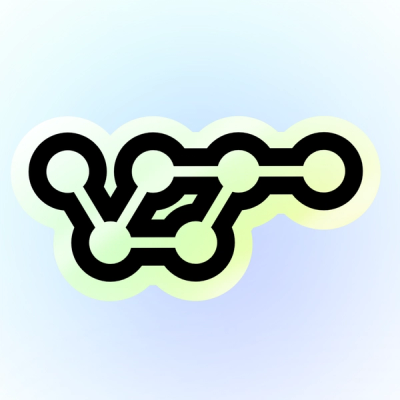
Security News
vlt Debuts New JavaScript Package Manager and Serverless Registry at NodeConf EU
vlt introduced its new package manager and a serverless registry this week, innovating in a space where npm has stagnated.
The rc-image npm package is a React component library for displaying images in a more versatile and enhanced manner. It provides features such as modal viewing, image rotation, zooming, and dragging, making it a powerful tool for implementing image galleries or for enhancing the image viewing experience in web applications.
Modal Image Viewing
This feature allows images to be viewed in a modal (popup) when clicked, providing a closer look. The code sample demonstrates how to enable modal viewing with visibility control.
{"import React from 'react';\nimport Image from 'rc-image';\n\nexport default () => (\n <Image\n preview={{\n visible: true,\n onVisibleChange: (vis) => console.log('visible:', vis),\n }}\n src='path/to/image.jpg'\n />\n);"}
Image Rotation
Enables the rotation of images. This example shows how to rotate an image by 90 degrees using inline styles.
{"import React from 'react';\nimport Image from 'rc-image';\n\nexport default () => (\n <Image\n style={{ transform: 'rotate(90deg)' }}\n src='path/to/image.jpg'\n />\n);"}
Zoom and Drag
This feature allows users to zoom in/out on images and drag them around for better viewing. The code sample demonstrates enabling zoom and drag capabilities in the preview modal.
{"import React from 'react';\nimport Image from 'rc-image';\n\nexport default () => (\n <Image\n preview={{\n zoomable: true,\n draggable: true,\n }}\n src='path/to/image.jpg'\n />\n);"}
A flexible and responsive lightbox component for displaying images and videos in React. It offers similar modal viewing capabilities but is more focused on lightbox functionalities and does not support image rotation or dragging out of the box.
This package is designed for creating image galleries in React applications. It supports thumbnail navigation, fullscreen mode, and custom renderers but lacks the advanced image manipulation features like rotation and dragging found in rc-image.
Offers a rich set of features for image viewing in React, including pinch to zoom, slide to close, and rotation. It is similar to rc-image in terms of providing an enhanced image viewing experience but focuses more on touch interactions.
React Image.
npm install
npm start
import Image from 'rc-image';
export default () => (
<Image src="https://zos.alipayobjects.com/rmsportal/jkjgkEfvpUPVyRjUImniVslZfWPnJuuZ.png" />
);
Name | Type | Default | Description |
---|---|---|---|
preview | boolean | PreviewType | true | Whether to show preview |
prefixCls | string | rc-image | Classname prefix |
placeholder | boolean | ReactElement | - | if true will set default placeholder or use ReactElement set customize placeholder |
fallback | string | - | Load failed src |
previewPrefixCls | string | rc-image-preview | Preview classname prefix |
onError | (event: Event) => void | - | Load failed callback |
Name | Type | Default | Description |
---|---|---|---|
visible | boolean | - | Whether the preview is open or not |
closeIcon | React.ReactNode | - | Custom close icon |
src | string | - | Customize preview src |
movable | boolean | true | Enable drag |
scaleStep | number | 0.5 | The number to which the scale is increased or decreased |
minScale | number | 1 | Min scale |
maxScale | number | 50 | Max scale |
forceRender | boolean | - | Force render preview |
getContainer | string | HTMLElement | (() => HTMLElement) | false | document.body | Return the mount node for preview |
imageRender | (originalNode: React.ReactElement, info: { transform: TransformType }) => React.ReactNode | - | Customize image |
toolbarRender | (originalNode: React.ReactElement, info: Omit<ToolbarRenderInfoType, 'current' | 'total'>) => React.ReactNode | - | Customize toolbar |
onVisibleChange | (visible: boolean, prevVisible: boolean) => void | - | Callback when visible is changed |
onTransform | { transform: TransformType, action: TransformAction } | - | Callback when transform is changed |
preview the merged src
import Image from 'rc-image';
export default () => (
<Image.PreviewGroup>
<Image src="https://zos.alipayobjects.com/rmsportal/jkjgkEfvpUPVyRjUImniVslZfWPnJuuZ.png" />
<Image src="https://gw.alipayobjects.com/mdn/rms_08e378/afts/img/A*P0S-QIRUbsUAAAAAAAAAAABkARQnAQ" />
</Image.PreviewGroup>
);
Name | Type | Default | Description |
---|---|---|---|
preview | boolean | PreviewGroupType | true | Whether to show preview, current: If Preview the show img index, default 0 |
previewPrefixCls | string | rc-image-preview | Preview classname prefix |
icons | { [iconKey]?: ReactNode } | - | Icons in the top operation bar, iconKey: 'rotateLeft' | 'rotateRight' | 'zoomIn' | 'zoomOut' | 'close' | 'left' | 'right' |
fallback | string | - | Load failed src |
items | (string | { src: string, alt: string, crossOrigin: string, ... })[] | - | preview group |
Name | Type | Default | Description |
---|---|---|---|
visible | boolean | - | Whether the preview is open or not |
movable | boolean | true | Enable drag |
current | number | - | Current index |
closeIcon | React.ReactNode | - | Custom close icon |
scaleStep | number | 0.5 | The number to which the scale is increased or decreased |
minScale | number | 1 | Min scale |
maxScale | number | 50 | Max scale |
forceRender | boolean | - | Force render preview |
getContainer | string | HTMLElement | (() => HTMLElement) | false | document.body | Return the mount node for preview |
countRender | (current: number, total: number) => ReactNode | - | Customize count |
imageRender | (originalNode: React.ReactElement, info: { transform: TransformType, current: number }) => React.ReactNode | - | Customize image |
toolbarRender | (originalNode: React.ReactElement, info: ToolbarRenderInfoType) => React.ReactNode | - | Customize toolbar |
onVisibleChange | (visible: boolean, prevVisible: boolean, current: number) => void | - | Callback when visible is changed |
onTransform | { transform: TransformType, action: TransformAction } | - | Callback when transform is changed |
{
x: number;
y: number;
rotate: number;
scale: number;
flipX: boolean;
flipY: boolean;
}
type TransformAction =
| 'flipY'
| 'flipX'
| 'rotateLeft'
| 'rotateRight'
| 'zoomIn'
| 'zoomOut'
| 'close'
| 'prev'
| 'next'
| 'wheel'
| 'doubleClick'
| 'move'
| 'dragRebound';
{
icons: {
flipYIcon: React.ReactNode;
flipXIcon: React.ReactNode;
rotateLeftIcon: React.ReactNode;
rotateRightIcon: React.ReactNode;
zoomOutIcon: React.ReactNode;
zoomInIcon: React.ReactNode;
};
actions: {
onFlipY: () => void;
onFlipX: () => void;
onRotateLeft: () => void;
onRotateRight: () => void;
onZoomOut: () => void;
onZoomIn: () => void;
};
transform: {
x: number;
y: number;
rotate: number;
scale: number;
flipX: boolean;
flipY: boolean;
},
current: number;
total: number;
}
http://localhost:8003/examples/
npm test
npm run coverage
rc-image is released under the MIT license.
FAQs
React easy to use image component
The npm package rc-image receives a total of 959,329 weekly downloads. As such, rc-image popularity was classified as popular.
We found that rc-image demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt introduced its new package manager and a serverless registry this week, innovating in a space where npm has stagnated.
Security News
Research
The Socket Research Team uncovered a malicious Python package typosquatting the popular 'fabric' SSH library, silently exfiltrating AWS credentials from unsuspecting developers.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.