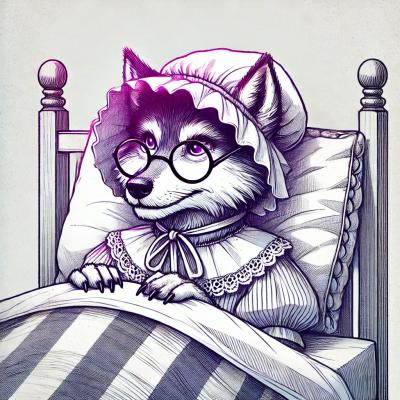
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
rc-pagination
Advanced tools
The rc-pagination package is a React component for creating paginated interfaces, allowing users to navigate through pages of items. It provides a range of customization options to tailor the pagination behavior and appearance to the needs of different applications.
Basic Pagination
This code sample demonstrates how to render a basic pagination component with a total of 50 items.
import Pagination from 'rc-pagination';
import React from 'react';
import ReactDOM from 'react-dom';
ReactDOM.render(
<Pagination total={50} />,
document.getElementById('container')
);
Custom Page Size
This code sample shows how to set a custom page size and enable a size changer dropdown for the user to select how many items to display per page.
import Pagination from 'rc-pagination';
import React from 'react';
import ReactDOM from 'react-dom';
ReactDOM.render(
<Pagination total={500} defaultPageSize={20} showSizeChanger />,
document.getElementById('container')
);
Controlled Component
This code sample illustrates how to create a controlled pagination component where the current page state is managed by the parent component.
import Pagination from 'rc-pagination';
import React, { useState } from 'react';
import ReactDOM from 'react-dom';
const App = () => {
const [current, setCurrent] = useState(1);
const onChange = page => {
setCurrent(page);
};
return (
<Pagination current={current} onChange={onChange} total={100} />
);
};
ReactDOM.render(<App />, document.getElementById('container'));
Jump to Page
This code sample demonstrates how to enable the 'quick jumper' feature, allowing users to jump to a specific page directly.
import Pagination from 'rc-pagination';
import React from 'react';
import ReactDOM from 'react-dom';
ReactDOM.render(
<Pagination total={100} showQuickJumper />,
document.getElementById('container')
);
react-paginate is a React component to create pagination. It provides built-in styles and is highly customizable. Compared to rc-pagination, react-paginate might be more suitable for those who prefer a component with out-of-the-box styled elements.
react-js-pagination is another React component for pagination. It is similar to rc-pagination but offers different customization options and styles. It might be a good alternative for users looking for a slightly different look and feel.
material-ui is a set of React components that implement Google's Material Design, including a pagination component. It is a comprehensive UI framework that offers a Material Design styled pagination component, which is different from the minimalistic approach of rc-pagination.
React Pagination Component.
npm install
npm start
Online example: https://pagination-react-component.vercel.app
Local example: npm run start
then http://localhost:9001
import Pagination from 'rc-pagination';
ReactDOM.render(<Pagination />, container);
Parameter | Description | Type | Default |
---|---|---|---|
disabled | disable pagination | Bool | - |
defaultCurrent | uncontrolled current page | Number | 1 |
current | current page | Number | undefined |
total | items total count | Number | 0 |
defaultPageSize | default items per page | Number | 10 |
pageSize | items per page | Number | 10 |
onChange | page change callback | Function(current, pageSize) | - |
showSizeChanger | show pageSize changer | Bool | false when total less then totalBoundaryShowSizeChanger , true when otherwise |
totalBoundaryShowSizeChanger | when total larger than it, showSizeChanger will be true | number | 50 |
pageSizeOptions | specify the sizeChanger selections | Array | ['10', '20', '50', '100'] |
onShowSizeChange | pageSize change callback | Function(current, size) | - |
hideOnSinglePage | hide on single page | Bool | false |
showPrevNextJumpers | show jump-prev, jump-next | Bool | true |
showQuickJumper | show quick goto jumper | Bool / Object | false / {goButton: true} |
showTotal | show total records and range | Function(total, [from, to]) | - |
className | className of pagination | String | - |
simple | when set, show simple pager | Object | null |
locale | to set l10n config | Object | zh_CN |
style | the style of pagination | Object | {} |
showLessItems | show less page items | Bool | false |
showTitle | show page items title | Bool | true |
itemRender | custom page item renderer | Function(current, type: 'page' | 'prev' | 'next' | 'jump-prev' | 'jump-next', element): React.ReactNode | (current, type, element) => element | |
prevIcon | specify the default previous icon | ReactNode | (props: PaginationProps) => ReactNode | |
nextIcon | specify the default next icon | ReactNode | (props: PaginationProps) => ReactNode | |
jumpPrevIcon | specify the default previous icon | ReactNode | (props: PaginationProps) => ReactNode | |
jumpNextIcon | specify the default next icon | ReactNode | (props: PaginationProps) => ReactNode |
rc-pagination is released under the MIT license.
FAQs
pagination ui component for react
We found that rc-pagination demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.