React-ArcGIS

React-ArcGIS is a library of React components which use the ArcGIS API for JavaScript. React-ArcGIS uses esri-loader internally to load and interact with the AMD ArcGIS API for JavaScript, and provide a base for building mapping applications.
Installation:
- Run
npm i react-arcgis
(if you decide you like it, you can even include --save
)
Version 3.2.0:
- You can now include loader options directly in react-arcgis components. This provides an easy way to migrate to esri's newer async/await compatible promises in your react-arcgis application. For example:
render() {
return (
<Map
loaderOptions={{
dojoConfig: {
has: {
"esri-promise-compatibility": 1
}
}
}}
/>
);
}
Basic Usage:
Don't forget to load the js api stylesheet! https://js.arcgis.com/4.6/esri/css/main.css
If you need to support browsers lacking a native promise implementation, you will have to add a global Promise
constructor polyfill to your project, as react-arcgis does not include one. I recommend es6-promise.
Render a simple map in React:
import * as React from 'react';
import * as ReactDOM from 'react-dom';
import { Map } from 'react-arcgis';
ReactDOM.render(
<Map />,
document.getElementById('container')
);
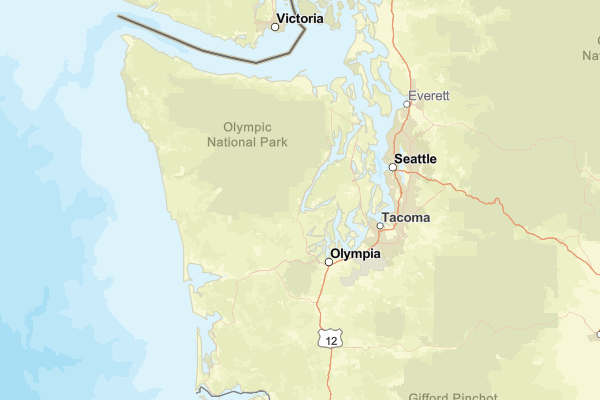
Or, render a 3D web-scene:
import * as ReactDOM from 'react-dom';
import { Scene } from 'react-arcgis';
ReactDOM.render(
<Scene />,
document.getElementById('container')
);
You can also add webmaps and webscenes from ArcGIS Online:
import * as React from 'react';
import * as ReactDOM from 'react-dom';
import { WebMap, WebScene } from 'react-arcgis';
ReactDOM.render(
<div style={{ width: '100vw', height: '100vh' }}>
<WebMap id="6627e1dd5f594160ac60f9dfc411673f" />
<WebScene id="f8aa0c25485a40a1ada1e4b600522681" />
</div>,
document.getElementById('container')
);
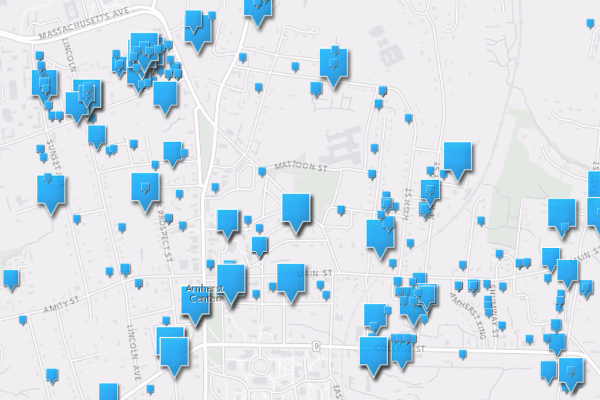
If you want to change the style of the Map
or Scene
, just give it a class:
import * as React from 'react';
import * as ReactDOM from 'react-dom';
import { Scene } from 'react-arcgis';
ReactDOM.render(
<Scene className="full-screen-map" />,
document.getElementById('container')
);
You can also pass properties into the Map
, MapView
, or SceneView
via the viewProperties or mapProperties props:
import * as React from 'react';
import { Map } from 'react-arcgis';
export default (props) => (
<Map
class="full-screen-map"
mapProperties={{ basemap: 'satellite' }}
/>
)
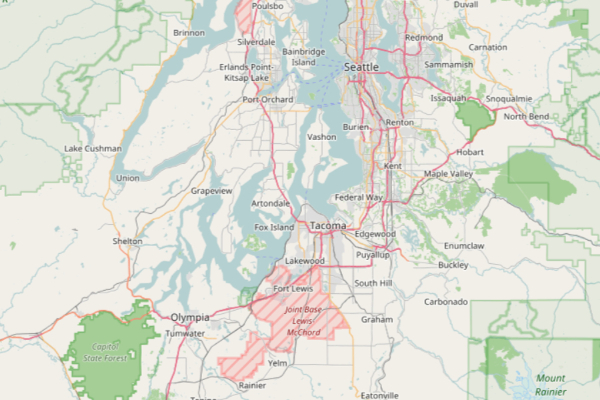
These properties are passed directly to the available properties on the corresponding ArcGIS API classes:
import * as React from 'react';
import { Scene } from 'react-arcgis';
export default (props) => (
<Scene
style={{ width: '100vw', height: '100vh' }}
mapProperties={{ basemap: 'satellite' }}
viewProperties={{
center: [-122.4443, 47.2529],
zoom: 6
}}
/>
)
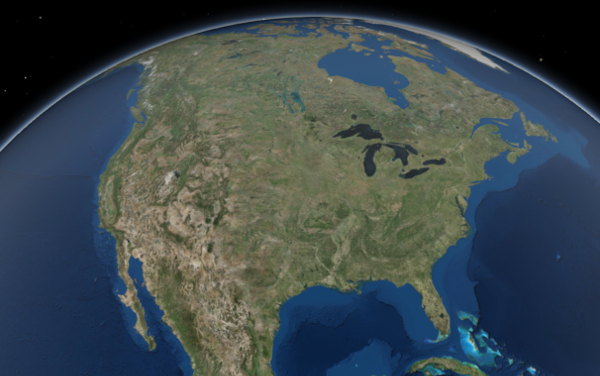
If you want to access the map
and view
instances directly after they are loaded, pass in an onLoad
handler:
import * as React from 'react';
import { Map } from 'react-arcgis';
export default class MakeAMap extends React.Component {
constructor(props) {
super(props);
this.state = {
map: null,
view: null
};
this.handleMapLoad = this.handleMapLoad.bind(this)
}
render() {
return <Map className="full-screen-map" onLoad={this.handleMapLoad} />;
}
handleMapLoad(map, view) {
this.setState({ map, view });
}
}
Don't forget an onFail
handler in case something goes wrong:
import * as React from 'react';
import { WebScene } from 'react-arcgis';
export default class MakeAScene extends React.Component {
constructor(props) {
super(props);
this.state = {
status: 'loading'
};
this.handleFail = this.handleFail.bind(this);
}
render() {
return <WebScene className="full-screen-map" id="foobar" onFail={this.handleFail} />;
}
handleFail(e) {
console.error(e);
this.setState({ status: 'failed' });
}
}
"Advanced" Usage:
The functionality available through the ArcGIS API for JavaScript goes well beyond just rendering maps, and if your application needs to do more with the map than simply show it, you will quickly find that you need access to the rest of Esri's API.
React-arcgis provides the children of <Map />
, <Scene />
, <WebMap />
, and <WebScene />
with access to their parent's map
and view
instances through props. Combined with esriPromise
, we can use this to easily get other functionality from the ArcGIS JS API and use it within our react application.
For example, let's convert a Bermuda Triangle graphic from this example into a react component:
import * as React from 'react';
import { esriPromise } from 'react-arcgis';
export default class BermudaTriangle extends React.Component {
constructor(props) {
super(props);
this.state = {
graphic: null
};
}
render() {
return null;
}
componentWillMount() {
esriPromise(['esri/Graphic']).then(([ Graphic ]) => {
const polygon = {
type: "polygon",
rings: [
[-64.78, 32.3],
[-66.07, 18.45],
[-80.21, 25.78],
[-64.78, 32.3]
]
};
const fillSymbol = {
type: "simple-fill",
color: [227, 139, 79, 0.8],
outline: {
color: [255, 255, 255],
width: 1
}
};
const graphic = new Graphic({
geometry: polygon,
symbol: fillSymbol
});
this.setState({ graphic });
this.props.view.graphics.add(graphic);
})).catch((err) => console.error(err));
}
componentWillUnmount() {
this.props.view.graphics.remove(this.state.graphic);
}
}
Now we can use the <BermudaTriangle />
component within our <Map />
, <Scene />
, <WebMap />
, or <WebScene />
, and the map
and view
props will automatically be supplied by react-arcgis:
import * as React from 'react';
import { Scene } from 'react-arcgis';
import BermudaTriangle from './BermudaTriangle';
export default (props) => (
<Scene class="full-screen-map">
<BermudaTriangle />
</Scene>
)
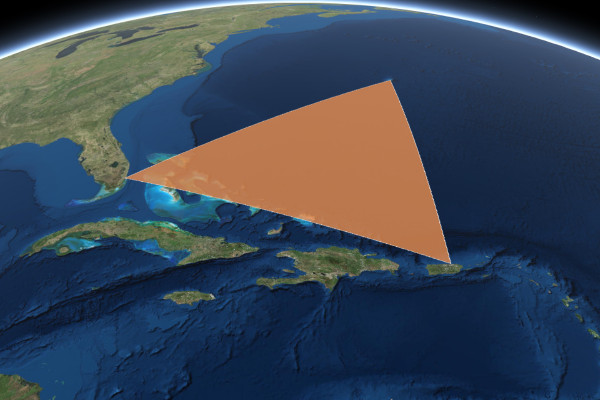
Contributions
Anyone is welcome to contribute to this package. My only "rule" is that your contribution must either pass the existing unit tests, or include additional unit tests to cover new functionality.
Here are some commands that may be helpful for development:
npm test
: Runs the unit testsnpm run build
: Builds the application
License
MIT