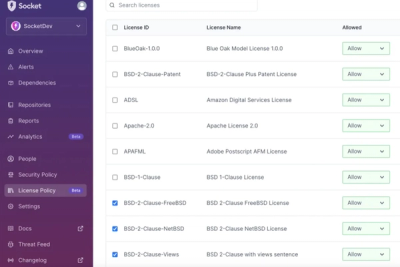
Product
Introducing License Enforcement in Socket
Ensure open-source compliance with Socket’s License Enforcement Beta. Set up your License Policy and secure your software!
rate-limit-redis
Advanced tools
Provides a Redis store for the express-rate-limit RateLimit middleware.
The rate-limit-redis npm package is a Redis-backed store for the express-rate-limit middleware. It allows you to implement rate limiting in your Express applications using Redis as the storage backend. This is particularly useful for distributed applications where you need to share rate limit data across multiple instances.
Basic Rate Limiting
This code demonstrates how to set up basic rate limiting in an Express application using Redis as the storage backend. The rate limiter is configured to allow 100 requests per 15 minutes per IP address.
const rateLimit = require('express-rate-limit');
const RedisStore = require('rate-limit-redis');
const redisClient = require('redis').createClient();
const limiter = rateLimit({
store: new RedisStore({
client: redisClient
}),
max: 100, // limit each IP to 100 requests per windowMs
windowMs: 15 * 60 * 1000 // 15 minutes
});
const express = require('express');
const app = express();
app.use(limiter);
app.get('/', (req, res) => {
res.send('Hello, world!');
});
app.listen(3000, () => {
console.log('Server running on port 3000');
});
Custom Redis Client
This example shows how to use a custom Redis client configuration with the rate-limit-redis package. This is useful if you need to connect to a Redis instance with specific connection settings.
const rateLimit = require('express-rate-limit');
const RedisStore = require('rate-limit-redis');
const redis = require('redis');
const customRedisClient = redis.createClient({
host: 'custom-redis-host',
port: 6379,
password: 'your-redis-password'
});
const limiter = rateLimit({
store: new RedisStore({
client: customRedisClient
}),
max: 50, // limit each IP to 50 requests per windowMs
windowMs: 10 * 60 * 1000 // 10 minutes
});
const express = require('express');
const app = express();
app.use(limiter);
app.get('/', (req, res) => {
res.send('Hello, world!');
});
app.listen(3000, () => {
console.log('Server running on port 3000');
});
Dynamic Rate Limiting
This code demonstrates how to implement dynamic rate limiting based on user properties. In this example, premium users are allowed more requests compared to regular users.
const rateLimit = require('express-rate-limit');
const RedisStore = require('rate-limit-redis');
const redisClient = require('redis').createClient();
const dynamicLimiter = rateLimit({
store: new RedisStore({
client: redisClient
}),
max: (req, res) => {
if (req.user && req.user.isPremium) {
return 200; // higher limit for premium users
}
return 100; // default limit
},
windowMs: 15 * 60 * 1000 // 15 minutes
});
const express = require('express');
const app = express();
app.use(dynamicLimiter);
app.get('/', (req, res) => {
res.send('Hello, world!');
});
app.listen(3000, () => {
console.log('Server running on port 3000');
});
The express-rate-limit package is a middleware for rate limiting in Express applications. It does not include a built-in store for Redis, but it can be extended with custom stores like rate-limit-redis. It is a good choice if you need a simple rate limiting solution without a specific storage backend.
The rate-limiter-flexible package is a highly flexible rate limiting library that supports various backends including Redis, MongoDB, and in-memory storage. It offers more advanced features and customization options compared to rate-limit-redis, making it suitable for complex rate limiting requirements.
The redis-rate-limiter package is a Redis-based rate limiter that can be used with any Node.js application. It provides a simple API for rate limiting and is not tied to Express, making it a versatile choice for different types of applications.
FAQs
A Redis store for the `express-rate-limit` middleware
The npm package rate-limit-redis receives a total of 164,739 weekly downloads. As such, rate-limit-redis popularity was classified as popular.
We found that rate-limit-redis demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Ensure open-source compliance with Socket’s License Enforcement Beta. Set up your License Policy and secure your software!
Product
We're launching a new set of license analysis and compliance features for analyzing, managing, and complying with licenses across a range of supported languages and ecosystems.
Product
We're excited to introduce Socket Optimize, a powerful CLI command to secure open source dependencies with tested, optimized package overrides.