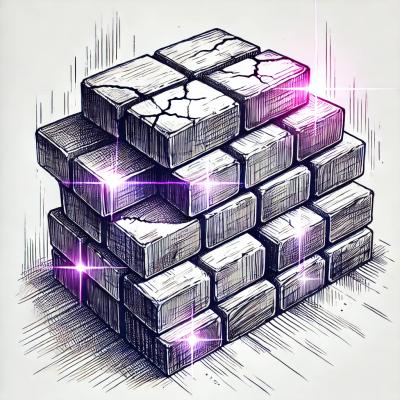
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
react-cookie
Advanced tools
The react-cookie package is a library for handling cookies in React applications. It provides hooks and components to easily set, get, and remove cookies, making it simple to manage user sessions and preferences.
Setting a Cookie
This feature allows you to set a cookie using the `useCookies` hook. In this example, a cookie named 'user' is set with the value 'John Doe' when the login button is clicked.
import { useCookies } from 'react-cookie';
function App() {
const [cookies, setCookie] = useCookies(['user']);
const handleLogin = () => {
setCookie('user', 'John Doe', { path: '/' });
};
return (
<div>
<button onClick={handleLogin}>Login</button>
</div>
);
}
Getting a Cookie
This feature allows you to get a cookie using the `useCookies` hook. In this example, the value of the 'user' cookie is displayed in a paragraph.
import { useCookies } from 'react-cookie';
function App() {
const [cookies] = useCookies(['user']);
return (
<div>
<p>User: {cookies.user}</p>
</div>
);
}
Removing a Cookie
This feature allows you to remove a cookie using the `useCookies` hook. In this example, the 'user' cookie is removed when the logout button is clicked.
import { useCookies } from 'react-cookie';
function App() {
const [cookies, setCookie, removeCookie] = useCookies(['user']);
const handleLogout = () => {
removeCookie('user', { path: '/' });
};
return (
<div>
<button onClick={handleLogout}>Logout</button>
</div>
);
}
js-cookie is a simple, lightweight JavaScript API for handling cookies. It is not specific to React but can be used in any JavaScript project. Compared to react-cookie, js-cookie requires more manual handling of cookies but offers more flexibility for non-React environments.
universal-cookie is a library for handling cookies in both client and server environments. It provides a consistent API for managing cookies in JavaScript and is often used in conjunction with server-side rendering frameworks. Compared to react-cookie, universal-cookie is more versatile for isomorphic applications.
react-use-cookie is a React hook for managing cookies. It is similar to react-cookie but offers a simpler API with fewer features. It is suitable for projects that need basic cookie management without the additional functionality provided by react-cookie.
Universal cookies for React
universal-cookie
- Universal cookies for JavaScriptuniversal-cookie-express
- Hook cookies get/set on Express for server-renderingnpm install react-cookie
<CookiesProvider />
Set the user cookies
On the server, the cookies
props must be set using req.universalCookies
or new Cookie(cookieHeader)
withCookies(Component)
Give access to your cookies anywhere. Add the following props to your component:
get(name, [options])
Get a cookie value
getAll([options])
Get all cookies
set(name, value, [options])
Set a cookie value
/
as the path if you want your cookie to be accessible on all pagesremove(name, [options])
Remove a cookie
/
as the path if you want your cookie to be accessible on all pages// Root.jsx
import React from 'react';
import App from './App';
import { CookiesProvider } from 'react-cookie';
export default function Root() {
return (
<CookiesProvider>
<App />
</CookiesProvider>
);
}
// App.jsx
import React, { Component } from 'react';
import { instanceOf } from 'prop-types';
import { withCookies, Cookies } from 'react-cookie';
import NameForm from './NameForm';
class App extends Component {
static propTypes = {
cookies: instanceOf(Cookies).isRequired
};
constructor(props) {
super(props);
const { cookies } = props;
this.state = {
name: cookies.get('name') || 'Ben'
};
}
handleNameChange(name) {
const { cookies } = this.props;
cookies.set('name', name, { path: '/' });
this.setState({ name });
}
render() {
const { name } = this.state;
return (
<div>
<NameForm name={name} onChange={this.handleNameChange.bind(this)} />
{this.state.name && <h1>Hello {this.state.name}!</h1>}
</div>
);
}
}
export default withCookies(App);
// src/components/App.js
import React, { Component } from 'react';
import { instanceOf } from 'prop-types';
import { withCookies, Cookies } from 'react-cookie';
import NameForm from './NameForm';
class App extends Component {
static propTypes = {
cookies: instanceOf(Cookies).isRequired
};
constructor(props) {
super(props);
const { cookies } = props;
this.state = {
name: cookies.get('name') || 'Ben'
};
}
handleNameChange(name) {
const { cookies } = this.props;
cookies.set('name', name, { path: '/' });
this.setState({ name });
}
render() {
const { name } = this.state;
return (
<div>
<NameForm name={name} onChange={this.handleNameChange.bind(this)} />
{this.state.name && <h1>Hello {this.state.name}!</h1>}
</div>
);
}
}
export default withCookies(App);
// src/server.js
import React from 'react';
import ReactDOMServer from 'react-dom/server';
import { CookiesProvider } from 'react-cookie';
import Html from './components/Html';
import App from './components/App';
export default function middleware(req, res) {
const markup = ReactDOMServer.renderToString(
<CookiesProvider cookies={req.universalCookies}>
<App />
</CookiesProvider>
);
const html = ReactDOMServer.renderToStaticMarkup(<Html markup={markup} />);
res.send('<!DOCTYPE html>' + html);
}
// src/client.js
import React from 'react';
import ReactDOM from 'react-dom';
import { CookiesProvider } from 'react-cookie';
import App from './components/App';
const appEl = document.getElementById('main-app');
ReactDOM.render(
<CookiesProvider>
<App />
</CookiesProvider>,
appEl
);
// server.js
require('@babel/register');
const express = require('express');
const serverMiddleware = require('./src/server').default;
const cookiesMiddleware = require('universal-cookie-express');
const app = express();
app
.use('/assets', express.static('dist'))
.use(cookiesMiddleware())
.use(serverMiddleware);
app.listen(8080, function() {
console.log('Listening on 8080...'); // eslint-disable-line no-console
});
FAQs
Universal cookies for React
The npm package react-cookie receives a total of 497,088 weekly downloads. As such, react-cookie popularity was classified as popular.
We found that react-cookie demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.