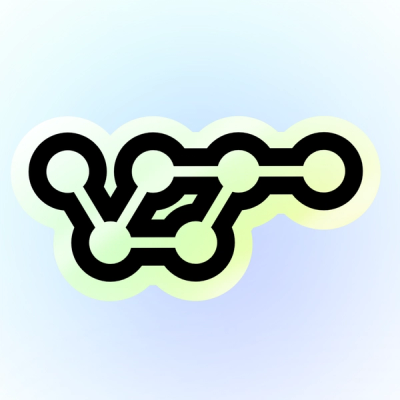
Security News
vlt Debuts New JavaScript Package Manager and Serverless Registry at NodeConf EU
vlt introduced its new package manager and a serverless registry this week, innovating in a space where npm has stagnated.
Bindings for RE2: fast, safe alternative to backtracking regular expression engines.
The re2 npm package is a wrapper around Google's RE2 regular expression library, which is designed to be fast and safe. It provides a way to perform regular expression operations without the risk of catastrophic backtracking, which can occur with some other regular expression engines.
Basic Matching
This feature allows you to perform basic matching operations using regular expressions. The code sample demonstrates how to create a new RE2 instance with a pattern and test a string against it.
const RE2 = require('re2');
const re = new RE2('hello');
console.log(re.test('hello world')); // true
Capturing Groups
This feature allows you to use capturing groups in your regular expressions. The code sample shows how to capture groups of digits separated by a hyphen and access the captured groups.
const RE2 = require('re2');
const re = new RE2('(\d+)-(\d+)');
const match = re.exec('123-456');
console.log(match[1]); // 123
console.log(match[2]); // 456
Global Matching
This feature allows you to perform global matching to find all occurrences of a pattern in a string. The code sample demonstrates how to find all sequences of digits in a string.
const RE2 = require('re2');
const re = new RE2('\d+', 'g');
const matches = '123 456 789'.match(re);
console.log(matches); // ['123', '456', '789']
Replacing
This feature allows you to replace parts of a string that match a pattern with a replacement string. The code sample shows how to replace 'world' with 'RE2' in a given string.
const RE2 = require('re2');
const re = new RE2('world');
const result = 'hello world'.replace(re, 'RE2');
console.log(result); // 'hello RE2'
The 'regexp' package provides a simple interface for working with regular expressions in JavaScript. It is similar to re2 but does not offer the same level of performance and safety guarantees against catastrophic backtracking.
The 'xregexp' package extends JavaScript's native RegExp with additional features and syntax. It offers more functionality than re2 but may not be as performant or safe in terms of avoiding catastrophic backtracking.
The 'pcre-to-regexp' package allows you to convert Perl-compatible regular expressions (PCRE) to JavaScript RegExp objects. While it provides compatibility with PCRE syntax, it does not offer the same performance and safety benefits as re2.
This project provides bindings for RE2: fast, safe alternative to backtracking regular expression engines written by Russ Cox. To learn more about RE2, start with an overview Regular Expression Matching in the Wild. More resources can be found at his Implementing Regular Expressions page.
RE2's regular expression language is almost a superset of what is provided by RegExp
(see Syntax),
but it lacks one feature: backreferences. See below for more details.
RE2
object emulates standard RegExp
making it a practical drop-in replacement in most cases.
RE2
is extended to provide String
-based regular expression methods as well. To help converting
RegExp
objects to RE2
its constructor can take RegExp
directly honoring all properties.
It can work with node.js buffers directly reducing overhead on recoding and copying characters, and making processing/parsing long files fast.
RE2
object can be created just like RegExp
:
Supported properties:
Supported methods:
RE2
object can be created from a regular expression:
var re1 = new RE2(/ab*/ig); // from a RegExp object
var re2 = new RE2(re1); // from another RE2 object
String
methodsStandard String
defines four more methods that can use regular expressions. RE2
provides them as methods
exchanging positions of a string, and a regular expression:
re2.match(str)
re2.replace(str, newSubStr|function)
re2.search(str)
re2.split(str[, limit])
Buffer
supportIn order to support Buffer
directly, most methods can accept buffers instead of strings. It speeds up all operations.
Following signatures are supported:
re2.exec(buf)
re2.test(buf)
re2.match(buf)
re2.search(buf)
re2.split(buf[, limit])
re2.replace(buf, replacer)
Differences with their string-based versions:
Buffer
objects, even in composite objects. A buffer can be converted to a string with
buf.toString()
.When re2.replace()
is used with a replacer function, the replacer can return a buffer, or a string. But all arguments
(except for an input object) will be strings, and an offset will be in characters. If you prefer to deal
with buffers and byte offsets in a replacer function, set a property useBuffers
to true
on the function:
function strReplacer(match, offset, input) {
// typeof match == "string"
return "<= " + offset + " characters|";
}
RE2("б").replace("абв", strReplacer);
// "а<= 1 characters|в"
function bufReplacer(match, offset, input) {
// typeof match == "string"
return "<= " + offset + " bytes|";
}
bufReplacer.useBuffers = true;
RE2("б").replace("абв", bufReplacer);
// "а<= 2 bytes|в"
This feature works for string and buffer inputs. If a buffer was used as an input, its output will be returned as a buffer too, otherwise a string will be returned.
Two functions to calculate string sizes between
UTF-8 and
UTF-16 are exposed on RE2
:
RE2.getUtf8Length(str)
— calculates a buffer size in bytes to encode a UTF-16 string as
a UTF-8 buffer.RE2.getUtf16Length(buf)
— calculates a string size in characters to encode a UTF-8 buffer as
a UTF-16 string.JavaScript supports UCS-2 strings with 16-bit characters, while node.js 0.11 supports full UTF-16 as a default string.
Installation:
npm install re2
It is used just like a RegExp
object.
var RE2 = require("re2");
// with default flags
var re = new RE2("a(b*)");
var result = re.exec("abbc");
console.log(result[0]); // "abb"
console.log(result[1]); // "bb"
result = re.exec("aBbC");
console.log(result[0]); // "a"
console.log(result[1]); // ""
// with explicit flags
re = new RE2("a(b*)", "i");
result = re.exec("aBbC");
console.log(result[0]); // "aBb"
console.log(result[1]); // "Bb"
// from regular expression object
var regexp = new RegExp("a(b*)", "i");
re = new RE2(regexp);
result = re.exec("aBbC");
console.log(result[0]); // "aBb"
console.log(result[1]); // "Bb"
// from regular expression literal
re = new RE2(/a(b*)/i);
result = re.exec("aBbC");
console.log(result[0]); // "aBb"
console.log(result[1]); // "Bb"
// from another RE2 object
var rex = new RE2(re);
result = rex.exec("aBbC");
console.log(result[0]); // "aBb"
console.log(result[1]); // "Bb"
// shortcut
result = new RE2("ab*").exec("abba");
// factory
result = RE2("ab*").exec("abba");
Unlike RegExp
, RE2
doesn't support backreferences, which are numbered references to previously
matched groups, like so: \1
, \2
, and so on. Example of backrefrences:
/(cat|dog)\1/.test("catcat"); // true
/(cat|dog)\1/.test("dogdog"); // true
/(cat|dog)\1/.test("catdog"); // false
/(cat|dog)\1/.test("dogcat"); // false
If your application uses this kind of matching, you should continue to use RegExp
.
\c
and \u
commands.RegExp
methods, and all relevant String
methodsFAQs
Bindings for RE2: fast, safe alternative to backtracking regular expression engines.
The npm package re2 receives a total of 654,633 weekly downloads. As such, re2 popularity was classified as popular.
We found that re2 demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt introduced its new package manager and a serverless registry this week, innovating in a space where npm has stagnated.
Security News
Research
The Socket Research Team uncovered a malicious Python package typosquatting the popular 'fabric' SSH library, silently exfiltrating AWS credentials from unsuspecting developers.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.