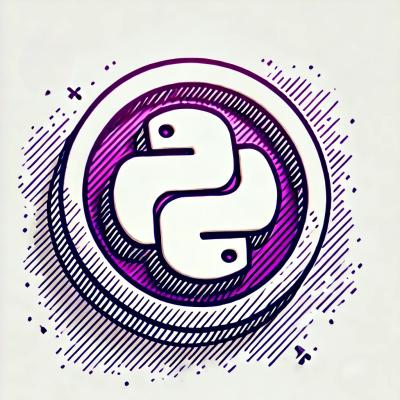
Security News
Introducing the Socket Python SDK
The initial version of the Socket Python SDK is now on PyPI, enabling developers to more easily interact with the Socket REST API in Python projects.
rc-progress
Advanced tools
The rc-progress npm package provides React components for displaying progress information. It supports both line and circle (or pie) progress bars, allowing for customizable colors, sizes, and other properties to visually represent progress in a React application.
Line Progress
This feature allows you to create a linear progress bar. You can customize the percentage of completion, the stroke width, and the color of the progress bar.
import { Line } from 'rc-progress';
<Line percent="70" strokeWidth="4" strokeColor="#D3D3D3" />
Circle Progress
This feature enables the creation of a circular progress bar. Similar to the line progress, you can customize the completion percentage, stroke width, and color.
import { Circle } from 'rc-progress';
<Circle percent="30" strokeWidth="4" strokeColor="#00FF00" />
This package provides a circular progress bar component for React. It is similar to the Circle component of rc-progress but focuses solely on circular progress bars. It offers a different set of customization options and styles.
progressbar.js is a vanilla JavaScript library for creating both line and circle progress bars. It is not React-specific like rc-progress but can be integrated into React projects. It offers a wide range of customization options and animations.
nprogress is a lightweight JavaScript library for creating slim progress bars, typically used at the top of the page to indicate loading progress. Unlike rc-progress, it is not React-specific and does not offer circular progress bars, focusing instead on a minimalistic approach.
Progress Bar.
https://progress.react-component.vercel.app/
import { Line, Circle } from 'rc-progress';
export default () => (
<>
<Line percent={10} strokeWidth={4} strokeColor="#D3D3D3" />
<Circle percent={10} strokeWidth={4} strokeColor="#D3D3D3" />
</>
);
![]() IE / Edge | ![]() Firefox | ![]() Chrome | ![]() Safari | ![]() Electron |
---|---|---|---|---|
IE11, Edge | last 2 versions | last 2 versions | last 2 versions | last 2 versions |
name | type | default | description |
---|---|---|---|
strokeWidth | Number | 1 | Width of the stroke. Unit is percentage of SVG canvas size. |
strokeColor | String | #2db7f5 | Stroke color. |
trailWidth | Number | 1 | Width of the trail stroke. Unit is percentage of SVG canvas size. Trail is always centered relative to actual progress path. If trailWidth is not defined, it is the same as strokeWidth. |
trailColor | String | #D9D9D9 | Color for lighter trail stroke underneath the actual progress path. |
strokeLinecap | String | 'round' | The shape to be used at the end of the progress bar: can be `butt`, `square` or `round`. |
prefixCls | String | rc-progress | prefix className for component |
className | String | customized className | |
style | Object | style object will be added to svg element | |
percent | Number | Number[] | 0 | the percent of the progress |
gapDegree | Number | 0 | the gap degree of half circle, 0 - 360 |
gapPosition | String | top | the gap position: can be `top`, `bottom`, `left`, or `right`. |
npm install --save rc-progress
npm install
npm start
rc-progress is released under the MIT license.
FAQs
progress ui component for react
The npm package rc-progress receives a total of 1,201,704 weekly downloads. As such, rc-progress popularity was classified as popular.
We found that rc-progress demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 7 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The initial version of the Socket Python SDK is now on PyPI, enabling developers to more easily interact with the Socket REST API in Python projects.
Security News
Floating dependency ranges in npm can introduce instability and security risks into your project by allowing unverified or incompatible versions to be installed automatically, leading to unpredictable behavior and potential conflicts.
Security News
A new Rust RFC proposes "Trusted Publishing" for Crates.io, introducing short-lived access tokens via OIDC to improve security and reduce risks associated with long-lived API tokens.