rax-checkbox
Advanced tools
Comparing version 0.6.5 to 1.0.0-beta.1
@@ -1,57 +0,28 @@ | ||
import * as Rax from "rax"; | ||
import {BaseProps} from "rax"; | ||
import * as React from "react"; | ||
/** | ||
* component:checkbox (选择框) | ||
* document address(文档地址): | ||
* https://alibaba.github.io/rax/component/checkbox | ||
*/ | ||
export interface CheckBoxProps extends BaseProps { | ||
import { CSSProperties, FunctionComponent } from "rax"; | ||
import "./index.css"; | ||
export interface CheckBoxProps { | ||
/** | ||
* selected state (选中状态) | ||
* selected state | ||
*/ | ||
checked: boolean; | ||
/** | ||
* picture selected (处于选中状态中的图片) | ||
*/ | ||
checkedImage: string; | ||
/** | ||
* unselected picture (非选中状态中的图片) | ||
* unselected picture | ||
*/ | ||
uncheckedImage: string; | ||
/** | ||
* select box container style (选择框容器样式) | ||
* select box container style | ||
*/ | ||
containerStyle: React.CSSProperties; | ||
containerStyle: CSSProperties; | ||
/** | ||
* select box picture style (选择框图片样式) | ||
* select box picture style | ||
*/ | ||
checkboxStyle: React.CSSProperties; | ||
checkboxStyle: CSSProperties; | ||
/** | ||
* change event (选择事件) | ||
* @param checked checked(是否选中) | ||
* change event | ||
* @param checked checked | ||
*/ | ||
onChange: (checked) => void; | ||
onChange: (checked?: boolean) => void; | ||
} | ||
declare class CheckBox extends Rax.Component<CheckBoxProps, any> { | ||
static defaultProps: { | ||
checked: boolean; | ||
checkedImage: string; | ||
uncheckedImage: string; | ||
}; | ||
render(): JSX.Element; | ||
} | ||
declare const CheckBox: FunctionComponent<CheckBoxProps>; | ||
export default CheckBox; |
167
lib/index.js
"use strict"; | ||
Object.defineProperty(exports, "__esModule", { | ||
value: true | ||
}); | ||
exports.__esModule = true; | ||
exports.default = void 0; | ||
@@ -14,128 +12,63 @@ | ||
var _raxTouchable = _interopRequireDefault(require("rax-touchable")); | ||
require("./index.css"); | ||
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; } | ||
function _typeof(obj) { if (typeof Symbol === "function" && typeof Symbol.iterator === "symbol") { _typeof = function _typeof(obj) { return typeof obj; }; } else { _typeof = function _typeof(obj) { return obj && typeof Symbol === "function" && obj.constructor === Symbol && obj !== Symbol.prototype ? "symbol" : typeof obj; }; } return _typeof(obj); } | ||
var CHECKED_ICON = "data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAAABoAAAAaCAMAAACelLz8AAAABGdBTUEAALGPC/xhBQAAAAFzUkdCAK7OHOkAAAAwUExURUxpcTMzMzMzMzMzMzMzMzMzMzMzMzMzMzMzMzMzMzMzMzMzMzMzMzMzMzMzMzMzMzLRwScAAAAPdFJOUwDvEI8wz69QQL/fIHCAYDHs4yUAAACoSURBVCjPfdIBDoMgDAXQ31JE1K33v60UZSJ2NjEmvvgpUIAD6aMoMMBR3YqMoBpmDDXbV1B5nApKUB3/kU9ZR1QLDcKklACPJmvCpWSSPWLbJK0e1bgNDrW4J/3iLmLbSov7oqNyjtFsM5nQUzyOc61xfKPlOOpsr4QbSb0x6uKuNqTdZovrmm+W8KDTJjhUbeGB5LS8SDcChV4GwB0bqWPzMmz/R3QHJwAPwC8jHWQAAAAASUVORK5CYII="; | ||
var UNCHECKED_ICON = "data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAAABoAAAAaCAMAAACelLz8AAAABGdBTUEAALGPC/xhBQAAAAFzUkdCAK7OHOkAAAAYUExURUxpcTMzMzMzMzMzMzMzMzMzMzMzMzMzM2vW5DoAAAAHdFJOUwCPEO9AzzBOX/xUAAAASklEQVQoz+2SMRKAQAwCIcmF///YaKl4tYU7Q8O2CwRTD5IBxJJlBSixcKPOFzkzUAmpnGppFCy/+qpqZ2rUJgCbTV/ZbGJ7T/QAwyIE71akwQMAAAAASUVORK5CYII="; | ||
function _classCallCheck(instance, Constructor) { if (!(instance instanceof Constructor)) { throw new TypeError("Cannot call a class as a function"); } } | ||
var CheckBox = function CheckBox(_ref) { | ||
var _ref$checked = _ref.checked, | ||
checked = _ref$checked === void 0 ? false : _ref$checked, | ||
_ref$checkedImage = _ref.checkedImage, | ||
checkedImage = _ref$checkedImage === void 0 ? CHECKED_ICON : _ref$checkedImage, | ||
_ref$uncheckedImage = _ref.uncheckedImage, | ||
uncheckedImage = _ref$uncheckedImage === void 0 ? UNCHECKED_ICON : _ref$uncheckedImage, | ||
_ref$containerStyle = _ref.containerStyle, | ||
containerStyle = _ref$containerStyle === void 0 ? {} : _ref$containerStyle, | ||
_ref$checkboxStyle = _ref.checkboxStyle, | ||
checkboxStyle = _ref$checkboxStyle === void 0 ? {} : _ref$checkboxStyle, | ||
onChange = _ref.onChange; | ||
function _defineProperties(target, props) { for (var i = 0; i < props.length; i++) { var descriptor = props[i]; descriptor.enumerable = descriptor.enumerable || false; descriptor.configurable = true; if ("value" in descriptor) descriptor.writable = true; Object.defineProperty(target, descriptor.key, descriptor); } } | ||
var _useState = (0, _rax.useState)(checked), | ||
isChecked = _useState[0], | ||
setIsChecked = _useState[1]; | ||
function _createClass(Constructor, protoProps, staticProps) { if (protoProps) _defineProperties(Constructor.prototype, protoProps); if (staticProps) _defineProperties(Constructor, staticProps); return Constructor; } | ||
var handleChange = function handleChange() { | ||
var _checked = !isChecked; | ||
function _possibleConstructorReturn(self, call) { if (call && (_typeof(call) === "object" || typeof call === "function")) { return call; } return _assertThisInitialized(self); } | ||
setIsChecked(_checked); | ||
function _getPrototypeOf(o) { _getPrototypeOf = Object.setPrototypeOf ? Object.getPrototypeOf : function _getPrototypeOf(o) { return o.__proto__ || Object.getPrototypeOf(o); }; return _getPrototypeOf(o); } | ||
function _inherits(subClass, superClass) { if (typeof superClass !== "function" && superClass !== null) { throw new TypeError("Super expression must either be null or a function"); } subClass.prototype = Object.create(superClass && superClass.prototype, { constructor: { value: subClass, writable: true, configurable: true } }); if (superClass) _setPrototypeOf(subClass, superClass); } | ||
function _setPrototypeOf(o, p) { _setPrototypeOf = Object.setPrototypeOf || function _setPrototypeOf(o, p) { o.__proto__ = p; return o; }; return _setPrototypeOf(o, p); } | ||
function _assertThisInitialized(self) { if (self === void 0) { throw new ReferenceError("this hasn't been initialised - super() hasn't been called"); } return self; } | ||
function _defineProperty(obj, key, value) { if (key in obj) { Object.defineProperty(obj, key, { value: value, enumerable: true, configurable: true, writable: true }); } else { obj[key] = value; } return obj; } | ||
var CHECKED_ICON = 'data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAAABoAAAAaCAMAAACelLz8AAAABGdBTUEAALGPC/xhBQAAAAFzUkdCAK7OHOkAAAAwUExURUxpcTMzMzMzMzMzMzMzMzMzMzMzMzMzMzMzMzMzMzMzMzMzMzMzMzMzMzMzMzMzMzLRwScAAAAPdFJOUwDvEI8wz69QQL/fIHCAYDHs4yUAAACoSURBVCjPfdIBDoMgDAXQ31JE1K33v60UZSJ2NjEmvvgpUIAD6aMoMMBR3YqMoBpmDDXbV1B5nApKUB3/kU9ZR1QLDcKklACPJmvCpWSSPWLbJK0e1bgNDrW4J/3iLmLbSov7oqNyjtFsM5nQUzyOc61xfKPlOOpsr4QbSb0x6uKuNqTdZovrmm+W8KDTJjhUbeGB5LS8SDcChV4GwB0bqWPzMmz/R3QHJwAPwC8jHWQAAAAASUVORK5CYII='; | ||
var UNCHECKED_ICON = 'data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAAABoAAAAaCAMAAACelLz8AAAABGdBTUEAALGPC/xhBQAAAAFzUkdCAK7OHOkAAAAYUExURUxpcTMzMzMzMzMzMzMzMzMzMzMzMzMzM2vW5DoAAAAHdFJOUwCPEO9AzzBOX/xUAAAASklEQVQoz+2SMRKAQAwCIcmF///YaKl4tYU7Q8O2CwRTD5IBxJJlBSixcKPOFzkzUAmpnGppFCy/+qpqZ2rUJgCbTV/ZbGJ7T/QAwyIE71akwQMAAAAASUVORK5CYII='; | ||
var CheckBox = | ||
/*#__PURE__*/ | ||
function (_Component) { | ||
_inherits(CheckBox, _Component); | ||
function CheckBox() { | ||
var _getPrototypeOf2; | ||
var _this; | ||
_classCallCheck(this, CheckBox); | ||
for (var _len = arguments.length, args = new Array(_len), _key = 0; _key < _len; _key++) { | ||
args[_key] = arguments[_key]; | ||
if (onChange) { | ||
onChange(_checked); | ||
} | ||
}; | ||
_this = _possibleConstructorReturn(this, (_getPrototypeOf2 = _getPrototypeOf(CheckBox)).call.apply(_getPrototypeOf2, [this].concat(args))); | ||
_defineProperty(_assertThisInitialized(_assertThisInitialized(_this)), "state", { | ||
checked: Boolean(_this.props.checked) | ||
}); | ||
_defineProperty(_assertThisInitialized(_assertThisInitialized(_this)), "onChange", function () { | ||
var onChange = _this.props.onChange; | ||
var checked = !_this.state.checked; | ||
_this.setState({ | ||
checked: checked | ||
}); | ||
if (onChange) { | ||
onChange(checked); | ||
} | ||
}); | ||
return _this; | ||
} | ||
_createClass(CheckBox, [{ | ||
key: "componentWillReceiveProps", | ||
value: function componentWillReceiveProps(nextProps) { | ||
if (this.props.checked !== nextProps.checked) { | ||
this.setState({ | ||
checked: Boolean(nextProps.checked) | ||
}); | ||
} | ||
(0, _rax.useEffect)(function () { | ||
setIsChecked(Boolean(checked)); | ||
}, [checked]); | ||
return (0, _rax.createElement)(_raxView.default, { | ||
role: "checkbox", | ||
"aria-checked": isChecked, | ||
onClick: handleChange, | ||
className: "flexContainer" | ||
}, (0, _rax.createElement)(_raxView.default, { | ||
className: "container", | ||
style: Object.assign({ | ||
flexDirection: "row", | ||
alignItems: "center", | ||
marginBottom: 10 | ||
}, containerStyle) | ||
}, (0, _rax.createElement)(_raxImage.default, { | ||
style: Object.assign({ | ||
width: 40, | ||
height: 40 | ||
}, checkboxStyle), | ||
source: { | ||
uri: isChecked ? checkedImage : uncheckedImage | ||
} | ||
}, { | ||
key: "render", | ||
value: function render() { | ||
var _this$props = this.props, | ||
checkedImage = _this$props.checkedImage, | ||
uncheckedImage = _this$props.uncheckedImage, | ||
containerStyle = _this$props.containerStyle, | ||
checkboxStyle = _this$props.checkboxStyle; | ||
var imageSource = this.state.checked ? checkedImage : uncheckedImage; | ||
}))); | ||
}; | ||
if (typeof imageSource === 'string') { | ||
imageSource = { | ||
uri: imageSource | ||
}; | ||
} | ||
return (0, _rax.createElement)(_raxTouchable.default, { | ||
role: "checkbox", | ||
"aria-checked": this.state.checked, | ||
onPress: this.onChange, | ||
style: styles.flexContainer | ||
}, (0, _rax.createElement)(_raxView.default, { | ||
style: [styles.container, containerStyle] | ||
}, (0, _rax.createElement)(_raxImage.default, { | ||
style: [styles.checkbox, checkboxStyle], | ||
source: imageSource | ||
}))); | ||
} | ||
}]); | ||
return CheckBox; | ||
}(_rax.Component); | ||
_defineProperty(CheckBox, "defaultProps", { | ||
checked: false, | ||
checkedImage: CHECKED_ICON, | ||
uncheckedImage: UNCHECKED_ICON | ||
}); | ||
var styles = { | ||
container: { | ||
flexDirection: 'row', | ||
alignItems: 'center', | ||
marginBottom: 10 | ||
}, | ||
checkbox: { | ||
width: 40, | ||
height: 40 | ||
} | ||
}; | ||
var _default = CheckBox; | ||
exports.default = _default; | ||
module.exports = exports["default"]; | ||
exports.default = _default; |
{ | ||
"name": "rax-checkbox", | ||
"version": "0.6.5", | ||
"description": "CheckBox component for Rax.", | ||
"version": "1.0.0-beta.1", | ||
"description": "Checkbox component for Rax.", | ||
"license": "BSD-3-Clause", | ||
"main": "lib/index.js", | ||
"repository": { | ||
"type": "git", | ||
"url": "git+https://github.com/alibaba/rax.git" | ||
"main": "src/index.js", | ||
"types": "lib/index.d.ts", | ||
"scripts": { | ||
"clean": "rm -rf ./lib && rm -rf ./package-lock.json && rm -rf ./yarn.lock", | ||
"build": "npm run clean && rax-scripts build --type 'component'", | ||
"start": "rax-scripts start --type 'component' -p 8000", | ||
"miniapp": "rax-scripts start --type 'component-miniapp'", | ||
"prepublishOnly": "npm run build", | ||
"test": "npm run build && rax-scripts test", | ||
"lint": "eslint --ext .js --ext .jsx --ext .ts --ext .tsx ./src" | ||
}, | ||
"keywords": [ | ||
"Rax", | ||
"rax-component", | ||
"react-component" | ||
"rax-component" | ||
], | ||
"bugs": { | ||
"url": "https://github.com/alibaba/rax/issues" | ||
"miniappConfig": { | ||
"main": "lib/miniapp/index" | ||
}, | ||
"homepage": "https://github.com/alibaba/rax#readme", | ||
"engines": { | ||
@@ -24,13 +28,22 @@ "npm": ">=3.0.0" | ||
"dependencies": { | ||
"rax-image": "^0.6.5", | ||
"rax-touchable": "^0.6.5", | ||
"rax-view": "^0.6.5" | ||
"rax-image": "^1.0.3", | ||
"rax-view": "^1.0.2" | ||
}, | ||
"peerDependencies": { | ||
"rax": "0.x" | ||
"rax": "^1.0.0" | ||
}, | ||
"devDependencies": { | ||
"rax-test-renderer": "^0.6.5", | ||
"rax-text": "^0.6.5" | ||
"@rax-types/rax": "^1.0.5", | ||
"@types/ali-app": "^1.0.0", | ||
"@types/jest": "^24.0.12", | ||
"@typescript-eslint/eslint-plugin": "^1.7.0", | ||
"@typescript-eslint/parser": "^1.7.0", | ||
"driver-universal": "^1.0.1", | ||
"eslint": "^5.16.0", | ||
"eslint-config-rax": "^0.0.0", | ||
"rax": "^1.0.0", | ||
"rax-scripts": "^1.2.0", | ||
"rax-test-renderer": "^1.0.0", | ||
"rax-text": "^1.0.1" | ||
} | ||
} |
@@ -1,8 +0,8 @@ | ||
# CheckBox | ||
# CheckBox 选择框 | ||
[](https://www.npmjs.com/package/rax-checkbox) | ||
CheckBox 是基础的选择框,选择框用图片实现,支持用户使用自己的图片进行替换 | ||
CheckBox is the basis of the selection box, select the box with a picture to achieve, to support users to use their own images to replace | ||
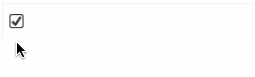 | ||
## Install | ||
## 安装 | ||
@@ -13,44 +13,41 @@ ```bash | ||
## Import | ||
## 引用 | ||
```jsx | ||
import CheckBox from 'rax-checkbox'; | ||
import CheckBox from "rax-checkbox"; | ||
``` | ||
## Props | ||
## 属性 | ||
| name | type | default | describe | | ||
| :------ | :------- | :--- | :--- | | ||
| checked | Boolean | | selected state | | ||
| checkedImage | String | | selected picture | | ||
| uncheckedImage | String | | unselected picture | | ||
| containerStyle | Object | | select container style | | ||
| checkboxStyle | Object | | select picture style | | ||
| onChange | Function | | select event | | ||
| 名称 | 类型 | 默认值 | 描述 | | ||
| :------------- | :------- | :----- | :------------- | | ||
| checked | Boolean | | 选中状态 | | ||
| checkedImage | String | | 选中图片 | | ||
| uncheckedImage | String | | 非选中图片 | | ||
| containerStyle | Object | | 选择框容器样式 | | ||
| checkboxStyle | Object | | 选择框图片样式 | | ||
| onChange | Function | | 选择事件 | | ||
## Example | ||
## 基本示例 | ||
```jsx | ||
// demo | ||
import {createElement, Component, render} from 'rax'; | ||
/* eslint-disable import/no-extraneous-dependencies */ | ||
import { createElement, render, useRef, useEffect } from 'rax'; | ||
import DU from 'driver-universal'; | ||
import View from 'rax-view'; | ||
import Button from 'rax-checkbox'; | ||
import CheckBox from 'rax-checkbox'; | ||
class App extends Component { | ||
render() { | ||
return ( | ||
<View style={{ width: 750 }}> | ||
<CheckBox | ||
containerStyle={{ | ||
marginTop: 10, | ||
}} | ||
onChange={(checked) => { | ||
console.log('checked', checked); | ||
}} /> | ||
</View> | ||
); | ||
} | ||
} | ||
const App = () => { | ||
const checkboxRef = useRef(null); | ||
useEffect(() => { | ||
console.log(checkboxRef); | ||
}); | ||
return <View ><CheckBox ref={checkboxRef} /></View>; | ||
}; | ||
render(<App />); | ||
``` | ||
render(<App />, document.body, { | ||
driver: DU | ||
}); | ||
``` |
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
Deprecated
MaintenanceThe maintainer of the package marked it as deprecated. This could indicate that a single version should not be used, or that the package is no longer maintained and any new vulnerabilities will not be fixed.
Found 1 instance in 1 package
Major refactor
Supply chain riskPackage has recently undergone a major refactor. It may be unstable or indicate significant internal changes. Use caution when updating to versions that include significant changes.
Found 1 instance in 1 package
New author
Supply chain riskA new npm collaborator published a version of the package for the first time. New collaborators are usually benign additions to a project, but do indicate a change to the security surface area of a package.
Found 1 instance in 1 package
No bug tracker
MaintenancePackage does not have a linked bug tracker in package.json.
Found 1 instance in 1 package
No repository
Supply chain riskPackage does not have a linked source code repository. Without this field, a package will have no reference to the location of the source code use to generate the package.
Found 1 instance in 1 package
No website
QualityPackage does not have a website.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
Major refactor
Supply chain riskPackage has recently undergone a major refactor. It may be unstable or indicate significant internal changes. Use caution when updating to versions that include significant changes.
Found 1 instance in 1 package
Uses eval
Supply chain riskPackage uses dynamic code execution (e.g., eval()), which is a dangerous practice. This can prevent the code from running in certain environments and increases the risk that the code may contain exploits or malicious behavior.
Found 1 instance in 1 package
Minified code
QualityThis package contains minified code. This may be harmless in some cases where minified code is included in packaged libraries, however packages on npm should not minify code.
Found 1 instance in 1 package
3
30
2
2
16310
12
434
3
1
53
1
+ Added@babel/runtime@7.26.0(transitive)
+ Added@uni/env@1.1.1(transitive)
+ Addedclassnames@2.5.1(transitive)
+ Addedjs-tokens@4.0.0(transitive)
+ Addedloose-envify@1.4.0(transitive)
+ Addedobject-assign@4.1.1(transitive)
+ Addedprop-types@15.8.1(transitive)
+ Addedrax@1.2.3(transitive)
+ Addedrax-children@1.0.0(transitive)
+ Addedrax-clone-element@1.0.0(transitive)
+ Addedrax-create-factory@1.0.0(transitive)
+ Addedrax-image@1.1.3(transitive)
+ Addedrax-is-valid-element@1.0.1(transitive)
+ Addedrax-view@1.2.0(transitive)
+ Addedreact-is@16.13.1(transitive)
+ Addedregenerator-runtime@0.14.1(transitive)
+ Addeduniversal-env@1.0.73.3.3(transitive)
- Removedrax-touchable@^0.6.5
- Removeddriver-browser@0.6.7(transitive)
- Removeddriver-server@0.6.5(transitive)
- Removeddriver-weex@0.6.5(transitive)
- Removedrax@0.6.7(transitive)
- Removedrax-image@0.6.8(transitive)
- Removedrax-touchable@0.6.5(transitive)
- Removedrax-view@0.6.5(transitive)
- Removedstyle-unit@0.6.5(transitive)
- Removeduniversal-env@0.6.6(transitive)
Updatedrax-image@^1.0.3
Updatedrax-view@^1.0.2