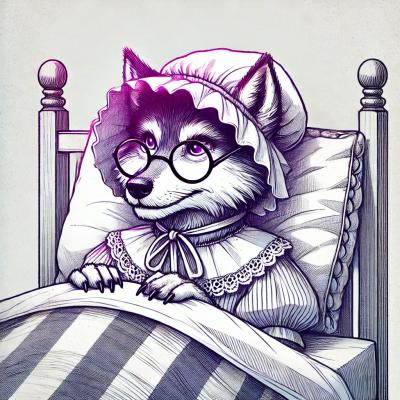
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
random-access-file
Advanced tools
Continuous reading or writing to a file using random offsets and lengths
Continuous reading or writing to a file using random offsets and lengths
npm install random-access-file
If you are receiving a file in multiple pieces in a distributed system it can be useful to write these pieces to disk one by one in various places throughout the file without having to open and close a file descriptor all the time.
random-access-file allows you to do just this.
var randomAccessFile = require('random-access-file')
var file = randomAccessFile('my-file.txt')
file.write(10, new Buffer('hello'), function(err) {
// write a buffer to offset 10
file.read(10, 5, function(err, buffer) {
console.log(buffer) // read 5 bytes from offset 10
file.close(function() {
console.log('file is closed')
})
})
})
file will use an open file descriptor. When you are done with the file you should call file.close()
.
var file = randomAccessFile(filename, [options])
Create a new file. Options include:
{
truncate: false, // truncate the file before reading / writing
length: someLength, // truncate the file to this size first
readable: true, // should the file be opened as readable?
writable: true // should the file be opened as writable?
}
file.write(offset, buffer, [callback])
Write a buffer at a specific offset.
file.read(offset, length, callback)
Read a buffer at a specific offset. Callback is called with the buffer read.
file.del(offset, length, callback)
Will truncate the file if offset + length is larger than the current file length. Is otherwise a noop.
file.end([options], callback)
Call this method when the entire file has been written. Options include:
{
mtime: mtime, // set the file's mtime
atime: atime // set the file's atime
}
file.close([callback])
Close the underlying file descriptor.
file.unlink([callback])
Unlink the underlying file.
file.on('open')
Emitted when the file descriptor has been opened. You can access the fd using file.fd
.
You do not need to wait for this event before doing any reads/writes.
file.on('close')
Emitted when the file has been closed.
MIT
FAQs
Continuous reading or writing to a file using random offsets and lengths
The npm package random-access-file receives a total of 1,290 weekly downloads. As such, random-access-file popularity was classified as popular.
We found that random-access-file demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.