 1, val => val.a === 2 ]
expect(anyPass(conditionArr)(obj)).toBeTrue()
})
test('when returns false + curry', () => {
const conditionArr = [ val => val.a === 2, val => val.b === 3 ]
expect(anyPass(conditionArr)(obj)).toBeFalse()
})
test('with empty predicates list', () => {
expect(anyPass([])(3)).toBeFalse()
})
test('works with multiple inputs', () => {
const fn = function (
w, x, y, z
){
console.log(
w, x, y, z
)
return w + x === y + z
}
expect(anyPass([ fn ])(
3, 3, 3, 3
)).toBeTrue()
})
import {anyPass, filter} from 'rambda'
describe('anyPass', () => {
it('happy', () => {
const x = anyPass<number>([
y => {
y // $ExpectType number
return typeof y === 'number'
},
y => {
return y > 0
},
])(11)
x // $ExpectType boolean
})
it('issue #604', () => {
const plusEq = function(w: number, x: number, y: number, z: number) {
return w + x === y + z
}
const result = anyPass([plusEq])(3, 3, 3, 3)
result // $ExpectType boolean
})
it('issue #642', () => {
const isGreater = (num: number) => num > 5
const pred = anyPass([isGreater])
const xs = [0, 1, 2, 3]
const filtered1 = filter(pred)(xs)
filtered1 // $ExpectType number[]
const filtered2 = xs.filter(pred)
filtered2 // $ExpectType number[]
})
it('functions as a type guard', () => {
const isString = (x: unknown): x is string => typeof x === 'string'
const isNumber = (x: unknown): x is number => typeof x === 'number'
const isBoolean = (x: unknown): x is boolean => typeof x === 'boolean'
const isStringNumberOrBoolean = anyPass([isString, isNumber, isBoolean])
isStringNumberOrBoolean // $ExpectType (input: unknown) => boolean
const aValue: unknown = 1
if (isStringNumberOrBoolean(aValue)) {
aValue // $ExpectType unknown
}
})
})
ap<T, U>(fns: Array<(a: T) => U>[], vs: T[]): U[]
It takes a list of functions and a list of values. Then it returns a list of values obtained by applying each function to each value.
Try this R.ap example in Rambda REPL
ap<T, U>(fns: Array<(a: T) => U>[], vs: T[]): U[];
ap<T, U>(fns: Array<(a: T) => U>): (vs: T[]) => U[];
ap<R, A, B>(fn: (r: R, a: A) => B, fn1: (r: R) => A): (r: R) => B;
export function ap(functions, input){
if (arguments.length === 1){
return _inputs => ap(functions, _inputs)
}
return functions.reduce((acc, fn) => [ ...acc, ...input.map(fn) ], [])
}
import { ap } from './ap.js'
function mult2(x){
return x * 2
}
function plus3(x){
return x + 3
}
test('happy', () => {
expect(ap([ mult2, plus3 ], [ 1, 2, 3 ])).toEqual([ 2, 4, 6, 4, 5, 6 ])
})
aperture<N extends number, T>(n: N, list: T[]): Array<Tuple<T, N>> | []
It returns a new list, composed of consecutive n
-tuples from a list
.
Try this R.aperture example in Rambda REPL
aperture<N extends number, T>(n: N, list: T[]): Array<Tuple<T, N>> | [];
aperture<N extends number>(n: N): <T>(list: T[]) => Array<Tuple<T, N>> | [];
export function aperture(step, list){
if (arguments.length === 1){
return _list => aperture(step, _list)
}
if (step > list.length) return []
let idx = 0
const limit = list.length - (step - 1)
const acc = new Array(limit)
while (idx < limit){
acc[ idx ] = list.slice(idx, idx + step)
idx += 1
}
return acc
}
import { aperture } from './aperture.js'
const list = [ 1, 2, 3, 4, 5, 6, 7 ]
test('happy', () => {
expect(aperture(1, list)).toEqual([ [ 1 ], [ 2 ], [ 3 ], [ 4 ], [ 5 ], [ 6 ], [ 7 ] ])
expect(aperture(2, list)).toEqual([
[ 1, 2 ],
[ 2, 3 ],
[ 3, 4 ],
[ 4, 5 ],
[ 5, 6 ],
[ 6, 7 ],
])
expect(aperture(3, list)).toEqual([
[ 1, 2, 3 ],
[ 2, 3, 4 ],
[ 3, 4, 5 ],
[ 4, 5, 6 ],
[ 5, 6, 7 ],
])
expect(aperture(8, list)).toEqual([])
})
append<T>(xToAppend: T, iterable: T[]): T[]
It adds element x
at the end of iterable
.
Try this R.append example in Rambda REPL
append<T>(xToAppend: T, iterable: T[]): T[];
append<T, U>(xToAppend: T, iterable: IsFirstSubtypeOfSecond<T, U>[]) : U[];
append<T>(xToAppend: T): <U>(iterable: IsFirstSubtypeOfSecond<T, U>[]) => U[];
append<T>(xToAppend: T): (iterable: T[]) => T[];
import { cloneList } from './_internals/cloneList.js'
export function append(x, input){
if (arguments.length === 1) return _input => append(x, _input)
if (typeof input === 'string') return input.split('').concat(x)
const clone = cloneList(input)
clone.push(x)
return clone
}
import { append } from './append.js'
test('happy', () => {
expect(append('tests', [ 'write', 'more' ])).toEqual([
'write',
'more',
'tests',
])
})
test('append to empty array', () => {
expect(append('tests')([])).toEqual([ 'tests' ])
})
test('with strings', () => {
expect(append('o', 'fo')).toEqual([ 'f', 'o', 'o' ])
})
import {append, prepend} from 'rambda'
const listOfNumbers = [1, 2, 3]
const listOfNumbersAndStrings = [1, 'b', 3]
describe('R.append/R.prepend', () => {
describe("with the same primitive type as the array's elements", () => {
it('uncurried', () => {
// @ts-expect-error
append('d', listOfNumbers)
// @ts-expect-error
prepend('d', listOfNumbers)
append(4, listOfNumbers) // $ExpectType number[]
prepend(4, listOfNumbers) // $ExpectType number[]
})
it('curried', () => {
// @ts-expect-error
append('d')(listOfNumbers)
append(4)(listOfNumbers) // $ExpectType number[]
prepend(4)(listOfNumbers) // $ExpectType number[]
})
})
describe("with a subtype of the array's elements", () => {
it('uncurried', () => {
// @ts-expect-error
append(true, listOfNumbersAndStrings)
append(4, listOfNumbersAndStrings) // $ExpectType (string | number)[]
prepend(4, listOfNumbersAndStrings) // $ExpectType (string | number)[]
})
it('curried', () => {
// @ts-expect-error
append(true)(listOfNumbersAndStrings)
append(4)(listOfNumbersAndStrings) // $ExpectType (string | number)[]
prepend(4)(listOfNumbersAndStrings) // $ExpectType (string | number)[]
})
})
describe("expanding the type of the array's elements", () => {
it('uncurried', () => {
// @ts-expect-error
append('d', listOfNumbers)
append<string | number>('d', listOfNumbers) // $ExpectType (string | number)[]
prepend<string | number>('d', listOfNumbers) // $ExpectType (string | number)[]
})
it('curried', () => {
// @ts-expect-error
append('d')(listOfNumbers)
const appendD = append('d')
appendD<string | number>(listOfNumbers) // $ExpectType (string | number)[]
const prependD = prepend('d')
prependD<string | number>(listOfNumbers) // $ExpectType (string | number)[]
})
})
})
apply<T = any>(fn: (...args: any[]) => T, args: any[]): T
It applies function fn
to the list of arguments.
This is useful for creating a fixed-arity function from a variadic function. fn
should be a bound function if context is significant.
Try this R.apply example in Rambda REPL
apply<T = any>(fn: (...args: any[]) => T, args: any[]): T;
apply<T = any>(fn: (...args: any[]) => T): (args: any[]) => T;
export function apply(fn, args){
if (arguments.length === 1){
return _args => apply(fn, _args)
}
return fn.apply(this, args)
}
import { apply } from './apply.js'
import { bind } from './bind.js'
import { identity } from './identity.js'
test('happy', () => {
expect(apply(identity, [ 1, 2, 3 ])).toBe(1)
})
test('applies function to argument list', () => {
expect(apply(Math.max, [ 1, 2, 3, -99, 42, 6, 7 ])).toBe(42)
})
test('provides no way to specify context', () => {
const obj = {
method (){
return this === obj
},
}
expect(apply(obj.method, [])).toBeFalse()
expect(apply(bind(obj.method, obj), [])).toBeTrue()
})
import {apply, identity} from 'rambda'
describe('R.apply', () => {
it('happy', () => {
const result = apply<number>(identity, [1, 2, 3])
result // $ExpectType number
})
it('curried', () => {
const fn = apply<number>(identity)
const result = fn([1, 2, 3])
result // $ExpectType number
})
})
applySpec<Spec extends Record<string, AnyFunction>>(
spec: Spec
): (
...args: Parameters<ValueOfRecord<Spec>>
) => { [Key in keyof Spec]: ReturnType<Spec[Key]> }
Try this R.applySpec example in Rambda REPL
applySpec<Spec extends Record<string, AnyFunction>>(
spec: Spec
): (
...args: Parameters<ValueOfRecord<Spec>>
) => { [Key in keyof Spec]: ReturnType<Spec[Key]> };
applySpec<T>(spec: any): (...args: unknown[]) => T;
import { isArray } from './_internals/isArray.js'
// recursively traverse the given spec object to find the highest arity function
export function __findHighestArity(spec, max = 0){
for (const key in spec){
if (spec.hasOwnProperty(key) === false || key === 'constructor') continue
if (typeof spec[ key ] === 'object'){
max = Math.max(max, __findHighestArity(spec[ key ]))
}
if (typeof spec[ key ] === 'function'){
max = Math.max(max, spec[ key ].length)
}
}
return max
}
function __filterUndefined(){
const defined = []
let i = 0
const l = arguments.length
while (i < l){
if (typeof arguments[ i ] === 'undefined') break
defined[ i ] = arguments[ i ]
i++
}
return defined
}
function __applySpecWithArity(
spec, arity, cache
){
const remaining = arity - cache.length
if (remaining === 1)
return x =>
__applySpecWithArity(
spec, arity, __filterUndefined(...cache, x)
)
if (remaining === 2)
return (x, y) =>
__applySpecWithArity(
spec, arity, __filterUndefined(
...cache, x, y
)
)
if (remaining === 3)
return (
x, y, z
) =>
__applySpecWithArity(
spec, arity, __filterUndefined(
...cache, x, y, z
)
)
if (remaining === 4)
return (
x, y, z, a
) =>
__applySpecWithArity(
spec,
arity,
__filterUndefined(
...cache, x, y, z, a
)
)
if (remaining > 4)
return (...args) =>
__applySpecWithArity(
spec, arity, __filterUndefined(...cache, ...args)
)
// handle spec as Array
if (isArray(spec)){
const ret = []
let i = 0
const l = spec.length
for (; i < l; i++){
// handle recursive spec inside array
if (typeof spec[ i ] === 'object' || isArray(spec[ i ])){
ret[ i ] = __applySpecWithArity(
spec[ i ], arity, cache
)
}
// apply spec to the key
if (typeof spec[ i ] === 'function'){
ret[ i ] = spec[ i ](...cache)
}
}
return ret
}
// handle spec as Object
const ret = {}
// apply callbacks to each property in the spec object
for (const key in spec){
if (spec.hasOwnProperty(key) === false || key === 'constructor') continue
// apply the spec recursively
if (typeof spec[ key ] === 'object'){
ret[ key ] = __applySpecWithArity(
spec[ key ], arity, cache
)
continue
}
// apply spec to the key
if (typeof spec[ key ] === 'function'){
ret[ key ] = spec[ key ](...cache)
}
}
return ret
}
export function applySpec(spec, ...args){
// get the highest arity spec function, cache the result and pass to __applySpecWithArity
const arity = __findHighestArity(spec)
if (arity === 0){
return () => ({})
}
const toReturn = __applySpecWithArity(
spec, arity, args
)
return toReturn
}
import { applySpec as applySpecRamda, nAry } from 'ramda'
import {
add,
always,
compose,
dec,
inc,
map,
path,
prop,
T,
} from '../rambda.js'
import { applySpec } from './applySpec.js'
test('different than Ramda when bad spec', () => {
const result = applySpec({ sum : { a : 1 } })(1, 2)
const ramdaResult = applySpecRamda({ sum : { a : 1 } })(1, 2)
expect(result).toEqual({})
expect(ramdaResult).toEqual({ sum : { a : {} } })
})
test('works with empty spec', () => {
expect(applySpec({})()).toEqual({})
expect(applySpec([])(1, 2)).toEqual({})
expect(applySpec(null)(1, 2)).toEqual({})
})
test('works with unary functions', () => {
const result = applySpec({
v : inc,
u : dec,
})(1)
const expected = {
v : 2,
u : 0,
}
expect(result).toEqual(expected)
})
test('works with binary functions', () => {
const result = applySpec({ sum : add })(1, 2)
expect(result).toEqual({ sum : 3 })
})
test('works with nested specs', () => {
const result = applySpec({
unnested : always(0),
nested : { sum : add },
})(1, 2)
const expected = {
unnested : 0,
nested : { sum : 3 },
}
expect(result).toEqual(expected)
})
test('works with arrays of nested specs', () => {
const result = applySpec({
unnested : always(0),
nested : [ { sum : add } ],
})(1, 2)
expect(result).toEqual({
unnested : 0,
nested : [ { sum : 3 } ],
})
})
test('works with arrays of spec objects', () => {
const result = applySpec([ { sum : add } ])(1, 2)
expect(result).toEqual([ { sum : 3 } ])
})
test('works with arrays of functions', () => {
const result = applySpec([ map(prop('a')), map(prop('b')) ])([
{
a : 'a1',
b : 'b1',
},
{
a : 'a2',
b : 'b2',
},
])
const expected = [
[ 'a1', 'a2' ],
[ 'b1', 'b2' ],
]
expect(result).toEqual(expected)
})
test('works with a spec defining a map key', () => {
expect(applySpec({ map : prop('a') })({ a : 1 })).toEqual({ map : 1 })
})
test('cannot retains the highest arity', () => {
const f = applySpec({
f1 : nAry(2, T),
f2 : nAry(5, T),
})
const fRamda = applySpecRamda({
f1 : nAry(2, T),
f2 : nAry(5, T),
})
expect(f).toHaveLength(0)
expect(fRamda).toHaveLength(5)
})
test('returns a curried function', () => {
expect(applySpec({ sum : add })(1)(2)).toEqual({ sum : 3 })
})
// Additional tests
// ============================================
test('arity', () => {
const spec = {
one : x1 => x1,
two : (x1, x2) => x1 + x2,
three : (
x1, x2, x3
) => x1 + x2 + x3,
}
expect(applySpec(
spec, 1, 2, 3
)).toEqual({
one : 1,
two : 3,
three : 6,
})
})
test('arity over 5 arguments', () => {
const spec = {
one : x1 => x1,
two : (x1, x2) => x1 + x2,
three : (
x1, x2, x3
) => x1 + x2 + x3,
four : (
x1, x2, x3, x4
) => x1 + x2 + x3 + x4,
five : (
x1, x2, x3, x4, x5
) => x1 + x2 + x3 + x4 + x5,
}
expect(applySpec(
spec, 1, 2, 3, 4, 5
)).toEqual({
one : 1,
two : 3,
three : 6,
four : 10,
five : 15,
})
})
test('curried', () => {
const spec = {
one : x1 => x1,
two : (x1, x2) => x1 + x2,
three : (
x1, x2, x3
) => x1 + x2 + x3,
}
expect(applySpec(spec)(1)(2)(3)).toEqual({
one : 1,
two : 3,
three : 6,
})
})
test('curried over 5 arguments', () => {
const spec = {
one : x1 => x1,
two : (x1, x2) => x1 + x2,
three : (
x1, x2, x3
) => x1 + x2 + x3,
four : (
x1, x2, x3, x4
) => x1 + x2 + x3 + x4,
five : (
x1, x2, x3, x4, x5
) => x1 + x2 + x3 + x4 + x5,
}
expect(applySpec(spec)(1)(2)(3)(4)(5)).toEqual({
one : 1,
two : 3,
three : 6,
four : 10,
five : 15,
})
})
test('undefined property', () => {
const spec = { prop : path([ 'property', 'doesnt', 'exist' ]) }
expect(applySpec(spec, {})).toEqual({ prop : undefined })
})
test('restructure json object', () => {
const spec = {
id : path('user.id'),
name : path('user.firstname'),
profile : path('user.profile'),
doesntExist : path('user.profile.doesntExist'),
info : { views : compose(inc, prop('views')) },
type : always('playa'),
}
const data = {
user : {
id : 1337,
firstname : 'john',
lastname : 'shaft',
profile : 'shaft69',
},
views : 42,
}
expect(applySpec(spec, data)).toEqual({
id : 1337,
name : 'john',
profile : 'shaft69',
doesntExist : undefined,
info : { views : 43 },
type : 'playa',
})
})
import {multiply, applySpec, inc, dec, add} from 'rambda'
describe('applySpec', () => {
it('ramda 1', () => {
const result = applySpec({
v: inc,
u: dec,
})(1)
result // $ExpectType { v: number; u: number; }
})
it('ramda 1', () => {
interface Output {
sum: number,
multiplied: number,
}
const result = applySpec<Output>({
sum: add,
multiplied: multiply,
})(1, 2)
result // $ExpectType Output
})
})
Try this R.applyTo example in Rambda REPL
Try this R.ascend example in Rambda REPL
It makes a shallow clone of obj
with setting or overriding the property prop
with newValue
.
Try this R.assoc example in Rambda REPL
assocPath<Output>(path: Path, newValue: any, obj: object): Output
It makes a shallow clone of obj
with setting or overriding with newValue
the property found with path
.
Try this R.assocPath example in Rambda REPL
assocPath<Output>(path: Path, newValue: any, obj: object): Output;
assocPath<Output>(path: Path, newValue: any): (obj: object) => Output;
assocPath<Output>(path: Path): (newValue: any) => (obj: object) => Output;
import { cloneList } from './_internals/cloneList.js'
import { createPath } from './_internals/createPath.js'
import { isArray } from './_internals/isArray.js'
import { isIndexInteger } from './_internals/isInteger.js'
import { assocFn } from './assoc.js'
import { curry } from './curry.js'
export function assocPathFn(
path, newValue, input
){
const pathArrValue = createPath(path)
if (pathArrValue.length === 0) return newValue
const index = pathArrValue[ 0 ]
if (pathArrValue.length > 1){
const condition =
typeof input !== 'object' ||
input === null ||
!input.hasOwnProperty(index)
const nextInput = condition ?
isIndexInteger(pathArrValue[ 1 ]) ?
[] :
{} :
input[ index ]
newValue = assocPathFn(
Array.prototype.slice.call(pathArrValue, 1),
newValue,
nextInput
)
}
if (isIndexInteger(index) && isArray(input)){
const arr = cloneList(input)
arr[ index ] = newValue
return arr
}
return assocFn(
index, newValue, input
)
}
export const assocPath = curry(assocPathFn)
import { assocPathFn } from './assocPath.js'
test.only('happy', () => {
const path = 'a.c.1'
const input = {
a : {
b : 1,
c : [ 1, 2 ],
},
}
assocPathFn(
path, 3, input
)
expect(input).toEqual({
a : {
b : 1,
c : [ 1, 2 ],
},
})
})
test('string can be used as path input', () => {
const testObj = {
a : [ { b : 1 }, { b : 2 } ],
d : 3,
}
const result1 = assocPathFn(
[ 'a', 0, 'b' ], 10, testObj
)
const result2 = assocPathFn(
'a.0.b', 10, testObj
)
const expected = {
a : [ { b : 10 }, { b : 2 } ],
d : 3,
}
expect(result1).toEqual(expected)
expect(result2).toEqual(expected)
})
test('difference with ramda - doesn\'t overwrite primitive values with keys in the path', () => {
const obj = { a : 'str' }
const result = assocPath(
[ 'a', 'b' ], 42, obj
)
expect(result).toEqual({
a : {
0 : 's',
1 : 't',
2 : 'r',
b : 42,
},
})
})
test('bug', () => {
/*
https://github.com/selfrefactor/rambda/issues/524
*/
const state = {}
const withDateLike = assocPath(
[ 'outerProp', '2020-03-10' ],
{ prop : 2 },
state
)
const withNumber = assocPath(
[ 'outerProp', '5' ], { prop : 2 }, state
)
const withDateLikeExpected = { outerProp : { '2020-03-10' : { prop : 2 } } }
const withNumberExpected = { outerProp : { 5 : { prop : 2 } } }
expect(withDateLike).toEqual(withDateLikeExpected)
expect(withNumber).toEqual(withNumberExpected)
})
test('adds a key to an empty object', () => {
expect(assocPath(
[ 'a' ], 1, {}
)).toEqual({ a : 1 })
})
test('adds a key to a non-empty object', () => {
expect(assocPath(
'b', 2, { a : 1 }
)).toEqual({
a : 1,
b : 2,
})
})
test('adds a nested key to a non-empty object', () => {
expect(assocPath(
'b.c', 2, { a : 1 }
)).toEqual({
a : 1,
b : { c : 2 },
})
})
test('adds a nested key to a nested non-empty object - curry case 1', () => {
expect(assocPath('b.d',
3)({
a : 1,
b : { c : 2 },
})).toEqual({
a : 1,
b : {
c : 2,
d : 3,
},
})
})
test('adds a key to a non-empty object - curry case 1', () => {
expect(assocPath('b', 2)({ a : 1 })).toEqual({
a : 1,
b : 2,
})
})
test('adds a nested key to a non-empty object - curry case 1', () => {
expect(assocPath('b.c', 2)({ a : 1 })).toEqual({
a : 1,
b : { c : 2 },
})
})
test('adds a key to a non-empty object - curry case 2', () => {
expect(assocPath('b')(2, { a : 1 })).toEqual({
a : 1,
b : 2,
})
})
test('adds a key to a non-empty object - curry case 3', () => {
const result = assocPath('b')(2)({ a : 1 })
expect(result).toEqual({
a : 1,
b : 2,
})
})
test('changes an existing key', () => {
expect(assocPath(
'a', 2, { a : 1 }
)).toEqual({ a : 2 })
})
test('undefined is considered an empty object', () => {
expect(assocPath(
'a', 1, undefined
)).toEqual({ a : 1 })
})
test('null is considered an empty object', () => {
expect(assocPath(
'a', 1, null
)).toEqual({ a : 1 })
})
test('value can be null', () => {
expect(assocPath(
'a', null, null
)).toEqual({ a : null })
})
test('value can be undefined', () => {
expect(assocPath(
'a', undefined, null
)).toEqual({ a : undefined })
})
test('assignment is shallow', () => {
expect(assocPath(
'a', { b : 2 }, { a : { c : 3 } }
)).toEqual({ a : { b : 2 } })
})
test('empty array as path', () => {
const result = assocPath(
[], 3, {
a : 1,
b : 2,
}
)
expect(result).toBe(3)
})
test('happy', () => {
const expected = { foo : { bar : { baz : 42 } } }
const result = assocPath(
[ 'foo', 'bar', 'baz' ], 42, { foo : null }
)
expect(result).toEqual(expected)
})
import {assocPath} from 'rambda'
interface Output {
a: number,
foo: {bar: number},
}
describe('R.assocPath - user must explicitly set type of output', () => {
it('with array as path input', () => {
const result = assocPath<Output>(['foo', 'bar'], 2, {a: 1})
result // $ExpectType Output
})
it('with string as path input', () => {
const result = assocPath<Output>('foo.bar', 2, {a: 1})
result // $ExpectType Output
})
})
describe('R.assocPath - curried', () => {
it('with array as path input', () => {
const result = assocPath<Output>(['foo', 'bar'], 2)({a: 1})
result // $ExpectType Output
})
it('with string as path input', () => {
const result = assocPath<Output>('foo.bar', 2)({a: 1})
result // $ExpectType Output
})
})
Try this R.binary example in Rambda REPL
bind<F extends AnyFunction, T>(fn: F, thisObj: T): (...args: Parameters<F>) => ReturnType<F>
Creates a function that is bound to a context.
Try this R.bind example in Rambda REPL
bind<F extends AnyFunction, T>(fn: F, thisObj: T): (...args: Parameters<F>) => ReturnType<F>;
bind<F extends AnyFunction, T>(fn: F): (thisObj: T) => (...args: Parameters<F>) => ReturnType<F>;
import { curryN } from './curryN.js'
export function bind(fn, thisObj){
if (arguments.length === 1){
return _thisObj => bind(fn, _thisObj)
}
return curryN(fn.length, (...args) => fn.apply(thisObj, args))
}
import { bind } from './bind.js'
function Foo(x){
this.x = x
}
function add(x){
return this.x + x
}
function Bar(x, y){
this.x = x
this.y = y
}
Bar.prototype = new Foo()
Bar.prototype.getX = function (){
return 'prototype getX'
}
test('returns a function', () => {
expect(typeof bind(add)(Foo)).toBe('function')
})
test('returns a function bound to the specified context object', () => {
const f = new Foo(12)
function isFoo(){
return this instanceof Foo
}
const isFooBound = bind(isFoo, f)
expect(isFoo()).toBeFalse()
expect(isFooBound()).toBeTrue()
})
test('works with built-in types', () => {
const abc = bind(String.prototype.toLowerCase, 'ABCDEFG')
expect(typeof abc).toBe('function')
expect(abc()).toBe('abcdefg')
})
test('works with user-defined types', () => {
const f = new Foo(12)
function getX(){
return this.x
}
const getXFooBound = bind(getX, f)
expect(getXFooBound()).toBe(12)
})
test('works with plain objects', () => {
const pojso = { x : 100 }
function incThis(){
return this.x + 1
}
const incPojso = bind(incThis, pojso)
expect(typeof incPojso).toBe('function')
expect(incPojso()).toBe(101)
})
test('does not interfere with existing object methods', () => {
const b = new Bar('a', 'b')
function getX(){
return this.x
}
const getXBarBound = bind(getX, b)
expect(b.getX()).toBe('prototype getX')
expect(getXBarBound()).toBe('a')
})
test('preserves arity', () => {
const f0 = function (){
return 0
}
const f1 = function (a){
return a
}
const f2 = function (a, b){
return a + b
}
const f3 = function (
a, b, c
){
return a + b + c
}
expect(bind(f0, {})).toHaveLength(0)
expect(bind(f1, {})).toHaveLength(1)
expect(bind(f2, {})).toHaveLength(2)
expect(bind(f3, {})).toHaveLength(3)
})
import {bind} from 'rambda'
class Foo {}
function isFoo<T = any>(this: T): boolean {
return this instanceof Foo
}
describe('R.bind', () => {
it('happy', () => {
const foo = new Foo()
const result = bind(isFoo, foo)()
result // $ExpectType boolean
})
})
both(pred1: Pred, pred2: Pred): Pred
It returns a function with input
argument.
This function will return true
, if both firstCondition
and secondCondition
return true
when input
is passed as their argument.
Try this R.both example in Rambda REPL
both(pred1: Pred, pred2: Pred): Pred;
both<T>(pred1: Predicate<T>, pred2: Predicate<T>): Predicate<T>;
both<T>(pred1: Predicate<T>): (pred2: Predicate<T>) => Predicate<T>;
both(pred1: Pred): (pred2: Pred) => Pred;
export function both(f, g){
if (arguments.length === 1) return _g => both(f, _g)
return (...input) => f(...input) && g(...input)
}
import { both } from './both.js'
const firstFn = val => val > 0
const secondFn = val => val < 10
test('with curry', () => {
expect(both(firstFn)(secondFn)(17)).toBeFalse()
})
test('without curry', () => {
expect(both(firstFn, secondFn)(7)).toBeTrue()
})
test('with multiple inputs', () => {
const between = function (
a, b, c
){
return a < b && b < c
}
const total20 = function (
a, b, c
){
return a + b + c === 20
}
const fn = both(between, total20)
expect(fn(
5, 7, 8
)).toBeTrue()
})
test('skip evaluation of the second expression', () => {
let effect = 'not evaluated'
const F = function (){
return false
}
const Z = function (){
effect = 'Z got evaluated'
}
both(F, Z)()
expect(effect).toBe('not evaluated')
})
import {both} from 'rambda'
describe('R.both', () => {
it('with passed type', () => {
const fn = both<number>(
x => x > 1,
x => x % 2 === 0
)
fn // $ExpectType Predicate<number>
const result = fn(2) // $ExpectType boolean
result // $ExpectType boolean
})
it('with passed type - curried', () => {
const fn = both<number>(x => x > 1)(x => x % 2 === 0)
fn // $ExpectType Predicate<number>
const result = fn(2)
result // $ExpectType boolean
})
it('no type passed', () => {
const fn = both(
x => {
x // $ExpectType any
return x > 1
},
x => {
x // $ExpectType any
return x % 2 === 0
}
)
const result = fn(2)
result // $ExpectType boolean
})
it('no type passed - curried', () => {
const fn = both((x: number) => {
x // $ExpectType number
return x > 1
})((x: number) => {
x // $ExpectType number
return x % 2 === 0
})
const result = fn(2)
result // $ExpectType boolean
})
})
Try this R.call example in Rambda REPL
chain<T, U>(fn: (n: T) => U[], list: T[]): U[]
The method is also known as flatMap
.
Try this R.chain example in Rambda REPL
chain<T, U>(fn: (n: T) => U[], list: T[]): U[];
chain<T, U>(fn: (n: T) => U[]): (list: T[]) => U[];
export function chain(fn, list){
if (arguments.length === 1){
return _list => chain(fn, _list)
}
return [].concat(...list.map(fn))
}
import { chain as chainRamda } from 'ramda'
import { chain } from './chain.js'
const duplicate = n => [ n, n ]
test('happy', () => {
const fn = x => [ x * 2 ]
const list = [ 1, 2, 3 ]
const result = chain(fn, list)
expect(result).toEqual([ 2, 4, 6 ])
})
test('maps then flattens one level', () => {
expect(chain(duplicate, [ 1, 2, 3 ])).toEqual([ 1, 1, 2, 2, 3, 3 ])
})
test('maps then flattens one level - curry', () => {
expect(chain(duplicate)([ 1, 2, 3 ])).toEqual([ 1, 1, 2, 2, 3, 3 ])
})
test('flattens only one level', () => {
const nest = n => [ [ n ] ]
expect(chain(nest, [ 1, 2, 3 ])).toEqual([ [ 1 ], [ 2 ], [ 3 ] ])
})
test('can compose', () => {
function dec(x){
return [ x - 1 ]
}
function times2(x){
return [ x * 2 ]
}
const mdouble = chain(times2)
const mdec = chain(dec)
expect(mdec(mdouble([ 10, 20, 30 ]))).toEqual([ 19, 39, 59 ])
})
test('@types/ramda broken test', () => {
const score = {
maths : 90,
physics : 80,
}
const calculateTotal = score => {
const { maths, physics } = score
return maths + physics
}
const assocTotalToScore = (total, score) => ({
...score,
total,
})
const calculateAndAssocTotalToScore = chainRamda(assocTotalToScore,
calculateTotal)
expect(() =>
calculateAndAssocTotalToScore(score)).toThrowErrorMatchingInlineSnapshot('"fn(...) is not a function"')
})
import {chain} from 'rambda'
const list = [1, 2, 3]
const fn = (x: number) => [`${x}`, `${x}`]
describe('R.chain', () => {
it('without passing type', () => {
const result = chain(fn, list)
result // $ExpectType string[]
const curriedResult = chain(fn)(list)
curriedResult // $ExpectType string[]
})
})
Restrict a number input
to be within min
and max
limits.
If input
is bigger than max
, then the result is max
.
If input
is smaller than min
, then the result is min
.
Try this R.clamp example in Rambda REPL
It creates a deep copy of the input
, which may contain (nested) Arrays and Objects, Numbers, Strings, Booleans and Dates.
Try this R.clone example in Rambda REPL
Try this R.collectBy example in Rambda REPL
It returns a comparator function that can be used in sort
method.
Try this R.comparator example in Rambda REPL
It returns inverted
version of origin
function that accept input
as argument.
The return value of inverted
is the negative boolean value of origin(input)
.
Try this R.complement example in Rambda REPL
It performs right-to-left function composition.
Try this R.compose example in Rambda REPL
Try this R.composeWith example in Rambda REPL
It returns a new string or array, which is the result of merging x
and y
.
Try this R.concat example in Rambda REPL
It takes list with conditions
and returns a new function fn
that expects input
as argument.
This function will start evaluating the conditions
in order to find the first winner(order of conditions matter).
The winner is this condition, which left side returns true
when input
is its argument. Then the evaluation of the right side of the winner will be the final result.
If no winner is found, then fn
returns undefined
.
Try this R.cond example in Rambda REPL
Accepts a converging function and a list of branching functions and returns a new function. When invoked, this new function is applied to some arguments, each branching function is applied to those same arguments. The results of each branching function are passed as arguments to the converging function to produce the return value.
Try this R.converge example in Rambda REPL
It counts how many times predicate
function returns true
, when supplied with iteration of list
.
Try this R.count example in Rambda REPL
countBy<T extends unknown>(transformFn: (x: T) => any, list: T[]): Record<string, number>
It counts elements in a list after each instance of the input list is passed through transformFn
function.
Try this R.countBy example in Rambda REPL
countBy<T extends unknown>(transformFn: (x: T) => any, list: T[]): Record<string, number>;
countBy<T extends unknown>(transformFn: (x: T) => any): (list: T[]) => Record<string, number>;
export function countBy(fn, list){
if (arguments.length === 1){
return _list => countBy(fn, _list)
}
const willReturn = {}
list.forEach(item => {
const key = fn(item)
if (!willReturn[ key ]){
willReturn[ key ] = 1
} else {
willReturn[ key ]++
}
})
return willReturn
}
import { countBy } from './countBy.js'
const list = [ 'a', 'A', 'b', 'B', 'c', 'C' ]
test('happy', () => {
const result = countBy(x => x.toLowerCase(), list)
expect(result).toEqual({
a : 2,
b : 2,
c : 2,
})
})
import {countBy} from 'rambda'
const transformFn = (x: string) => x.toLowerCase()
const list = ['a', 'A', 'b', 'B', 'c', 'C']
describe('R.countBy', () => {
it('happy', () => {
const result = countBy(transformFn, list)
result // $ExpectType Record<string, number>
})
it('curried', () => {
const result = countBy(transformFn)(list)
result // $ExpectType Record<string, number>
})
})
It expects a function as input and returns its curried version.
Try this R.curry example in Rambda REPL
It returns a curried equivalent of the provided function, with the specified arity.
It decrements a number.
Try this R.dec example in Rambda REPL
defaultTo<T>(defaultValue: T, input: T | null | undefined): T
It returns defaultValue
, if all of inputArguments
are undefined
, null
or NaN
.
Else, it returns the first truthy inputArguments
instance(from left to right).
Try this R.defaultTo example in Rambda REPL
defaultTo<T>(defaultValue: T, input: T | null | undefined): T;
defaultTo<T>(defaultValue: T): (input: T | null | undefined) => T;
function isFalsy(input){
return (
input === undefined || input === null || Number.isNaN(input) === true
)
}
export function defaultTo(defaultArgument, input){
if (arguments.length === 1){
return _input => defaultTo(defaultArgument, _input)
}
return isFalsy(input) ? defaultArgument : input
}
import { defaultTo } from './defaultTo.js'
test('with undefined', () => {
expect(defaultTo('foo')(undefined)).toBe('foo')
})
test('with null', () => {
expect(defaultTo('foo')(null)).toBe('foo')
})
test('with NaN', () => {
expect(defaultTo('foo')(NaN)).toBe('foo')
})
test('with empty string', () => {
expect(defaultTo('foo', '')).toBe('')
})
test('with false', () => {
expect(defaultTo('foo', false)).toBeFalse()
})
test('when inputArgument passes initial check', () => {
expect(defaultTo('foo', 'bar')).toBe('bar')
})
import {defaultTo} from 'rambda'
describe('R.defaultTo with Ramda spec', () => {
it('happy', () => {
const result = defaultTo('foo', '')
result // $ExpectType "" | "foo"
})
it('with explicit type', () => {
const result = defaultTo<string>('foo', null)
result // $ExpectType string
})
})
Try this R.descend example in Rambda REPL
difference<T>(a: T[], b: T[]): T[]
It returns the uniq set of all elements in the first list a
not contained in the second list b
.
R.equals
is used to determine equality.
Try this R.difference example in Rambda REPL
difference<T>(a: T[], b: T[]): T[];
difference<T>(a: T[]): (b: T[]) => T[];
import { includes } from './includes.js'
import { uniq } from './uniq.js'
export function difference(a, b){
if (arguments.length === 1) return _b => difference(a, _b)
return uniq(a).filter(aInstance => !includes(aInstance, b))
}
import { difference as differenceRamda } from 'ramda'
import { difference } from './difference.js'
test('difference', () => {
const a = [ 1, 2, 3, 4 ]
const b = [ 3, 4, 5, 6 ]
expect(difference(a)(b)).toEqual([ 1, 2 ])
expect(difference([], [])).toEqual([])
})
test('difference with objects', () => {
const a = [ { id : 1 }, { id : 2 }, { id : 3 }, { id : 4 } ]
const b = [ { id : 3 }, { id : 4 }, { id : 5 }, { id : 6 } ]
expect(difference(a, b)).toEqual([ { id : 1 }, { id : 2 } ])
})
test('no duplicates in first list', () => {
const M2 = [ 1, 2, 3, 4, 1, 2, 3, 4 ]
const N2 = [ 3, 3, 4, 4, 5, 5, 6, 6 ]
expect(difference(M2, N2)).toEqual([ 1, 2 ])
})
test('should use R.equals', () => {
expect(difference([ 1 ], [ 1 ])).toHaveLength(0)
expect(differenceRamda([ NaN ], [ NaN ])).toHaveLength(0)
})
import {difference} from 'rambda'
const list1 = [1, 2, 3]
const list2 = [1, 2, 4]
describe('R.difference', () => {
it('happy', () => {
const result = difference(list1, list2)
result // $ExpectType number[]
})
it('curried', () => {
const result = difference(list1)(list2)
result // $ExpectType number[]
})
})
differenceWith<T1, T2>(
pred: (a: T1, b: T2) => boolean,
list1: T1[],
list2: T2[],
): T1[]
Try this R.differenceWith example in Rambda REPL
differenceWith<T1, T2>(
pred: (a: T1, b: T2) => boolean,
list1: T1[],
list2: T2[],
): T1[];
differenceWith<T1, T2>(
pred: (a: T1, b: T2) => boolean,
): (list1: T1[], list2: T2[]) => T1[];
differenceWith<T1, T2>(
pred: (a: T1, b: T2) => boolean,
list1: T1[],
): (list2: T2[]) => T1[];
import { curry } from './curry.js'
import { _indexOf } from './equals.js'
export function differenceWithFn(
fn, a, b
){
const willReturn = []
const [ first, second ] = a.length > b.length ? [ a, b ] : [ b, a ]
first.forEach(item => {
const hasItem = second.some(secondItem => fn(item, secondItem))
if (!hasItem && _indexOf(item, willReturn) === -1){
willReturn.push(item)
}
})
return willReturn
}
export const differenceWith = curry(differenceWithFn)
import { differenceWith } from './differenceWith.js'
test('happy', () => {
const foo = [ { a : 1 }, { a : 2 }, { a : 3 } ]
const bar = [ { a : 3 }, { a : 4 } ]
const fn = function (r, s){
return r.a === s.a
}
const result = differenceWith(
fn, foo, bar
)
expect(result).toEqual([ { a : 1 }, { a : 2 } ])
})
It returns a new object that does not contain property prop
.
Try this R.dissoc example in Rambda REPL
Try this R.dissocPath example in Rambda REPL
Try this R.divide example in Rambda REPL
drop<T>(howMany: number, input: T[]): T[]
It returns howMany
items dropped from beginning of list or string input
.
Try this R.drop example in Rambda REPL
drop<T>(howMany: number, input: T[]): T[];
drop(howMany: number, input: string): string;
drop<T>(howMany: number): {
<T>(input: T[]): T[];
(input: string): string;
};
export function drop(howManyToDrop, listOrString){
if (arguments.length === 1) return _list => drop(howManyToDrop, _list)
return listOrString.slice(howManyToDrop > 0 ? howManyToDrop : 0)
}
import assert from 'assert'
import { drop } from './drop.js'
test('with array', () => {
expect(drop(2)([ 'foo', 'bar', 'baz' ])).toEqual([ 'baz' ])
expect(drop(3, [ 'foo', 'bar', 'baz' ])).toEqual([])
expect(drop(4, [ 'foo', 'bar', 'baz' ])).toEqual([])
})
test('with string', () => {
expect(drop(3, 'rambda')).toBe('bda')
})
test('with non-positive count', () => {
expect(drop(0, [ 1, 2, 3 ])).toEqual([ 1, 2, 3 ])
expect(drop(-1, [ 1, 2, 3 ])).toEqual([ 1, 2, 3 ])
expect(drop(-Infinity, [ 1, 2, 3 ])).toEqual([ 1, 2, 3 ])
})
test('should return copy', () => {
const xs = [ 1, 2, 3 ]
assert.notStrictEqual(drop(0, xs), xs)
assert.notStrictEqual(drop(-1, xs), xs)
})
import {drop} from 'rambda'
const list = [1, 2, 3, 4]
const str = 'foobar'
const howMany = 2
describe('R.drop - array', () => {
it('happy', () => {
const result = drop(howMany, list)
result // $ExpectType number[]
})
it('curried', () => {
const result = drop(howMany)(list)
result // $ExpectType number[]
})
})
describe('R.drop - string', () => {
it('happy', () => {
const result = drop(howMany, str)
result // $ExpectType string
})
it('curried', () => {
const result = drop(howMany)(str)
result // $ExpectType string
})
})
dropLast<T>(howMany: number, input: T[]): T[]
It returns howMany
items dropped from the end of list or string input
.
Try this R.dropLast example in Rambda REPL
dropLast<T>(howMany: number, input: T[]): T[];
dropLast(howMany: number, input: string): string;
dropLast<T>(howMany: number): {
<T>(input: T[]): T[];
(input: string): string;
};
export function dropLast(howManyToDrop, listOrString){
if (arguments.length === 1){
return _listOrString => dropLast(howManyToDrop, _listOrString)
}
return howManyToDrop > 0 ?
listOrString.slice(0, -howManyToDrop) :
listOrString.slice()
}
import assert from 'assert'
import { dropLast } from './dropLast.js'
test('with array', () => {
expect(dropLast(2)([ 'foo', 'bar', 'baz' ])).toEqual([ 'foo' ])
expect(dropLast(3, [ 'foo', 'bar', 'baz' ])).toEqual([])
expect(dropLast(4, [ 'foo', 'bar', 'baz' ])).toEqual([])
})
test('with string', () => {
expect(dropLast(3, 'rambda')).toBe('ram')
})
test('with non-positive count', () => {
expect(dropLast(0, [ 1, 2, 3 ])).toEqual([ 1, 2, 3 ])
expect(dropLast(-1, [ 1, 2, 3 ])).toEqual([ 1, 2, 3 ])
expect(dropLast(-Infinity, [ 1, 2, 3 ])).toEqual([ 1, 2, 3 ])
})
test('should return copy', () => {
const xs = [ 1, 2, 3 ]
assert.notStrictEqual(dropLast(0, xs), xs)
assert.notStrictEqual(dropLast(-1, xs), xs)
})
import {dropLast} from 'rambda'
const list = [1, 2, 3, 4]
const str = 'foobar'
const howMany = 2
describe('R.dropLast - array', () => {
it('happy', () => {
const result = dropLast(howMany, list)
result // $ExpectType number[]
})
it('curried', () => {
const result = dropLast(howMany)(list)
result // $ExpectType number[]
})
})
describe('R.dropLast - string', () => {
it('happy', () => {
const result = dropLast(howMany, str)
result // $ExpectType string
})
it('curried', () => {
const result = dropLast(howMany)(str)
result // $ExpectType string
})
})
Try this R.dropLastWhile example in Rambda REPL
dropRepeats<T>(list: T[]): T[]
It removes any successive duplicates according to R.equals
.
Try this R.dropRepeats example in Rambda REPL
dropRepeats<T>(list: T[]): T[];
import { isArray } from './_internals/isArray.js'
import { equals } from './equals.js'
export function dropRepeats(list){
if (!isArray(list)){
throw new Error(`${ list } is not a list`)
}
const toReturn = []
list.reduce((prev, current) => {
if (!equals(prev, current)){
toReturn.push(current)
}
return current
}, undefined)
return toReturn
}
import { dropRepeats as dropRepeatsRamda } from 'ramda'
import { compareCombinations } from './_internals/testUtils.js'
import { add } from './add.js'
import { dropRepeats } from './dropRepeats.js'
const list = [ 1, 2, 2, 2, 3, 4, 4, 5, 5, 3, 2, 2, { a : 1 }, { a : 1 } ]
const listClean = [ 1, 2, 3, 4, 5, 3, 2, { a : 1 } ]
test('happy', () => {
const result = dropRepeats(list)
expect(result).toEqual(listClean)
})
const possibleLists = [
[ add(1), async () => {}, [ 1 ], [ 1 ], [ 2 ], [ 2 ] ],
[ add(1), add(1), add(2) ],
[],
1,
/foo/g,
Promise.resolve(1),
]
describe('brute force', () => {
compareCombinations({
firstInput : possibleLists,
callback : errorsCounters => {
expect(errorsCounters).toMatchInlineSnapshot(`
{
"ERRORS_MESSAGE_MISMATCH": 0,
"ERRORS_TYPE_MISMATCH": 0,
"RESULTS_MISMATCH": 1,
"SHOULD_NOT_THROW": 3,
"SHOULD_THROW": 0,
"TOTAL_TESTS": 6,
}
`)
},
fn : dropRepeats,
fnRamda : dropRepeatsRamda,
})
})
import {dropRepeats} from 'rambda'
describe('R.dropRepeats', () => {
it('happy', () => {
const result = dropRepeats([1, 2, 2, 3])
result // $ExpectType number[]
})
})
Try this R.dropRepeatsBy example in Rambda REPL
Try this R.dropRepeatsWith example in Rambda REPL
Try this R.dropWhile example in Rambda REPL
either(firstPredicate: Pred, secondPredicate: Pred): Pred
It returns a new predicate
function from firstPredicate
and secondPredicate
inputs.
This predicate
function will return true
, if any of the two input predicates return true
.
Try this R.either example in Rambda REPL
either(firstPredicate: Pred, secondPredicate: Pred): Pred;
either<T>(firstPredicate: Predicate<T>, secondPredicate: Predicate<T>): Predicate<T>;
either<T>(firstPredicate: Predicate<T>): (secondPredicate: Predicate<T>) => Predicate<T>;
either(firstPredicate: Pred): (secondPredicate: Pred) => Pred;
export function either(firstPredicate, secondPredicate){
if (arguments.length === 1){
return _secondPredicate => either(firstPredicate, _secondPredicate)
}
return (...input) =>
Boolean(firstPredicate(...input) || secondPredicate(...input))
}
import { either } from './either.js'
test('with multiple inputs', () => {
const between = function (
a, b, c
){
return a < b && b < c
}
const total20 = function (
a, b, c
){
return a + b + c === 20
}
const fn = either(between, total20)
expect(fn(
7, 8, 5
)).toBeTrue()
})
test('skip evaluation of the second expression', () => {
let effect = 'not evaluated'
const F = function (){
return true
}
const Z = function (){
effect = 'Z got evaluated'
}
either(F, Z)()
expect(effect).toBe('not evaluated')
})
test('case 1', () => {
const firstFn = val => val > 0
const secondFn = val => val * 5 > 10
expect(either(firstFn, secondFn)(1)).toBeTrue()
})
test('case 2', () => {
const firstFn = val => val > 0
const secondFn = val => val === -10
const fn = either(firstFn)(secondFn)
expect(fn(-10)).toBeTrue()
})
import {either} from 'rambda'
describe('R.either', () => {
it('with passed type', () => {
const fn = either<number>(
x => x > 1,
x => x % 2 === 0
)
fn // $ExpectType Predicate<number>
const result = fn(2) // $ExpectType boolean
result // $ExpectType boolean
})
it('with passed type - curried', () => {
const fn = either<number>(x => x > 1)(x => x % 2 === 0)
fn // $ExpectType Predicate<number>
const result = fn(2)
result // $ExpectType boolean
})
it('no type passed', () => {
const fn = either(
x => {
x // $ExpectType any
return x > 1
},
x => {
x // $ExpectType any
return x % 2 === 0
}
)
const result = fn(2)
result // $ExpectType boolean
})
it('no type passed - curried', () => {
const fn = either((x: number) => {
x // $ExpectType number
return x > 1
})((x: number) => {
x // $ExpectType number
return x % 2 === 0
})
const result = fn(2)
result // $ExpectType boolean
})
})
Try this R.empty example in Rambda REPL
endsWith<T extends string>(question: T, str: string): boolean
When iterable is a string, then it behaves as String.prototype.endsWith
.
When iterable is a list, then it uses R.equals to determine if the target list ends in the same way as the given target.
Try this R.endsWith example in Rambda REPL
endsWith<T extends string>(question: T, str: string): boolean;
endsWith<T extends string>(question: T): (str: string) => boolean;
endsWith<T>(question: T[], list: T[]): boolean;
endsWith<T>(question: T[]): (list: T[]) => boolean;
import { isArray } from './_internals/isArray.js'
import { equals } from './equals.js'
export function endsWith(target, iterable){
if (arguments.length === 1) return _iterable => endsWith(target, _iterable)
if (typeof iterable === 'string'){
return iterable.endsWith(target)
}
if (!isArray(target)) return false
const diff = iterable.length - target.length
let correct = true
const filtered = target.filter((x, index) => {
if (!correct) return false
const result = equals(x, iterable[ index + diff ])
if (!result) correct = false
return result
})
return filtered.length === target.length
}
import { endsWith as endsWithRamda } from 'ramda'
import { compareCombinations } from './_internals/testUtils.js'
import { endsWith } from './endsWith.js'
test('with string', () => {
expect(endsWith('bar', 'foo-bar')).toBeTrue()
expect(endsWith('baz')('foo-bar')).toBeFalse()
})
test('use R.equals with array', () => {
const list = [ { a : 1 }, { a : 2 }, { a : 3 } ]
expect(endsWith({ a : 3 }, list)).toBeFalse(),
expect(endsWith([ { a : 3 } ], list)).toBeTrue()
expect(endsWith([ { a : 2 }, { a : 3 } ], list)).toBeTrue()
expect(endsWith(list, list)).toBeTrue()
expect(endsWith([ { a : 1 } ], list)).toBeFalse()
})
export const possibleTargets = [
NaN,
[ NaN ],
/foo/,
[ /foo/ ],
Promise.resolve(1),
[ Promise.resolve(1) ],
Error('foo'),
[ Error('foo') ],
]
export const possibleIterables = [
[ Promise.resolve(1), Promise.resolve(2) ],
[ /foo/, /bar/ ],
[ NaN ],
[ Error('foo'), Error('bar') ],
]
describe('brute force', () => {
compareCombinations({
fn : endsWith,
fnRamda : endsWithRamda,
firstInput : possibleTargets,
secondInput : possibleIterables,
callback : errorsCounters => {
expect(errorsCounters).toMatchInlineSnapshot(`
{
"ERRORS_MESSAGE_MISMATCH": 0,
"ERRORS_TYPE_MISMATCH": 0,
"RESULTS_MISMATCH": 0,
"SHOULD_NOT_THROW": 0,
"SHOULD_THROW": 0,
"TOTAL_TESTS": 32,
}
`)
},
})
})
import {endsWith} from 'rambda'
describe('R.endsWith - array', () => {
const target = [{a: 2}]
const input = [{a: 1}, {a: 2}]
it('happy', () => {
const result = endsWith(target, input)
result // $ExpectType boolean
})
it('curried', () => {
const result = endsWith(target)(input)
result // $ExpectType boolean
})
})
describe('R.endsWith - string', () => {
const target = 'bar'
const input = 'foo bar'
it('happy', () => {
const result = endsWith(target, input)
result // $ExpectType boolean
})
it('curried', () => {
const result = endsWith(target)(input)
result // $ExpectType boolean
})
})
Try this R.eqBy example in Rambda REPL
It returns true
if property prop
in obj1
is equal to property prop
in obj2
according to R.equals
.
Try this R.eqProps example in Rambda REPL
equals<T>(x: T, y: T): boolean
It deeply compares x
and y
and returns true
if they are equal.
Try this R.equals example in Rambda REPL
equals<T>(x: T, y: T): boolean;
equals<T>(x: T): (y: T) => boolean;
import { isArray } from './_internals/isArray.js'
import { type } from './type.js'
export function _lastIndexOf(valueToFind, list){
if (!isArray(list))
throw new Error(`Cannot read property 'indexOf' of ${ list }`)
const typeOfValue = type(valueToFind)
if (![ 'Array', 'NaN', 'Object', 'RegExp' ].includes(typeOfValue))
return list.lastIndexOf(valueToFind)
const { length } = list
let index = length
let foundIndex = -1
while (--index > -1 && foundIndex === -1)
if (equals(list[ index ], valueToFind))
foundIndex = index
return foundIndex
}
export function _indexOf(valueToFind, list){
if (!isArray(list))
throw new Error(`Cannot read property 'indexOf' of ${ list }`)
const typeOfValue = type(valueToFind)
if (![ 'Array', 'NaN', 'Object', 'RegExp' ].includes(typeOfValue))
return list.indexOf(valueToFind)
let index = -1
let foundIndex = -1
const { length } = list
while (++index < length && foundIndex === -1)
if (equals(list[ index ], valueToFind))
foundIndex = index
return foundIndex
}
function _arrayFromIterator(iter){
const list = []
let next
while (!(next = iter.next()).done)
list.push(next.value)
return list
}
function _compareSets(a, b){
if (a.size !== b.size)
return false
const aList = _arrayFromIterator(a.values())
const bList = _arrayFromIterator(b.values())
const filtered = aList.filter(aInstance => _indexOf(aInstance, bList) === -1)
return filtered.length === 0
}
function compareErrors(a, b){
if (a.message !== b.message) return false
if (a.toString !== b.toString) return false
return a.toString() === b.toString()
}
function parseDate(maybeDate){
if (!maybeDate.toDateString) return [ false ]
return [ true, maybeDate.getTime() ]
}
function parseRegex(maybeRegex){
if (maybeRegex.constructor !== RegExp) return [ false ]
return [ true, maybeRegex.toString() ]
}
export function equals(a, b){
if (arguments.length === 1) return _b => equals(a, _b)
const aType = type(a)
if (aType !== type(b)) return false
if (aType === 'Function')
return a.name === undefined ? false : a.name === b.name
if ([ 'NaN', 'Null', 'Undefined' ].includes(aType)) return true
if ([ 'BigInt', 'Number' ].includes(aType)){
if (Object.is(-0, a) !== Object.is(-0, b)) return false
return a.toString() === b.toString()
}
if ([ 'Boolean', 'String' ].includes(aType))
return a.toString() === b.toString()
if (aType === 'Array'){
const aClone = Array.from(a)
const bClone = Array.from(b)
if (aClone.toString() !== bClone.toString())
return false
let loopArrayFlag = true
aClone.forEach((aCloneInstance, aCloneIndex) => {
if (loopArrayFlag)
if (
aCloneInstance !== bClone[ aCloneIndex ] &&
!equals(aCloneInstance, bClone[ aCloneIndex ])
)
loopArrayFlag = false
})
return loopArrayFlag
}
const aRegex = parseRegex(a)
const bRegex = parseRegex(b)
if (aRegex[ 0 ])
return bRegex[ 0 ] ? aRegex[ 1 ] === bRegex[ 1 ] : false
else if (bRegex[ 0 ]) return false
const aDate = parseDate(a)
const bDate = parseDate(b)
if (aDate[ 0 ])
return bDate[ 0 ] ? aDate[ 1 ] === bDate[ 1 ] : false
else if (bDate[ 0 ]) return false
if (a instanceof Error){
if (!(b instanceof Error)) return false
return compareErrors(a, b)
}
if (aType === 'Set')
return _compareSets(a, b)
if (aType === 'Object'){
const aKeys = Object.keys(a)
if (aKeys.length !== Object.keys(b).length)
return false
let loopObjectFlag = true
aKeys.forEach(aKeyInstance => {
if (loopObjectFlag){
const aValue = a[ aKeyInstance ]
const bValue = b[ aKeyInstance ]
if (aValue !== bValue && !equals(aValue, bValue))
loopObjectFlag = false
}
})
return loopObjectFlag
}
return false
}
import { equals as equalsRamda } from 'ramda'
import { compareCombinations } from './_internals/testUtils.js'
import { variousTypes } from './benchmarks/_utils.js'
import { equals } from './equals.js'
test('compare functions', () => {
function foo(){}
function bar(){}
const baz = () => {}
const expectTrue = equals(foo, foo)
const expectFalseFirst = equals(foo, bar)
const expectFalseSecond = equals(foo, baz)
expect(expectTrue).toBeTrue()
expect(expectFalseFirst).toBeFalse()
expect(expectFalseSecond).toBeFalse()
})
test('with array of objects', () => {
const list1 = [ { a : 1 }, [ { b : 2 } ] ]
const list2 = [ { a : 1 }, [ { b : 2 } ] ]
const list3 = [ { a : 1 }, [ { b : 3 } ] ]
expect(equals(list1, list2)).toBeTrue()
expect(equals(list1, list3)).toBeFalse()
})
test('with regex', () => {
expect(equals(/s/, /s/)).toBeTrue()
expect(equals(/s/, /d/)).toBeFalse()
expect(equals(/a/gi, /a/gi)).toBeTrue()
expect(equals(/a/gim, /a/gim)).toBeTrue()
expect(equals(/a/gi, /a/i)).toBeFalse()
})
test('not a number', () => {
expect(equals([ NaN ], [ NaN ])).toBeTrue()
})
test('new number', () => {
expect(equals(new Number(0), new Number(0))).toBeTrue()
expect(equals(new Number(0), new Number(1))).toBeFalse()
expect(equals(new Number(1), new Number(0))).toBeFalse()
})
test('new string', () => {
expect(equals(new String(''), new String(''))).toBeTrue()
expect(equals(new String(''), new String('x'))).toBeFalse()
expect(equals(new String('x'), new String(''))).toBeFalse()
expect(equals(new String('foo'), new String('foo'))).toBeTrue()
expect(equals(new String('foo'), new String('bar'))).toBeFalse()
expect(equals(new String('bar'), new String('foo'))).toBeFalse()
})
test('new Boolean', () => {
expect(equals(new Boolean(true), new Boolean(true))).toBeTrue()
expect(equals(new Boolean(false), new Boolean(false))).toBeTrue()
expect(equals(new Boolean(true), new Boolean(false))).toBeFalse()
expect(equals(new Boolean(false), new Boolean(true))).toBeFalse()
})
test('new Error', () => {
expect(equals(new Error('XXX'), {})).toBeFalse()
expect(equals(new Error('XXX'), new TypeError('XXX'))).toBeFalse()
expect(equals(new Error('XXX'), new Error('YYY'))).toBeFalse()
expect(equals(new Error('XXX'), new Error('XXX'))).toBeTrue()
expect(equals(new Error('XXX'), new TypeError('YYY'))).toBeFalse()
expect(equals(new Error('XXX'), new Error('XXX'))).toBeTrue()
})
test('with dates', () => {
expect(equals(new Date(0), new Date(0))).toBeTrue()
expect(equals(new Date(1), new Date(1))).toBeTrue()
expect(equals(new Date(0), new Date(1))).toBeFalse()
expect(equals(new Date(1), new Date(0))).toBeFalse()
expect(equals(new Date(0), {})).toBeFalse()
expect(equals({}, new Date(0))).toBeFalse()
})
test('ramda spec', () => {
expect(equals({}, {})).toBeTrue()
expect(equals({
a : 1,
b : 2,
},
{
a : 1,
b : 2,
})).toBeTrue()
expect(equals({
a : 2,
b : 3,
},
{
a : 2,
b : 3,
})).toBeTrue()
expect(equals({
a : 2,
b : 3,
},
{
a : 3,
b : 3,
})).toBeFalse()
expect(equals({
a : 2,
b : 3,
c : 1,
},
{
a : 2,
b : 3,
})).toBeFalse()
})
test('works with boolean tuple', () => {
expect(equals([ true, false ], [ true, false ])).toBeTrue()
expect(equals([ true, false ], [ true, true ])).toBeFalse()
})
test('works with equal objects within array', () => {
const objFirst = {
a : {
b : 1,
c : 2,
d : [ 1 ],
},
}
const objSecond = {
a : {
b : 1,
c : 2,
d : [ 1 ],
},
}
const x = [ 1, 2, objFirst, null, '', [] ]
const y = [ 1, 2, objSecond, null, '', [] ]
expect(equals(x, y)).toBeTrue()
})
test('works with different objects within array', () => {
const objFirst = { a : { b : 1 } }
const objSecond = { a : { b : 2 } }
const x = [ 1, 2, objFirst, null, '', [] ]
const y = [ 1, 2, objSecond, null, '', [] ]
expect(equals(x, y)).toBeFalse()
})
test('works with undefined as second argument', () => {
expect(equals(1, undefined)).toBeFalse()
expect(equals(undefined, undefined)).toBeTrue()
})
test('compare sets', () => {
const toCompareDifferent = new Set([ { a : 1 }, { a : 2 } ])
const toCompareSame = new Set([ { a : 1 }, { a : 2 }, { a : 1 } ])
const testSet = new Set([ { a : 1 }, { a : 2 }, { a : 1 } ])
expect(equals(toCompareSame, testSet)).toBeTruthy()
expect(equals(toCompareDifferent, testSet)).toBeFalsy()
expect(equalsRamda(toCompareSame, testSet)).toBeTruthy()
expect(equalsRamda(toCompareDifferent, testSet)).toBeFalsy()
})
test('compare simple sets', () => {
const testSet = new Set([ '2', '3', '3', '2', '1' ])
expect(equals(new Set([ '3', '2', '1' ]), testSet)).toBeTruthy()
expect(equals(new Set([ '3', '2', '0' ]), testSet)).toBeFalsy()
})
test('various examples', () => {
expect(equals([ 1, 2, 3 ])([ 1, 2, 3 ])).toBeTrue()
expect(equals([ 1, 2, 3 ], [ 1, 2 ])).toBeFalse()
expect(equals(1, 1)).toBeTrue()
expect(equals(1, '1')).toBeFalse()
expect(equals({}, {})).toBeTrue()
expect(equals({
a : 1,
b : 2,
},
{
a : 1,
b : 2,
})).toBeTrue()
expect(equals({
a : 1,
b : 2,
},
{
a : 1,
b : 1,
})).toBeFalse()
expect(equals({
a : 1,
b : false,
},
{
a : 1,
b : 1,
})).toBeFalse()
expect(equals({
a : 1,
b : 2,
},
{
a : 1,
b : 2,
c : 3,
})).toBeFalse()
expect(equals({
x : {
a : 1,
b : 2,
},
},
{
x : {
a : 1,
b : 2,
c : 3,
},
})).toBeFalse()
expect(equals({
a : 1,
b : 2,
},
{
a : 1,
b : 3,
})).toBeFalse()
expect(equals({ a : { b : { c : 1 } } }, { a : { b : { c : 1 } } })).toBeTrue()
expect(equals({ a : { b : { c : 1 } } }, { a : { b : { c : 2 } } })).toBeFalse()
expect(equals({ a : {} }, { a : {} })).toBeTrue()
expect(equals('', '')).toBeTrue()
expect(equals('foo', 'foo')).toBeTrue()
expect(equals('foo', 'bar')).toBeFalse()
expect(equals(0, false)).toBeFalse()
expect(equals(/\s/g, null)).toBeFalse()
expect(equals(null, null)).toBeTrue()
expect(equals(false)(null)).toBeFalse()
})
test('with custom functions', () => {
function foo(){
return 1
}
foo.prototype.toString = () => ''
const result = equals(foo, foo)
expect(result).toBeTrue()
})
test('with classes', () => {
class Foo{}
const foo = new Foo()
const result = equals(foo, foo)
expect(result).toBeTrue()
})
test('with negative zero', () => {
expect(equals(-0, -0)).toBeTrue()
expect(equals(-0, 0)).toBeFalse()
expect(equals(0, 0)).toBeTrue()
expect(equals(-0, 1)).toBeFalse()
})
test('with big int', () => {
const a = BigInt(9007199254740991)
const b = BigInt(9007199254740991)
const c = BigInt(7007199254740991)
expect(equals(a, b)).toBeTrue()
expect(equals(a, c)).toBeFalse()
})
describe('brute force', () => {
compareCombinations({
callback : errorsCounters => {
expect(errorsCounters).toMatchInlineSnapshot(`
{
"ERRORS_MESSAGE_MISMATCH": 0,
"ERRORS_TYPE_MISMATCH": 0,
"RESULTS_MISMATCH": 8,
"SHOULD_NOT_THROW": 0,
"SHOULD_THROW": 0,
"TOTAL_TESTS": 289,
}
`)
},
firstInput : variousTypes,
fn : equals,
fnRamda : equalsRamda,
secondInput : variousTypes,
})
})
import {equals} from 'rambda'
describe('R.equals', () => {
it('happy', () => {
const result = equals(4, 1)
result // $ExpectType boolean
})
it('with object', () => {
const foo = {a: 1}
const bar = {a: 2}
const result = equals(foo, bar)
result // $ExpectType boolean
})
it('curried', () => {
const result = equals(4)(1)
result // $ExpectType boolean
})
})
evolve<T, U>(rules: ((x: T) => U)[], list: T[]): U[]
It takes object or array of functions as set of rules. These rules
are applied to the iterable
input to produce the result.
Try this R.evolve example in Rambda REPL
evolve<T, U>(rules: ((x: T) => U)[], list: T[]): U[];
evolve<T, U>(rules: ((x: T) => U)[]) : (list: T[]) => U[];
evolve<E extends Evolver, V extends Evolvable<E>>(rules: E, obj: V): Evolve<V, E>;
evolve<E extends Evolver>(rules: E): <V extends Evolvable<E>>(obj: V) => Evolve<V, E>;
import { isArray } from './_internals/isArray.js'
import { mapArray, mapObject } from './map.js'
import { type } from './type.js'
export function evolveArray(rules, list){
return mapArray(
(x, i) => {
if (type(rules[ i ]) === 'Function'){
return rules[ i ](x)
}
return x
},
list,
true
)
}
export function evolveObject(rules, iterable){
return mapObject((x, prop) => {
if (type(x) === 'Object'){
const typeRule = type(rules[ prop ])
if (typeRule === 'Function'){
return rules[ prop ](x)
}
if (typeRule === 'Object'){
return evolve(rules[ prop ], x)
}
return x
}
if (type(rules[ prop ]) === 'Function'){
return rules[ prop ](x)
}
return x
}, iterable)
}
export function evolve(rules, iterable){
if (arguments.length === 1){
return _iterable => evolve(rules, _iterable)
}
const rulesType = type(rules)
const iterableType = type(iterable)
if (iterableType !== rulesType){
throw new Error('iterableType !== rulesType')
}
if (![ 'Object', 'Array' ].includes(rulesType)){
throw new Error(`'iterable' and 'rules' are from wrong type ${ rulesType }`)
}
if (iterableType === 'Object'){
return evolveObject(rules, iterable)
}
return evolveArray(rules, iterable)
}
import { evolve as evolveRamda } from 'ramda'
import { add } from '../rambda.js'
import { compareCombinations, compareToRamda } from './_internals/testUtils.js'
import { evolve } from './evolve.js'
test('happy', () => {
const rules = {
foo : add(1),
nested : { bar : x => Object.keys(x).length },
}
const input = {
a : 1,
foo : 2,
nested : { bar : { z : 3 } },
}
const result = evolve(rules, input)
expect(result).toEqual({
a : 1,
foo : 3,
nested : { bar : 1 },
})
})
test('nested rule is wrong', () => {
const rules = {
foo : add(1),
nested : { bar : 10 },
}
const input = {
a : 1,
foo : 2,
nested : { bar : { z : 3 } },
}
const result = evolve(rules)(input)
expect(result).toEqual({
a : 1,
foo : 3,
nested : { bar : { z : 3 } },
})
})
test('is recursive', () => {
const rules = {
nested : {
second : add(-1),
third : add(1),
},
}
const object = {
first : 1,
nested : {
second : 2,
third : 3,
},
}
const expected = {
first : 1,
nested : {
second : 1,
third : 4,
},
}
const result = evolve(rules, object)
expect(result).toEqual(expected)
})
test('ignores primitive values', () => {
const rules = {
n : 2,
m : 'foo',
}
const object = {
n : 0,
m : 1,
}
const expected = {
n : 0,
m : 1,
}
const result = evolve(rules, object)
expect(result).toEqual(expected)
})
test('with array', () => {
const rules = [ add(1), add(-1) ]
const list = [ 100, 1400 ]
const expected = [ 101, 1399 ]
const result = evolve(rules, list)
expect(result).toEqual(expected)
})
const rulesObject = { a : add(1) }
const rulesList = [ add(1) ]
const possibleIterables = [ null, undefined, '', 42, [], [ 1 ], { a : 1 } ]
const possibleRules = [ ...possibleIterables, rulesList, rulesObject ]
describe('brute force', () => {
compareCombinations({
firstInput : possibleRules,
callback : errorsCounters => {
expect(errorsCounters).toMatchInlineSnapshot(`
{
"ERRORS_MESSAGE_MISMATCH": 0,
"ERRORS_TYPE_MISMATCH": 4,
"RESULTS_MISMATCH": 0,
"SHOULD_NOT_THROW": 51,
"SHOULD_THROW": 0,
"TOTAL_TESTS": 63,
}
`)
},
secondInput : possibleIterables,
fn : evolve,
fnRamda : evolveRamda,
})
})
import {evolve, add} from 'rambda'
describe('R.evolve', () => {
it('happy', () => {
const input = {
foo: 2,
nested: {
a: 1,
bar: 3,
},
}
const rules = {
foo: add(1),
nested: {
a: add(-1),
bar: add(1),
},
}
const result = evolve(rules, input)
const curriedResult = evolve(rules)(input)
result.nested.a // $ExpectType number
curriedResult.nested.a // $ExpectType number
result.nested.bar // $ExpectType number
result.foo // $ExpectType number
})
it('with array', () => {
const rules = [String, String]
const input = [100, 1400]
const result = evolve(rules, input)
const curriedResult = evolve(rules)(input)
result // $ExpectType string[]
curriedResult // $ExpectType string[]
})
})
F(): boolean
Try this R.F example in Rambda REPL
F(): boolean;
export function F(){
return false
}
filter<T>(predicate: Predicate<T>): (input: T[]) => T[]
It filters list or object input
using a predicate
function.
Try this R.filter example in Rambda REPL
filter<T>(predicate: Predicate<T>): (input: T[]) => T[];
filter<T>(predicate: Predicate<T>, input: T[]): T[];
filter<T, U>(predicate: ObjectPredicate<T>): (x: Dictionary<T>) => Dictionary<T>;
filter<T>(predicate: ObjectPredicate<T>, x: Dictionary<T>): Dictionary<T>;
import { isArray } from './_internals/isArray.js'
export function filterObject(predicate, obj){
const willReturn = {}
for (const prop in obj){
if (predicate(
obj[ prop ], prop, obj
)){
willReturn[ prop ] = obj[ prop ]
}
}
return willReturn
}
export function filterArray(
predicate, list, indexed = false
){
let index = 0
const len = list.length
const willReturn = []
while (index < len){
const predicateResult = indexed ?
predicate(list[ index ], index) :
predicate(list[ index ])
if (predicateResult){
willReturn.push(list[ index ])
}
index++
}
return willReturn
}
export function filter(predicate, iterable){
if (arguments.length === 1)
return _iterable => filter(predicate, _iterable)
if (!iterable){
throw new Error('Incorrect iterable input')
}
if (isArray(iterable)) return filterArray(
predicate, iterable, false
)
return filterObject(predicate, iterable)
}
import { filter as filterRamda } from 'ramda'
import { filter } from './filter.js'
import { T } from './T.js'
const sampleObject = {
a : 1,
b : 2,
c : 3,
d : 4,
}
test('happy', () => {
const isEven = n => n % 2 === 0
expect(filter(isEven, [ 1, 2, 3, 4 ])).toEqual([ 2, 4 ])
expect(filter(isEven, {
a : 1,
b : 2,
d : 3,
})).toEqual({ b : 2 })
})
test('predicate when input is object', () => {
const obj = {
a : 1,
b : 2,
}
const predicate = (
val, prop, inputObject
) => {
expect(inputObject).toEqual(obj)
expect(typeof prop).toBe('string')
return val < 2
}
expect(filter(predicate, obj)).toEqual({ a : 1 })
})
test('with object', () => {
const isEven = n => n % 2 === 0
const result = filter(isEven, sampleObject)
const expectedResult = {
b : 2,
d : 4,
}
expect(result).toEqual(expectedResult)
})
test('bad inputs difference between Ramda and Rambda', () => {
expect(() => filter(T, null)).toThrowWithMessage(Error,
'Incorrect iterable input')
expect(() => filter(T)(undefined)).toThrowWithMessage(Error,
'Incorrect iterable input')
expect(() => filterRamda(T, null)).toThrowWithMessage(TypeError,
'Cannot read properties of null (reading \'fantasy-land/filter\')')
expect(() => filterRamda(T, undefined)).toThrowWithMessage(TypeError,
'Cannot read properties of undefined (reading \'fantasy-land/filter\')')
})
import {filter} from 'rambda'
const list = [1, 2, 3]
const obj = {a: 1, b: 2}
describe('R.filter with array', () => {
it('happy', () => {
const result = filter<number>(x => {
x // $ExpectType number
return x > 1
}, list)
result // $ExpectType number[]
})
it('curried', () => {
const result = filter<number>(x => {
x // $ExpectType number
return x > 1
})(list)
result // $ExpectType number[]
})
})
describe('R.filter with objects', () => {
it('happy', () => {
const result = filter<number>((val, prop, origin) => {
val // $ExpectType number
prop // $ExpectType string
origin // $ExpectType Dictionary<number>
return val > 1
}, obj)
result // $ExpectType Dictionary<number>
})
it('curried version requires second dummy type', () => {
const result = filter<number, any>((val, prop, origin) => {
val // $ExpectType number
prop // $ExpectType string
origin // $ExpectType Dictionary<number>
return val > 1
})(obj)
result // $ExpectType Dictionary<number>
})
})
find<T>(predicate: (x: T) => boolean, list: T[]): T | undefined
It returns the first element of list
that satisfy the predicate
.
If there is no such element, it returns undefined
.
Try this R.find example in Rambda REPL
find<T>(predicate: (x: T) => boolean, list: T[]): T | undefined;
find<T>(predicate: (x: T) => boolean): (list: T[]) => T | undefined;
export function find(predicate, list){
if (arguments.length === 1) return _list => find(predicate, _list)
let index = 0
const len = list.length
while (index < len){
const x = list[ index ]
if (predicate(x)){
return x
}
index++
}
}
import { find } from './find.js'
import { propEq } from './propEq.js'
const list = [ { a : 1 }, { a : 2 }, { a : 3 } ]
test('happy', () => {
const fn = propEq(2, 'a')
expect(find(fn, list)).toEqual({ a : 2 })
})
test('with curry', () => {
const fn = propEq(4, 'a')
expect(find(fn)(list)).toBeUndefined()
})
test('with empty list', () => {
expect(find(() => true, [])).toBeUndefined()
})
import {find} from 'rambda'
const list = [1, 2, 3]
describe('R.find', () => {
it('happy', () => {
const predicate = (x: number) => x > 2
const result = find(predicate, list)
result // $ExpectType number | undefined
})
it('curried', () => {
const predicate = (x: number) => x > 2
const result = find(predicate)(list)
result // $ExpectType number | undefined
})
})
findIndex<T>(predicate: (x: T) => boolean, list: T[]): number
It returns the index of the first element of list
satisfying the predicate
function.
If there is no such element, then -1
is returned.
Try this R.findIndex example in Rambda REPL
findIndex<T>(predicate: (x: T) => boolean, list: T[]): number;
findIndex<T>(predicate: (x: T) => boolean): (list: T[]) => number;
export function findIndex(predicate, list){
if (arguments.length === 1) return _list => findIndex(predicate, _list)
const len = list.length
let index = -1
while (++index < len){
if (predicate(list[ index ])){
return index
}
}
return -1
}
import { findIndex } from './findIndex.js'
import { propEq } from './propEq.js'
const list = [ { a : 1 }, { a : 2 }, { a : 3 } ]
test('happy', () => {
expect(findIndex(propEq(2, 'a'), list)).toBe(1)
expect(findIndex(propEq(1, 'a'))(list)).toBe(0)
expect(findIndex(propEq(4, 'a'))(list)).toBe(-1)
})
import {findIndex} from 'rambda'
const list = [1, 2, 3]
describe('R.findIndex', () => {
it('happy', () => {
const predicate = (x: number) => x > 2
const result = findIndex(predicate, list)
result // $ExpectType number
})
it('curried', () => {
const predicate = (x: number) => x > 2
const result = findIndex(predicate)(list)
result // $ExpectType number
})
})
findLast<T>(fn: (x: T) => boolean, list: T[]): T | undefined
It returns the last element of list
satisfying the predicate
function.
If there is no such element, then undefined
is returned.
Try this R.findLast example in Rambda REPL
findLast<T>(fn: (x: T) => boolean, list: T[]): T | undefined;
findLast<T>(fn: (x: T) => boolean): (list: T[]) => T | undefined;
export function findLast(predicate, list){
if (arguments.length === 1) return _list => findLast(predicate, _list)
let index = list.length
while (--index >= 0){
if (predicate(list[ index ])){
return list[ index ]
}
}
return undefined
}
import { findLast } from './findLast.js'
test('happy', () => {
const result = findLast(x => x > 1, [ 1, 1, 1, 2, 3, 4, 1 ])
expect(result).toBe(4)
expect(findLast(x => x === 0, [ 0, 1, 1, 2, 3, 4, 1 ])).toBe(0)
})
test('with curry', () => {
expect(findLast(x => x > 1)([ 1, 1, 1, 2, 3, 4, 1 ])).toBe(4)
})
const obj1 = { x : 100 }
const obj2 = { x : 200 }
const a = [ 11, 10, 9, 'cow', obj1, 8, 7, 100, 200, 300, obj2, 4, 3, 2, 1, 0 ]
const even = function (x){
return x % 2 === 0
}
const gt100 = function (x){
return x > 100
}
const isStr = function (x){
return typeof x === 'string'
}
const xGt100 = function (o){
return o && o.x > 100
}
test('ramda 1', () => {
expect(findLast(even, a)).toBe(0)
expect(findLast(gt100, a)).toBe(300)
expect(findLast(isStr, a)).toBe('cow')
expect(findLast(xGt100, a)).toEqual(obj2)
})
test('ramda 2', () => {
expect(findLast(even, [ 'zing' ])).toBeUndefined()
})
test('ramda 3', () => {
expect(findLast(even, [ 2, 3, 5 ])).toBe(2)
})
test('ramda 4', () => {
expect(findLast(even, [])).toBeUndefined()
})
import {findLast} from 'rambda'
const list = [1, 2, 3]
describe('R.findLast', () => {
it('happy', () => {
const predicate = (x: number) => x > 2
const result = findLast(predicate, list)
result // $ExpectType number | undefined
})
it('curried', () => {
const predicate = (x: number) => x > 2
const result = findLast(predicate)(list)
result // $ExpectType number | undefined
})
})
findLastIndex<T>(predicate: (x: T) => boolean, list: T[]): number
It returns the index of the last element of list
satisfying the predicate
function.
If there is no such element, then -1
is returned.
Try this R.findLastIndex example in Rambda REPL
findLastIndex<T>(predicate: (x: T) => boolean, list: T[]): number;
findLastIndex<T>(predicate: (x: T) => boolean): (list: T[]) => number;
export function findLastIndex(fn, list){
if (arguments.length === 1) return _list => findLastIndex(fn, _list)
let index = list.length
while (--index >= 0){
if (fn(list[ index ])){
return index
}
}
return -1
}
import { findLastIndex } from './findLastIndex.js'
test('happy', () => {
const result = findLastIndex(x => x > 1, [ 1, 1, 1, 2, 3, 4, 1 ])
expect(result).toBe(5)
expect(findLastIndex(x => x === 0, [ 0, 1, 1, 2, 3, 4, 1 ])).toBe(0)
})
test('with curry', () => {
expect(findLastIndex(x => x > 1)([ 1, 1, 1, 2, 3, 4, 1 ])).toBe(5)
})
const obj1 = { x : 100 }
const obj2 = { x : 200 }
const a = [ 11, 10, 9, 'cow', obj1, 8, 7, 100, 200, 300, obj2, 4, 3, 2, 1, 0 ]
const even = function (x){
return x % 2 === 0
}
const gt100 = function (x){
return x > 100
}
const isStr = function (x){
return typeof x === 'string'
}
const xGt100 = function (o){
return o && o.x > 100
}
test('ramda 1', () => {
expect(findLastIndex(even, a)).toBe(15)
expect(findLastIndex(gt100, a)).toBe(9)
expect(findLastIndex(isStr, a)).toBe(3)
expect(findLastIndex(xGt100, a)).toBe(10)
})
test('ramda 2', () => {
expect(findLastIndex(even, [ 'zing' ])).toBe(-1)
})
test('ramda 3', () => {
expect(findLastIndex(even, [ 2, 3, 5 ])).toBe(0)
})
test('ramda 4', () => {
expect(findLastIndex(even, [])).toBe(-1)
})
import {findLastIndex} from 'rambda'
const list = [1, 2, 3]
describe('R.findLastIndex', () => {
it('happy', () => {
const predicate = (x: number) => x > 2
const result = findLastIndex(predicate, list)
result // $ExpectType number
})
it('curried', () => {
const predicate = (x: number) => x > 2
const result = findLastIndex(predicate)(list)
result // $ExpectType number
})
})
flatten<T>(list: any[]): T[]
It deeply flattens an array.
Try this R.flatten example in Rambda REPL
flatten<T>(list: any[]): T[];
import { isArray } from './_internals/isArray.js'
export function flatten(list, input){
const willReturn = input === undefined ? [] : input
for (let i = 0; i < list.length; i++){
if (isArray(list[ i ])){
flatten(list[ i ], willReturn)
} else {
willReturn.push(list[ i ])
}
}
return willReturn
}
import { flatten } from './flatten.js'
test('happy', () => {
expect(flatten([ 1, 2, 3, [ [ [ [ [ 4 ] ] ] ] ] ])).toEqual([ 1, 2, 3, 4 ])
expect(flatten([ 1, [ 2, [ [ 3 ] ] ], [ 4 ] ])).toEqual([ 1, 2, 3, 4 ])
expect(flatten([ 1, [ 2, [ [ [ 3 ] ] ] ], [ 4 ] ])).toEqual([ 1, 2, 3, 4 ])
expect(flatten([ 1, 2, [ 3, 4 ], 5, [ 6, [ 7, 8, [ 9, [ 10, 11 ], 12 ] ] ] ])).toEqual([ 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12 ])
})
test('readme example', () => {
const result = flatten([ 1, 2, [ 3, 30, [ 300 ] ], [ 4 ] ])
expect(result).toEqual([ 1, 2, 3, 30, 300, 4 ])
})
import {flatten} from 'rambda'
describe('flatten', () => {
it('happy', () => {
const result = flatten<number>([1, 2, [3, [4]]])
result // $ExpectType number[]
})
})
It returns function which calls fn
with exchanged first and second argument.
Try this R.flip example in Rambda REPL
forEach<T>(fn: Iterator<T, void>, list: T[]): T[]
It applies iterable
function over all members of list
and returns list
.
Try this R.forEach example in Rambda REPL
forEach<T>(fn: Iterator<T, void>, list: T[]): T[];
forEach<T>(fn: Iterator<T, void>): (list: T[]) => T[];
forEach<T>(fn: ObjectIterator<T, void>, list: Dictionary<T>): Dictionary<T>;
forEach<T, U>(fn: ObjectIterator<T, void>): (list: Dictionary<T>) => Dictionary<T>;
import { isArray } from './_internals/isArray.js'
import { forEachObjIndexedFn } from './forEachObjIndexed.js'
export function forEach(fn, iterable){
if (arguments.length === 1) return _list => forEach(fn, _list)
if (iterable === undefined) return
if (isArray(iterable)){
let index = 0
const len = iterable.length
while (index < len){
fn(iterable[ index ])
index++
}
} else return forEachObjIndexedFn(fn, iterable)
return iterable
}
import { forEach } from './forEach.js'
import { type } from './type.js'
test('happy', () => {
const sideEffect = {}
forEach(x => sideEffect[ `foo${ x }` ] = x + 10)([ 1, 2 ])
expect(sideEffect).toEqual({
foo1 : 11,
foo2 : 12,
})
})
test('iterate over object', () => {
const obj = {
a : 1,
b : [ 1, 2 ],
c : { d : 7 },
f : 'foo',
}
const result = {}
const returned = forEach((
val, prop, inputObj
) => {
expect(type(inputObj)).toBe('Object')
result[ prop ] = `${ prop }-${ type(val) }`
})(obj)
const expected = {
a : 'a-Number',
b : 'b-Array',
c : 'c-Object',
f : 'f-String',
}
expect(result).toEqual(expected)
expect(returned).toEqual(obj)
})
test('with empty list', () => {
const list = []
const result = forEach(x => x * x)(list)
expect(result).toEqual(list)
})
test('with wrong input', () => {
const list = undefined
const result = forEach(x => x * x)(list)
expect(result).toBeUndefined()
})
test('returns the input', () => {
const list = [ 1, 2, 3 ]
const result = forEach(x => x * x)(list)
expect(result).toEqual(list)
})
import {forEach} from 'rambda'
const list = [1, 2, 3]
const obj = {a: 1, b: 2}
describe('R.forEach with arrays', () => {
it('happy', () => {
const result = forEach(a => {
a // $ExpectType number
}, list)
result // $ExpectType number[]
})
it('curried require an explicit typing', () => {
const result = forEach<number>(a => {
a // $ExpectType number
})(list)
result // $ExpectType number[]
})
})
describe('R.forEach with objects', () => {
it('happy', () => {
const result = forEach((a, b, c) => {
a // $ExpectType number
b // $ExpectType string
c // $ExpectType Dictionary<number>
return `${a}`
}, obj)
result // $ExpectType Dictionary<number>
})
it('curried require an input typing and a dummy third typing', () => {
// Required in order all typings to work
const result = forEach<number, any>((a, b, c) => {
a // $ExpectType number
b // $ExpectType string
c // $ExpectType Dictionary<number>
})(obj)
result // $ExpectType Dictionary<number>
})
it('iterator without property', () => {
const result = forEach(a => {
a // $ExpectType number
}, obj)
result // $ExpectType Dictionary<number>
})
})
It transforms a listOfPairs
to an object.
Try this R.fromPairs example in Rambda REPL
It splits list
according to a provided groupFn
function and returns an object.
Try this R.groupBy example in Rambda REPL
It returns separated version of list or string input
, where separation is done with equality compareFn
function.
Try this R.groupWith example in Rambda REPL
Try this R.gt example in Rambda REPL
Try this R.gte example in Rambda REPL
has<T>(prop: string, obj: T): boolean
It returns true
if obj
has property prop
.
Try this R.has example in Rambda REPL
has<T>(prop: string, obj: T): boolean;
has(prop: string): <T>(obj: T) => boolean;
export function has(prop, obj){
if (arguments.length === 1) return _obj => has(prop, _obj)
if (!obj) return false
return obj.hasOwnProperty(prop)
}
import { has } from './has.js'
test('happy', () => {
expect(has('a')({ a : 1 })).toBeTrue()
expect(has('b', { a : 1 })).toBeFalse()
})
test('with non-object', () => {
expect(has('a', undefined)).toBeFalse()
expect(has('a', null)).toBeFalse()
expect(has('a', true)).toBeFalse()
expect(has('a', '')).toBeFalse()
expect(has('a', /a/)).toBeFalse()
})
import {has} from 'rambda'
describe('R.has', () => {
it('happy', () => {
const result = has('foo', {a: 1})
const curriedResult = has('bar')({a: 1})
result // $ExpectType boolean
curriedResult // $ExpectType boolean
})
})
Try this R.hasIn example in Rambda REPL
hasPath<T>(
path: string | string[],
input: object
): boolean
It will return true, if input
object has truthy path
(calculated with R.path
).
Try this R.hasPath example in Rambda REPL
hasPath<T>(
path: string | string[],
input: object
): boolean;
hasPath<T>(
path: string | string[]
): (input: object) => boolean;
import { path } from './path.js'
export function hasPath(pathInput, obj){
if (arguments.length === 1){
return objHolder => hasPath(pathInput, objHolder)
}
return path(pathInput, obj) !== undefined
}
import { hasPath } from './hasPath.js'
test('when true', () => {
const path = 'a.b'
const obj = { a : { b : [] } }
const result = hasPath(path)(obj)
const expectedResult = true
expect(result).toEqual(expectedResult)
})
test('when false', () => {
const path = 'a.b'
const obj = {}
const result = hasPath(path, obj)
const expectedResult = false
expect(result).toEqual(expectedResult)
})
import {hasPath} from 'rambda'
describe('R.hasPath', () => {
it('string path', () => {
const obj = {a: {b: 1}}
const result = hasPath('a.b', obj)
const curriedResult = hasPath('a.c')(obj)
result // $ExpectType boolean
curriedResult // $ExpectType boolean
})
it('array path', () => {
const obj = {a: {b: 1}}
const result = hasPath(['a', 'b'], obj)
const curriedResult = hasPath(['a', 'c'])(obj)
result // $ExpectType boolean
curriedResult // $ExpectType boolean
})
})
head(str: string): string
It returns the first element of list or string input
.
Try this R.head example in Rambda REPL
head(str: string): string;
head(str: ''): undefined;
head<T>(list: never[]): undefined;
head<T extends unknown[]>(array: T): FirstArrayElement<T>
head<T extends readonly unknown[]>(array: T): FirstArrayElement<T>
export function head(listOrString){
if (typeof listOrString === 'string') return listOrString[ 0 ] || ''
return listOrString[ 0 ]
}
import { head } from './head.js'
test('head', () => {
expect(head([ 'fi', 'fo', 'fum' ])).toBe('fi')
expect(head([])).toBeUndefined()
expect(head('foo')).toBe('f')
expect(head('')).toBe('')
})
import {
emptyList,
emptyString,
mixedList,
mixedListConst,
numberList,
numberListConst,
string,
} from '_internals/typescriptTestUtils'
import {head, last} from 'rambda'
describe('R.head', () => {
it('string', () => {
head(string) // $ExpectType string
last(string) // $ExpectType string
})
it('empty string', () => {
head(emptyString) // $ExpectType undefined
last(emptyString) // $ExpectType undefined
})
it('array', () => {
head(numberList) // $ExpectType number
head(numberListConst) // $ExpectType 1
last(numberList) // $ExpectType number
last(numberListConst) // $ExpectType 3
})
it('empty array', () => {
const list = [] as const
head(emptyList) // $ExpectType undefined
head(list) // $ExpectType never
last(emptyList) // $ExpectType undefined
last(list) // $ExpectType never
})
it('mixed', () => {
head(mixedList) // $ExpectType string | number
head(mixedListConst) // $ExpectType 1
last(mixedList) // $ExpectType string | number
last(mixedListConst) // $ExpectType "bar"
})
})
It returns true
if its arguments a
and b
are identical.
Otherwise, it returns false
.
Try this R.identical example in Rambda REPL
identity<T>(input: T): T
It just passes back the supplied input
argument.
Try this R.identity example in Rambda REPL
identity<T>(input: T): T;
export function identity(x){
return x
}
import { identity } from './identity.js'
test('happy', () => {
expect(identity(7)).toBe(7)
expect(identity(true)).toBeTrue()
expect(identity({ a : 1 })).toEqual({ a : 1 })
})
import {identity} from 'rambda'
describe('R.identity', () => {
it('happy', () => {
const result = identity(4)
result // $ExpectType 4
})
})
ifElse<T, TFiltered extends T, TOnTrueResult, TOnFalseResult>(
pred: (a: T) => a is TFiltered,
onTrue: (a: TFiltered) => TOnTrueResult,
onFalse: (a: Exclude<T, TFiltered>) => TOnFalseResult,
): (a: T) => TOnTrueResult | TOnFalseResult
It expects condition
, onTrue
and onFalse
functions as inputs and it returns a new function with example name of fn
.
When fn`` is called with
inputargument, it will return either
onTrue(input)or
onFalse(input)depending on
condition(input)` evaluation.
Try this R.ifElse example in Rambda REPL
ifElse<T, TFiltered extends T, TOnTrueResult, TOnFalseResult>(
pred: (a: T) => a is TFiltered,
onTrue: (a: TFiltered) => TOnTrueResult,
onFalse: (a: Exclude<T, TFiltered>) => TOnFalseResult,
): (a: T) => TOnTrueResult | TOnFalseResult;
ifElse<TArgs extends any[], TOnTrueResult, TOnFalseResult>(fn: (...args: TArgs) => boolean, onTrue: (...args: TArgs) => TOnTrueResult, onFalse: (...args: TArgs) => TOnFalseResult): (...args: TArgs) => TOnTrueResult | TOnFalseResult;
import { curry } from './curry.js'
function ifElseFn(
condition, onTrue, onFalse
){
return (...input) => {
const conditionResult =
typeof condition === 'boolean' ? condition : condition(...input)
if (conditionResult === true){
return onTrue(...input)
}
return onFalse(...input)
}
}
export const ifElse = curry(ifElseFn)
import { always } from './always.js'
import { has } from './has.js'
import { identity } from './identity.js'
import { ifElse } from './ifElse.js'
import { prop } from './prop.js'
const condition = has('foo')
const v = function (a){
return typeof a === 'number'
}
const t = function (a){
return a + 1
}
const ifFn = x => prop('foo', x).length
const elseFn = () => false
test('happy', () => {
const fn = ifElse(condition, ifFn)(elseFn)
expect(fn({ foo : 'bar' })).toBe(3)
expect(fn({ fo : 'bar' })).toBeFalse()
})
test('ramda spec', () => {
const ifIsNumber = ifElse(v)
expect(ifIsNumber(t, identity)(15)).toBe(16)
expect(ifIsNumber(t, identity)('hello')).toBe('hello')
})
test('pass all arguments', () => {
const identity = function (a){
return a
}
const v = function (){
return true
}
const onTrue = function (a, b){
expect(a).toBe(123)
expect(b).toBe('abc')
}
ifElse(
v, onTrue, identity
)(123, 'abc')
})
test('accept constant as condition', () => {
const fn = ifElse(true)(always(true))(always(false))
expect(fn()).toBeTrue()
})
test('accept constant as condition - case 2', () => {
const fn = ifElse(
false, always(true), always(false)
)
expect(fn()).toBeFalse()
})
test('curry 1', () => {
const fn = ifElse(condition, ifFn)(elseFn)
expect(fn({ foo : 'bar' })).toBe(3)
expect(fn({ fo : 'bar' })).toBeFalse()
})
test('curry 2', () => {
const fn = ifElse(condition)(ifFn)(elseFn)
expect(fn({ foo : 'bar' })).toBe(3)
expect(fn({ fo : 'bar' })).toBeFalse()
})
test('simple arity of 1', () => {
const condition = x => x > 5
const onTrue = x => x + 1
const onFalse = x => x + 10
const result = ifElse(
condition, onTrue, onFalse
)(1)
expect(result).toBe(11)
})
test('simple arity of 2', () => {
const condition = (x, y) => x + y > 5
const onTrue = (x, y) => x + y + 1
const onFalse = (x, y) => x + y + 10
const result = ifElse(
condition, onTrue, onFalse
)(1, 10)
expect(result).toBe(12)
})
import {ifElse} from 'rambda'
describe('R.ifElse', () => {
it('happy', () => {
const condition = (x: number) => x > 5
const onTrue = (x: number) => `foo${x}`
const onFalse = (x: number) => `bar${x}`
const fn = ifElse(condition, onTrue, onFalse)
fn // $ExpectType (x: number) => string
const result = fn(3)
result // $ExpectType string
})
it('arity of 2', () => {
const condition = (x: number, y: string) => x + y.length > 5
const onTrue = (x: number, y: string) => `foo${x}-${y}`
const onFalse = (x: number, y: string) => `bar${x}-${y}`
const fn = ifElse(condition, onTrue, onFalse)
fn // $ExpectType (x: number, y: string) => string
const result = fn(3, 'hello')
result // $ExpectType string
})
test('DefinitelyTyped#59291', () => {
const getLengthIfStringElseDouble = ifElse(
(a: string | number): a is string => true,
a => a.length,
a => a * 2
)
getLengthIfStringElseDouble('foo') // $ExpectType number
getLengthIfStringElseDouble(3) // $ExpectType number
const result = ifElse(
(a: {
foo?: string,
bar: number | string,
}): a is {foo: string, bar: string} => true,
(a): [string, string] => [a.foo, a.bar],
(a): [string | undefined, string | number] => [a.foo, a.bar]
)
result // $ExpectType (a: { foo?: string | undefined; bar: string | number; }) => [string, string] | [string | undefined, string | number]
})
})
It increments a number.
Try this R.inc example in Rambda REPL
includes<T extends string>(valueToFind: T, input: string): boolean
If input
is string, then this method work as native String.includes
.
If input
is array, then R.equals
is used to define if valueToFind
belongs to the list.
Try this R.includes example in Rambda REPL
includes<T extends string>(valueToFind: T, input: string): boolean;
includes<T extends string>(valueToFind: T): (input: string) => boolean;
includes<T>(valueToFind: T, input: T[]): boolean;
includes<T>(valueToFind: T): (input: T[]) => boolean;
import { isArray } from './_internals/isArray.js'
import { _indexOf } from './equals.js'
export function includes(valueToFind, iterable){
if (arguments.length === 1)
return _iterable => includes(valueToFind, _iterable)
if (typeof iterable === 'string'){
return iterable.includes(valueToFind)
}
if (!iterable){
throw new TypeError(`Cannot read property \'indexOf\' of ${ iterable }`)
}
if (!isArray(iterable)) return false
return _indexOf(valueToFind, iterable) > -1
}
import { includes as includesRamda } from 'ramda'
import { includes } from './includes.js'
test('with string as iterable', () => {
const str = 'foo bar'
expect(includes('bar')(str)).toBeTrue()
expect(includesRamda('bar')(str)).toBeTrue()
expect(includes('never', str)).toBeFalse()
expect(includesRamda('never', str)).toBeFalse()
})
test('with array as iterable', () => {
const arr = [ 1, 2, 3 ]
expect(includes(2)(arr)).toBeTrue()
expect(includesRamda(2)(arr)).toBeTrue()
expect(includes(4, arr)).toBeFalse()
expect(includesRamda(4, arr)).toBeFalse()
})
test('with list of objects as iterable', () => {
const arr = [ { a : 1 }, { b : 2 }, { c : 3 } ]
expect(includes({ c : 3 }, arr)).toBeTrue()
expect(includesRamda({ c : 3 }, arr)).toBeTrue()
})
test('with NaN', () => {
const result = includes(NaN, [ NaN ])
const ramdaResult = includesRamda(NaN, [ NaN ])
expect(result).toBeTrue()
expect(ramdaResult).toBeTrue()
})
test('with wrong input that does not throw', () => {
const result = includes(1, /foo/g)
const ramdaResult = includesRamda(1, /foo/g)
expect(result).toBeFalse()
expect(ramdaResult).toBeFalse()
})
test('throws on wrong input - match ramda behaviour', () => {
expect(() => includes(2, null)).toThrowWithMessage(TypeError,
'Cannot read property \'indexOf\' of null')
expect(() => includesRamda(2, null)).toThrowWithMessage(TypeError,
'Cannot read properties of null (reading \'indexOf\')')
expect(() => includes(2, undefined)).toThrowWithMessage(TypeError,
'Cannot read property \'indexOf\' of undefined')
expect(() => includesRamda(2, undefined)).toThrowWithMessage(TypeError,
'Cannot read properties of undefined (reading \'indexOf\')')
})
import {includes} from 'rambda'
const list = [{a: {b: '1'}}, {a: {c: '2'}}, {a: {b: '3'}}]
describe('R.includes', () => {
it('happy', () => {
const result = includes({a: {b: '1'}}, list)
result // $ExpectType boolean
const result2 = includes('oo', ['f', 'oo'])
result2 // $ExpectType boolean
})
it('with string', () => {
const str = 'foo' as 'foo' | 'bar'
const result = includes('oo', str)
const curriedResult = includes('oo')(str)
result // $ExpectType boolean
curriedResult // $ExpectType boolean
})
})
It generates object with properties provided by condition
and values provided by list
array.
If condition
is a function, then all list members are passed through it.
If condition
is a string, then all list members are passed through R.path(condition)
.
Try this R.indexBy example in Rambda REPL
It returns the index of the first element of list
equals to valueToFind
.
If there is no such element, it returns -1
.
Try this R.indexOf example in Rambda REPL
init<T extends unknown[]>(input: T): T extends readonly [...infer U, any] ? U : [...T]
It returns all but the last element of list or string input
.
Try this R.init example in Rambda REPL
init<T extends unknown[]>(input: T): T extends readonly [...infer U, any] ? U : [...T];
init(input: string): string;
import baseSlice from './_internals/baseSlice.js'
export function init(listOrString){
if (typeof listOrString === 'string') return listOrString.slice(0, -1)
return listOrString.length ?
baseSlice(
listOrString, 0, -1
) :
[]
}
import { init } from './init.js'
test('with array', () => {
expect(init([ 1, 2, 3 ])).toEqual([ 1, 2 ])
expect(init([ 1, 2 ])).toEqual([ 1 ])
expect(init([ 1 ])).toEqual([])
expect(init([])).toEqual([])
expect(init([])).toEqual([])
expect(init([ 1 ])).toEqual([])
})
test('with string', () => {
expect(init('foo')).toBe('fo')
expect(init('f')).toBe('')
expect(init('')).toBe('')
})
import {init} from 'rambda'
describe('R.init', () => {
it('with string', () => {
const result = init('foo')
result // $ExpectType string
})
it('with list - one type', () => {
const result = init([1, 2, 3])
result // $ExpectType number[]
})
it('with list - mixed types', () => {
const result = init([1, 2, 3, 'foo', 'bar'])
result // $ExpectType (string | number)[]
})
})
It returns a new list by applying a predicate
function to all elements of list1
and list2
and keeping only these elements where predicate
returns true
.
Try this R.innerJoin example in Rambda REPL
It loops through listA
and listB
and returns the intersection of the two according to R.equals
.
Try this R.intersection example in Rambda REPL
It adds a separator
between members of list
.
Try this R.intersperse example in Rambda REPL
It returns true
if x
is instance of targetPrototype
.
Try this R.is example in Rambda REPL
isEmpty<T>(x: T): boolean
It returns true
if x
is empty
.
Try this R.isEmpty example in Rambda REPL
isEmpty<T>(x: T): boolean;
import { type } from './type.js'
export function isEmpty(input){
const inputType = type(input)
if ([ 'Undefined', 'NaN', 'Number', 'Null' ].includes(inputType))
return false
if (!input) return true
if (inputType === 'Object'){
return Object.keys(input).length === 0
}
if (inputType === 'Array'){
return input.length === 0
}
return false
}
import { isEmpty } from './isEmpty.js'
test('happy', () => {
expect(isEmpty(undefined)).toBeFalse()
expect(isEmpty('')).toBeTrue()
expect(isEmpty(null)).toBeFalse()
expect(isEmpty(' ')).toBeFalse()
expect(isEmpty(new RegExp(''))).toBeFalse()
expect(isEmpty([])).toBeTrue()
expect(isEmpty([ [] ])).toBeFalse()
expect(isEmpty({})).toBeTrue()
expect(isEmpty({ x : 0 })).toBeFalse()
expect(isEmpty(0)).toBeFalse()
expect(isEmpty(NaN)).toBeFalse()
expect(isEmpty([ '' ])).toBeFalse()
})
import {isEmpty} from 'rambda'
describe('R.isEmpty', () => {
it('happy', () => {
const result = isEmpty('foo')
result // $ExpectType boolean
})
})
isNil(x: any): x is null | undefined
It returns true
if x
is either null
or undefined
.
Try this R.isNil example in Rambda REPL
isNil(x: any): x is null | undefined;
export function isNil(x){
return x === undefined || x === null
}
import { isNil } from './isNil.js'
test('happy', () => {
expect(isNil(null)).toBeTrue()
expect(isNil(undefined)).toBeTrue()
expect(isNil([])).toBeFalse()
})
join<T>(glue: string, list: T[]): string
It returns a string of all list
instances joined with a glue
.
Try this R.join example in Rambda REPL
join<T>(glue: string, list: T[]): string;
join<T>(glue: string): (list: T[]) => string;
export function join(glue, list){
if (arguments.length === 1) return _list => join(glue, _list)
return list.join(glue)
}
import { join } from './join.js'
test('curry', () => {
expect(join('|')([ 'foo', 'bar', 'baz' ])).toBe('foo|bar|baz')
expect(join('|', [ 1, 2, 3 ])).toBe('1|2|3')
const spacer = join(' ')
expect(spacer([ 'a', 2, 3.4 ])).toBe('a 2 3.4')
})
import {join} from 'rambda'
describe('R.join', () => {
it('happy', () => {
const result = join('|', [1, 2, 3])
result // $ExpectType string
})
})
juxt<A extends any[], R1>(fns: [(...a: A) => R1]): (...a: A) => [R1]
It applies list of function to a list of inputs.
Try this R.juxt example in Rambda REPL
juxt<A extends any[], R1>(fns: [(...a: A) => R1]): (...a: A) => [R1];
juxt<A extends any[], R1, R2>(fns: [(...a: A) => R1, (...a: A) => R2]): (...a: A) => [R1, R2];
juxt<A extends any[], R1, R2, R3>(fns: [(...a: A) => R1, (...a: A) => R2, (...a: A) => R3]): (...a: A) => [R1, R2, R3];
juxt<A extends any[], R1, R2, R3, R4>(fns: [(...a: A) => R1, (...a: A) => R2, (...a: A) => R3, (...a: A) => R4]): (...a: A) => [R1, R2, R3, R4];
juxt<A extends any[], R1, R2, R3, R4, R5>(fns: [(...a: A) => R1, (...a: A) => R2, (...a: A) => R3, (...a: A) => R4, (...a: A) => R5]): (...a: A) => [R1, R2, R3, R4, R5];
juxt<A extends any[], U>(fns: Array<(...args: A) => U>): (...args: A) => U[];
export function juxt(listOfFunctions){
return (...args) => listOfFunctions.map(fn => fn(...args))
}
import { juxt } from './juxt.js'
test('happy', () => {
const fn = juxt([ Math.min, Math.max, Math.min ])
const result = fn(
3, 4, 9, -3
)
expect(result).toEqual([ -3, 9, -3 ])
})
import {juxt} from 'rambda'
describe('R.juxt', () => {
it('happy', () => {
const fn = juxt([Math.min, Math.max])
const result = fn(3, 4, 9, -3)
result // $ExpectType [number, number]
})
})
keys<T extends object>(x: T): (keyof T & string)[]
It applies Object.keys
over x
and returns its keys.
Try this R.keys example in Rambda REPL
keys<T extends object>(x: T): (keyof T & string)[];
keys<T>(x: T): string[];
export function keys(x){
return Object.keys(x)
}
import { keys } from './keys.js'
test('happy', () => {
expect(keys({ a : 1 })).toEqual([ 'a' ])
})
last(str: ''): undefined
It returns the last element of input
, as the input
can be either a string or an array.
Try this R.last example in Rambda REPL
last(str: ''): undefined;
last(str: string): string;
last(list: never[]): undefined;
last<T extends unknown[]>(array: T): LastArrayElement<T>
last<T extends readonly unknown[]>(array: T): LastArrayElement<T>
export function last(listOrString){
if (typeof listOrString === 'string'){
return listOrString[ listOrString.length - 1 ] || ''
}
return listOrString[ listOrString.length - 1 ]
}
import { last } from './last.js'
test('with list', () => {
expect(last([ 1, 2, 3 ])).toBe(3)
expect(last([])).toBeUndefined()
})
test('with string', () => {
expect(last('abc')).toBe('c')
expect(last('')).toBe('')
})
lastIndexOf<T>(target: T, list: T[]): number
It returns the last index of target
in list
array.
R.equals
is used to determine equality between target
and members of list
.
If there is no such index, then -1
is returned.
Try this R.lastIndexOf example in Rambda REPL
lastIndexOf<T>(target: T, list: T[]): number;
lastIndexOf<T>(target: T): (list: T[]) => number;
import { _lastIndexOf } from './equals.js'
export function lastIndexOf(valueToFind, list){
if (arguments.length === 1){
return _list => _lastIndexOf(valueToFind, _list)
}
return _lastIndexOf(valueToFind, list)
}
import { lastIndexOf as lastIndexOfRamda } from 'ramda'
import { compareCombinations } from './_internals/testUtils.js'
import { possibleIterables, possibleTargets } from './indexOf.spec.js'
import { lastIndexOf } from './lastIndexOf.js'
test('with NaN', () => {
expect(lastIndexOf(NaN, [ NaN ])).toBe(0)
})
test('will throw with bad input', () => {
expect(lastIndexOfRamda([], true)).toBe(-1)
expect(() => indexOf([], true)).toThrowErrorMatchingInlineSnapshot('"indexOf is not defined"')
})
test('without list of objects - no R.equals', () => {
expect(lastIndexOf(3, [ 1, 2, 3, 4 ])).toBe(2)
expect(lastIndexOf(10)([ 1, 2, 3, 4 ])).toBe(-1)
})
test('list of objects uses R.equals', () => {
const listOfObjects = [ { a : 1 }, { b : 2 }, { c : 3 } ]
expect(lastIndexOf({ c : 4 }, listOfObjects)).toBe(-1)
expect(lastIndexOf({ c : 3 }, listOfObjects)).toBe(2)
})
test('list of arrays uses R.equals', () => {
const listOfLists = [ [ 1 ], [ 2, 3 ], [ 2, 3, 4 ], [ 2, 3 ], [ 1 ], [] ]
expect(lastIndexOf([], listOfLists)).toBe(5)
expect(lastIndexOf([ 1 ], listOfLists)).toBe(4)
expect(lastIndexOf([ 2, 3, 4 ], listOfLists)).toBe(2)
expect(lastIndexOf([ 2, 3, 5 ], listOfLists)).toBe(-1)
})
test('with string as iterable', () => {
expect(() => lastIndexOf('a', 'abc')).toThrowErrorMatchingInlineSnapshot('"Cannot read property \'indexOf\' of abc"')
expect(lastIndexOfRamda('a', 'abc')).toBe(0)
})
describe('brute force', () => {
compareCombinations({
fn : lastIndexOf,
fnRamda : lastIndexOfRamda,
firstInput : possibleTargets,
secondInput : possibleIterables,
callback : errorsCounters => {
expect(errorsCounters).toMatchInlineSnapshot(`
{
"ERRORS_MESSAGE_MISMATCH": 0,
"ERRORS_TYPE_MISMATCH": 34,
"RESULTS_MISMATCH": 0,
"SHOULD_NOT_THROW": 51,
"SHOULD_THROW": 0,
"TOTAL_TESTS": 170,
}
`)
},
})
})
import {lastIndexOf} from 'rambda'
const list = [1, 2, 3]
describe('R.lastIndexOf', () => {
it('happy', () => {
const result = lastIndexOf(2, list)
result // $ExpectType number
})
it('curried', () => {
const result = lastIndexOf(2)(list)
result // $ExpectType number
})
})
length<T>(input: T[]): number
It returns the length
property of list or string input
.
Try this R.length example in Rambda REPL
length<T>(input: T[]): number;
import { isArray } from './_internals/isArray.js'
export function length(x){
if (isArray(x)) return x.length
if (typeof x === 'string') return x.length
return NaN
}
import { length as lengthRamda } from 'ramda'
import { length } from './length.js'
test('happy', () => {
expect(length('foo')).toBe(3)
expect(length([ 1, 2, 3 ])).toBe(3)
expect(length([])).toBe(0)
})
test('with empty string', () => {
expect(length('')).toBe(0)
})
test('with bad input returns NaN', () => {
expect(length(0)).toBeNaN()
expect(length({})).toBeNaN()
expect(length(null)).toBeNaN()
expect(length(undefined)).toBeNaN()
})
test('with length as property', () => {
const input1 = { length : '123' }
const input2 = { length : null }
const input3 = { length : '' }
expect(length(input1)).toBeNaN()
expect(lengthRamda(input1)).toBeNaN()
expect(length(input2)).toBeNaN()
expect(lengthRamda(input2)).toBeNaN()
expect(length(input3)).toBeNaN()
expect(lengthRamda(input3)).toBeNaN()
})
lens<S, A>(getter: (s: S) => A, setter: (a: A, s: S) => S): Lens<S, A>
It returns a lens
for the given getter
and setter
functions.
The getter
gets the value of the focus; the setter
sets the value of the focus.
The setter should not mutate the data structure.
Try this R.lens example in Rambda REPL
lens<S, A>(getter: (s: S) => A, setter: (a: A, s: S) => S): Lens<S, A>;
export function lens(getter, setter){
return function (functor){
return function (target){
return functor(getter(target)).map(focus => setter(focus, target))
}
}
}
import {lens, assoc, lensProp, view, lensIndex, lensPath} from 'rambda'
interface Input {
foo: string,
}
const testObject: Input = {
foo: 'Jazz',
}
describe('R.lens', () => {
it('happy', () => {
const fn = lens<Input, string>((x: Input) => {
x.foo // $ExpectType string
return x.foo
}, assoc('name'))
fn // $ExpectType Lens<Input, string>
})
})
describe('R.lensProp', () => {
it('happy', () => {
const result = view<Input, string>(lensProp('foo'), testObject)
result // $ExpectType string
})
})
describe('R.lensIndex', () => {
const testList: Input[] = [{foo: 'bar'}, {foo: 'baz'}]
it('happy', () => {
const result = view<Input[], Input>(lensIndex(0), testList)
result // $ExpectType Input
result.foo // $ExpectType string
})
})
describe('R.lensPath', () => {
const path = lensPath(['bar', 'a'])
it('happy', () => {
const result = view<Input, string>(path, testObject)
result // $ExpectType string
})
})
describe('R.view', () => {
const fooLens = lens<Input, string>((x: Input) => {
return x.foo
}, assoc('foo'))
it('happt', () => {
const result = view<Input, string>(fooLens, testObject)
result // $ExpectType string
})
})
lensIndex<A>(n: number): Lens<A[], A>
It returns a lens that focuses on specified index
.
Try this R.lensIndex example in Rambda REPL
lensIndex<A>(n: number): Lens<A[], A>;
lensIndex<A extends any[], N extends number>(n: N): Lens<A, A[N]>;
import { lens } from './lens.js'
import { nth } from './nth.js'
import { update } from './update.js'
export function lensIndex(index){
return lens(nth(index), update(index))
}
import { compose } from './compose.js'
import { keys } from './keys.js'
import { lensIndex } from './lensIndex.js'
import { over } from './over.js'
import { set } from './set.js'
import { view } from './view.js'
const testList = [ { a : 1 }, { b : 2 }, { c : 3 } ]
test('focuses list element at the specified index', () => {
expect(view(lensIndex(0), testList)).toEqual({ a : 1 })
})
test('returns undefined if the specified index does not exist', () => {
expect(view(lensIndex(10), testList)).toBeUndefined()
})
test('sets the list value at the specified index', () => {
expect(set(
lensIndex(0), 0, testList
)).toEqual([ 0, { b : 2 }, { c : 3 } ])
})
test('applies function to the value at the specified list index', () => {
expect(over(
lensIndex(2), keys, testList
)).toEqual([ { a : 1 }, { b : 2 }, [ 'c' ] ])
})
test('can be composed', () => {
const nestedList = [ 0, [ 10, 11, 12 ], 1, 2 ]
const composedLens = compose(lensIndex(1), lensIndex(0))
expect(view(composedLens, nestedList)).toBe(10)
})
test('set s (get s) === s', () => {
expect(set(
lensIndex(0), view(lensIndex(0), testList), testList
)).toEqual(testList)
})
test('get (set s v) === v', () => {
expect(view(lensIndex(0), set(
lensIndex(0), 0, testList
))).toBe(0)
})
test('get (set(set s v1) v2) === v2', () => {
expect(view(lensIndex(0),
set(
lensIndex(0), 11, set(
lensIndex(0), 10, testList
)
))).toBe(11)
})
It returns a lens that focuses on specified path
.
Try this R.lensPath example in Rambda REPL
lensProp<S, K extends keyof S = keyof S>(prop: K): Lens<S, S[K]>
It returns a lens that focuses on specified property prop
.
Try this R.lensProp example in Rambda REPL
lensProp<S, K extends keyof S = keyof S>(prop: K): Lens<S, S[K]>;
import { assoc } from './assoc.js'
import { lens } from './lens.js'
import { prop } from './prop.js'
export function lensProp(key){
return lens(prop(key), assoc(key))
}
import { compose } from './compose.js'
import { identity } from './identity.js'
import { inc } from './inc.js'
import { lensProp } from './lensProp.js'
import { over } from './over.js'
import { set } from './set.js'
import { view } from './view.js'
const testObj = {
a : 1,
b : 2,
c : 3,
}
test('focuses object the specified object property', () => {
expect(view(lensProp('a'), testObj)).toBe(1)
})
test('returns undefined if the specified property does not exist', () => {
expect(view(lensProp('X'), testObj)).toBeUndefined()
})
test('sets the value of the object property specified', () => {
expect(set(
lensProp('a'), 0, testObj
)).toEqual({
a : 0,
b : 2,
c : 3,
})
})
test('adds the property to the object if it doesn\'t exist', () => {
expect(set(
lensProp('d'), 4, testObj
)).toEqual({
a : 1,
b : 2,
c : 3,
d : 4,
})
})
test('applies function to the value of the specified object property', () => {
expect(over(
lensProp('a'), inc, testObj
)).toEqual({
a : 2,
b : 2,
c : 3,
})
})
test('applies function to undefined and adds the property if it doesn\'t exist', () => {
expect(over(
lensProp('X'), identity, testObj
)).toEqual({
a : 1,
b : 2,
c : 3,
X : undefined,
})
})
test('can be composed', () => {
const nestedObj = {
a : { b : 1 },
c : 2,
}
const composedLens = compose(lensProp('a'), lensProp('b'))
expect(view(composedLens, nestedObj)).toBe(1)
})
test('set s (get s) === s', () => {
expect(set(
lensProp('a'), view(lensProp('a'), testObj), testObj
)).toEqual(testObj)
})
test('get (set s v) === v', () => {
expect(view(lensProp('a'), set(
lensProp('a'), 0, testObj
))).toBe(0)
})
test('get (set(set s v1) v2) === v2', () => {
expect(view(lensProp('a'),
set(
lensProp('a'), 11, set(
lensProp('a'), 10, testObj
)
))).toBe(11)
})
Try this R.lt example in Rambda REPL
map<T, U>(fn: ObjectIterator<T, U>, iterable: Dictionary<T>): Dictionary<U>
It returns the result of looping through iterable
with fn
.
It works with both array and object.
Try this R.map example in Rambda REPL
map<T, U>(fn: ObjectIterator<T, U>, iterable: Dictionary<T>): Dictionary<U>;
map<T, U>(fn: Iterator<T, U>, iterable: T[]): U[];
map<T, U>(fn: Iterator<T, U>): (iterable: T[]) => U[];
map<T, U, S>(fn: ObjectIterator<T, U>): (iterable: Dictionary<T>) => Dictionary<U>;
map<T>(fn: Iterator<T, T>): (iterable: T[]) => T[];
map<T>(fn: Iterator<T, T>, iterable: T[]): T[];
import { INCORRECT_ITERABLE_INPUT } from './_internals/constants.js'
import { isArray } from './_internals/isArray.js'
import { keys } from './_internals/keys.js'
export function mapArray(
fn, list, isIndexed = false
){
let index = 0
const willReturn = Array(list.length)
while (index < list.length){
willReturn[ index ] = isIndexed ? fn(list[ index ], index) : fn(list[ index ])
index++
}
return willReturn
}
export function mapObject(fn, obj){
if (arguments.length === 1){
return _obj => mapObject(fn, _obj)
}
let index = 0
const objKeys = keys(obj)
const len = objKeys.length
const willReturn = {}
while (index < len){
const key = objKeys[ index ]
willReturn[ key ] = fn(
obj[ key ], key, obj
)
index++
}
return willReturn
}
export const mapObjIndexed = mapObject
export function map(fn, iterable){
if (arguments.length === 1) return _iterable => map(fn, _iterable)
if (!iterable){
throw new Error(INCORRECT_ITERABLE_INPUT)
}
if (isArray(iterable)) return mapArray(fn, iterable)
return mapObject(fn, iterable)
}
import { map as mapRamda } from 'ramda'
import { map } from './map.js'
const double = x => x * 2
describe('with array', () => {
it('happy', () => {
expect(map(double, [ 1, 2, 3 ])).toEqual([ 2, 4, 6 ])
})
it('curried', () => {
expect(map(double)([ 1, 2, 3 ])).toEqual([ 2, 4, 6 ])
})
})
describe('with object', () => {
const obj = {
a : 1,
b : 2,
}
it('happy', () => {
expect(map(double, obj)).toEqual({
a : 2,
b : 4,
})
})
it('property as second and input object as third argument', () => {
const obj = {
a : 1,
b : 2,
}
const iterator = (
val, prop, inputObject
) => {
expect(prop).toBeString()
expect(inputObject).toEqual(obj)
return val * 2
}
expect(map(iterator)(obj)).toEqual({
a : 2,
b : 4,
})
})
})
test('bad inputs difference between Ramda and Rambda', () => {
expect(() => map(double, null)).toThrowErrorMatchingInlineSnapshot('"Incorrect iterable input"')
expect(() => map(double)(undefined)).toThrowErrorMatchingInlineSnapshot('"Incorrect iterable input"')
expect(() => mapRamda(double, null)).toThrowErrorMatchingInlineSnapshot('"Cannot read properties of null (reading \'fantasy-land/map\')"')
expect(() =>
mapRamda(double, undefined)).toThrowErrorMatchingInlineSnapshot('"Cannot read properties of undefined (reading \'fantasy-land/map\')"')
})
import {map} from 'rambda'
describe('R.map with arrays', () => {
it('iterable returns the same type as the input', () => {
const result = map<number>(
(x: number) => {
x // $ExpectType number
return x + 2
},
[1, 2, 3]
)
result // $ExpectType number[]
})
it('iterable returns the same type as the input - curried', () => {
const result = map<number>((x: number) => {
x // $ExpectType number
return x + 2
})([1, 2, 3])
result // $ExpectType number[]
})
it('iterable returns different type as the input', () => {
const result = map<number, string>(
(x: number) => {
x // $ExpectType number
return String(x)
},
[1, 2, 3]
)
result // $ExpectType string[]
})
})
describe('R.map with objects', () => {
it('iterable with all three arguments - curried', () => {
// It requires dummy third typing argument
// in order to identify compared to curry typings for arrays
// ============================================
const result = map<number, string, any>((a, b, c) => {
a // $ExpectType number
b // $ExpectType string
c // $ExpectType Dictionary<number>
return `${a}`
})({a: 1, b: 2})
result // $ExpectType Dictionary<string>
})
it('iterable with all three arguments', () => {
const result = map<number, string>(
(a, b, c) => {
a // $ExpectType number
b // $ExpectType string
c // $ExpectType Dictionary<number>
return `${a}`
},
{a: 1, b: 2}
)
result // $ExpectType Dictionary<string>
})
it('iterable with property argument', () => {
const result = map<number, string>(
(a, b) => {
a // $ExpectType number
b // $ExpectType string
return `${a}`
},
{a: 1, b: 2}
)
result // $ExpectType Dictionary<string>
})
it('iterable with no property argument', () => {
const result = map<number, string>(
a => {
a // $ExpectType number
return `${a}`
},
{a: 1, b: 2}
)
result // $ExpectType Dictionary<string>
})
})
mapObjIndexed<T>(fn: ObjectIterator<T, T>, iterable: Dictionary<T>): Dictionary<T>
It works the same way as R.map
does for objects. It is added as Ramda also has this method.
Try this R.mapObjIndexed example in Rambda REPL
mapObjIndexed<T>(fn: ObjectIterator<T, T>, iterable: Dictionary<T>): Dictionary<T>;
mapObjIndexed<T, U>(fn: ObjectIterator<T, U>, iterable: Dictionary<T>): Dictionary<U>;
mapObjIndexed<T>(fn: ObjectIterator<T, T>): (iterable: Dictionary<T>) => Dictionary<T>;
mapObjIndexed<T, U>(fn: ObjectIterator<T, U>): (iterable: Dictionary<T>) => Dictionary<U>;
import {mapObjIndexed} from 'rambda'
const obj = {a: 1, b: 2, c: 3}
describe('R.mapObjIndexed', () => {
it('without type transform', () => {
const result = mapObjIndexed((x, prop, obj) => {
x // $ExpectType number
prop // $ExpectType string
obj // $ExpectType Dictionary<number>
return x + 2
}, obj)
result // $ExpectType Dictionary<number>
})
it('without type transform - curried', () => {
const result = mapObjIndexed<number>((x, prop, obj) => {
x // $ExpectType number
prop // $ExpectType string
obj // $ExpectType Dictionary<number>
return x + 2
})(obj)
result // $ExpectType Dictionary<number>
})
it('change of type', () => {
const result = mapObjIndexed((x, prop, obj) => {
x // $ExpectType number
prop // $ExpectType string
obj // $ExpectType Dictionary<number>
return String(x + 2)
}, obj)
result // $ExpectType Dictionary<string>
})
it('change of type - curried', () => {
const result = mapObjIndexed<number, string>((x, prop, obj) => {
x // $ExpectType number
prop // $ExpectType string
obj // $ExpectType Dictionary<number>
return String(x + 2)
})(obj)
result // $ExpectType Dictionary<string>
})
})
match(regExpression: RegExp, str: string): string[]
Curried version of String.prototype.match
which returns empty array, when there is no match.
Try this R.match example in Rambda REPL
match(regExpression: RegExp, str: string): string[];
match(regExpression: RegExp): (str: string) => string[];
export function match(pattern, input){
if (arguments.length === 1) return _input => match(pattern, _input)
const willReturn = input.match(pattern)
return willReturn === null ? [] : willReturn
}
import { equals } from './equals.js'
import { match } from './match.js'
test('happy', () => {
expect(match(/a./g)('foo bar baz')).toEqual([ 'ar', 'az' ])
})
test('fallback', () => {
expect(match(/a./g)('foo')).toEqual([])
})
test('with string', () => {
expect(match('a', 'foo')).toEqual([])
expect(equals(match('o', 'foo'), [ 'o' ])).toBeTrue()
})
test('throwing', () => {
expect(() => {
match(/a./g, null)
}).toThrowErrorMatchingInlineSnapshot('"Cannot read properties of null (reading \'match\')"')
})
import {match} from 'rambda'
const str = 'foo bar'
describe('R.match', () => {
it('happy', () => {
const result = match(/foo/, str)
result // $ExpectType string[]
})
it('curried', () => {
const result = match(/foo/)(str)
result // $ExpectType string[]
})
})
R.mathMod
behaves like the modulo operator should mathematically, unlike the %
operator (and by extension, R.modulo
). So while -17 % 5
is -2
, mathMod(-17, 5)
is 3
.
Try this R.mathMod example in Rambda REPL
It returns the greater value between x
and y
.
Try this R.max example in Rambda REPL
It returns the greater value between x
and y
according to compareFn
function.
Try this R.maxBy example in Rambda REPL
mean(list: number[]): number
It returns the mean value of list
input.
Try this R.mean example in Rambda REPL
mean(list: number[]): number;
import { sum } from './sum.js'
export function mean(list){
return sum(list) / list.length
}
import { mean } from './mean.js'
test('happy', () => {
expect(mean([ 2, 7 ])).toBe(4.5)
})
test('with NaN', () => {
expect(mean([])).toBeNaN()
})
import {mean} from 'rambda'
describe('R.mean', () => {
it('happy', () => {
const result = mean([1, 2, 3])
result // $ExpectType number
})
})
median(list: number[]): number
It returns the median value of list
input.
Try this R.median example in Rambda REPL
median(list: number[]): number;
import { mean } from './mean.js'
export function median(list){
const len = list.length
if (len === 0) return NaN
const width = 2 - len % 2
const idx = (len - width) / 2
return mean(Array.prototype.slice
.call(list, 0)
.sort((a, b) => {
if (a === b) return 0
return a < b ? -1 : 1
})
.slice(idx, idx + width))
}
import { median } from './median.js'
test('happy', () => {
expect(median([ 2 ])).toBe(2)
expect(median([ 7, 2, 10, 2, 9 ])).toBe(7)
})
test('with empty array', () => {
expect(median([])).toBeNaN()
})
import {median} from 'rambda'
describe('R.median', () => {
it('happy', () => {
const result = median([1, 2, 3])
result // $ExpectType number
})
})
Same as R.mergeRight
.
mergeAll<T>(list: object[]): T
It merges all objects of list
array sequentially and returns the result.
Try this R.mergeAll example in Rambda REPL
mergeAll<T>(list: object[]): T;
mergeAll(list: object[]): object;
import { map } from './map.js'
import { mergeRight } from './mergeRight.js'
export function mergeAll(arr){
let willReturn = {}
map(val => {
willReturn = mergeRight(willReturn, val)
}, arr)
return willReturn
}
import { mergeAll } from './mergeAll.js'
test('case 1', () => {
const arr = [ { a : 1 }, { b : 2 }, { c : 3 } ]
const expectedResult = {
a : 1,
b : 2,
c : 3,
}
expect(mergeAll(arr)).toEqual(expectedResult)
})
test('case 2', () => {
expect(mergeAll([ { foo : 1 }, { bar : 2 }, { baz : 3 } ])).toEqual({
foo : 1,
bar : 2,
baz : 3,
})
})
describe('acts as if nil values are simply empty objects', () => {
it('if the first object is nil', () => {
expect(mergeAll([ null, { foo : 1 }, { foo : 2 }, { bar : 2 } ])).toEqual({
foo : 2,
bar : 2,
})
})
it('if the last object is nil', () => {
expect(mergeAll([ { foo : 1 }, { foo : 2 }, { bar : 2 }, undefined ])).toEqual({
foo : 2,
bar : 2,
})
})
it('if an intermediate object is nil', () => {
expect(mergeAll([ { foo : 1 }, { foo : 2 }, null, { bar : 2 } ])).toEqual({
foo : 2,
bar : 2,
})
})
})
import {mergeAll} from 'rambda'
describe('R.mergeAll', () => {
it('with passing type', () => {
interface Output {
foo: number,
bar: number,
}
const result = mergeAll<Output>([{foo: 1}, {bar: 2}])
result.foo // $ExpectType number
result.bar // $ExpectType number
})
it('without passing type', () => {
const result = mergeAll([{foo: 1}, {bar: 2}])
result // $ExpectType unknown
})
})
mergeDeepRight<Output>(target: object, newProps: object): Output
Creates a new object with the own properties of the first object merged with the own properties of the second object. If a key exists in both objects:
mergeDeepRight<Output>(target: object, newProps: object): Output;
mergeDeepRight<Output>(target: object): (newProps: object) => Output;
import { clone } from './clone.js'
import { type } from './type.js'
export function mergeDeepRight(target, source){
if (arguments.length === 1){
return sourceHolder => mergeDeepRight(target, sourceHolder)
}
const willReturn = clone(target)
Object.keys(source).forEach(key => {
if (type(source[ key ]) === 'Object'){
if (type(target[ key ]) === 'Object'){
willReturn[ key ] = mergeDeepRight(target[ key ], source[ key ])
} else {
willReturn[ key ] = source[ key ]
}
} else {
willReturn[ key ] = source[ key ]
}
})
return willReturn
}
import { mergeDeepRight } from './mergeDeepRight.js'
const student = {
name : 'foo',
age : 10,
contact : {
a : 1,
email : 'foo@example.com',
},
}
const teacher = {
age : 40,
contact : { email : 'baz@example.com' },
songs : { title : 'Remains the same' },
}
test('when merging object with lists inside them', () => {
const a = {
a : [ 1, 2, 3 ],
b : [ 4, 5, 6 ],
}
const b = {
a : [ 7, 8, 9 ],
b : [ 10, 11, 12 ],
}
const result = mergeDeepRight(a, b)
const expected = {
a : [ 7, 8, 9 ],
b : [ 10, 11, 12 ],
}
expect(result).toEqual(expected)
})
test('happy', () => {
const result = mergeDeepRight(student, teacher)
const curryResult = mergeDeepRight(student)(teacher)
const expected = {
age : 40,
name : 'foo',
contact : {
a : 1,
email : 'baz@example.com',
},
songs : { title : 'Remains the same' },
}
expect(result).toEqual(expected)
expect(curryResult).toEqual(expected)
})
test('issue 650', () => {
expect(Object.keys(mergeDeepRight({ a : () => {} }, { b : () => {} }))).toEqual([
'a',
'b',
])
})
test('ramda compatible test 1', () => {
const a = {
w : 1,
x : 2,
y : { z : 3 },
}
const b = {
a : 4,
b : 5,
c : { d : 6 },
}
const result = mergeDeepRight(a, b)
const expected = {
w : 1,
x : 2,
y : { z : 3 },
a : 4,
b : 5,
c : { d : 6 },
}
expect(result).toEqual(expected)
})
test('ramda compatible test 2', () => {
const a = {
a : {
b : 1,
c : 2,
},
y : 0,
}
const b = {
a : {
b : 3,
d : 4,
},
z : 0,
}
const result = mergeDeepRight(a, b)
const expected = {
a : {
b : 3,
c : 2,
d : 4,
},
y : 0,
z : 0,
}
expect(result).toEqual(expected)
})
test('ramda compatible test 3', () => {
const a = {
w : 1,
x : { y : 2 },
}
const result = mergeDeepRight(a, { x : { y : 3 } })
const expected = {
w : 1,
x : { y : 3 },
}
expect(result).toEqual(expected)
})
test('functions are not discarded', () => {
const obj = { foo : () => {} }
expect(typeof mergeDeepRight(obj, {}).foo).toBe('function')
})
import {mergeDeepRight} from 'rambda'
interface Output {
foo: {
bar: number,
},
}
describe('R.mergeDeepRight', () => {
const result = mergeDeepRight<Output>({foo: {bar: 1}}, {foo: {bar: 2}})
result.foo.bar // $ExpectType number
})
mergeLeft<Output>(newProps: object, target: object): Output
Same as R.merge
, but in opposite direction.
Try this R.mergeLeft example in Rambda REPL
mergeLeft<Output>(newProps: object, target: object): Output;
mergeLeft<Output>(newProps: object): (target: object) => Output;
import { mergeRight } from './mergeRight.js'
export function mergeLeft(x, y){
if (arguments.length === 1) return _y => mergeLeft(x, _y)
return mergeRight(y, x)
}
import { mergeLeft } from './mergeLeft.js'
const obj = {
foo : 1,
bar : 2,
}
test('happy', () => {
expect(mergeLeft({ bar : 20 }, obj)).toEqual({
foo : 1,
bar : 20,
})
})
test('curry', () => {
expect(mergeLeft({ baz : 3 })(obj)).toEqual({
foo : 1,
bar : 2,
baz : 3,
})
})
test('when undefined or null instead of object', () => {
expect(mergeLeft(null, undefined)).toEqual({})
expect(mergeLeft(obj, null)).toEqual(obj)
expect(mergeLeft(obj, undefined)).toEqual(obj)
expect(mergeLeft(undefined, obj)).toEqual(obj)
})
import {mergeLeft} from 'rambda'
interface Output {
foo: number,
bar: number,
}
describe('R.mergeLeft', () => {
const result = mergeLeft<Output>({foo: 1}, {bar: 2})
const curriedResult = mergeLeft<Output>({foo: 1})({bar: 2})
result.foo // $ExpectType number
result.bar // $ExpectType number
curriedResult.bar // $ExpectType number
})
It creates a copy of target
object with overwritten newProps
properties. Previously known as R.merge
but renamed after Ramda did the same.
Try this R.mergeRight example in Rambda REPL
mergeWith(fn: (x: any, z: any) => any, a: Record<string, unknown>, b: Record<string, unknown>): Record<string, unknown>
It takes two objects and a function, which will be used when there is an overlap between the keys.
Try this R.mergeWith example in Rambda REPL
mergeWith(fn: (x: any, z: any) => any, a: Record<string, unknown>, b: Record<string, unknown>): Record<string, unknown>;
mergeWith<Output>(fn: (x: any, z: any) => any, a: Record<string, unknown>, b: Record<string, unknown>): Output;
mergeWith(fn: (x: any, z: any) => any, a: Record<string, unknown>): (b: Record<string, unknown>) => Record<string, unknown>;
mergeWith<Output>(fn: (x: any, z: any) => any, a: Record<string, unknown>): (b: Record<string, unknown>) => Output;
mergeWith(fn: (x: any, z: any) => any): <U, V>(a: U, b: V) => Record<string, unknown>;
mergeWith<Output>(fn: (x: any, z: any) => any): <U, V>(a: U, b: V) => Output;
import { curry } from './curry.js'
export function mergeWithFn(
mergeFn, aInput, bInput
){
const a = aInput ?? {}
const b = bInput ?? {}
const willReturn = {}
Object.keys(a).forEach(key => {
if (b[ key ] === undefined) willReturn[ key ] = a[ key ]
else willReturn[ key ] = mergeFn(a[ key ], b[ key ])
})
Object.keys(b).forEach(key => {
if (willReturn[ key ] !== undefined) return
if (a[ key ] === undefined) willReturn[ key ] = b[ key ]
else willReturn[ key ] = mergeFn(a[ key ], b[ key ])
})
return willReturn
}
export const mergeWith = curry(mergeWithFn)
import { concat } from './concat.js'
import { mergeWithFn } from './mergeWith.js'
test('happy', () => {
const result = mergeWithFn(
concat,
{
a : true,
values : [ 10, 20 ],
},
{
b : true,
values : [ 15, 35 ],
}
)
const expected = {
a : true,
b : true,
values : [ 10, 20, 15, 35 ],
}
expect(result).toEqual(expected)
})
// https://github.com/ramda/ramda/pull/3222/files#diff-d925d9188b478d2f1d4b26012c6dddac374f9e9d7a336604d654b9a113bfc857
describe('acts as if nil values are simply empty objects', () => {
it('if the first object is nil and the second empty', () => {
expect(mergeWithFn(
concat, undefined, {}
)).toEqual({})
})
it('if the first object is empty and the second nil', () => {
expect(mergeWithFn(
concat, {}, null
)).toEqual({})
})
it('if both objects are nil', () => {
expect(mergeWithFn(
concat, undefined, null
)).toEqual({})
})
it('if the first object is not empty and the second is nil', () => {
expect(mergeWithFn(
concat, { a : 'a' }, null
)).toEqual({ a : 'a' })
})
it('if the first object is nil and the second is not empty', () => {
expect(mergeWithFn(
concat, undefined, { a : 'a' }
)).toEqual({ a : 'a' })
})
})
import {concat, mergeWith} from 'rambda'
interface Output {
a: boolean,
b: boolean,
values: number[],
}
const A = {
a: true,
values: [10, 20],
}
const B = {
b: true,
values: [15, 35],
}
describe('R.mergeWith', () => {
test('no curry | without explicit types', () => {
const result = mergeWith(concat, A, B)
result // $ExpectType Record<string, unknown>
})
test('no curry | with explicit types', () => {
const result = mergeWith<Output>(concat, A, B)
result // $ExpectType Output
})
test('curry 1 | without explicit types', () => {
const result = mergeWith(concat, A)(B)
result // $ExpectType Record<string, unknown>
})
test('curry 1 | with explicit types', () => {
const result = mergeWith<Output>(concat, A)(B)
result // $ExpectType Output
})
test('curry 2 | without explicit types', () => {
const result = mergeWith(concat)(A, B)
result // $ExpectType Record<string, unknown>
})
test('curry 2 | with explicit types', () => {
const result = mergeWith<Output>(concat)(A, B)
result // $ExpectType Output
})
})
It returns the lesser value between x
and y
.
Try this R.min example in Rambda REPL
It returns the lesser value between x
and y
according to compareFn
function.
Try this R.minBy example in Rambda REPL
modify<T extends object, K extends keyof T, P>(
prop: K,
fn: (a: T[K]) => P,
obj: T,
): Omit<T, K> & Record<K, P>
Try this R.modify example in Rambda REPL
modify<T extends object, K extends keyof T, P>(
prop: K,
fn: (a: T[K]) => P,
obj: T,
): Omit<T, K> & Record<K, P>;
modify<K extends string, A, P>(
prop: K,
fn: (a: A) => P,
): <T extends Record<K, A>>(target: T) => Omit<T, K> & Record<K, P>;
import { isArray } from './_internals/isArray.js'
import { isIterable } from './_internals/isIterable.js'
import { curry } from './curry.js'
import { updateFn } from './update.js'
function modifyFn(
property, fn, iterable
){
if (!isIterable(iterable)) return iterable
if (iterable[ property ] === undefined) return iterable
if (isArray(iterable)){
return updateFn(
property, fn(iterable[ property ]), iterable
)
}
return {
...iterable,
[ property ] : fn(iterable[ property ]),
}
}
export const modify = curry(modifyFn)
import { modify as modifyRamda } from 'ramda'
import { compareCombinations, FALSY_VALUES } from './_internals/testUtils.js'
import { add } from './add.js'
import { compose } from './compose.js'
import { modify } from './modify.js'
const person = {
name : 'foo',
age : 20,
}
test('happy', () => {
expect(modify(
'age', x => x + 1, person
)).toEqual({
name : 'foo',
age : 21,
})
})
test('property is missing', () => {
expect(modify(
'foo', x => x + 1, person
)).toEqual(person)
})
test('adjust if `array` at the given key with the `transformation` function', () => {
expect(modify(
1, add(1), [ 100, 1400 ]
)).toEqual([ 100, 1401 ])
})
describe('ignores transformations if the input value is not Array and Object', () => {
;[ 42, undefined, null, '' ].forEach(value => {
it(`${ value }`, () => {
expect(modify(
'a', add(1), value
)).toEqual(value)
})
})
})
const possibleProperties = [ ...FALSY_VALUES, 'foo', 0 ]
const possibleTransformers = [
...FALSY_VALUES,
add(1),
add('foo'),
compose,
String,
]
const possibleObjects = [
...FALSY_VALUES,
{},
[ 1, 2, 3 ],
{
a : 1,
foo : 2,
},
{
a : 1,
foo : [ 1 ],
},
{
a : 1,
foo : 'bar',
},
]
describe('brute force', () => {
compareCombinations({
fn : modify,
fnRamda : modifyRamda,
firstInput : possibleProperties,
secondInput : possibleTransformers,
thirdInput : possibleObjects,
callback : errorsCounters => {
expect(errorsCounters).toMatchInlineSnapshot(`
{
"ERRORS_MESSAGE_MISMATCH": 0,
"ERRORS_TYPE_MISMATCH": 0,
"RESULTS_MISMATCH": 0,
"SHOULD_NOT_THROW": 0,
"SHOULD_THROW": 0,
"TOTAL_TESTS": 630,
}
`)
},
})
})
import {modify, add} from 'rambda'
const person = {name: 'James', age: 20}
describe('R.modify', () => {
it('happy', () => {
const {age} = modify('age', add(1), person)
const {age: ageAsString} = modify('age', String, person)
age // $ExpectType number
ageAsString // $ExpectType string
})
it('curried', () => {
const {age} = modify('age', add(1))(person)
age // $ExpectType number
})
})
modifyPath<T extends Record<string, unknown>>(path: Path, fn: (x: any) => unknown, object: Record<string, unknown>): T
It changes a property of object on the base of provided path and transformer function.
Try this R.modifyPath example in Rambda REPL
modifyPath<T extends Record<string, unknown>>(path: Path, fn: (x: any) => unknown, object: Record<string, unknown>): T;
modifyPath<T extends Record<string, unknown>>(path: Path, fn: (x: any) => unknown): (object: Record<string, unknown>) => T;
modifyPath<T extends Record<string, unknown>>(path: Path): (fn: (x: any) => unknown) => (object: Record<string, unknown>) => T;
import { createPath } from './_internals/createPath.js'
import { isArray } from './_internals/isArray.js'
import { assoc } from './assoc.js'
import { curry } from './curry.js'
import { path as pathModule } from './path.js'
export function modifyPathFn(
pathInput, fn, object
){
const path = createPath(pathInput)
if (path.length === 1){
return {
...object,
[ path[ 0 ] ] : fn(object[ path[ 0 ] ]),
}
}
if (pathModule(path, object) === undefined) return object
const val = modifyPath(
Array.prototype.slice.call(path, 1),
fn,
object[ path[ 0 ] ]
)
if (val === object[ path[ 0 ] ]){
return object
}
return assoc(
path[ 0 ], val, object
)
}
export const modifyPath = curry(modifyPathFn)
import { modifyPath } from './modifyPath.js'
test('happy', () => {
const result = modifyPath(
'a.b.c', x => x + 1, { a : { b : { c : 1 } } }
)
expect(result).toEqual({ a : { b : { c : 2 } } })
})
test('with array', () => {
const input = { foo : [ { bar : '123' } ] }
const result = modifyPath(
'foo.0.bar', x => x + 'foo', input
)
expect(result).toEqual({ foo : { 0 : { bar : '123foo' } } })
})
import {modifyPath} from 'rambda'
const obj = {a: {b: {c: 1}}}
describe('R.modifyPath', () => {
it('happy', () => {
const result = modifyPath('a.b.c', (x: number) => x + 1, obj)
result // $ExpectType Record<string, unknown>
})
it('explicit return type', () => {
interface Foo extends Record<string, unknown> {
a: 1,
}
const result = modifyPath<Foo>('a.b.c', (x: number) => x + 1, obj)
result // $ExpectType Foo
})
})
Curried version of x%y
.
Try this R.modulo example in Rambda REPL
It returns a copy of list
with exchanged fromIndex
and toIndex
elements.
Try this R.move example in Rambda REPL
Curried version of x*y
.
Try this R.multiply example in Rambda REPL
Try this R.negate example in Rambda REPL
none<T>(predicate: (x: T) => boolean, list: T[]): boolean
It returns true
, if all members of array list
returns false
, when applied as argument to predicate
function.
Try this R.none example in Rambda REPL
none<T>(predicate: (x: T) => boolean, list: T[]): boolean;
none<T>(predicate: (x: T) => boolean): (list: T[]) => boolean;
export function none(predicate, list){
if (arguments.length === 1) return _list => none(predicate, _list)
for (let i = 0; i < list.length; i++){
if (predicate(list[ i ])) return false
}
return true
}
import { none } from './none.js'
const isEven = n => n % 2 === 0
test('when true', () => {
expect(none(isEven, [ 1, 3, 5, 7 ])).toBeTrue()
})
test('when false curried', () => {
expect(none(input => input > 1, [ 1, 2, 3 ])).toBeFalse()
})
import {none} from 'rambda'
describe('R.none', () => {
it('happy', () => {
const result = none(
x => {
x // $ExpectType number
return x > 0
},
[1, 2, 3]
)
result // $ExpectType boolean
})
it('curried needs a type', () => {
const result = none<number>(x => {
x // $ExpectType number
return x > 0
})([1, 2, 3])
result // $ExpectType boolean
})
})
not(input: any): boolean
It returns a boolean negated version of input
.
Try this R.not example in Rambda REPL
not(input: any): boolean;
export function not(input){
return !input
}
import { not } from './not.js'
test('not', () => {
expect(not(false)).toBeTrue()
expect(not(true)).toBeFalse()
expect(not(0)).toBeTrue()
expect(not(1)).toBeFalse()
})
import {not} from 'rambda'
describe('R.not', () => {
it('happy', () => {
const result = not(4)
result // $ExpectType boolean
})
})
nth(index: number, input: string): string
Curried version of input[index]
.
Try this R.nth example in Rambda REPL
nth(index: number, input: string): string;
nth<T>(index: number, input: T[]): T | undefined;
nth(n: number): {
<T>(input: T[]): T | undefined;
(input: string): string;
};
export function nth(index, input){
if (arguments.length === 1) return _input => nth(index, _input)
const idx = index < 0 ? input.length + index : index
return Object.prototype.toString.call(input) === '[object String]' ?
input.charAt(idx) :
input[ idx ]
}
import { nth } from './nth.js'
test('happy', () => {
expect(nth(2, [ 1, 2, 3, 4 ])).toBe(3)
})
test('with curry', () => {
expect(nth(2)([ 1, 2, 3, 4 ])).toBe(3)
})
test('with string and correct index', () => {
expect(nth(2)('foo')).toBe('o')
})
test('with string and invalid index', () => {
expect(nth(20)('foo')).toBe('')
})
test('with negative index', () => {
expect(nth(-3)([ 1, 2, 3, 4 ])).toBe(2)
})
import {nth} from 'rambda'
const list = [1, 2, 3]
describe('R.nth', () => {
it('happy', () => {
const result = nth(4, list)
result // $ExpectType number | undefined
})
it('curried', () => {
const result = nth(1)(list)
result // $ExpectType number | undefined
})
})
describe('R.nth - string', () => {
const str = 'abc'
it('happy', () => {
const result = nth(4, str)
result // $ExpectType string
})
it('curried', () => {
const result = nth(1)(str)
result // $ExpectType string
})
})
It creates an object with a single key-value pair.
Try this R.objOf example in Rambda REPL
of<T>(x: T): T[]
Try this R.of example in Rambda REPL
of<T>(x: T): T[];
export function of(value){
return [ value ]
}
import { of } from './of.js'
test('happy', () => {
expect(of(3)).toEqual([ 3 ])
expect(of(null)).toEqual([ null ])
})
import {of} from 'rambda'
const list = [1, 2, 3]
describe('R.of', () => {
it('happy', () => {
const result = of(4)
result // $ExpectType number[]
})
it('curried', () => {
const result = of(list)
result // $ExpectType number[][]
})
})
omit<T, K extends string>(propsToOmit: K[], obj: T): Omit<T, K>
It returns a partial copy of an obj
without propsToOmit
properties.
Try this R.omit example in Rambda REPL
omit<T, K extends string>(propsToOmit: K[], obj: T): Omit<T, K>;
omit<K extends string>(propsToOmit: K[]): <T>(obj: T) => Omit<T, K>;
omit<T, U>(propsToOmit: string, obj: T): U;
omit<T, U>(propsToOmit: string): (obj: T) => U;
omit<T>(propsToOmit: string, obj: object): T;
omit<T>(propsToOmit: string): (obj: object) => T;
import { createPath } from './_internals/createPath.js'
import { includes } from './_internals/includes.js'
export function omit(propsToOmit, obj){
if (arguments.length === 1) return _obj => omit(propsToOmit, _obj)
if (obj === null || obj === undefined)
return undefined
const propsToOmitValue = createPath(propsToOmit, ',')
const willReturn = {}
for (const key in obj)
if (!includes(key, propsToOmitValue))
willReturn[ key ] = obj[ key ]
return willReturn
}
import { omit } from './omit.js'
test('with string as condition', () => {
const obj = {
a : 1,
b : 2,
c : 3,
}
const result = omit('a,c', obj)
const resultCurry = omit('a,c')(obj)
const expectedResult = { b : 2 }
expect(result).toEqual(expectedResult)
expect(resultCurry).toEqual(expectedResult)
})
test.only('with number as property to omit', () => {
const obj = {
1 : 1,
b : 2,
}
const result = omit([ 1 ], obj)
expect(result).toEqual({ b : 2 })
})
test('with null', () => {
expect(omit('a,b', null)).toBeUndefined()
})
test('happy', () => {
expect(omit([ 'a', 'c' ])({
a : 'foo',
b : 'bar',
c : 'baz',
})).toEqual({ b : 'bar' })
})
import {omit} from 'rambda'
describe('R.omit with array as props input', () => {
it('allow Typescript to infer object type', () => {
const input = {a: 'foo', b: 2, c: 3, d: 4}
const result = omit(['b,c'], input)
result.a // $ExpectType string
result.d // $ExpectType number
const curriedResult = omit(['a,c'], input)
curriedResult.a // $ExpectType string
curriedResult.d // $ExpectType number
})
it('declare type of input object', () => {
interface Input {
a: string,
b: number,
c: number,
d: number,
}
const input: Input = {a: 'foo', b: 2, c: 3, d: 4}
const result = omit(['b,c'], input)
result // $ExpectType Omit<Input, "b,c">
result.a // $ExpectType string
result.d // $ExpectType number
const curriedResult = omit(['a,c'], input)
curriedResult.a // $ExpectType string
curriedResult.d // $ExpectType number
})
})
describe('R.omit with string as props input', () => {
interface Output {
b: number,
d: number,
}
it('explicitly declare output', () => {
const result = omit<Output>('a,c', {a: 1, b: 2, c: 3, d: 4})
result // $ExpectType Output
result.b // $ExpectType number
const curriedResult = omit<Output>('a,c')({a: 1, b: 2, c: 3, d: 4})
curriedResult.b // $ExpectType number
})
it('explicitly declare input and output', () => {
interface Input {
a: number,
b: number,
c: number,
d: number,
}
const result = omit<Input, Output>('a,c', {a: 1, b: 2, c: 3, d: 4})
result // $ExpectType Output
result.b // $ExpectType number
const curriedResult = omit<Input, Output>('a,c')({
a: 1,
b: 2,
c: 3,
d: 4,
})
curriedResult.b // $ExpectType number
})
it('without passing type', () => {
const result = omit('a,c', {a: 1, b: 2, c: 3, d: 4})
result // $ExpectType unknown
})
})
It passes the two inputs through unaryFn
and then the results are passed as inputs the the binaryFn
to receive the final result(binaryFn(unaryFn(FIRST_INPUT), unaryFn(SECOND_INPUT))
).
This method is also known as P combinator.
Try this R.on example in Rambda REPL
once<T extends AnyFunction>(func: T): T
It returns a function, which invokes only once fn
function.
Try this R.once example in Rambda REPL
once<T extends AnyFunction>(func: T): T;
import { curry } from './curry.js'
function onceFn(fn, context){
let result
return function (){
if (fn){
result = fn.apply(context || this, arguments)
fn = null
}
return result
}
}
export function once(fn, context){
if (arguments.length === 1){
const wrap = onceFn(fn, context)
return curry(wrap)
}
return onceFn(fn, context)
}
import { once } from './once.js'
test('with counter', () => {
let counter = 0
const runOnce = once(x => {
counter++
return x + 2
})
expect(runOnce(1)).toBe(3)
runOnce(1)
runOnce(1)
runOnce(1)
expect(counter).toBe(1)
})
test('happy path', () => {
const addOneOnce = once((
a, b, c
) => a + b + c, 1)
expect(addOneOnce(
10, 20, 30
)).toBe(60)
expect(addOneOnce(40)).toBe(60)
})
import {once} from 'rambda'
describe('R.once', () => {
it('happy', () => {
const runOnce = once((x: number) => {
return x + 2
})
const result = runOnce(1)
result // $ExpectType number
})
})
Logical OR
Try this R.or example in Rambda REPL
over<S, A>(lens: Lens<S, A>): {
(fn: (a: A) => A): (value: S) => S
It returns a copied Object or Array with modified value received by applying function fn
to lens
focus.
Try this R.over example in Rambda REPL
over<S, A>(lens: Lens<S, A>): {
(fn: (a: A) => A): (value: S) => S;
(fn: (a: A) => A, value: S): S;
};
over<S, A>(lens: Lens<S, A>, fn: (a: A) => A): (value: S) => S;
over<S, A>(lens: Lens<S, A>, fn: (a: A) => A, value: S): S;
import { curry } from './curry.js'
const Identity = x => ({
x,
map : fn => Identity(fn(x)),
})
function overFn(
lens, fn, object
){
return lens(x => Identity(fn(x)))(object).x
}
export const over = curry(overFn)
import { assoc } from './assoc.js'
import { lens } from './lens.js'
import { lensIndex } from './lensIndex.js'
import { lensPath } from './lensPath.js'
import { over } from './over.js'
import { prop } from './prop.js'
import { toUpper } from './toUpper.js'
const testObject = {
foo : 'bar',
baz : {
a : 'x',
b : 'y',
},
}
test('assoc lens', () => {
const assocLens = lens(prop('foo'), assoc('foo'))
const result = over(
assocLens, toUpper, testObject
)
const expected = {
...testObject,
foo : 'BAR',
}
expect(result).toEqual(expected)
})
test('path lens', () => {
const pathLens = lensPath('baz.a')
const result = over(
pathLens, toUpper, testObject
)
const expected = {
...testObject,
baz : {
a : 'X',
b : 'y',
},
}
expect(result).toEqual(expected)
})
test('index lens', () => {
const indexLens = lensIndex(0)
const result = over(indexLens, toUpper)([ 'foo', 'bar' ])
expect(result).toEqual([ 'FOO', 'bar' ])
})
partial<V0, V1, T>(fn: (x0: V0, x1: V1) => T, args: [V0]): (x1: V1) => T
It is very similar to R.curry
, but you can pass initial arguments when you create the curried function.
R.partial
will keep returning a function until all the arguments that the function fn
expects are passed.
The name comes from the fact that you partially inject the inputs.
Try this R.partial example in Rambda REPL
partial<V0, V1, T>(fn: (x0: V0, x1: V1) => T, args: [V0]): (x1: V1) => T;
partial<V0, V1, V2, T>(fn: (x0: V0, x1: V1, x2: V2) => T, args: [V0, V1]): (x2: V2) => T;
partial<V0, V1, V2, T>(fn: (x0: V0, x1: V1, x2: V2) => T, args: [V0]): (x1: V1, x2: V2) => T;
partial<V0, V1, V2, V3, T>(
fn: (x0: V0, x1: V1, x2: V2, x3: V3) => T,
args: [V0, V1, V2],
): (x2: V3) => T;
partial<V0, V1, V2, V3, T>(
fn: (x0: V0, x1: V1, x2: V2, x3: V3) => T,
args: [V0, V1],
): (x2: V2, x3: V3) => T;
partial<V0, V1, V2, V3, T>(
fn: (x0: V0, x1: V1, x2: V2, x3: V3) => T,
args: [V0],
): (x1: V1, x2: V2, x3: V3) => T;
partial<T>(fn: (...a: any[]) => T, args: any[]): (...a: any[]) => T;
import { isArray } from './_internals/isArray.js'
export function partial(fn, ...args){
const len = fn.length
// If a single array argument is given, those are the args (a la Ramda).
// Otherwise, the variadic arguments are the args.
const argList = args.length === 1 && isArray(args[0]) ? args[0] : args
return (...rest) => {
if (argList.length + rest.length >= len){
return fn(...argList, ...rest)
}
return partial(fn, ...[ ...argList, ...rest ])
}
}
import { partial } from './partial.js'
import { type } from './type.js'
const greet = (
salutation, title, firstName, lastName
) =>
[salutation, title, firstName, lastName]
test('happy', () => {
const canPassAnyNumberOfArguments = partial(
greet, 'Hello', 'Ms.'
)
const fn = canPassAnyNumberOfArguments('foo')
const sayHello = partial(greet, [ 'Hello' ])
const sayHelloRamda = partial(sayHello, [ 'Ms.' ])
expect(type(fn)).toBe('Function')
expect(fn('bar')).toStrictEqual(['Hello', 'Ms.', 'foo', 'bar'])
expect(sayHelloRamda('foo', 'bar')).toStrictEqual(['Hello', 'Ms.', 'foo', 'bar'])
})
test('extra arguments are ignored', () => {
const canPassAnyNumberOfArguments = partial(
greet, 'Hello', 'Ms.'
)
const fn = canPassAnyNumberOfArguments('foo')
expect(type(fn)).toBe('Function')
expect(fn(
'bar', 1, 2
)).toStrictEqual(['Hello', 'Ms.', 'foo', 'bar'])
})
test('when array is input', () => {
const fooFn = (
a, b, c, d
) => ({
a,
b,
c,
d,
})
const barFn = partial(
fooFn, [ 1, 2 ], []
)
expect(barFn(1, 2)).toEqual({
a : [ 1, 2 ],
b : [],
c : 1,
d : 2,
})
})
test('ramda spec', () => {
const sayHello = partial(greet, 'Hello')
const sayHelloToMs = partial(sayHello, 'Ms.')
expect(sayHelloToMs('Jane', 'Jones')).toStrictEqual(['Hello', 'Ms.', 'Jane', 'Jones'])
})
import {partial} from 'rambda'
describe('R.partial', () => {
it('happy', () => {
function fn(
aString: string,
aNumber: number,
aBoolean: boolean,
aNull: null
) {
return {aString, aNumber, aBoolean, aNull}
}
// @ts-expect-error
partial(fn, 1)
const fn1 = partial(fn, ['a'])
partial(fn1, ['b'])
const fn2 = partial(fn1, [2])
const result = fn2(true, null)
result // $ExpectType { aString: string; aNumber: number; aBoolean: boolean; aNull: null; }
})
})
partialObject<Input, PartialInput, Output>(
fn: (input: Input) => Output,
partialInput: PartialInput,
): (input: Pick<Input, Exclude<keyof Input, keyof PartialInput>>) => Output
R.partialObject
is a curry helper designed specifically for functions accepting object as a single argument.
Initially the function knows only a part from the whole input object and then R.partialObject
helps in preparing the function for the second part, when it receives the rest of the input.
Try this R.partialObject example in Rambda REPL
partialObject<Input, PartialInput, Output>(
fn: (input: Input) => Output,
partialInput: PartialInput,
): (input: Pick<Input, Exclude<keyof Input, keyof PartialInput>>) => Output;
import { mergeDeepRight } from './mergeDeepRight.js'
export function partialObject(fn, input){
return nextInput => fn(mergeDeepRight(nextInput, input))
}
import { delay } from './delay.js'
import { partialObject } from './partialObject.js'
import { type } from './type.js'
test('with plain function', () => {
const fn = ({ a, b, c }) => a + b + c
const curried = partialObject(fn, { a : 1 })
expect(type(curried)).toBe('Function')
expect(curried({
b : 2,
c : 3,
})).toBe(6)
})
test('with function that throws an error', () => {
const fn = ({ a, b, c }) => {
throw new Error('foo')
}
const curried = partialObject(fn, { a : 1 })
expect(type(curried)).toBe('Function')
expect(() =>
curried({
b : 2,
c : 3,
})).toThrowErrorMatchingInlineSnapshot('"foo"')
})
test('with async', async () => {
const fn = async ({ a, b, c }) => {
await delay(100)
return a + b + c
}
const curried = partialObject(fn, { a : 1 })
const result = await curried({
b : 2,
c : 3,
})
expect(result).toBe(6)
})
test('async function throwing an error', async () => {
const fn = async ({ a, b, c }) => {
await delay(100)
throw new Error('foo')
}
const curried = partialObject(fn, { a : 1 })
try {
await curried({
b : 2,
c : 3,
})
expect(true).toBeFalsy()
} catch (e){
expect(e.message).toBe('foo')
}
})
import {partialObject, delay} from 'rambda'
describe('R.partialObject', () => {
it('happy', () => {
interface Input {
a: number,
b: number,
c: string,
}
const fn = ({a, b, c}: Input) => a + b + c
const curried = partialObject(fn, {a: 1})
const result = curried({
b: 2,
c: 'foo',
})
result // $ExpectType string
})
it('asynchronous', async() => {
interface Input {
a: number,
b: number,
c: string,
}
const fn = async({a, b, c}: Input) => {
await delay(100)
return a + b + c
}
const curried = partialObject(fn, {a: 1})
const result = await curried({
b: 2,
c: 'foo',
})
result // $ExpectType string
})
})
partition<T>(
predicate: Predicate<T>,
input: T[]
): [T[], T[]]
It will return array of two objects/arrays according to predicate
function. The first member holds all instances of input
that pass the predicate
function, while the second member - those who doesn't.
Try this R.partition example in Rambda REPL
partition<T>(
predicate: Predicate<T>,
input: T[]
): [T[], T[]];
partition<T>(
predicate: Predicate<T>
): (input: T[]) => [T[], T[]];
partition<T>(
predicate: (x: T, prop?: string) => boolean,
input: { [key: string]: T}
): [{ [key: string]: T}, { [key: string]: T}];
partition<T>(
predicate: (x: T, prop?: string) => boolean
): (input: { [key: string]: T}) => [{ [key: string]: T}, { [key: string]: T}];
import { isArray } from './_internals/isArray.js'
export function partitionObject(predicate, iterable){
const yes = {}
const no = {}
Object.entries(iterable).forEach(([ prop, value ]) => {
if (predicate(value, prop)){
yes[ prop ] = value
} else {
no[ prop ] = value
}
})
return [ yes, no ]
}
export function partitionArray(
predicate, list, indexed = false
){
const yes = []
const no = []
let counter = -1
while (counter++ < list.length - 1){
if (
indexed ? predicate(list[ counter ], counter) : predicate(list[ counter ])
){
yes.push(list[ counter ])
} else {
no.push(list[ counter ])
}
}
return [ yes, no ]
}
export function partition(predicate, iterable){
if (arguments.length === 1){
return listHolder => partition(predicate, listHolder)
}
if (!isArray(iterable)) return partitionObject(predicate, iterable)
return partitionArray(predicate, iterable)
}
import { partition } from './partition.js'
test('with array', () => {
const predicate = x => x > 2
const list = [ 1, 2, 3, 4 ]
const result = partition(predicate, list)
const expectedResult = [
[ 3, 4 ],
[ 1, 2 ],
]
expect(result).toEqual(expectedResult)
})
test('with object', () => {
const predicate = (value, prop) => {
expect(typeof prop).toBe('string')
return value > 2
}
const hash = {
a : 1,
b : 2,
c : 3,
d : 4,
}
const result = partition(predicate)(hash)
const expectedResult = [
{
c : 3,
d : 4,
},
{
a : 1,
b : 2,
},
]
expect(result).toEqual(expectedResult)
})
test('readme example', () => {
const list = [ 1, 2, 3 ]
const obj = {
a : 1,
b : 2,
c : 3,
}
const predicate = x => x > 2
const result = [ partition(predicate, list), partition(predicate, obj) ]
const expected = [
[ [ 3 ], [ 1, 2 ] ],
[
{ c : 3 },
{
a : 1,
b : 2,
},
],
]
expect(result).toEqual(expected)
})
import {partition} from 'rambda'
describe('R.partition', () => {
it('with array', () => {
const predicate = (x: number) => {
return x > 2
}
const list = [1, 2, 3, 4]
const result = partition(predicate, list)
const curriedResult = partition(predicate)(list)
result // $ExpectType [number[], number[]]
curriedResult // $ExpectType [number[], number[]]
})
/*
revert to old version of `dtslint` and `R.partition` typing
as there is diff between VSCode types(correct) and dtslint(incorrect)
it('with object', () => {
const predicate = (value: number, prop?: string) => {
return value > 2
}
const hash = {
a: 1,
b: 2,
c: 3,
d: 4,
}
const result = partition(predicate, hash)
const curriedResult = partition(predicate)(hash)
result[0] // $xExpectType { [key: string]: number; }
result[1] // $xExpectType { [key: string]: number; }
curriedResult[0] // $xExpectType { [key: string]: number; }
curriedResult[1] // $xExpectType { [key: string]: number; }
})
*/
})
path<S, K0 extends keyof S = keyof S>(path: [K0], obj: S): S[K0]
If pathToSearch
is 'a.b'
then it will return 1
if obj
is {a:{b:1}}
.
It will return undefined
, if such path is not found.
Try this R.path example in Rambda REPL
path<S, K0 extends keyof S = keyof S>(path: [K0], obj: S): S[K0];
path<S, K0 extends keyof S = keyof S, K1 extends keyof S[K0] = keyof S[K0]>(path: [K0, K1], obj: S): S[K0][K1];
path<
S,
K0 extends keyof S = keyof S,
K1 extends keyof S[K0] = keyof S[K0],
K2 extends keyof S[K0][K1] = keyof S[K0][K1]
>(path: [K0, K1, K2], obj: S): S[K0][K1][K2];
path<
S,
K0 extends keyof S = keyof S,
K1 extends keyof S[K0] = keyof S[K0],
K2 extends keyof S[K0][K1] = keyof S[K0][K1],
K3 extends keyof S[K0][K1][K2] = keyof S[K0][K1][K2],
>(path: [K0, K1, K2, K3], obj: S): S[K0][K1][K2][K3];
path<
S,
K0 extends keyof S = keyof S,
K1 extends keyof S[K0] = keyof S[K0],
K2 extends keyof S[K0][K1] = keyof S[K0][K1],
K3 extends keyof S[K0][K1][K2] = keyof S[K0][K1][K2],
K4 extends keyof S[K0][K1][K2][K3] = keyof S[K0][K1][K2][K3],
>(path: [K0, K1, K2, K3, K4], obj: S): S[K0][K1][K2][K3][K4];
path<
S,
K0 extends keyof S = keyof S,
K1 extends keyof S[K0] = keyof S[K0],
K2 extends keyof S[K0][K1] = keyof S[K0][K1],
K3 extends keyof S[K0][K1][K2] = keyof S[K0][K1][K2],
K4 extends keyof S[K0][K1][K2][K3] = keyof S[K0][K1][K2][K3],
K5 extends keyof S[K0][K1][K2][K3][K4] = keyof S[K0][K1][K2][K3][K4],
>(path: [K0, K1, K2, K3, K4, K5], obj: S): S[K0][K1][K2][K3][K4][K5];
path<T>(pathToSearch: string, obj: any): T | undefined;
path<T>(pathToSearch: string): (obj: any) => T | undefined;
path<T>(pathToSearch: RamdaPath): (obj: any) => T | undefined;
path<T>(pathToSearch: RamdaPath, obj: any): T | undefined;
import { createPath } from './_internals/createPath.js'
export function pathFn(pathInput, obj){
let willReturn = obj
let counter = 0
const pathArrValue = createPath(pathInput)
while (counter < pathArrValue.length){
if (willReturn === null || willReturn === undefined){
return undefined
}
if (willReturn[ pathArrValue[ counter ] ] === null) return undefined
willReturn = willReturn[ pathArrValue[ counter ] ]
counter++
}
return willReturn
}
export function path(pathInput, obj){
if (arguments.length === 1) return _obj => path(pathInput, _obj)
if (obj === null || obj === undefined){
return undefined
}
return pathFn(pathInput, obj)
}
import { path } from './path.js'
test('with array inside object', () => {
const obj = { a : { b : [ 1, { c : 1 } ] } }
expect(path('a.b.1.c', obj)).toBe(1)
})
test('works with undefined', () => {
const obj = { a : { b : { c : 1 } } }
expect(path('a.b.c.d.f', obj)).toBeUndefined()
expect(path('foo.babaz', undefined)).toBeUndefined()
expect(path('foo.babaz')(undefined)).toBeUndefined()
})
test('works with string instead of array', () => {
expect(path('foo.bar.baz')({ foo : { bar : { baz : 'yes' } } })).toBe('yes')
})
test('path', () => {
expect(path([ 'foo', 'bar', 'baz' ])({ foo : { bar : { baz : 'yes' } } })).toBe('yes')
expect(path([ 'foo', 'bar', 'baz' ])(null)).toBeUndefined()
expect(path([ 'foo', 'bar', 'baz' ])({ foo : { bar : 'baz' } })).toBeUndefined()
})
test('null is not a valid path', () => {
expect(path('audio_tracks', {
a : 1,
audio_tracks : null,
})).toBeUndefined()
})
import {path} from 'rambda'
const input = {a: {b: {c: true}}}
describe('R.path with string as path', () => {
it('without specified output type', () => {
// $ExpectType unknown
path('a.b.c', input)
// $ExpectType unknown
path('a.b.c')(input)
})
it('with specified output type', () => {
// $ExpectType boolean | undefined
path<boolean>('a.b.c', input)
// $ExpectType boolean | undefined
path<boolean>('a.b.c')(input)
})
})
describe('R.path with list as path', () => {
it('with array as path', () => {
// $ExpectType boolean
path(['a', 'b', 'c'], input)
// $ExpectType unknown
path(['a', 'b', 'c'])(input)
})
test('shallow property', () => {
// $ExpectType number
path(['a'], {a: 1})
// $ExpectType unknown
path(['b'], {a: 1})
})
test('deep property', () => {
const testObject = {a: {b: {c: {d: {e: {f: 1}}}}}}
const result = path(['a', 'b', 'c', 'd', 'e', 'f'], testObject)
// $ExpectType number
result
const curriedResult = path(['a', 'b', 'c', 'd', 'e', 'f'])(testObject)
// $ExpectType unknown
curriedResult
})
test('issue #668 - path is not correct', () => {
const object = {
is: {
a: 'path',
},
}
const result = path(['is', 'not', 'a'], object)
// $ExpectType unknown
result
const curriedResult = path(['is', 'not', 'a'])(object)
// $ExpectType unknown
curriedResult
})
})
pathEq(pathToSearch: Path, target: any, input: any): boolean
It returns true
if pathToSearch
of input
object is equal to target
value.
pathToSearch
is passed to R.path
, which means that it can be either a string or an array. Also equality between target
and the found value is determined by R.equals
.
Try this R.pathEq example in Rambda REPL
pathEq(pathToSearch: Path, target: any, input: any): boolean;
pathEq(pathToSearch: Path, target: any): (input: any) => boolean;
pathEq(pathToSearch: Path): (target: any) => (input: any) => boolean;
import { curry } from './curry.js'
import { equals } from './equals.js'
import { path } from './path.js'
function pathEqFn(
pathToSearch, target, input
){
return equals(path(pathToSearch, input), target)
}
export const pathEq = curry(pathEqFn)
import { pathEq } from './pathEq.js'
test('when true', () => {
const path = 'a.b'
const obj = { a : { b : { c : 1 } } }
const target = { c : 1 }
expect(pathEq(
path, target, obj
)).toBeTrue()
})
test('when false', () => {
const path = 'a.b'
const obj = { a : { b : 1 } }
const target = 2
expect(pathEq(path, target)(obj)).toBeFalse()
})
test('when wrong path', () => {
const path = 'foo.bar'
const obj = { a : { b : 1 } }
const target = 2
expect(pathEq(
path, target, obj
)).toBeFalse()
})
import {pathEq} from 'rambda'
describe('R.pathEq', () => {
it('with string path', () => {
const pathToSearch = 'a.b.c'
const input = {a: {b: {c: 1}}}
const target = {c: 1}
const result = pathEq(pathToSearch, input, target)
const curriedResult = pathEq(pathToSearch, input, target)
result // $ExpectType boolean
curriedResult // $ExpectType boolean
})
it('with array path', () => {
const pathToSearch = ['a', 'b', 'c']
const input = {a: {b: {c: 1}}}
const target = {c: 1}
const result = pathEq(pathToSearch, input, target)
const curriedResult = pathEq(pathToSearch, input, target)
result // $ExpectType boolean
curriedResult // $ExpectType boolean
})
})
describe('with ramda specs', () => {
const testPath = ['x', 0, 'y']
const testObj = {
x: [
{y: 2, z: 3},
{y: 4, z: 5},
],
}
const result1 = pathEq(testPath, 2, testObj)
const result2 = pathEq(testPath, 2)(testObj)
const result3 = pathEq(testPath)(2)(testObj)
result1 // $ExpectType boolean
result2 // $ExpectType boolean
result3 // $ExpectType boolean
})
pathOr<T>(defaultValue: T, pathToSearch: Path, obj: any): T
It reads obj
input and returns either R.path(pathToSearch, Record<string, unknown>)
result or defaultValue
input.
Try this R.pathOr example in Rambda REPL
pathOr<T>(defaultValue: T, pathToSearch: Path, obj: any): T;
pathOr<T>(defaultValue: T, pathToSearch: Path): (obj: any) => T;
pathOr<T>(defaultValue: T): (pathToSearch: Path) => (obj: any) => T;
import { curry } from './curry.js'
import { defaultTo } from './defaultTo.js'
import { path } from './path.js'
function pathOrFn(
defaultValue, pathInput, obj
){
return defaultTo(defaultValue, path(pathInput, obj))
}
export const pathOr = curry(pathOrFn)
import { pathOr } from './pathOr.js'
test('with undefined', () => {
const result = pathOr(
'foo', 'x.y', { x : { y : 1 } }
)
expect(result).toBe(1)
})
test('with null', () => {
const result = pathOr(
'foo', 'x.y', null
)
expect(result).toBe('foo')
})
test('with NaN', () => {
const result = pathOr(
'foo', 'x.y', NaN
)
expect(result).toBe('foo')
})
test('curry case (x)(y)(z)', () => {
const result = pathOr('foo')('x.y.z')({ x : { y : { a : 1 } } })
expect(result).toBe('foo')
})
test('curry case (x)(y,z)', () => {
const result = pathOr('foo', 'x.y.z')({ x : { y : { a : 1 } } })
expect(result).toBe('foo')
})
test('curry case (x,y)(z)', () => {
const result = pathOr('foo')('x.y.z', { x : { y : { a : 1 } } })
expect(result).toBe('foo')
})
import {pathOr} from 'rambda'
describe('R.pathOr', () => {
it('with string path', () => {
const x = pathOr<string>('foo', 'x.y', {x: {y: 'bar'}})
x // $ExpectType string
})
it('with array path', () => {
const x = pathOr<string>('foo', ['x', 'y'], {x: {y: 'bar'}})
x // $ExpectType string
})
it('without passing type looks bad', () => {
const x = pathOr('foo', 'x.y', {x: {y: 'bar'}})
x // $ExpectType "foo"
})
it('curried', () => {
const x = pathOr<string>('foo', 'x.y')({x: {y: 'bar'}})
x // $ExpectType string
})
})
paths<Input, T>(pathsToSearch: Path[], obj: Input): (T | undefined)[]
It loops over members of pathsToSearch
as singlePath
and returns the array produced by R.path(singlePath, Record<string, unknown>)
.
Because it calls R.path
, then singlePath
can be either string or a list.
Try this R.paths example in Rambda REPL
paths<Input, T>(pathsToSearch: Path[], obj: Input): (T | undefined)[];
paths<Input, T>(pathsToSearch: Path[]): (obj: Input) => (T | undefined)[];
paths<T>(pathsToSearch: Path[], obj: any): (T | undefined)[];
paths<T>(pathsToSearch: Path[]): (obj: any) => (T | undefined)[];
import { path } from './path.js'
export function paths(pathsToSearch, obj){
if (arguments.length === 1){
return _obj => paths(pathsToSearch, _obj)
}
return pathsToSearch.map(singlePath => path(singlePath, obj))
}
import { paths } from './paths.js'
const obj = {
a : {
b : {
c : 1,
d : 2,
},
},
p : [ { q : 3 } ],
x : {
y : 'FOO',
z : [ [ {} ] ],
},
}
test('with string path + curry', () => {
const pathsInput = [ 'a.b.d', 'p.q' ]
const expected = [ 2, undefined ]
const result = paths(pathsInput, obj)
const curriedResult = paths(pathsInput)(obj)
expect(result).toEqual(expected)
expect(curriedResult).toEqual(expected)
})
test('with array path', () => {
const result = paths([
[ 'a', 'b', 'c' ],
[ 'x', 'y' ],
],
obj)
expect(result).toEqual([ 1, 'FOO' ])
})
test('takes a paths that contains indices into arrays', () => {
expect(paths([
[ 'p', 0, 'q' ],
[ 'x', 'z', 0, 0 ],
],
obj)).toEqual([ 3, {} ])
expect(paths([
[ 'p', 0, 'q' ],
[ 'x', 'z', 2, 1 ],
],
obj)).toEqual([ 3, undefined ])
})
test('gets a deep property\'s value from objects', () => {
expect(paths([ [ 'a', 'b' ] ], obj)).toEqual([ obj.a.b ])
expect(paths([ [ 'p', 0 ] ], obj)).toEqual([ obj.p[ 0 ] ])
})
test('returns undefined for items not found', () => {
expect(paths([ [ 'a', 'x', 'y' ] ], obj)).toEqual([ undefined ])
expect(paths([ [ 'p', 2 ] ], obj)).toEqual([ undefined ])
})
import {paths} from 'rambda'
interface Input {
a: number,
b: number,
c: number,
}
const input: Input = {a: 1, b: 2, c: 3}
describe('R.paths', () => {
it('with dot notation', () => {
const result = paths<number>(['a.b.c', 'foo.bar'], input)
result // $ExpectType (number | undefined)[]
})
it('without type', () => {
const result = paths(['a.b.c', 'foo.bar'], input)
result // $ExpectType unknown[]
})
it('with array as path', () => {
const result = paths<number>([['a', 'b', 'c'], ['foo.bar']], input)
result // $ExpectType (number | undefined)[]
})
it('curried', () => {
const result = paths<number>([['a', 'b', 'c'], ['foo.bar']])(input)
result // $ExpectType (number | undefined)[]
})
})
pick<T, K extends string | number | symbol>(propsToPick: K[], input: T): Pick<T, Exclude<keyof T, Exclude<keyof T, K>>>
It returns a partial copy of an input
containing only propsToPick
properties.
input
can be either an object or an array.
String annotation of propsToPick
is one of the differences between Rambda
and Ramda
.
Try this R.pick example in Rambda REPL
pick<T, K extends string | number | symbol>(propsToPick: K[], input: T): Pick<T, Exclude<keyof T, Exclude<keyof T, K>>>;
pick<K extends string | number | symbol>(propsToPick: K[]): <T>(input: T) => Pick<T, Exclude<keyof T, Exclude<keyof T, K>>>;
pick<T, U>(propsToPick: string, input: T): U;
pick<T, U>(propsToPick: string): (input: T) => U;
pick<T>(propsToPick: string, input: object): T;
pick<T>(propsToPick: string): (input: object) => T;
import { createPath } from './_internals/createPath.js'
export function pick(propsToPick, input){
if (arguments.length === 1) return _input => pick(propsToPick, _input)
if (input === null || input === undefined){
return undefined
}
const keys = createPath(propsToPick, ',')
const willReturn = {}
let counter = 0
while (counter < keys.length){
if (keys[ counter ] in input){
willReturn[ keys[ counter ] ] = input[ keys[ counter ] ]
}
counter++
}
return willReturn
}
import { pick } from './pick.js'
const obj = {
a : 1,
b : 2,
c : 3,
}
test('props to pick is a string', () => {
const result = pick('a,c', obj)
const resultCurry = pick('a,c')(obj)
const expectedResult = {
a : 1,
c : 3,
}
expect(result).toEqual(expectedResult)
expect(resultCurry).toEqual(expectedResult)
})
test('when prop is missing', () => {
const result = pick('a,d,f', obj)
expect(result).toEqual({ a : 1 })
})
test('with list indexes as props', () => {
const list = [ 1, 2, 3 ]
const expected = {
0 : 1,
2 : 3,
}
expect(pick([ 0, 2, 3 ], list)).toEqual(expected)
expect(pick('0,2,3', list)).toEqual(expected)
})
test('props to pick is an array', () => {
expect(pick([ 'a', 'c' ])({
a : 'foo',
b : 'bar',
c : 'baz',
})).toEqual({
a : 'foo',
c : 'baz',
})
expect(pick([ 'a', 'd', 'e', 'f' ])({
a : 'foo',
b : 'bar',
c : 'baz',
})).toEqual({ a : 'foo' })
expect(pick('a,d,e,f')(null)).toBeUndefined()
})
test('works with list as input and number as props - props to pick is an array', () => {
const result = pick([ 1, 2 ], [ 'a', 'b', 'c', 'd' ])
expect(result).toEqual({
1 : 'b',
2 : 'c',
})
})
test('works with list as input and number as props - props to pick is a string', () => {
const result = pick('1,2', [ 'a', 'b', 'c', 'd' ])
expect(result).toEqual({
1 : 'b',
2 : 'c',
})
})
test('with symbol', () => {
const symbolProp = Symbol('s')
expect(pick([ symbolProp ], { [ symbolProp ] : 'a' })).toMatchInlineSnapshot(`
{
Symbol(s): "a",
}
`)
})
import {pick} from 'rambda'
const input = {a: 'foo', b: 2, c: 3, d: 4}
describe('R.pick with array as props input', () => {
it('without passing type', () => {
const result = pick(['a', 'c'], input)
result.a // $ExpectType string
result.c // $ExpectType number
})
})
describe('R.pick with string as props input', () => {
interface Input {
a: string,
b: number,
c: number,
d: number,
}
interface Output {
a: string,
c: number,
}
it('explicitly declare output', () => {
const result = pick<Output>('a,c', input)
result // $ExpectType Output
result.a // $ExpectType string
result.c // $ExpectType number
const curriedResult = pick<Output>('a,c')(input)
curriedResult.a // $ExpectType string
})
it('explicitly declare input and output', () => {
const result = pick<Input, Output>('a,c', input)
result // $ExpectType Output
result.a // $ExpectType string
const curriedResult = pick<Input, Output>('a,c')(input)
curriedResult.a // $ExpectType string
})
it('without passing type', () => {
const result = pick('a,c', input)
result // $ExpectType unknown
})
})
pickAll<T, K extends keyof T>(propsToPicks: K[], input: T): Pick<T, K>
Same as R.pick
but it won't skip the missing props, i.e. it will assign them to undefined
.
Try this R.pickAll example in Rambda REPL
pickAll<T, K extends keyof T>(propsToPicks: K[], input: T): Pick<T, K>;
pickAll<T, U>(propsToPicks: string[], input: T): U;
pickAll(propsToPicks: string[]): <T, U>(input: T) => U;
pickAll<T, U>(propsToPick: string, input: T): U;
pickAll<T, U>(propsToPick: string): (input: T) => U;
import { createPath } from './_internals/createPath.js'
export function pickAll(propsToPick, obj){
if (arguments.length === 1) return _obj => pickAll(propsToPick, _obj)
if (obj === null || obj === undefined){
return undefined
}
const keysValue = createPath(propsToPick, ',')
const willReturn = {}
let counter = 0
while (counter < keysValue.length){
if (keysValue[ counter ] in obj){
willReturn[ keysValue[ counter ] ] = obj[ keysValue[ counter ] ]
} else {
willReturn[ keysValue[ counter ] ] = undefined
}
counter++
}
return willReturn
}
import { pickAll } from './pickAll.js'
test('when input is undefined or null', () => {
expect(pickAll('a', null)).toBeUndefined()
expect(pickAll('a', undefined)).toBeUndefined()
})
test('with string as condition', () => {
const obj = {
a : 1,
b : 2,
c : 3,
}
const result = pickAll('a,c', obj)
const resultCurry = pickAll('a,c')(obj)
const expectedResult = {
a : 1,
b : undefined,
c : 3,
}
expect(result).toEqual(expectedResult)
expect(resultCurry).toEqual(expectedResult)
})
test('with array as condition', () => {
expect(pickAll([ 'a', 'b', 'c' ], {
a : 'foo',
c : 'baz',
})).toEqual({
a : 'foo',
b : undefined,
c : 'baz',
})
})
import {pickAll} from 'rambda'
interface Input {
a: string,
b: number,
c: number,
d: number,
}
interface Output {
a?: string,
c?: number,
}
const input = {a: 'foo', b: 2, c: 3, d: 4}
describe('R.pickAll with array as props input', () => {
it('without passing type', () => {
const result = pickAll(['a', 'c'], input)
result.a // $ExpectType string
result.c // $ExpectType number
})
it('without passing type + curry', () => {
const result = pickAll(['a', 'c'])(input)
result // $ExpectType unknown
})
it('explicitly passing types', () => {
const result = pickAll<Input, Output>(['a', 'c'], input)
result.a // $ExpectType string | undefined
result.c // $ExpectType number | undefined
})
})
describe('R.pickAll with string as props input', () => {
it('without passing type', () => {
const result = pickAll('a,c', input)
result // $ExpectType unknown
})
it('without passing type + curry', () => {
const result = pickAll('a,c')(input)
result // $ExpectType unknown
})
it('explicitly passing types', () => {
const result = pickAll<Input, Output>('a,c', input)
result.a // $ExpectType string | undefined
result.c // $ExpectType number | undefined
})
it('explicitly passing types + curry', () => {
const result = pickAll<Input, Output>('a,c')(input)
result.a // $ExpectType string | undefined
result.c // $ExpectType number | undefined
})
})
It performs left-to-right function composition.
Try this R.pipe example in Rambda REPL
pluck<K extends keyof T, T>(property: K, list: T[]): T[K][]
It returns list of the values of property
taken from the all objects inside list
.
Try this R.pluck example in Rambda REPL
pluck<K extends keyof T, T>(property: K, list: T[]): T[K][];
pluck<T>(property: number, list: { [k: number]: T }[]): T[];
pluck<P extends string>(property: P): <T>(list: Record<P, T>[]) => T[];
pluck(property: number): <T>(list: { [k: number]: T }[]) => T[];
import { map } from './map.js'
export function pluck(property, list){
if (arguments.length === 1) return _list => pluck(property, _list)
const willReturn = []
map(x => {
if (x[ property ] !== undefined){
willReturn.push(x[ property ])
}
}, list)
return willReturn
}
import { pluck } from './pluck.js'
test('happy', () => {
expect(pluck('a')([ { a : 1 }, { a : 2 }, { b : 1 } ])).toEqual([ 1, 2 ])
})
test('with undefined', () => {
expect(pluck(undefined)([ { a : 1 }, { a : 2 }, { b : 1 } ])).toEqual([ ])
})
test('with number', () => {
const input = [
[ 1, 2 ],
[ 3, 4 ],
]
expect(pluck(0, input)).toEqual([ 1, 3 ])
})
import {pluck} from 'rambda'
describe('R.pluck', () => {
it('with object', () => {
interface ListMember {
a: number,
b: string,
}
const input: ListMember[] = [
{a: 1, b: 'foo'},
{a: 2, b: 'bar'},
]
const resultA = pluck('a', input)
const resultB = pluck('b')(input)
resultA // $ExpectType number[]
resultB // $ExpectType string[]
})
it('with array', () => {
const input = [
[1, 2],
[3, 4],
[5, 6],
]
const result = pluck(0, input)
const resultCurry = pluck(0)(input)
result // $ExpectType number[]
resultCurry // $ExpectType number[]
})
})
prepend<T>(xToPrepend: T, iterable: T[]): T[]
It adds element x
at the beginning of list
.
Try this R.prepend example in Rambda REPL
prepend<T>(xToPrepend: T, iterable: T[]): T[];
prepend<T, U>(xToPrepend: T, iterable: IsFirstSubtypeOfSecond<T, U>[]) : U[];
prepend<T>(xToPrepend: T): <U>(iterable: IsFirstSubtypeOfSecond<T, U>[]) => U[];
prepend<T>(xToPrepend: T): (iterable: T[]) => T[];
export function prepend(x, input){
if (arguments.length === 1) return _input => prepend(x, _input)
if (typeof input === 'string') return [ x ].concat(input.split(''))
return [ x ].concat(input)
}
import { prepend } from './prepend.js'
test('happy', () => {
expect(prepend('yes', [ 'foo', 'bar', 'baz' ])).toEqual([
'yes',
'foo',
'bar',
'baz',
])
})
test('with empty list', () => {
expect(prepend('foo')([])).toEqual([ 'foo' ])
})
test('with string instead of array', () => {
expect(prepend('foo')('bar')).toEqual([ 'foo', 'b', 'a', 'r' ])
})
product(list: number[]): number
Try this R.product example in Rambda REPL
product(list: number[]): number;
import { multiply } from './multiply.js'
import { reduce } from './reduce.js'
export const product = reduce(multiply, 1)
import { product } from './product.js'
test('happy', () => {
expect(product([ 2, 3, 4 ])).toBe(24)
})
test('bad input', () => {
expect(product([ null ])).toBe(0)
expect(product([])).toBe(1)
})
import {product} from 'rambda'
describe('R.product', () => {
it('happy', () => {
const result = product([1, 2, 3])
result // $ExpectType number
})
})
prop<_, P extends keyof never, T>(p: P, value: T): Prop<T, P>
It returns the value of property propToFind
in obj
.
If there is no such property, it returns undefined
.
Try this R.prop example in Rambda REPL
prop<_, P extends keyof never, T>(p: P, value: T): Prop<T, P>;
prop<V>(p: keyof never, value: unknown): V;
prop<_, P extends keyof never>(p: P): <T>(value: T) => Prop<T, P>;
prop<V>(p: keyof never): (value: unknown) => V;
export function propFn(searchProperty, obj){
if (!obj) return undefined
return obj[ searchProperty ]
}
export function prop(searchProperty, obj){
if (arguments.length === 1) return _obj => prop(searchProperty, _obj)
return propFn(searchProperty, obj)
}
import { prop } from './prop.js'
test('prop', () => {
expect(prop('foo')({ foo : 'baz' })).toBe('baz')
expect(prop('bar')({ foo : 'baz' })).toBeUndefined()
expect(prop('bar')(null)).toBeUndefined()
})
import {prop} from 'rambda'
describe('R.prop', () => {
interface Foo {
a: number,
b: string,
c?: number,
}
const obj: Foo = {a: 1, b: 'foo'}
it('issue #553', () => {
const result = {
a: prop('a', obj),
b: prop('b', obj),
c: prop('c', obj),
}
const curriedResult = {
a: prop('a')(obj),
b: prop('b')(obj),
c: prop('c')(obj),
}
result // $ExpectType { a: number; b: string; c: number | undefined; }
curriedResult // $ExpectType { a: number; b: string; c: number | undefined; }
})
})
describe('with number as prop', () => {
const list = [1, 2, 3]
const index = 1
it('happy', () => {
const result = prop(index, list)
result // $ExpectType number
})
it('curried require explicit type', () => {
const result = prop<number>(index)(list)
result // $ExpectType number
})
})
propEq<K extends string | number>(valueToMatch: any, propToFind: K, obj: Record<K, any>): boolean
It returns true if obj
has property propToFind
and its value is equal to valueToMatch
.
Try this R.propEq example in Rambda REPL
propEq<K extends string | number>(valueToMatch: any, propToFind: K, obj: Record<K, any>): boolean;
propEq<K extends string | number>(valueToMatch: any, propToFind: K): (obj: Record<K, any>) => boolean;
propEq(valueToMatch: any): {
<K extends string | number>(propToFind: K, obj: Record<K, any>): boolean;
<K extends string | number>(propToFind: K): (obj: Record<K, any>) => boolean;
};
import { curry } from './curry.js'
import { equals } from './equals.js'
import { prop } from './prop.js'
function propEqFn(
valueToMatch, propToFind, obj
){
if (!obj) return false
return equals(valueToMatch, prop(propToFind, obj))
}
export const propEq = curry(propEqFn)
import { BAR, FOO } from './_internals/testUtils.js'
import { propEq } from './propEq.js'
test('happy', () => {
const obj = { [ FOO ] : BAR }
expect(propEq(BAR, FOO)(obj)).toBeTrue()
expect(propEq(1, FOO)(obj)).toBeFalse()
expect(propEq(1)(FOO)(obj)).toBeFalse()
expect(propEq(
1, 1, null
)).toBeFalse()
})
test('returns false if called with a null or undefined object', () => {
expect(propEq(
'name', 'Abby', null
)).toBeFalse()
expect(propEq(
'name', 'Abby', undefined
)).toBeFalse()
})
import {propEq} from 'rambda'
const property = 'foo'
const numberProperty = 1
const value = 'bar'
const obj = {[property]: value}
const objWithNumberIndex = {[numberProperty]: value}
describe('R.propEq', () => {
it('happy', () => {
const result = propEq(value, property, obj)
result // $ExpectType boolean
})
it('number is property', () => {
const result = propEq(value, 1, objWithNumberIndex)
result // $ExpectType boolean
})
it('with optional property', () => {
interface MyType {
optional?: string | number,
}
const myObject: MyType = {}
const valueToFind = '1111'
// @ts-expect-error
propEq(valueToFind, 'optional', myObject)
})
it('imported from @types/ramda', () => {
interface A {
foo: string | null,
}
const obj: A = {
foo: 'bar',
}
const value = ''
const result = propEq(value, 'foo')(obj)
result // $ExpectType boolean
// @ts-expect-error
propEq(value, 'bar')(obj)
})
})
propIs<C extends AnyFunction, K extends keyof any>(type: C, name: K, obj: any): obj is Record<K, ReturnType<C>>
It returns true
if property
of obj
is from target
type.
Try this R.propIs example in Rambda REPL
propIs<C extends AnyFunction, K extends keyof any>(type: C, name: K, obj: any): obj is Record<K, ReturnType<C>>;
propIs<C extends AnyConstructor, K extends keyof any>(type: C, name: K, obj: any): obj is Record<K, InstanceType<C>>;
propIs<C extends AnyFunction, K extends keyof any>(type: C, name: K): (obj: any) => obj is Record<K, ReturnType<C>>;
propIs<C extends AnyConstructor, K extends keyof any>(type: C, name: K): (obj: any) => obj is Record<K, InstanceType<C>>;
propIs<C extends AnyFunction>(type: C): {
<K extends keyof any>(name: K, obj: any): obj is Record<K, ReturnType<C>>;
<K extends keyof any>(name: K): (obj: any) => obj is Record<K, ReturnType<C>>;
};
import { curry } from './curry.js'
import { is } from './is.js'
function propIsFn(
targetPrototype, property, obj
){
return is(targetPrototype, obj[ property ])
}
export const propIs = curry(propIsFn)
import { propIs } from './propIs.js'
const obj = {
a : 1,
b : 'foo',
}
test('when true', () => {
expect(propIs(
Number, 'a', obj
)).toBeTrue()
expect(propIs(
String, 'b', obj
)).toBeTrue()
})
test('when false', () => {
expect(propIs(
String, 'a', obj
)).toBeFalse()
expect(propIs(
Number, 'b', obj
)).toBeFalse()
})
import {propIs} from 'rambda'
const property = 'a'
const obj = {a: 1}
describe('R.propIs', () => {
it('happy', () => {
const result = propIs(Number, property, obj)
result // $ExpectType boolean
})
it('curried', () => {
const result = propIs(Number, property)(obj)
result // $ExpectType boolean
})
})
propOr<T, P extends string>(defaultValue: T, property: P, obj: Partial<Record<P, T>> | undefined): T
It returns either defaultValue
or the value of property
in obj
.
Try this R.propOr example in Rambda REPL
propOr<T, P extends string>(defaultValue: T, property: P, obj: Partial<Record<P, T>> | undefined): T;
propOr<T, P extends string>(defaultValue: T, property: P): (obj: Partial<Record<P, T>> | undefined) => T;
propOr<T>(defaultValue: T): {
<P extends string>(property: P, obj: Partial<Record<P, T>> | undefined): T;
<P extends string>(property: P): (obj: Partial<Record<P, T>> | undefined) => T;
}
import { curry } from './curry.js'
import { defaultTo } from './defaultTo.js'
function propOrFn(
defaultValue, property, obj
){
if (!obj) return defaultValue
return defaultTo(defaultValue, obj[ property ])
}
export const propOr = curry(propOrFn)
import { propOr } from './propOr.js'
test('propOr (result)', () => {
const obj = { a : 1 }
expect(propOr(
'default', 'a', obj
)).toBe(1)
expect(propOr(
'default', 'notExist', obj
)).toBe('default')
expect(propOr(
'default', 'notExist', null
)).toBe('default')
})
test('propOr (currying)', () => {
const obj = { a : 1 }
expect(propOr('default')('a', obj)).toBe(1)
expect(propOr('default', 'a')(obj)).toBe(1)
expect(propOr('default')('notExist', obj)).toBe('default')
expect(propOr('default', 'notExist')(obj)).toBe('default')
})
import {propOr} from 'rambda'
const obj = {foo: 'bar'}
const property = 'foo'
const fallback = 'fallback'
describe('R.propOr', () => {
it('happy', () => {
const result = propOr(fallback, property, obj)
result // $ExpectType string
})
it('curry 1', () => {
const result = propOr(fallback)(property, obj)
result // $ExpectType string
})
it('curry 2', () => {
const result = propOr(fallback, property)(obj)
result // $ExpectType string
})
it('curry 3', () => {
const result = propOr(fallback)(property)(obj)
result // $ExpectType string
})
})
props<P extends string, T>(propsToPick: P[], obj: Record<P, T>): T[]
It takes list with properties propsToPick
and returns a list with property values in obj
.
Try this R.props example in Rambda REPL
props<P extends string, T>(propsToPick: P[], obj: Record<P, T>): T[];
props<P extends string>(propsToPick: P[]): <T>(obj: Record<P, T>) => T[];
props<P extends string, T>(propsToPick: P[]): (obj: Record<P, T>) => T[];
import { isArray } from './_internals/isArray.js'
import { mapArray } from './map.js'
export function props(propsToPick, obj){
if (arguments.length === 1){
return _obj => props(propsToPick, _obj)
}
if (!isArray(propsToPick)){
throw new Error('propsToPick is not a list')
}
return mapArray(prop => obj[ prop ], propsToPick)
}
import { props } from './props.js'
const obj = {
a : 1,
b : 2,
}
const propsToPick = [ 'a', 'c' ]
test('happy', () => {
const result = props(propsToPick, obj)
expect(result).toEqual([ 1, undefined ])
})
test('curried', () => {
const result = props(propsToPick)(obj)
expect(result).toEqual([ 1, undefined ])
})
test('wrong input', () => {
expect(() => props(null)(obj)).toThrowErrorMatchingInlineSnapshot('"propsToPick is not a list"')
})
import {props} from 'rambda'
const obj = {a: 1, b: 2}
describe('R.props', () => {
it('happy', () => {
const result = props(['a', 'b'], obj)
result // $ExpectType number[]
})
it('curried', () => {
const result = props(['a', 'b'])(obj)
result // $ExpectType number[]
})
})
propSatisfies<T>(predicate: Predicate<T>, property: string, obj: Record<string, T>): boolean
It returns true
if the object property satisfies a given predicate.
Try this R.propSatisfies example in Rambda REPL
propSatisfies<T>(predicate: Predicate<T>, property: string, obj: Record<string, T>): boolean;
propSatisfies<T>(predicate: Predicate<T>, property: string): (obj: Record<string, T>) => boolean;
import { curry } from './curry.js'
import { prop } from './prop.js'
function propSatisfiesFn(
predicate, property, obj
){
return predicate(prop(property, obj))
}
export const propSatisfies = curry(propSatisfiesFn)
import { propSatisfies } from './propSatisfies.js'
const obj = { a : 1 }
test('when true', () => {
expect(propSatisfies(
x => x > 0, 'a', obj
)).toBeTrue()
})
test('when false', () => {
expect(propSatisfies(x => x < 0, 'a')(obj)).toBeFalse()
})
import {propSatisfies} from 'rambda'
const obj = {a: 1}
describe('R.propSatisfies', () => {
it('happy', () => {
const result = propSatisfies(x => x > 0, 'a', obj)
result // $ExpectType boolean
})
it('curried requires explicit type', () => {
const result = propSatisfies<number>(x => x > 0, 'a')(obj)
result // $ExpectType boolean
})
})
range(startInclusive: number, endExclusive: number): number[]
It returns list of numbers between startInclusive
to endExclusive
markers.
Try this R.range example in Rambda REPL
range(startInclusive: number, endExclusive: number): number[];
range(startInclusive: number): (endExclusive: number) => number[];
export function range(start, end){
if (arguments.length === 1) return _end => range(start, _end)
if (Number.isNaN(Number(start)) || Number.isNaN(Number(end))){
throw new TypeError('Both arguments to range must be numbers')
}
if (end < start) return []
const len = end - start
const willReturn = Array(len)
for (let i = 0; i < len; i++){
willReturn[ i ] = start + i
}
return willReturn
}
import { range } from './range.js'
test('happy', () => {
expect(range(0, 10)).toEqual([ 0, 1, 2, 3, 4, 5, 6, 7, 8, 9 ])
})
test('end range is bigger than start range', () => {
expect(range(7, 3)).toEqual([])
expect(range(5, 5)).toEqual([])
})
test('with bad input', () => {
const throwMessage = 'Both arguments to range must be numbers'
expect(() => range('a', 6)).toThrowWithMessage(Error, throwMessage)
expect(() => range(6, 'z')).toThrowWithMessage(Error, throwMessage)
})
test('curry', () => {
expect(range(0)(10)).toEqual([ 0, 1, 2, 3, 4, 5, 6, 7, 8, 9 ])
})
import {range} from 'rambda'
describe('R.range', () => {
it('happy', () => {
const result = range(1, 4)
result // $ExpectType number[]
})
it('curried', () => {
const result = range(1)(4)
result // $ExpectType number[]
})
})
Try this R.reduce example in Rambda REPL
Try this R.reduceBy example in Rambda REPL
reject<T>(predicate: Predicate<T>, list: T[]): T[]
It has the opposite effect of R.filter
.
Try this R.reject example in Rambda REPL
reject<T>(predicate: Predicate<T>, list: T[]): T[];
reject<T>(predicate: Predicate<T>): (list: T[]) => T[];
reject<T>(predicate: Predicate<T>, obj: Dictionary<T>): Dictionary<T>;
reject<T, U>(predicate: Predicate<T>): (obj: Dictionary<T>) => Dictionary<T>;
import { filter } from './filter.js'
export function reject(predicate, list){
if (arguments.length === 1) return _list => reject(predicate, _list)
return filter(x => !predicate(x), list)
}
import { reject } from './reject.js'
const isOdd = n => n % 2 === 1
test('with array', () => {
expect(reject(isOdd)([ 1, 2, 3, 4 ])).toEqual([ 2, 4 ])
})
test('with object', () => {
const obj = {
a : 1,
b : 2,
c : 3,
d : 4,
}
expect(reject(isOdd, obj)).toEqual({
b : 2,
d : 4,
})
})
import {reject} from 'rambda'
describe('R.reject with array', () => {
it('happy', () => {
const result = reject(
x => {
x // $ExpectType number
return x > 1
},
[1, 2, 3]
)
result // $ExpectType number[]
})
it('curried require explicit type', () => {
const result = reject<number>(x => {
x // $ExpectType number
return x > 1
})([1, 2, 3])
result // $ExpectType number[]
})
})
describe('R.reject with objects', () => {
it('happy', () => {
const result = reject(
x => {
x // $ExpectType number
return x > 1
},
{a: 1, b: 2}
)
result // $ExpectType Dictionary<number>
})
it('curried require dummy type', () => {
const result = reject<number, any>(x => {
return x > 1
})({a: 1, b: 2})
result // $ExpectType Dictionary<number>
})
})
removeIndex<T>(index: number, list: T[]): T[]
It returns a copy of list
input with removed index
.
Try this R.removeIndex example in Rambda REPL
removeIndex<T>(index: number, list: T[]): T[];
removeIndex(index: number): <T>(list: T[]) => T[];
export function removeIndex(index, list){
if (arguments.length === 1) return _list => removeIndex(index, _list)
if (index <= 0) return list.slice(1)
if (index >= list.length - 1) return list.slice(0, list.length - 1)
return [ ...list.slice(0, index), ...list.slice(index + 1) ]
}
import { removeIndex } from './removeIndex.js'
const list = [ 1, 2, 3, 4 ]
test('first or before first index', () => {
expect(removeIndex(-2, list)).toEqual([ 2, 3, 4 ])
expect(removeIndex(-2)(list)).toEqual([ 2, 3, 4 ])
})
test('last or after last index', () => {
expect(removeIndex(4, list)).toEqual([ 1, 2, 3 ])
expect(removeIndex(10, list)).toEqual([ 1, 2, 3 ])
})
test('middle index', () => {
expect(removeIndex(1, list)).toEqual([ 1, 3, 4 ])
expect(removeIndex(2, list)).toEqual([ 1, 2, 4 ])
})
import {removeIndex} from 'rambda'
describe('R.removeIndex', () => {
it('happy', () => {
const result = removeIndex(1, [1, 2, 3])
result // $ExpectType number[]
})
it('curried', () => {
const result = removeIndex(1)([1, 2, 3])
result // $ExpectType number[]
})
})
repeat<T>(x: T): (timesToRepeat: number) => T[]
Try this R.repeat example in Rambda REPL
repeat<T>(x: T): (timesToRepeat: number) => T[];
repeat<T>(x: T, timesToRepeat: number): T[];
export function repeat(x, timesToRepeat){
if (arguments.length === 1){
return _timesToRepeat => repeat(x, _timesToRepeat)
}
return Array(timesToRepeat).fill(x)
}
import { repeat } from './repeat.js'
test('repeat', () => {
expect(repeat('')(3)).toEqual([ '', '', '' ])
expect(repeat('foo', 3)).toEqual([ 'foo', 'foo', 'foo' ])
const obj = {}
const arr = repeat(obj, 3)
expect(arr).toEqual([ {}, {}, {} ])
expect(arr[ 0 ] === arr[ 1 ]).toBeTrue()
})
import {repeat} from 'rambda'
describe('R.repeat', () => {
it('happy', () => {
const result = repeat(4, 7)
result // $ExpectType number[]
})
it('curried', () => {
const result = repeat(4)(7)
result // $ExpectType number[]
})
})
replace(strOrRegex: RegExp | string, replacer: RegExpReplacer, str: string): string
It replaces strOrRegex
found in str
with replacer
.
Try this R.replace example in Rambda REPL
replace(strOrRegex: RegExp | string, replacer: RegExpReplacer, str: string): string;
replace(strOrRegex: RegExp | string, replacer: RegExpReplacer): (str: string) => string;
replace(strOrRegex: RegExp | string): (replacer: RegExpReplacer) => (str: string) => string;
import { curry } from './curry.js'
function replaceFn(
pattern, replacer, str
){
return str.replace(pattern, replacer)
}
export const replace = curry(replaceFn)
import { replace } from './replace.js'
test('happy', () => {
expect(replace(
/\s/g, '|', 'foo bar baz'
)).toBe('foo|bar|baz')
})
test('with function as replacer input', () => {
expect(replace(
/\s/g,
(
match, offset, str
) => {
expect(match).toBe(' ')
expect([ 3, 7 ].includes(offset)).toBeTrue()
expect(str).toBe('foo bar baz')
return '|'
},
'foo bar baz'
)).toBe('foo|bar|baz')
})
import {replace} from 'rambda'
const str = 'foo bar foo'
const replacer = 'bar'
describe('R.replace', () => {
it('happy', () => {
const result = replace(/foo/g, replacer, str)
result // $ExpectType string
})
it('with string as search pattern', () => {
const result = replace('foo', replacer, str)
result // $ExpectType string
})
it('with function as replacer', () => {
const result = replace('f(o)o', (m: string, p1: string, offset: number) => {
m // $ExpectType string
p1 // $ExpectType string
offset // $ExpectType number
return p1
}, str)
result // $ExpectType string
})
})
describe('R.replace - curried', () => {
it('happy', () => {
const result = replace(/foo/g, replacer)(str)
result // $ExpectType string
})
it('with string as search pattern', () => {
const result = replace('foo', replacer)(str)
result // $ExpectType string
})
it('with function as replacer', () => {
const result = replace('f(o)o')((m: string, p1: string, offset: number) => {
m // $ExpectType string
p1 // $ExpectType string
offset // $ExpectType number
return p1
})(str)
result // $ExpectType string
})
})
reverse<T>(input: T[]): T[]
It returns a reversed copy of list or string input
.
Try this R.reverse example in Rambda REPL
reverse<T>(input: T[]): T[];
reverse(input: string): string;
export function reverse(listOrString) {
if (typeof listOrString === 'string') {
return listOrString.split('').reverse().join('')
}
const clone = listOrString.slice()
return clone.reverse()
}
import {reverse} from './reverse.js'
test('happy', () => {
expect(reverse([1, 2, 3])).toEqual([3, 2, 1])
})
test('with string', () => {
expect(reverse('baz')).toBe('zab')
})
test("it doesn't mutate", () => {
const arr = [1, 2, 3]
expect(reverse(arr)).toEqual([3, 2, 1])
expect(arr).toEqual([1, 2, 3])
})
import {reverse} from 'rambda'
const list = [1, 2, 3, 4, 5]
describe('R.reverse', () => {
it('happy', () => {
const result = reverse(list)
result // $ExpectType number[]
})
})
set<S, A>(lens: Lens<S, A>): {
(a: A): (obj: S) => S
(a: A, obj: S): S
}
It returns a copied Object or Array with modified lens
focus set to replacer
value.
Try this R.set example in Rambda REPL
set<S, A>(lens: Lens<S, A>): {
(a: A): (obj: S) => S
(a: A, obj: S): S
};
set<S, A>(lens: Lens<S, A>, a: A): (obj: S) => S;
set<S, A>(lens: Lens<S, A>, a: A, obj: S): S;
import {always} from './always.js'
import {curry} from './curry.js'
import {over} from './over.js'
function setFn(lens, replacer, x) {
return over(lens, always(replacer), x)
}
export const set = curry(setFn)
import {assoc} from './assoc.js'
import {lens} from './lens.js'
import {lensIndex} from './lensIndex.js'
import {lensPath} from './lensPath.js'
import {prop} from './prop.js'
import {set} from './set.js'
const testObject = {
foo: 'bar',
baz: {
a: 'x',
b: 'y',
},
}
test('assoc lens', () => {
const assocLens = lens(prop('foo'), assoc('foo'))
const result = set(assocLens, 'FOO', testObject)
const expected = {
...testObject,
foo: 'FOO',
}
expect(result).toEqual(expected)
})
test('path lens', () => {
const pathLens = lensPath('baz.a')
const result = set(pathLens, 'z', testObject)
const expected = {
...testObject,
baz: {
a: 'z',
b: 'y',
},
}
expect(result).toEqual(expected)
})
test('index lens', () => {
const indexLens = lensIndex(0)
const result = set(indexLens, 3, [1, 2])
expect(result).toEqual([3, 2])
})
slice(from: number, to: number, input: string): string
Try this R.slice example in Rambda REPL
slice(from: number, to: number, input: string): string;
slice<T>(from: number, to: number, input: T[]): T[];
slice(from: number, to: number): {
(input: string): string;
<T>(input: T[]): T[];
};
slice(from: number): {
(to: number, input: string): string;
<T>(to: number, input: T[]): T[];
};
import { curry } from './curry.js'
function sliceFn(
from, to, list
){
return list.slice(from, to)
}
export const slice = curry(sliceFn)
import { slice } from './slice.js'
test('slice', () => {
expect(slice(
1, 3, [ 'a', 'b', 'c', 'd' ]
)).toEqual([ 'b', 'c' ])
expect(slice(
1, Infinity, [ 'a', 'b', 'c', 'd' ]
)).toEqual([ 'b', 'c', 'd' ])
expect(slice(
0, -1, [ 'a', 'b', 'c', 'd' ]
)).toEqual([ 'a', 'b', 'c' ])
expect(slice(
-3, -1, [ 'a', 'b', 'c', 'd' ]
)).toEqual([ 'b', 'c' ])
expect(slice(
0, 3, 'ramda'
)).toBe('ram')
})
import {slice} from 'rambda'
const list = [1, 2, 3, 4, 5]
describe('R.slice', () => {
it('happy', () => {
const result = slice(1, 3, list)
result // $ExpectType number[]
})
it('curried', () => {
const result = slice(1, 3)(list)
result // $ExpectType number[]
})
})
sort<T>(sortFn: (a: T, b: T) => number, list: T[]): T[]
It returns copy of list
sorted by sortFn
function, where sortFn
needs to return only -1
, 0
or 1
.
Try this R.sort example in Rambda REPL
sort<T>(sortFn: (a: T, b: T) => number, list: T[]): T[];
sort<T>(sortFn: (a: T, b: T) => number): (list: T[]) => T[];
import { cloneList } from './_internals/cloneList.js'
export function sort(sortFn, list){
if (arguments.length === 1) return _list => sort(sortFn, _list)
return cloneList(list).sort(sortFn)
}
import { sort } from './sort.js'
const fn = (a, b) => a > b ? 1 : -1
test('sort', () => {
expect(sort((a, b) => a - b)([ 2, 3, 1 ])).toEqual([ 1, 2, 3 ])
})
test('it doesn\'t mutate', () => {
const list = [ 'foo', 'bar', 'baz' ]
expect(sort(fn, list)).toEqual([ 'bar', 'baz', 'foo' ])
expect(list[ 0 ]).toBe('foo')
expect(list[ 1 ]).toBe('bar')
expect(list[ 2 ]).toBe('baz')
})
import {sort} from 'rambda'
const list = [3, 0, 5, 2, 1]
function sortFn(a: number, b: number): number {
return a > b ? 1 : -1
}
describe('R.sort', () => {
it('happy', () => {
const result = sort(sortFn, list)
result // $ExpectType number[]
})
it('curried', () => {
const result = sort(sortFn)(list)
result // $ExpectType number[]
})
})
sortBy<T>(sortFn: (a: T) => Ord, list: T[]): T[]
It returns copy of list
sorted by sortFn
function, where sortFn
function returns a value to compare, i.e. it doesn't need to return only -1
, 0
or 1
.
Try this R.sortBy example in Rambda REPL
sortBy<T>(sortFn: (a: T) => Ord, list: T[]): T[];
sortBy<T>(sortFn: (a: T) => Ord): (list: T[]) => T[];
sortBy(sortFn: (a: any) => Ord): <T>(list: T[]) => T[];
import { cloneList } from './_internals/cloneList.js'
export function sortBy(sortFn, list){
if (arguments.length === 1) return _list => sortBy(sortFn, _list)
const clone = cloneList(list)
return clone.sort((a, b) => {
const aSortResult = sortFn(a)
const bSortResult = sortFn(b)
if (aSortResult === bSortResult) return 0
return aSortResult < bSortResult ? -1 : 1
})
}
import { compose } from './compose.js'
import { prop } from './prop.js'
import { sortBy } from './sortBy.js'
import { toLower } from './toLower.js'
test('happy', () => {
const input = [ { a : 2 }, { a : 1 }, { a : 1 }, { a : 3 } ]
const expected = [ { a : 1 }, { a : 1 }, { a : 2 }, { a : 3 } ]
const result = sortBy(x => x.a)(input)
expect(result).toEqual(expected)
})
test('with compose', () => {
const alice = {
name : 'ALICE',
age : 101,
}
const bob = {
name : 'Bob',
age : -10,
}
const clara = {
name : 'clara',
age : 314.159,
}
const people = [ clara, bob, alice ]
const sortByNameCaseInsensitive = sortBy(compose(toLower, prop('name')))
expect(sortByNameCaseInsensitive(people)).toEqual([ alice, bob, clara ])
})
import {sortBy, pipe} from 'rambda'
interface Input {
a: number,
}
describe('R.sortBy', () => {
it('passing type to sort function', () => {
function fn(x: any): number {
return x.a
}
function fn2(x: Input): number {
return x.a
}
const input = [{a: 2}, {a: 1}, {a: 0}]
const result = sortBy(fn, input)
const curriedResult = sortBy(fn2)(input)
result // $ExpectType { a: number; }[]
curriedResult // $ExpectType Input[]
result[0].a // $ExpectType number
curriedResult[0].a // $ExpectType number
})
it('passing type to sort function and list', () => {
function fn(x: Input): number {
return x.a
}
const input: Input[] = [{a: 2}, {a: 1}, {a: 0}]
const result = sortBy(fn, input)
const curriedResult = sortBy(fn)(input)
result // $ExpectType Input[]
curriedResult // $ExpectType Input[]
result[0].a // $ExpectType number
})
it('with R.pipe', () => {
interface Obj {
value: number,
}
const fn = pipe(sortBy<Obj>(x => x.value))
const result = fn([{value: 1}, {value: 2}])
result // $ExpectType Obj[]
})
})
Try this R.sortWith example in Rambda REPL
split(separator: string | RegExp): (str: string) => string[]
Curried version of String.prototype.split
Try this R.split example in Rambda REPL
split(separator: string | RegExp): (str: string) => string[];
split(separator: string | RegExp, str: string): string[];
export function split(separator, str){
if (arguments.length === 1) return _str => split(separator, _str)
return str.split(separator)
}
import { split } from './split.js'
const str = 'foo|bar|baz'
const splitChar = '|'
const expected = [ 'foo', 'bar', 'baz' ]
test('happy', () => {
expect(split(splitChar, str)).toEqual(expected)
})
test('curried', () => {
expect(split(splitChar)(str)).toEqual(expected)
})
import {split} from 'rambda'
const str = 'foo|bar|baz'
const splitChar = '|'
describe('R.split', () => {
it('happy', () => {
const result = split(splitChar, str)
result // $ExpectType string[]
})
it('curried', () => {
const result = split(splitChar)(str)
result // $ExpectType string[]
})
})
splitAt<T>(index: number, input: T[]): [T[], T[]]
It splits string or array at a given index.
Try this R.splitAt example in Rambda REPL
splitAt<T>(index: number, input: T[]): [T[], T[]];
splitAt(index: number, input: string): [string, string];
splitAt(index: number): {
<T>(input: T[]): [T[], T[]];
(input: string): [string, string];
};
import { isArray } from './_internals/isArray.js'
import { drop } from './drop.js'
import { maybe } from './maybe.js'
import { take } from './take.js'
export function splitAt(index, input){
if (arguments.length === 1){
return _list => splitAt(index, _list)
}
if (!input) throw new TypeError(`Cannot read property 'slice' of ${ input }`)
if (!isArray(input) && typeof input !== 'string') return [ [], [] ]
const correctIndex = maybe(
index < 0,
input.length + index < 0 ? 0 : input.length + index,
index
)
return [ take(correctIndex, input), drop(correctIndex, input) ]
}
import { splitAt as splitAtRamda } from 'ramda'
import { splitAt } from './splitAt.js'
const list = [ 1, 2, 3 ]
const str = 'foo bar'
test('with array', () => {
const result = splitAt(2, list)
expect(result).toEqual([ [ 1, 2 ], [ 3 ] ])
})
test('with array - index is negative number', () => {
const result = splitAt(-6, list)
expect(result).toEqual([ [], list ])
})
test('with array - index is out of scope', () => {
const result = splitAt(4, list)
expect(result).toEqual([ [ 1, 2, 3 ], [] ])
})
test('with string', () => {
const result = splitAt(4, str)
expect(result).toEqual([ 'foo ', 'bar' ])
})
test('with string - index is negative number', () => {
const result = splitAt(-2, str)
expect(result).toEqual([ 'foo b', 'ar' ])
})
test('with string - index is out of scope', () => {
const result = splitAt(10, str)
expect(result).toEqual([ str, '' ])
})
test('with array - index is out of scope', () => {
const result = splitAt(4)(list)
expect(result).toEqual([ [ 1, 2, 3 ], [] ])
})
const badInputs = [ 1, true, /foo/g, {} ]
const throwingBadInputs = [ null, undefined ]
test('with bad inputs', () => {
throwingBadInputs.forEach(badInput => {
expect(() => splitAt(1, badInput)).toThrowWithMessage(TypeError,
`Cannot read property 'slice' of ${ badInput }`)
expect(() => splitAtRamda(1, badInput)).toThrowWithMessage(TypeError,
`Cannot read properties of ${ badInput } (reading 'slice')`)
})
badInputs.forEach(badInput => {
const result = splitAt(1, badInput)
const ramdaResult = splitAtRamda(1, badInput)
expect(result).toEqual(ramdaResult)
})
})
import {splitAt} from 'rambda'
const index = 1
const str = 'foo'
const list = [1, 2, 3]
describe('R.splitAt with array', () => {
it('happy', () => {
const result = splitAt(index, list)
result // $ExpectType [number[], number[]]
})
it('curried', () => {
const result = splitAt(index)(list)
result // $ExpectType [number[], number[]]
})
})
describe('R.splitAt with string', () => {
it('happy', () => {
const result = splitAt(index, str)
result // $ExpectType [string, string]
})
it('curried', () => {
const result = splitAt(index)(str)
result // $ExpectType [string, string]
})
})
splitEvery<T>(sliceLength: number, input: T[]): (T[])[]
It splits input
into slices of sliceLength
.
Try this R.splitEvery example in Rambda REPL
splitEvery<T>(sliceLength: number, input: T[]): (T[])[];
splitEvery(sliceLength: number, input: string): string[];
splitEvery(sliceLength: number): {
(input: string): string[];
<T>(input: T[]): (T[])[];
};
export function splitEvery(sliceLength, listOrString){
if (arguments.length === 1){
return _listOrString => splitEvery(sliceLength, _listOrString)
}
if (sliceLength < 1){
throw new Error('First argument to splitEvery must be a positive integer')
}
const willReturn = []
let counter = 0
while (counter < listOrString.length){
willReturn.push(listOrString.slice(counter, counter += sliceLength))
}
return willReturn
}
import { splitEvery } from './splitEvery.js'
test('happy', () => {
expect(splitEvery(3, [ 1, 2, 3, 4, 5, 6, 7 ])).toEqual([
[ 1, 2, 3 ],
[ 4, 5, 6 ],
[ 7 ],
])
expect(splitEvery(3)('foobarbaz')).toEqual([ 'foo', 'bar', 'baz' ])
})
test('with bad input', () => {
expect(() =>
expect(splitEvery(0)('foo')).toEqual([ 'f', 'o', 'o' ])).toThrowErrorMatchingInlineSnapshot('"First argument to splitEvery must be a positive integer"')
})
import {splitEvery} from 'rambda'
const list = [1, 2, 3, 4, 5, 6, 7]
describe('R.splitEvery', () => {
it('happy', () => {
const result = splitEvery(3, list)
result // $ExpectType number[][]
})
it('curried', () => {
const result = splitEvery(3)(list)
result // $ExpectType number[][]
})
})
splitWhen<T, U>(predicate: Predicate<T>, list: U[]): (U[])[]
It splits list
to two arrays according to a predicate
function.
The first array contains all members of list
before predicate
returns true
.
Try this R.splitWhen example in Rambda REPL
splitWhen<T, U>(predicate: Predicate<T>, list: U[]): (U[])[];
splitWhen<T>(predicate: Predicate<T>): <U>(list: U[]) => (U[])[];
export function splitWhen(predicate, input){
if (arguments.length === 1){
return _input => splitWhen(predicate, _input)
}
if (!input)
throw new TypeError(`Cannot read property 'length' of ${ input }`)
const preFound = []
const postFound = []
let found = false
let counter = -1
while (counter++ < input.length - 1){
if (found){
postFound.push(input[ counter ])
} else if (predicate(input[ counter ])){
postFound.push(input[ counter ])
found = true
} else {
preFound.push(input[ counter ])
}
}
return [ preFound, postFound ]
}
import { splitWhen as splitWhenRamda } from 'ramda'
import { equals } from './equals.js'
import { splitWhen } from './splitWhen.js'
const list = [ 1, 2, 1, 2 ]
test('happy', () => {
const result = splitWhen(equals(2), list)
expect(result).toEqual([ [ 1 ], [ 2, 1, 2 ] ])
})
test('when predicate returns false', () => {
const result = splitWhen(equals(3))(list)
expect(result).toEqual([ list, [] ])
})
const badInputs = [ 1, true, /foo/g, {} ]
const throwingBadInputs = [ null, undefined ]
test('with bad inputs', () => {
throwingBadInputs.forEach(badInput => {
expect(() => splitWhen(equals(2), badInput)).toThrowWithMessage(TypeError,
`Cannot read property 'length' of ${ badInput }`)
expect(() => splitWhenRamda(equals(2), badInput)).toThrowWithMessage(TypeError,
`Cannot read properties of ${ badInput } (reading 'length')`)
})
badInputs.forEach(badInput => {
const result = splitWhen(equals(2), badInput)
const ramdaResult = splitWhenRamda(equals(2), badInput)
expect(result).toEqual(ramdaResult)
})
})
import {splitWhen} from 'rambda'
const list = [1, 2, 1, 2]
const predicate = (x: number) => x === 2
describe('R.splitWhen', () => {
it('happy', () => {
const result = splitWhen(predicate, list)
result // $ExpectType number[][]
})
it('curried', () => {
const result = splitWhen(predicate)(list)
result // $ExpectType number[][]
})
})
startsWith<T extends string>(question: T, input: string): boolean
When iterable is a string, then it behaves as String.prototype.startsWith
.
When iterable is a list, then it uses R.equals to determine if the target list starts in the same way as the given target.
Try this R.startsWith example in Rambda REPL
startsWith<T extends string>(question: T, input: string): boolean;
startsWith<T extends string>(question: T): (input: string) => boolean;
startsWith<T>(question: T[], input: T[]): boolean;
startsWith<T>(question: T[]): (input: T[]) => boolean;
import { isArray } from './_internals/isArray.js'
import { equals } from './equals.js'
export function startsWith(question, iterable){
if (arguments.length === 1)
return _iterable => startsWith(question, _iterable)
if (typeof iterable === 'string'){
return iterable.startsWith(question)
}
if (!isArray(question)) return false
let correct = true
const filtered = question.filter((x, index) => {
if (!correct) return false
const result = equals(x, iterable[ index ])
if (!result) correct = false
return result
})
return filtered.length === question.length
}
import { startsWith as startsWithRamda } from 'ramda'
import { compareCombinations } from './_internals/testUtils.js'
import { possibleIterables, possibleTargets } from './endsWith.spec.js'
import { startsWith } from './startsWith.js'
test('with string', () => {
expect(startsWith('foo', 'foo-bar')).toBeTrue()
expect(startsWith('baz')('foo-bar')).toBeFalse()
})
test('use R.equals with array', () => {
const list = [ { a : 1 }, { a : 2 }, { a : 3 } ]
expect(startsWith({ a : 1 }, list)).toBeFalse()
expect(startsWith([ { a : 1 } ], list)).toBeTrue()
expect(startsWith([ { a : 1 }, { a : 2 } ], list)).toBeTrue()
expect(startsWith(list, list)).toBeTrue()
expect(startsWith([ { a : 2 } ], list)).toBeFalse()
})
describe('brute force', () => {
compareCombinations({
fn : startsWith,
fnRamda : startsWithRamda,
firstInput : possibleTargets,
secondInput : possibleIterables,
callback : errorsCounters => {
expect(errorsCounters).toMatchInlineSnapshot(`
{
"ERRORS_MESSAGE_MISMATCH": 0,
"ERRORS_TYPE_MISMATCH": 0,
"RESULTS_MISMATCH": 0,
"SHOULD_NOT_THROW": 0,
"SHOULD_THROW": 0,
"TOTAL_TESTS": 32,
}
`)
},
})
})
import {startsWith} from 'rambda'
describe('R.startsWith - array', () => {
const question = [{a: 1}]
const iterable = [{a: 1}, {a: 2}]
it('happy', () => {
const result = startsWith(question, iterable)
result // $ExpectType boolean
})
it('curried', () => {
const result = startsWith(question)(iterable)
result // $ExpectType boolean
})
})
describe('R.startsWith - string', () => {
const question = 'foo'
const iterable = 'foo bar'
it('happy', () => {
const result = startsWith(question, iterable)
result // $ExpectType boolean
})
it('curried', () => {
const result = startsWith(question)(iterable)
result // $ExpectType boolean
})
})
Curried version of x - y
Try this R.subtract example in Rambda REPL
sum(list: number[]): number
Try this R.sum example in Rambda REPL
sum(list: number[]): number;
export function sum(list){
return list.reduce((prev, current) => prev + current, 0)
}
import { sum } from './sum.js'
test('happy', () => {
expect(sum([ 1, 2, 3, 4, 5 ])).toBe(15)
})
symmetricDifference<T>(x: T[], y: T[]): T[]
It returns a merged list of x
and y
with all equal elements removed.
R.equals
is used to determine equality.
Try this R.symmetricDifference example in Rambda REPL
symmetricDifference<T>(x: T[], y: T[]): T[];
symmetricDifference<T>(x: T[]): <T>(y: T[]) => T[];
import { concat } from './concat.js'
import { filter } from './filter.js'
import { includes } from './includes.js'
export function symmetricDifference(x, y){
if (arguments.length === 1){
return _y => symmetricDifference(x, _y)
}
return concat(filter(value => !includes(value, y), x),
filter(value => !includes(value, x), y))
}
import { symmetricDifference } from './symmetricDifference.js'
test('symmetricDifference', () => {
const list1 = [ 1, 2, 3, 4 ]
const list2 = [ 3, 4, 5, 6 ]
expect(symmetricDifference(list1)(list2)).toEqual([ 1, 2, 5, 6 ])
expect(symmetricDifference([], [])).toEqual([])
})
test('symmetricDifference with objects', () => {
const list1 = [ { id : 1 }, { id : 2 }, { id : 3 }, { id : 4 } ]
const list2 = [ { id : 3 }, { id : 4 }, { id : 5 }, { id : 6 } ]
expect(symmetricDifference(list1)(list2)).toEqual([
{ id : 1 },
{ id : 2 },
{ id : 5 },
{ id : 6 },
])
})
import {symmetricDifference} from 'rambda'
describe('R.symmetricDifference', () => {
it('happy', () => {
const list1 = [1, 2, 3, 4]
const list2 = [3, 4, 5, 6]
const result = symmetricDifference(list1, list2)
result // $ExpectType number[]
})
it('curried', () => {
const list1 = [{id: 1}, {id: 2}, {id: 3}, {id: 4}]
const list2 = [{id: 3}, {id: 4}, {id: 5}, {id: 6}]
const result = symmetricDifference(list1)(list2)
result // $ExpectType { id: number; }[]
})
})
T(): boolean
Try this R.T example in Rambda REPL
T(): boolean;
export function T(){
return true
}
tail<T extends unknown[]>(input: T): T extends [any, ...infer U] ? U : [...T]
It returns all but the first element of input
.
Try this R.tail example in Rambda REPL
tail<T extends unknown[]>(input: T): T extends [any, ...infer U] ? U : [...T];
tail(input: string): string;
import { drop } from './drop.js'
export function tail(listOrString){
return drop(1, listOrString)
}
import { tail } from './tail.js'
test('tail', () => {
expect(tail([ 1, 2, 3 ])).toEqual([ 2, 3 ])
expect(tail([ 1, 2 ])).toEqual([ 2 ])
expect(tail([ 1 ])).toEqual([])
expect(tail([])).toEqual([])
expect(tail('abc')).toBe('bc')
expect(tail('ab')).toBe('b')
expect(tail('a')).toBe('')
expect(tail('')).toBe('')
})
import {tail} from 'rambda'
describe('R.tail', () => {
it('with string', () => {
const result = tail('foo')
result // $ExpectType string
})
it('with list - one type', () => {
const result = tail([1, 2, 3])
result // $ExpectType number[]
})
it('with list - mixed types', () => {
const result = tail(['foo', 'bar', 1, 2, 3])
result // $ExpectType (string | number)[]
})
})
take<T>(howMany: number, input: T[]): T[]
It returns the first howMany
elements of input
.
Try this R.take example in Rambda REPL
take<T>(howMany: number, input: T[]): T[];
take(howMany: number, input: string): string;
take<T>(howMany: number): {
<T>(input: T[]): T[];
(input: string): string;
};
import baseSlice from './_internals/baseSlice.js'
export function take(howMany, listOrString){
if (arguments.length === 1)
return _listOrString => take(howMany, _listOrString)
if (howMany < 0) return listOrString.slice()
if (typeof listOrString === 'string') return listOrString.slice(0, howMany)
return baseSlice(
listOrString, 0, howMany
)
}
import { take } from './take.js'
test('happy', () => {
const arr = [ 'foo', 'bar', 'baz' ]
expect(take(1, arr)).toEqual([ 'foo' ])
expect(arr).toEqual([ 'foo', 'bar', 'baz' ])
expect(take(2)([ 'foo', 'bar', 'baz' ])).toEqual([ 'foo', 'bar' ])
expect(take(3, [ 'foo', 'bar', 'baz' ])).toEqual([ 'foo', 'bar', 'baz' ])
expect(take(4, [ 'foo', 'bar', 'baz' ])).toEqual([ 'foo', 'bar', 'baz' ])
expect(take(3)('rambda')).toBe('ram')
})
test('with negative index', () => {
expect(take(-1, [ 1, 2, 3 ])).toEqual([ 1, 2, 3 ])
expect(take(-Infinity, [ 1, 2, 3 ])).toEqual([ 1, 2, 3 ])
})
test('with zero index', () => {
expect(take(0, [ 1, 2, 3 ])).toEqual([])
})
import {take} from 'rambda'
const list = [1, 2, 3, 4]
const str = 'foobar'
const howMany = 2
describe('R.take - array', () => {
it('happy', () => {
const result = take(howMany, list)
result // $ExpectType number[]
})
it('curried', () => {
const result = take(howMany)(list)
result // $ExpectType number[]
})
})
describe('R.take - string', () => {
it('happy', () => {
const result = take(howMany, str)
result // $ExpectType string
})
it('curried', () => {
const result = take(howMany)(str)
result // $ExpectType string
})
})
takeLast<T>(howMany: number, input: T[]): T[]
It returns the last howMany
elements of input
.
Try this R.takeLast example in Rambda REPL
takeLast<T>(howMany: number, input: T[]): T[];
takeLast(howMany: number, input: string): string;
takeLast<T>(howMany: number): {
<T>(input: T[]): T[];
(input: string): string;
};
import baseSlice from './_internals/baseSlice.js'
export function takeLast(howMany, listOrString){
if (arguments.length === 1)
return _listOrString => takeLast(howMany, _listOrString)
const len = listOrString.length
if (howMany < 0) return listOrString.slice()
let numValue = howMany > len ? len : howMany
if (typeof listOrString === 'string')
return listOrString.slice(len - numValue)
numValue = len - numValue
return baseSlice(
listOrString, numValue, len
)
}
import { takeLast } from './takeLast.js'
test('with arrays', () => {
expect(takeLast(1, [ 'foo', 'bar', 'baz' ])).toEqual([ 'baz' ])
expect(takeLast(2)([ 'foo', 'bar', 'baz' ])).toEqual([ 'bar', 'baz' ])
expect(takeLast(3, [ 'foo', 'bar', 'baz' ])).toEqual([ 'foo', 'bar', 'baz' ])
expect(takeLast(4, [ 'foo', 'bar', 'baz' ])).toEqual([ 'foo', 'bar', 'baz' ])
expect(takeLast(10, [ 'foo', 'bar', 'baz' ])).toEqual([ 'foo', 'bar', 'baz' ])
})
test('with strings', () => {
expect(takeLast(3, 'rambda')).toBe('bda')
expect(takeLast(7, 'rambda')).toBe('rambda')
})
test('with negative index', () => {
expect(takeLast(-1, [ 1, 2, 3 ])).toEqual([ 1, 2, 3 ])
expect(takeLast(-Infinity, [ 1, 2, 3 ])).toEqual([ 1, 2, 3 ])
})
import {takeLast} from 'rambda'
const list = [1, 2, 3, 4]
const str = 'foobar'
const howMany = 2
describe('R.takeLast - array', () => {
it('happy', () => {
const result = takeLast(howMany, list)
result // $ExpectType number[]
})
it('curried', () => {
const result = takeLast(howMany)(list)
result // $ExpectType number[]
})
})
describe('R.takeLast - string', () => {
it('happy', () => {
const result = takeLast(howMany, str)
result // $ExpectType string
})
it('curried', () => {
const result = takeLast(howMany)(str)
result // $ExpectType string
})
})
takeLastWhile(predicate: (x: string) => boolean, input: string): string
Try this R.takeLastWhile example in Rambda REPL
takeLastWhile(predicate: (x: string) => boolean, input: string): string;
takeLastWhile(predicate: (x: string) => boolean): (input: string) => string;
takeLastWhile<T>(predicate: (x: T) => boolean, input: T[]): T[];
takeLastWhile<T>(predicate: (x: T) => boolean): <T>(input: T[]) => T[];
import { isArray } from './_internals/isArray.js'
export function takeLastWhile(predicate, input){
if (arguments.length === 1){
return _input => takeLastWhile(predicate, _input)
}
if (input.length === 0) return input
const toReturn = []
let counter = input.length
while (counter){
const item = input[ --counter ]
if (!predicate(item)){
break
}
toReturn.push(item)
}
return isArray(input) ? toReturn.reverse() : toReturn.reverse().join('')
}
import { takeLastWhile } from './takeLastWhile.js'
const assert = require('assert')
const list = [ 1, 2, 3, 4 ]
test('happy', () => {
const predicate = x => x > 2
const result = takeLastWhile(predicate, list)
expect(result).toEqual([ 3, 4 ])
})
test('predicate is always true', () => {
const predicate = () => true
const result = takeLastWhile(predicate)(list)
expect(result).toEqual(list)
})
test('predicate is always false', () => {
const predicate = () => false
const result = takeLastWhile(predicate, list)
expect(result).toEqual([])
})
test('with string', () => {
const result = takeLastWhile(x => x !== 'F', 'FOOBAR')
expect(result).toBe('OOBAR')
})
import {takeLastWhile} from 'rambda'
const list = [1, 2, 3]
const str = 'FOO'
describe('R.takeLastWhile', () => {
it('with array', () => {
const result = takeLastWhile(x => x > 1, list)
result // $ExpectType number[]
})
it('with array - curried', () => {
const result = takeLastWhile(x => x > 1, list)
result // $ExpectType number[]
})
it('with string', () => {
const result = takeLastWhile(x => x !== 'F', str)
result // $ExpectType string
})
it('with string - curried', () => {
const result = takeLastWhile(x => x !== 'F')(str)
result // $ExpectType string
})
})
Try this R.takeWhile example in Rambda REPL
tap<T>(fn: (x: T) => void, input: T): T
It applies function fn
to input x
and returns x
.
One use case is debugging in the middle of R.compose
.
Try this R.tap example in Rambda REPL
tap<T>(fn: (x: T) => void, input: T): T;
tap<T>(fn: (x: T) => void): (input: T) => T;
export function tap(fn, x){
if (arguments.length === 1) return _x => tap(fn, _x)
fn(x)
return x
}
import { tap } from './tap.js'
test('tap', () => {
let a = 1
const sayX = x => a = x
expect(tap(sayX, 100)).toBe(100)
expect(tap(sayX)(100)).toBe(100)
expect(a).toBe(100)
})
import {tap, pipe} from 'rambda'
describe('R.tap', () => {
it('happy', () => {
pipe(
tap(x => {
x // $ExpectType number[]
}),
(x: number[]) => x.length
)([1, 2])
})
})
test(regExpression: RegExp): (str: string) => boolean
It determines whether str
matches regExpression
.
Try this R.test example in Rambda REPL
test(regExpression: RegExp): (str: string) => boolean;
test(regExpression: RegExp, str: string): boolean;
export function test(pattern, str){
if (arguments.length === 1) return _str => test(pattern, _str)
if (typeof pattern === 'string'){
throw new TypeError(`R.test requires a value of type RegExp as its first argument; received "${ pattern }"`)
}
return str.search(pattern) !== -1
}
import { test as testMethod } from './test.js'
test('happy', () => {
expect(testMethod(/^x/, 'xyz')).toBeTrue()
expect(testMethod(/^y/)('xyz')).toBeFalse()
})
test('throws if first argument is not regex', () => {
expect(() => testMethod('foo', 'bar')).toThrowErrorMatchingInlineSnapshot('"R.test requires a value of type RegExp as its first argument; received "foo""')
})
import {test} from 'rambda'
const input = 'foo '
const regex = /foo/
describe('R.test', () => {
it('happy', () => {
const result = test(regex, input)
result // $ExpectType boolean
})
it('curried', () => {
const result = test(regex)(input)
result // $ExpectType boolean
})
})
times<T>(fn: (i: number) => T, howMany: number): T[]
It returns the result of applying function fn
over members of range array.
The range array includes numbers between 0
and howMany
(exclusive).
Try this R.times example in Rambda REPL
times<T>(fn: (i: number) => T, howMany: number): T[];
times<T>(fn: (i: number) => T): (howMany: number) => T[];
import { isInteger } from './_internals/isInteger.js'
import { map } from './map.js'
import { range } from './range.js'
export function times(fn, howMany){
if (arguments.length === 1) return _howMany => times(fn, _howMany)
if (!isInteger(howMany) || howMany < 0){
throw new RangeError('n must be an integer')
}
return map(fn, range(0, howMany))
}
import assert from 'assert'
import { identity } from './identity.js'
import { times } from './times.js'
test('happy', () => {
const result = times(identity, 5)
expect(result).toEqual([ 0, 1, 2, 3, 4 ])
})
test('with bad input', () => {
assert.throws(() => {
times(3)('cheers!')
}, RangeError)
assert.throws(() => {
times(identity, -1)
}, RangeError)
})
test('curry', () => {
const result = times(identity)(5)
expect(result).toEqual([ 0, 1, 2, 3, 4 ])
})
import {times, identity} from 'rambda'
describe('R.times', () => {
it('happy', () => {
const result = times(identity, 5)
result // $ExpectType number[]
})
})
toLower<S extends string>(str: S): Lowercase<S>
Try this R.toLower example in Rambda REPL
toLower<S extends string>(str: S): Lowercase<S>;
toLower(str: string): string;
export function toLower(str){
return str.toLowerCase()
}
import { toLower } from './toLower.js'
test('toLower', () => {
expect(toLower('FOO|BAR|BAZ')).toBe('foo|bar|baz')
})
toPairs<O extends object, K extends Extract<keyof O, string | number>>(obj: O): Array<{ [key in K]: [`${key}`, O[key]] }[K]>
It transforms an object to a list.
Try this R.toPairs example in Rambda REPL
toPairs<O extends object, K extends Extract<keyof O, string | number>>(obj: O): Array<{ [key in K]: [`${key}`, O[key]] }[K]>;
toPairs<S>(obj: Record<string | number, S>): Array<[string, S]>;
export function toPairs(obj){
return Object.entries(obj)
}
import { toPairs } from './toPairs.js'
const obj = {
a : 1,
b : 2,
c : [ 3, 4 ],
}
const expected = [
[ 'a', 1 ],
[ 'b', 2 ],
[ 'c', [ 3, 4 ] ],
]
test('happy', () => {
expect(toPairs(obj)).toEqual(expected)
})
toString(x: unknown): string
Try this R.toString example in Rambda REPL
toString(x: unknown): string;
export function toString(x){
return x.toString()
}
import { toString } from './toString.js'
test('happy', () => {
expect(toString([ 1, 2, 3 ])).toBe('1,2,3')
})
toUpper<S extends string>(str: S): Uppercase<S>
Try this R.toUpper example in Rambda REPL
toUpper<S extends string>(str: S): Uppercase<S>;
toUpper(str: string): string;
export function toUpper(str){
return str.toUpperCase()
}
import { toUpper } from './toUpper.js'
test('toUpper', () => {
expect(toUpper('foo|bar|baz')).toBe('FOO|BAR|BAZ')
})
transpose<T>(list: (T[])[]): (T[])[]
Try this R.transpose example in Rambda REPL
transpose<T>(list: (T[])[]): (T[])[];
import { isArray } from './_internals/isArray.js'
export function transpose(array){
return array.reduce((acc, el) => {
el.forEach((nestedEl, i) =>
isArray(acc[ i ]) ? acc[ i ].push(nestedEl) : acc.push([ nestedEl ]))
return acc
}, [])
}
import { transpose } from './transpose.js'
test('happy', () => {
const input = [
[ 'a', 1 ],
[ 'b', 2 ],
[ 'c', 3 ],
]
expect(transpose(input)).toEqual([
[ 'a', 'b', 'c' ],
[ 1, 2, 3 ],
])
})
test('when rows are shorter', () => {
const actual = transpose([ [ 10, 11 ], [ 20 ], [], [ 30, 31, 32 ] ])
const expected = [ [ 10, 20, 30 ], [ 11, 31 ], [ 32 ] ]
expect(actual).toEqual(expected)
})
test('with empty array', () => {
expect(transpose([])).toEqual([])
})
test('array with falsy values', () => {
const actual = transpose([
[ true, false, undefined, null ],
[ null, undefined, false, true ],
])
const expected = [
[ true, null ],
[ false, undefined ],
[ undefined, false ],
[ null, true ],
]
expect(actual).toEqual(expected)
})
import {transpose} from 'rambda'
const input = [
['a', 1],
['b', 2],
['c', 3],
]
describe('R.transpose', () => {
it('happy', () => {
const result = transpose(input)
result // $ExpectType (string | number)[][]
})
})
trim(str: string): string
Try this R.trim example in Rambda REPL
trim(str: string): string;
export function trim(str){
return str.trim()
}
import { trim } from './trim.js'
test('trim', () => {
expect(trim(' foo ')).toBe('foo')
})
It returns function that runs fn
in try/catch
block. If there was an error, then fallback
is used to return the result. Note that fn
can be value or asynchronous/synchronous function(unlike Ramda
where fallback can only be a synchronous function).
Try this R.tryCatch example in Rambda REPL
It accepts any input and it returns its type.
Try this R.type example in Rambda REPL
unapply<T = any>(fn: (args: any[]) => T): (...args: any[]) => T
It calls a function fn
with the list of values of the returned function.
R.unapply
is the opposite of R.apply
method.
Try this R.unapply example in Rambda REPL
unapply<T = any>(fn: (args: any[]) => T): (...args: any[]) => T;
export function unapply(fn){
return function (...args){
return fn.call(this, args)
}
}
import { apply } from './apply.js'
import { converge } from './converge.js'
import { identity } from './identity.js'
import { prop } from './prop.js'
import { sum } from './sum.js'
import { unapply } from './unapply.js'
test('happy', () => {
const fn = unapply(identity)
expect(fn(
1, 2, 3
)).toEqual([ 1, 2, 3 ])
expect(fn()).toEqual([])
})
test('returns a function which is always passed one argument', () => {
const fn = unapply(function (){
return arguments.length
})
expect(fn('x')).toBe(1)
expect(fn('x', 'y')).toBe(1)
expect(fn(
'x', 'y', 'z'
)).toBe(1)
})
test('forwards arguments to decorated function as an array', () => {
const fn = unapply(xs => '[' + xs + ']')
expect(fn(2)).toBe('[2]')
expect(fn(2, 4)).toBe('[2,4]')
expect(fn(
2, 4, 6
)).toBe('[2,4,6]')
})
test('returns a function with length 0', () => {
const fn = unapply(identity)
expect(fn).toHaveLength(0)
})
test('is the inverse of R.apply', () => {
let a, b, c, d, e, f, g, n
const rand = function (){
return Math.floor(200 * Math.random()) - 100
}
f = Math.max
g = unapply(apply(f))
n = 1
while (n <= 100){
a = rand()
b = rand()
c = rand()
d = rand()
e = rand()
expect(f(
a, b, c, d, e
)).toEqual(g(
a, b, c, d, e
))
n += 1
}
f = function (xs){
return '[' + xs + ']'
}
g = apply(unapply(f))
n = 1
while (n <= 100){
a = rand()
b = rand()
c = rand()
d = rand()
e = rand()
expect(f([ a, b, c, d, e ])).toEqual(g([ a, b, c, d, e ]))
n += 1
}
})
test('it works with converge', () => {
const fn = unapply(sum)
const convergeFn = converge(fn, [ prop('a'), prop('b'), prop('c') ])
const obj = {
a : 1337,
b : 42,
c : 1,
}
const expected = 1337 + 42 + 1
expect(convergeFn(obj)).toEqual(expected)
})
import {join, unapply, sum} from 'rambda'
describe('R.unapply', () => {
it('happy', () => {
const fn = unapply(sum)
fn(1, 2, 3) // $ExpectType number
})
it('joins a string', () => {
const fn = unapply(join(''))
fn('s', 't', 'r', 'i', 'n', 'g') // $ExpectType string
})
})
union<T>(x: T[], y: T[]): T[]
It takes two lists and return a new list containing a merger of both list with removed duplicates.
R.equals
is used to compare for duplication.
Try this R.union example in Rambda REPL
union<T>(x: T[], y: T[]): T[];
union<T>(x: T[]): (y: T[]) => T[];
import { cloneList } from './_internals/cloneList.js'
import { includes } from './includes.js'
export function union(x, y){
if (arguments.length === 1) return _y => union(x, _y)
const toReturn = cloneList(x)
y.forEach(yInstance => {
if (!includes(yInstance, x)) toReturn.push(yInstance)
})
return toReturn
}
import { union } from './union.js'
test('happy', () => {
expect(union([ 1, 2 ], [ 2, 3 ])).toEqual([ 1, 2, 3 ])
})
test('with list of objects', () => {
const list1 = [ { a : 1 }, { a : 2 } ]
const list2 = [ { a : 2 }, { a : 3 } ]
const result = union(list1)(list2)
})
import {union} from 'rambda'
describe('R.union', () => {
it('happy', () => {
const result = union([1, 2], [2, 3])
result // $ExpectType number[]
})
it('with array of objects - case 1', () => {
const list1 = [{a: 1}, {a: 2}]
const list2 = [{a: 2}, {a: 3}]
const result = union(list1, list2)
result // $ExpectType { a: number; }[]
})
it('with array of objects - case 2', () => {
const list1 = [{a: 1, b: 1}, {a: 2}]
const list2 = [{a: 2}, {a: 3, b: 3}]
const result = union(list1, list2)
result[0].a // $ExpectType number
result[0].b // $ExpectType number | undefined
})
})
describe('R.union - curried', () => {
it('happy', () => {
const result = union([1, 2])([2, 3])
result // $ExpectType number[]
})
it('with array of objects - case 1', () => {
const list1 = [{a: 1}, {a: 2}]
const list2 = [{a: 2}, {a: 3}]
const result = union(list1)(list2)
result // $ExpectType { a: number; }[]
})
it('with array of objects - case 2', () => {
const list1 = [{a: 1, b: 1}, {a: 2}]
const list2 = [{a: 2}, {a: 3, b: 3}]
const result = union(list1)(list2)
result[0].a // $ExpectType number
result[0].b // $ExpectType number | undefined
})
})
uniq<T>(list: T[]): T[]
It returns a new array containing only one copy of each element of list
.
R.equals
is used to determine equality.
Try this R.uniq example in Rambda REPL
uniq<T>(list: T[]): T[];
import { _Set } from './_internals/set.js'
export function uniq(list){
const set = new _Set()
const willReturn = []
list.forEach(item => {
if (set.checkUniqueness(item)){
willReturn.push(item)
}
})
return willReturn
}
import { uniq } from './uniq.js'
test('happy', () => {
const list = [ 1, 2, 3, 3, 3, 1, 2, 0 ]
expect(uniq(list)).toEqual([ 1, 2, 3, 0 ])
})
test('with object', () => {
const list = [ { a : 1 }, { a : 2 }, { a : 1 }, { a : 2 } ]
expect(uniq(list)).toEqual([ { a : 1 }, { a : 2 } ])
})
test('with nested array', () => {
expect(uniq([ [ 42 ], [ 42 ] ])).toEqual([ [ 42 ] ])
})
test('with booleans', () => {
expect(uniq([ [ false ], [ false ], [ true ] ])).toEqual([ [ false ], [ true ] ])
})
test('with falsy values', () => {
expect(uniq([ undefined, null ])).toEqual([ undefined, null ])
})
test('can distinct between string and number', () => {
expect(uniq([ 1, '1' ])).toEqual([ 1, '1' ])
})
import {uniq} from 'rambda'
describe('R.uniq', () => {
it('happy', () => {
const result = uniq([1, 2, 3, 3, 3, 1, 2, 0])
result // $ExpectType number[]
})
})
It applies uniqueness to input list based on function that defines what to be used for comparison between elements.
R.equals
is used to determine equality.
Try this R.uniqBy example in Rambda REPL
uniqWith<T, U>(predicate: (x: T, y: T) => boolean, list: T[]): T[]
It returns a new array containing only one copy of each element in list
according to predicate
function.
This predicate should return true, if two elements are equal.
Try this R.uniqWith example in Rambda REPL
uniqWith<T, U>(predicate: (x: T, y: T) => boolean, list: T[]): T[];
uniqWith<T, U>(predicate: (x: T, y: T) => boolean): (list: T[]) => T[];
function includesWith(
predicate, target, list
){
let willReturn = false
let index = -1
while (++index < list.length && !willReturn){
const value = list[ index ]
if (predicate(target, value)){
willReturn = true
}
}
return willReturn
}
export function uniqWith(predicate, list){
if (arguments.length === 1) return _list => uniqWith(predicate, _list)
let index = -1
const willReturn = []
while (++index < list.length){
const value = list[ index ]
if (!includesWith(
predicate, value, willReturn
)){
willReturn.push(value)
}
}
return willReturn
}
import { uniqWith as uniqWithRamda } from 'ramda'
import { uniqWith } from './uniqWith.js'
const list = [ { a : 1 }, { a : 1 } ]
test('happy', () => {
const fn = (x, y) => x.a === y.a
const result = uniqWith(fn, list)
expect(result).toEqual([ { a : 1 } ])
})
test('with list of strings', () => {
const fn = (x, y) => x.length === y.length
const list = [ '0', '11', '222', '33', '4', '55' ]
const result = uniqWith(fn)(list)
const resultRamda = uniqWithRamda(fn, list)
expect(result).toEqual([ '0', '11', '222' ])
expect(resultRamda).toEqual([ '0', '11', '222' ])
})
import {uniqWith} from 'rambda'
describe('R.uniqWith', () => {
it('happy', () => {
const list = [{a: 1}, {a: 1}]
const fn = (x: any, y: any) => x.a === y.a
const result = uniqWith(fn, list)
result // $ExpectType { a: number; }[]
})
})
unless<T, U>(predicate: (x: T) => boolean, whenFalseFn: (x: T) => U, x: T): T | U
The method returns function that will be called with argument input
.
If predicate(input)
returns false
, then the end result will be the outcome of whenFalse(input)
.
In the other case, the final output will be the input
itself.
Try this R.unless example in Rambda REPL
unless<T, U>(predicate: (x: T) => boolean, whenFalseFn: (x: T) => U, x: T): T | U;
unless<T, U>(predicate: (x: T) => boolean, whenFalseFn: (x: T) => U): (x: T) => T | U;
unless<T>(predicate: (x: T) => boolean, whenFalseFn: (x: T) => T, x: T): T;
unless<T>(predicate: (x: T) => boolean, whenFalseFn: (x: T) => T): (x: T) => T;
export function unless(predicate, whenFalse){
if (arguments.length === 1){
return _whenFalse => unless(predicate, _whenFalse)
}
return input => predicate(input) ? input : whenFalse(input)
}
import { inc } from './inc.js'
import { isNil } from './isNil.js'
import { unless } from './unless.js'
test('happy', () => {
const safeInc = unless(isNil, inc)
expect(safeInc(null)).toBeNull()
expect(safeInc(1)).toBe(2)
})
test('curried', () => {
const safeIncCurried = unless(isNil)(inc)
expect(safeIncCurried(null)).toBeNull()
})
import {unless, inc} from 'rambda'
describe('R.unless', () => {
it('happy', () => {
const fn = unless(x => x > 5, inc)
const result = fn(1)
result // $ExpectType number
})
it('with one explicit type', () => {
const result = unless(
x => {
x // $ExpectType number
return x > 5
},
x => {
x // $ExpectType number
return x + 1
},
1
)
result // $ExpectType number
})
it('with two different explicit types', () => {
const result = unless(
x => {
x // $ExpectType number
return x > 5
},
x => {
x // $ExpectType number
return `${x}-foo`
},
1
)
result // $ExpectType string | number
})
})
describe('R.unless - curried', () => {
it('happy', () => {
const fn = unless(x => x > 5, inc)
const result = fn(1)
result // $ExpectType number
})
it('with one explicit type', () => {
const fn = unless<number>(
x => {
x // $ExpectType number
return x > 5
},
x => {
x // $ExpectType number
return x + 1
}
)
const result = fn(1)
result // $ExpectType number
})
it('with two different explicit types', () => {
const fn = unless<number, string>(
x => {
x // $ExpectType number
return x > 5
},
x => {
x // $ExpectType number
return `${x}-foo`
}
)
const result = fn(1)
result // $ExpectType string | number
})
})
Try this R.unnest example in Rambda REPL
Try this R.unwind example in Rambda REPL
update<T>(index: number, newValue: T, list: T[]): T[]
It returns a copy of list
with updated element at index
with newValue
.
Try this R.update example in Rambda REPL
update<T>(index: number, newValue: T, list: T[]): T[];
update<T>(index: number, newValue: T): (list: T[]) => T[];
import { cloneList } from './_internals/cloneList.js'
import { curry } from './curry.js'
export function updateFn(
index, newValue, list
){
const clone = cloneList(list)
if (index === -1) return clone.fill(newValue, index)
return clone.fill(
newValue, index, index + 1
)
}
export const update = curry(updateFn)
import { update } from './update.js'
const list = [ 1, 2, 3 ]
test('happy', () => {
const newValue = 8
const index = 1
const result = update(
index, newValue, list
)
const curriedResult = update(index, newValue)(list)
const tripleCurriedResult = update(index)(newValue)(list)
const expected = [ 1, 8, 3 ]
expect(result).toEqual(expected)
expect(curriedResult).toEqual(expected)
expect(tripleCurriedResult).toEqual(expected)
})
test('list has no such index', () => {
const newValue = 8
const index = 10
const result = update(
index, newValue, list
)
expect(result).toEqual(list)
})
test('with negative index', () => {
expect(update(
-1, 10, [ 1 ]
)).toEqual([ 10 ])
expect(update(
-1, 10, []
)).toEqual([])
expect(update(
-1, 10, list
)).toEqual([ 1, 2, 10 ])
expect(update(
-2, 10, list
)).toEqual([ 1, 10, 3 ])
expect(update(
-3, 10, list
)).toEqual([ 10, 2, 3 ])
})
import {update} from 'rambda'
describe('R.update', () => {
it('happy', () => {
const result = update(1, 0, [1, 2, 3])
result // $ExpectType number[]
})
})
values<T extends object, K extends keyof T>(obj: T): T[K][]
With correct input, this is nothing more than Object.values(Record<string, unknown>)
. If obj
is not an object, then it returns an empty array.
Try this R.values example in Rambda REPL
values<T extends object, K extends keyof T>(obj: T): T[K][];
import { type } from './type.js'
export function values(obj){
if (type(obj) !== 'Object') return []
return Object.values(obj)
}
import { values } from './values.js'
test('happy', () => {
expect(values({
a : 1,
b : 2,
c : 3,
})).toEqual([ 1, 2, 3 ])
})
test('with bad input', () => {
expect(values(null)).toEqual([])
expect(values(undefined)).toEqual([])
expect(values(55)).toEqual([])
expect(values('foo')).toEqual([])
expect(values(true)).toEqual([])
expect(values(false)).toEqual([])
expect(values(NaN)).toEqual([])
expect(values(Infinity)).toEqual([])
expect(values([])).toEqual([])
})
import {values} from 'rambda'
describe('R.values', () => {
it('happy', () => {
const result = values({
a: 1,
b: 2,
c: 3,
})
result // $ExpectType number[]
})
})
view<S, A>(lens: Lens<S, A>): (obj: S) => A
It returns the value of lens
focus over target
object.
Try this R.view example in Rambda REPL
view<S, A>(lens: Lens<S, A>): (obj: S) => A;
view<S, A>(lens: Lens<S, A>, obj: S): A;
const Const = x => ({
x,
map : fn => Const(x),
})
export function view(lens, target){
if (arguments.length === 1) return _target => view(lens, _target)
return lens(Const)(target).x
}
import { assoc } from './assoc.js'
import { lens } from './lens.js'
import { prop } from './prop.js'
import { view } from './view.js'
const testObject = { foo : 'Led Zeppelin' }
const assocLens = lens(prop('foo'), assoc('foo'))
test('happy', () => {
expect(view(assocLens, testObject)).toBe('Led Zeppelin')
})
when<T, U>(predicate: (x: T) => boolean, whenTrueFn: (a: T) => U, input: T): T | U
It pass input
to predicate
function and if the result is true
, it will return the result of whenTrueFn(input)
.
If the predicate
returns false
, then it will simply return input
.
Try this R.when example in Rambda REPL
when<T, U>(predicate: (x: T) => boolean, whenTrueFn: (a: T) => U, input: T): T | U;
when<T, U>(predicate: (x: T) => boolean, whenTrueFn: (a: T) => U): (input: T) => T | U;
when<T, U>(predicate: (x: T) => boolean): ((whenTrueFn: (a: T) => U) => (input: T) => T | U);
import { curry } from './curry.js'
function whenFn(
predicate, whenTrueFn, input
){
if (!predicate(input)) return input
return whenTrueFn(input)
}
export const when = curry(whenFn)
import { add } from './add.js'
import { when } from './when.js'
const predicate = x => typeof x === 'number'
test('happy', () => {
const fn = when(predicate, add(11))
expect(fn(11)).toBe(22)
expect(fn('foo')).toBe('foo')
})
import {when} from 'rambda'
const predicate = (x: number) => x > 2
const whenTrueFn = (x: number) => String(x)
describe('R.when', () => {
it('happy', () => {
const result = when(predicate, whenTrueFn, 1)
result // $ExpectType string | 1
})
it('curry 1', () => {
const fn = when(predicate, whenTrueFn)
const result = fn(1)
result // $ExpectType string | number
})
it('curry 2 require explicit types', () => {
const fn = when<number, string>(predicate)(whenTrueFn)
const result = fn(1)
result // $ExpectType string | number
})
})
where<T, U>(conditions: T, input: U): boolean
It returns true
if all each property in conditions
returns true
when applied to corresponding property in input
object.
Try this R.where example in Rambda REPL
where<T, U>(conditions: T, input: U): boolean;
where<T>(conditions: T): <U>(input: U) => boolean;
where<ObjFunc2, U>(conditions: ObjFunc2, input: U): boolean;
where<ObjFunc2>(conditions: ObjFunc2): <U>(input: U) => boolean;
export function where(conditions, input){
if (input === undefined){
return _input => where(conditions, _input)
}
let flag = true
for (const prop in conditions){
if (!flag) continue
const result = conditions[ prop ](input[ prop ])
if (flag && result === false){
flag = false
}
}
return flag
}
import { equals } from './equals.js'
import { where } from './where.js'
test('when true', () => {
const result = where({
a : equals('foo'),
b : equals('bar'),
},
{
a : 'foo',
b : 'bar',
x : 11,
y : 19,
})
expect(result).toBeTrue()
})
test('when false | early exit', () => {
let counter = 0
const equalsFn = expected => input => {
console.log(expected, 'expected')
counter++
return input === expected
}
const predicate = where({
a : equalsFn('foo'),
b : equalsFn('baz'),
})
expect(predicate({
a : 'notfoo',
b : 'notbar',
})).toBeFalse()
expect(counter).toBe(1)
})
import {where, equals} from 'rambda'
describe('R.where', () => {
it('happy', () => {
const input = {
a: 'foo',
b: 'bar',
x: 11,
y: 19,
}
const conditions = {
a: equals('foo'),
b: equals('bar'),
}
const result = where(conditions, input)
const curriedResult = where(conditions)(input)
result // $ExpectType boolean
curriedResult // $ExpectType boolean
})
})
Same as R.where
, but it will return true
if at least one condition check returns true
.
Try this R.whereAny example in Rambda REPL
whereEq<T, U>(condition: T, input: U): boolean
It will return true
if all of input
object fully or partially include rule
object.
R.equals
is used to determine equality.
Try this R.whereEq example in Rambda REPL
whereEq<T, U>(condition: T, input: U): boolean;
whereEq<T>(condition: T): <U>(input: U) => boolean;
import { equals } from './equals.js'
import { filter } from './filter.js'
export function whereEq(condition, input){
if (arguments.length === 1){
return _input => whereEq(condition, _input)
}
const result = filter((conditionValue, conditionProp) =>
equals(conditionValue, input[ conditionProp ]),
condition)
return Object.keys(result).length === Object.keys(condition).length
}
import { whereEq } from './whereEq.js'
test('when true', () => {
const condition = { a : 1 }
const input = {
a : 1,
b : 2,
}
const result = whereEq(condition, input)
const expectedResult = true
expect(result).toEqual(expectedResult)
})
test('when false', () => {
const condition = { a : 1 }
const input = { b : 2 }
const result = whereEq(condition, input)
const expectedResult = false
expect(result).toEqual(expectedResult)
})
test('with nested object', () => {
const condition = { a : { b : 1 } }
const input = {
a : { b : 1 },
c : 2,
}
const result = whereEq(condition)(input)
const expectedResult = true
expect(result).toEqual(expectedResult)
})
test('with wrong input', () => {
const condition = { a : { b : 1 } }
expect(() => whereEq(condition, null)).toThrowErrorMatchingInlineSnapshot('"Cannot read properties of null (reading \'a\')"')
})
import {whereEq} from 'rambda'
describe('R.whereEq', () => {
it('happy', () => {
const result = whereEq({a: {b: 2}}, {b: 2})
const curriedResult = whereEq({a: {b: 2}})({b: 2})
result // $ExpectType boolean
curriedResult // $ExpectType boolean
})
})
without<T>(matchAgainst: T[], source: T[]): T[]
It will return a new array, based on all members of source
list that are not part of matchAgainst
list.
R.equals
is used to determine equality.
Try this R.without example in Rambda REPL
without<T>(matchAgainst: T[], source: T[]): T[];
without<T>(matchAgainst: T[]): (source: T[]) => T[];
import { _indexOf } from './equals.js'
import { reduce } from './reduce.js'
export function without(matchAgainst, source){
if (source === undefined){
return _source => without(matchAgainst, _source)
}
return reduce(
(prev, current) =>
_indexOf(current, matchAgainst) > -1 ? prev : prev.concat(current),
[],
source
)
}
import { without as withoutRamda } from 'ramda'
import { without } from './without.js'
test('should return a new list without values in the first argument', () => {
const itemsToOmit = [ 'A', 'B', 'C' ]
const collection = [ 'A', 'B', 'C', 'D', 'E', 'F' ]
expect(without(itemsToOmit, collection)).toEqual([ 'D', 'E', 'F' ])
expect(without(itemsToOmit)(collection)).toEqual([ 'D', 'E', 'F' ])
})
test('with list of objects', () => {
const itemsToOmit = [ { a : 1 }, { c : 3 } ]
const collection = [ { a : 1 }, { b : 2 }, { c : 3 }, { d : 4 } ]
const expected = [ { b : 2 }, { d : 4 } ]
expect(without(itemsToOmit, collection)).toEqual(expected)
expect(withoutRamda(itemsToOmit, collection)).toEqual(expected)
})
test('ramda accepts string as target input while rambda throws', () => {
expect(withoutRamda('0:1', [ '0', '0:1' ])).toEqual([ '0:1' ])
expect(() =>
without('0:1', [ '0', '0:1' ])).toThrowErrorMatchingInlineSnapshot('"Cannot read property \'indexOf\' of 0:1"')
expect(without([ '0:1' ], [ '0', '0:1' ])).toEqual([ '0' ])
})
test('ramda test', () => {
expect(without([ 1, 2 ])([ 1, 2, 1, 3, 4 ])).toEqual([ 3, 4 ])
})
import {without} from 'rambda'
const itemsToOmit = ['A', 'B', 'C']
const collection = ['A', 'B', 'C', 'D', 'E', 'F']
describe('R.without', () => {
it('happy', () => {
const result = without(itemsToOmit, collection)
result // $ExpectType string[]
})
it('curried', () => {
const result = without(itemsToOmit)(collection)
result // $ExpectType string[]
})
})
xor(x: boolean, y: boolean): boolean
Logical XOR
Try this R.xor example in Rambda REPL
xor(x: boolean, y: boolean): boolean;
xor(y: boolean): (y: boolean) => boolean;
export function xor(a, b){
if (arguments.length === 1) return _b => xor(a, _b)
return Boolean(a) && !b || Boolean(b) && !a
}
import { xor } from './xor.js'
test('compares two values with exclusive or', () => {
expect(xor(true, true)).toBeFalse()
expect(xor(true, false)).toBeTrue()
expect(xor(false, true)).toBeTrue()
expect(xor(false, false)).toBeFalse()
})
test('when both values are truthy, it should return false', () => {
expect(xor(true, 'foo')).toBeFalse()
expect(xor(42, true)).toBeFalse()
expect(xor('foo', 42)).toBeFalse()
expect(xor({}, true)).toBeFalse()
expect(xor(true, [])).toBeFalse()
expect(xor([], {})).toBeFalse()
expect(xor(new Date(), true)).toBeFalse()
expect(xor(true, Infinity)).toBeFalse()
expect(xor(Infinity, new Date())).toBeFalse()
})
test('when both values are falsy, it should return false', () => {
expect(xor(null, false)).toBeFalse()
expect(xor(false, undefined)).toBeFalse()
expect(xor(undefined, null)).toBeFalse()
expect(xor(0, false)).toBeFalse()
expect(xor(false, NaN)).toBeFalse()
expect(xor(NaN, 0)).toBeFalse()
expect(xor('', false)).toBeFalse()
})
test('when one argument is truthy and the other is falsy, it should return true', () => {
expect(xor('foo', null)).toBeTrue()
expect(xor(null, 'foo')).toBeTrue()
expect(xor(undefined, 42)).toBeTrue()
expect(xor(42, undefined)).toBeTrue()
expect(xor(Infinity, NaN)).toBeTrue()
expect(xor(NaN, Infinity)).toBeTrue()
expect(xor({}, '')).toBeTrue()
expect(xor('', {})).toBeTrue()
expect(xor(new Date(), 0)).toBeTrue()
expect(xor(0, new Date())).toBeTrue()
expect(xor([], null)).toBeTrue()
expect(xor(undefined, [])).toBeTrue()
})
import {xor} from 'rambda'
describe('R.xor', () => {
it('happy', () => {
xor(true, false) // $ExpectType boolean
})
it('curry', () => {
xor(true)(false) // $ExpectType boolean
})
})
zip<K, V>(x: K[], y: V[]): KeyValuePair<K, V>[]
It will return a new array containing tuples of equally positions items from both x
and y
lists.
The returned list will be truncated to match the length of the shortest supplied list.
Try this R.zip example in Rambda REPL
zip<K, V>(x: K[], y: V[]): KeyValuePair<K, V>[];
zip<K>(x: K[]): <V>(y: V[]) => KeyValuePair<K, V>[];
export function zip(left, right){
if (arguments.length === 1) return _right => zip(left, _right)
const result = []
const length = Math.min(left.length, right.length)
for (let i = 0; i < length; i++){
result[ i ] = [ left[ i ], right[ i ] ]
}
return result
}
import { zip } from './zip.js'
const array1 = [ 1, 2, 3 ]
const array2 = [ 'A', 'B', 'C' ]
test('should return an array', () => {
const actual = zip(array1)(array2)
expect(actual).toBeInstanceOf(Array)
})
test('should return and array or tuples', () => {
const expected = [
[ 1, 'A' ],
[ 2, 'B' ],
[ 3, 'C' ],
]
const actual = zip(array1, array2)
expect(actual).toEqual(expected)
})
test('should truncate result to length of shorted input list', () => {
const expectedA = [
[ 1, 'A' ],
[ 2, 'B' ],
]
const actualA = zip([ 1, 2 ], array2)
expect(actualA).toEqual(expectedA)
const expectedB = [
[ 1, 'A' ],
[ 2, 'B' ],
]
const actualB = zip(array1, [ 'A', 'B' ])
expect(actualB).toEqual(expectedB)
})
import {zip} from 'rambda'
describe('R.zip', () => {
it('happy', () => {
const array1 = [1, 2, 3]
const array2 = ['A', 'B', 'C']
const result = zip(array1)(array2)
result // $ExpectType KeyValuePair<number, string>[]
})
})
zipObj<T, K extends string>(keys: K[], values: T[]): { [P in K]: T }
It will return a new object with keys of keys
array and values of values
array.
Try this R.zipObj example in Rambda REPL
zipObj<T, K extends string>(keys: K[], values: T[]): { [P in K]: T };
zipObj<K extends string>(keys: K[]): <T>(values: T[]) => { [P in K]: T };
zipObj<T, K extends number>(keys: K[], values: T[]): { [P in K]: T };
zipObj<K extends number>(keys: K[]): <T>(values: T[]) => { [P in K]: T };
import { take } from './take.js'
export function zipObj(keys, values){
if (arguments.length === 1) return yHolder => zipObj(keys, yHolder)
return take(values.length, keys).reduce((
prev, xInstance, i
) => {
prev[ xInstance ] = values[ i ]
return prev
}, {})
}
import { equals } from './equals.js'
import { zipObj } from './zipObj.js'
test('zipObj', () => {
expect(zipObj([ 'a', 'b', 'c' ], [ 1, 2, 3 ])).toEqual({
a : 1,
b : 2,
c : 3,
})
})
test('0', () => {
expect(zipObj([ 'a', 'b' ])([ 1, 2, 3 ])).toEqual({
a : 1,
b : 2,
})
})
test('1', () => {
expect(zipObj([ 'a', 'b', 'c' ])([ 1, 2 ])).toEqual({
a : 1,
b : 2,
})
})
test('ignore extra keys', () => {
const result = zipObj([ 'a', 'b', 'c', 'd', 'e', 'f' ], [ 1, 2, 3 ])
const expected = {
a : 1,
b : 2,
c : 3,
}
expect(equals(result, expected)).toBeTrue()
})
import {zipObj} from 'rambda'
describe('R.zipObj', () => {
it('happy', () => {
// this is wrong since 24.10.2020 `@types/ramda` changes
const result = zipObj(['a', 'b', 'c', 'd'], [1, 2, 3])
;[result.a, result.b, result.c, result.d] // $ExpectType number[]
})
it('imported from @types/ramda', () => {
const result = zipObj(['a', 'b', 'c'], [1, 2, 3])
const curriedResult = zipObj(['a', 'b', 'c'])([1, 2, 3])
;[result.a, result.b, result.c] // $ExpectType number[]
;[curriedResult.a, curriedResult.b, curriedResult.c] // $ExpectType number[]
})
})
zipWith<T, U, TResult>(fn: (x: T, y: U) => TResult, list1: T[], list2: U[]): TResult[]
Try this R.zipWith example in Rambda REPL
zipWith<T, U, TResult>(fn: (x: T, y: U) => TResult, list1: T[], list2: U[]): TResult[];
zipWith<T, U, TResult>(fn: (x: T, y: U) => TResult, list1: T[]): (list2: U[]) => TResult[];
zipWith<T, U, TResult>(fn: (x: T, y: U) => TResult): (list1: T[], list2: U[]) => TResult[];
import { curry } from './curry.js'
import { take } from './take.js'
function zipWithFn(
fn, x, y
){
return take(x.length > y.length ? y.length : x.length, x).map((xInstance, i) => fn(xInstance, y[ i ]))
}
export const zipWith = curry(zipWithFn)
import { add } from './add.js'
import { zipWith } from './zipWith.js'
const list1 = [ 1, 2, 3 ]
const list2 = [ 10, 20, 30, 40 ]
const list3 = [ 100, 200 ]
test('when second list is shorter', () => {
const result = zipWith(
add, list1, list3
)
expect(result).toEqual([ 101, 202 ])
})
test('when second list is longer', () => {
const result = zipWith(
add, list1, list2
)
expect(result).toEqual([ 11, 22, 33 ])
})
import {zipWith} from 'rambda'
const list1 = [1, 2]
const list2 = [10, 20, 30]
describe('R.zipWith', () => {
it('happy', () => {
const result = zipWith(
(x, y) => {
x // $ExpectType number
y // $ExpectType number
return `${x}-${y}`
},
list1,
list2
)
result // $ExpectType string[]
})
it('curried', () => {
const result = zipWith((x, y) => {
x // $ExpectType unknown
y // $ExpectType unknown
return `${x}-${y}`
})(list1, list2)
result // $ExpectType string[]
})
})
9.1.0
Add these methods
9.0.1
9.0.0
Breaking change in TS definitions of lenses
as now they are synced to Ramda
types.
Add R.sortWith
- Issue #707
Add R.innerJoin
, R.gt
, R.gte
, R.reduceBy
, R.hasIn
8.6.0
Wrong typing for R.dissocPath
- Issue #709
Update build dependencies
8.5.0
Revert changes in R.anyPass
introduced in 8.4.0
release. The reason is that the change was breaking the library older than 5.2.0
TypeScript.
Wrong R.partial
TS definition - Issue #705
Add R.dropRepeatsBy
Add R.empty
Add R.eqBy
Add R.forEachObjIndexed
8.4.0
Add R.dissocPath
Fix TS definitions of R.head/R.last
and add missing handle of empty string
Add R.removeIndex
- method was before only in Rambdax
, but now since R.dissocPath
is using it, it is added to main library.
Allow R.omit
to pass numbers as part of properties to omit, i.e. R.omit(['a', 1], {a: {1: 1, 2: 2}})
R.keys always returns strings - MR #700
Improve R.prepend/R.append
type interference - MR #699
Change R.reduce
TS definitions so index is always received - MR #696
Functions as a type guard in R.anyPass
TS definitions - MR #695
Fix R.append's curried type - MR #694
Fix cannot compare errors in Deno
with R.equals
- Issue #704.
Fix cannot compare BigInt
with R.equals
8.3.0
Add the following methods:
8.2.0
Add the following methods:
8.1.0
Fix input order of TS definitions for R.propEq
method - Issue #688. The issue was due to 8.0.0 was shipped with TS definitions of 7.5.0
release.
Add R.differenceWith
method - Issue #91
8.0.0
handle falsy values in merge methods - https://github.com/ramda/ramda/pull/3222
R.head
/R.last
don't return undefined
for non-empty arrays
R.type
supports dates in TS definition - Rambda
already did support dates in JS.
Improve typings of R.endsWith/startsWith
with regard to string
input. - PR #622
Handle list as falsy value in R.reduce
- Ramda MR
R.nop
is removed - it will be moved to Rambdax
as R.noop
R.includes
is no longer using string literal in TypeScript definitions
Reason for breaking change - synchronize with Ramda
0.29.0
release:
R.propEq
- Ramda MR7.5.0
IMPORTANT: Remove export
property in package.json
in order to allow Rambda
support for projects with "type": "module"
in package.json
- Issue #667
Add R.unnest
- Rambdax issue 89
R.uniq
is not using R.equals
as Ramda does - Issue #88
Fix R.path(['non','existing','path'], obj)
TS definition as 7.4.0 release caused TS errors - Issue #668
7.4.0
Synchronize with @types/ramda
- R.prop
, R.path
, R.pickAll
Remove esm
Rollup output due to tree-shaking issues.
Upgrade all dev dependencies.
7.3.0
Important - changing import declaration in package.json
in order to fix tree-shaking issue - Issue #647
Add R.modify
Allow multiple inputs in TypeScript versions of R.anyPass
and R.allPass
- Issue #642
Using wrong clone of object in R.mergeDeepRight
- Issue #650
Missing early return in R.where
- Issue #648
R.allPass
doesn't accept more than 1 parameters for function predicates- Issue #604
7.2.1
Remove bad typings of R.propIs
which caused the library to cannot be build with TypeScript.
Drop support for Wallaby
as per https://github.com/wallabyjs/public/issues/3037
7.2.0
Wrong R.update
if index is -1
- PR #593
Wrong curried typings in R.anyPass
- Issue #642
R.modifyPath
not exported - Issue #640
Add new method R.uniqBy
. Implementation is coming from Ramda MR#2641
Apply the following changes from @types/rambda
:
-- [https://github.com/DefinitelyTyped/DefinitelyTyped/commit/bab47272d52fc7bb81e85da36dbe9c905a04d067](add AnyFunction
and AnyConstructor
)
-- Improve R.ifElse
typings - https://github.com/DefinitelyTyped/DefinitelyTyped/pull/59291
-- Make R.propEq
safe for null/undefined
arguments - https://github.com/ramda/ramda/pull/2594/files
7.1.4
R.mergeRight
not found on Deno
import - Issue #6337.1.0
Add R.mergeRight
- introduced by Ramda's latest release. While Ramda renames R.merge
, Rambda will keep R.merge
.
Rambda's pipe/compose
doesn't return proper length of composed function which leads to issue with R.applySpec
. It was fixed by using Ramda's pipe/compose
logic - Issue #627
Replace Async
with Promise
as return type of R.type
.
Add new types as TypeScript output for R.type
- "Map", "WeakMap", "Generator", "GeneratorFunction", "BigInt", "ArrayBuffer"
Add R.juxt
method
Add R.propSatisfies
method
Add new methods after Ramda
version upgrade to 0.28.0
:
-- R.count -- R.modifyPath -- R.on -- R.whereAny -- R.partialObject
7.0.3
Rambda.none has wrong logic introduced in version 7.0.0
- Issue #625
7.0.2
Rambda doesn't work with pnpm
due to wrong export configuration - Issue #619
7.0.1
package.json
- Issue #6147.0.0
R.compose
/R.pipe
with @types/ramda
. That is significant change so as safeguard, it will lead a major bump. Important - this lead to raising required TypeScript version to 4.2.2
. In other words, to use Rambda
you'll need TypeScript version 4.2.2
or newer.Related commit in @types/ramda
- https://github.com/DefinitelyTyped/DefinitelyTyped/commit/286eff4f76d41eb8f091e7437eabd8a60d97fc1f#diff-4f74803fa83a81e47cb17a7d8a4e46a7e451f4d9e5ce2f1bd7a70a72d91f4bc1
There are several other changes in @types/ramda
as stated in this comment. This leads to change of typings for the following methods in Rambda:
-- R.unless
-- R.toString
-- R.ifElse
-- R.always
-- R.complement
-- R.cond
-- R.is
-- R.sortBy
-- R.dissoc
-- R.toPairs
-- R.assoc
-- R.toLower
-- R.toUpper
One more reason for the breaking change is changing of export declarations in package.json
based on this blog post and this merged Ramda's PR. This also led to renaming of babel.config.js
to babel.config.cjs
.
Add R.apply
, R.bind
and R.unapply
R.startsWith/R.endsWith
now support lists as inputs. This way, it matches current Ramda behavior.
Remove unused typing for R.chain
.
R.map
/R.filter
no longer accept bad inputs as iterable. This way, Rambda behaves more like Ramda, which also throws.
Make R.lastIndexOf
follow the logic of R.indexOf
.
Change R.type
logic to Ramda logic. This way, R.type
can return Error
and Set
as results.
Add missing logic in R.equals
to compare sets - Issue #599
Improve list cloning - Issue #595
Handle multiple inputs with R.allPass
and R.anyPass
- Issue #604
Fix R.length
wrong logic with inputs as {length: 123}
- Issue #606.
Improve non-curry typings of R.merge
by using types from mobily/ts-belt.
Improve performance of R.uniqWith
.
Wrong R.update
if index is -1
- PR #593
Make R.eqProps
safe for falsy inputs - based on this opened Ramda PR.
Incorrect benchmarks for R.pipe/R.compose
- Issue #608
Fix R.last/R.head
typings - Issue #609
6.9.0
R.uniq
methods - Issue #581Fixing R.uniq
was done by improving R.indexOf
which has performance implication to all methods importing R.indexOf
:
R.includes
R.intersection
R.difference
R.excludes
R.symmetricDifference
R.union
R.without no longer support the following case - without('0:1', ['0', '0:1']) // => ['0']
. Now it throws as the first argument should be a list, not a string. Ramda, on the other hand, returns an empty list - https://github.com/ramda/ramda/issues/3086.
6.8.3
Fix TypeScript build process with rambda/immutable
- Issue #572
Add R.objOf
method
Add R.mapObjIndexed
method
Publish shorter README.md version to NPM
6.8.0
R.has
use Object.prototype.hasOwnProperty
- Issue #572
Expose immutable.ts
typings which are Rambda typings with readonly
statements - Issue #565
Fix R.intersection
wrong order compared to Ramda.
R.path
wrong return of null
instead of undefined
when path value is null
- PR #577
6.7.0
ts-toolbelt
types from TypeScript definitions. Most affected are the following methods, which lose one of its curried definitions:6.6.0
Change R.piped
typings to mimic that of R.pipe
. Main difference is that R.pipe
is focused on unary functions.
Fix wrong logic when R.without
use R.includes
while it should use array version of R.includes
.
Use uglify plugin for UMD bundle.
Remove dist
folder from .gitignore
in order to fix Deno
broken package. Issue #570
Improve R.fromPairs
typings - Issue #567
6.5.3
R.without
use R.includes
while it should use the array version of R.includes
This is Ramda bug, that Rambda also has before this release - https://github.com/ramda/ramda/issues/3086
6.5.2
Wrong R.defaultTo
typings - changes introduced in v6.5.0 are missing their TS equivalent.
Update dependencies
6.5.1
Fix wrong versions in changelog
6.5.0
R.defaultTo
no longer accepts infinite inputs, thus it follows Ramda implementation.
R.equals
supports equality of functions.
R.pipe
doesn't use R.compose
.
Close Issue #561 - export several internal TS interfaces and types
Close Issue #559 - improve R.propOr
typings
Add CHANGELOG.md
file in release files list
This is only part of the changelog. You can read the full text in CHANGELOG.md file.
Most influential contributors(in alphabetical order)
@farwayer - improving performance in R.find, R.filter; give the idea how to make benchmarks more reliable;
@thejohnfreeman - add R.assoc, R.chain;
@peeja - add several methods and fix mutiple issues; provides great MR documentation
@helmuthdu - add R.clone; help improve code style;
@jpgorman - add R.zip, R.reject, R.without, R.addIndex;
@ku8ar - add R.slice, R.propOr, R.identical, R.propIs and several math related methods; introduce the idea to display missing Ramda methods;
@romgrk - add R.groupBy, R.indexBy, R.findLast, R.findLastIndex;
@squidfunk - add R.assocPath, R.symmetricDifference, R.difference, R.intersperse;
@synthet1c - add all lenses methods; add R.applySpec, R.converge;
@vlad-zhukov - help with configuring Rollup, Babel; change export file to use ES module exports;
Rambda references
Links to Rambda
[ https://mailchi.mp/webtoolsweekly/web-tools-280 ]( Web Tools Weekly #280 )
Deprecated from
Used by
section
Rambda
since October/2020 commit that removes Rambda
Niketa themeCollection of 9 light VSCode themes |
Niketa dark themeCollection of 9 dark VSCode themes |
String-fnString utility library |
Useful Javascript librariesLarge collection of JavaScript,TypeScript and Angular related repos links |
Run-fnCLI commands for lint JS/TS files, commit git changes and upgrade of dependencies |
FAQs
Lightweight and faster alternative to Ramda with included TS definitions
The npm package rambda receives a total of 914,797 weekly downloads. As such, rambda popularity was classified as popular.
We found that rambda demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.
Security News
License exceptions modify the terms of open source licenses, impacting how software can be used, modified, and distributed. Developers should be aware of the legal implications of these exceptions.
Security News
A developer is accusing Tencent of violating the GPL by modifying a Python utility and changing its license to BSD, highlighting the importance of copyleft compliance.