What is rc-animate?
The rc-animate package is a React component for creating animations. It provides a simple way to animate elements as they enter and leave the DOM, making it useful for creating dynamic and interactive user interfaces.
What are rc-animate's main functionalities?
Basic Animation
This example demonstrates a basic animation using rc-animate. When the button is clicked, the 'Hello World' box will fade in and out.
const React = require('react');
const ReactDOM = require('react-dom');
const Animate = require('rc-animate');
class App extends React.Component {
state = { show: true };
toggle = () => {
this.setState({ show: !this.state.show });
};
render() {
return (
<div>
<button onClick={this.toggle}>Toggle</button>
<Animate transitionName="fade">
{this.state.show ? <div key="1" className="box">Hello World</div> : null}
</Animate>
</div>
);
}
}
ReactDOM.render(<App />, document.getElementById('root'));
Custom Animation
This example shows how to use custom CSS classes for animations. The 'custom-enter' and 'custom-leave' classes can be defined in your CSS to create custom animations.
const React = require('react');
const ReactDOM = require('react-dom');
const Animate = require('rc-animate');
class App extends React.Component {
state = { show: true };
toggle = () => {
this.setState({ show: !this.state.show });
};
render() {
return (
<div>
<button onClick={this.toggle}>Toggle</button>
<Animate
transitionName={{
enter: 'custom-enter',
leave: 'custom-leave'
}}
>
{this.state.show ? <div key="1" className="box">Hello World</div> : null}
</Animate>
</div>
);
}
}
ReactDOM.render(<App />, document.getElementById('root'));
Other packages similar to rc-animate
react-transition-group
react-transition-group is a popular package for managing animations in React. It provides more control over the animation lifecycle and is more flexible compared to rc-animate. It supports more complex animations and transitions.
framer-motion
framer-motion is a powerful animation library for React. It offers a wide range of animation capabilities, including gestures, keyframes, and layout animations. It is more feature-rich and modern compared to rc-animate.
react-spring
react-spring is a spring-physics-based animation library for React. It provides a more natural and fluid animation experience. It is highly customizable and can handle complex animation scenarios better than rc-animate.
rc-animate
animate react element easily

Screenshots
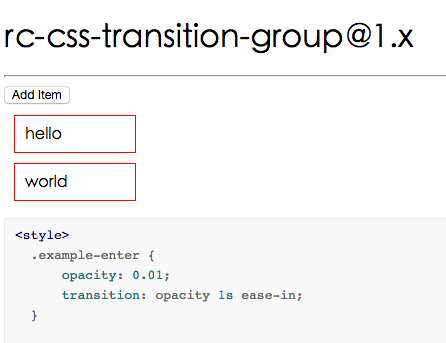
Feature
- support ie8,ie8+,chrome,firefox,safari
install

Usage
var Animate = require('rc-animate');
var React = require('react');
React.render(<Animate><p key="1">1</p><p key="2">2</p></Animate>, container);
API
props
name | type | default | description |
---|
component | React.Element/String | 'span' | wrap dom node or component for children. set to '' if you do not wrap for only one child |
showProp | String | | using prop for show and hide. [demo](http://react-component.github.io/animate/examples/hide-todo.html) |
exclusive | Boolean | | whether allow only one set of animations(enter and leave) at the same time. |
transitionName | String | | transitionName, need to specify corresponding css |
transitionEnter | Boolean | true | whether support transition enter anim |
transitionLeave | Boolean | true | whether support transition leave anim |
onEnd | function(key:String, enter:Boolean) | true | animation end callback |
animation | Object | {} |
to animate with js. for examples:
```js
{
enter: function(node, done){
node.style.display='none';
$(node).slideUp(done);
return {
stop:function(){
$(node).stop(true);
}
};
},
leave: function(node, done){
node.style.display='';
$(node).slideDown(done);
return {
stop:function(){
$(node).stop(true);
}
};
}
}
```
|
Development
npm install
npm start
Example
http://localhost:8000/examples/index.md
online example: http://react-component.github.io/animate/examples/
Test Case
http://localhost:8000/tests/runner.html?coverage
Coverage
http://localhost:8000/node_modules/rc-server/node_modules/node-jscover/lib/front-end/jscoverage.html?w=http://localhost:8000/tests/runner.html?coverage
License
rc-animate is released under the MIT license.