react-countup
Advanced tools
Comparing version 4.0.0-alpha.6 to 4.0.0
@@ -11,28 +11,110 @@ 'use strict'; | ||
var _createClass = function () { function defineProperties(target, props) { for (var i = 0; i < props.length; i++) { var descriptor = props[i]; descriptor.enumerable = descriptor.enumerable || false; descriptor.configurable = true; if ("value" in descriptor) descriptor.writable = true; Object.defineProperty(target, descriptor.key, descriptor); } } return function (Constructor, protoProps, staticProps) { if (protoProps) defineProperties(Constructor.prototype, protoProps); if (staticProps) defineProperties(Constructor, staticProps); return Constructor; }; }(); | ||
function _classCallCheck(instance, Constructor) { | ||
if (!(instance instanceof Constructor)) { | ||
throw new TypeError("Cannot call a class as a function"); | ||
} | ||
} | ||
function _classCallCheck(instance, Constructor) { if (!(instance instanceof Constructor)) { throw new TypeError("Cannot call a class as a function"); } } | ||
function _defineProperties(target, props) { | ||
for (var i = 0; i < props.length; i++) { | ||
var descriptor = props[i]; | ||
descriptor.enumerable = descriptor.enumerable || false; | ||
descriptor.configurable = true; | ||
if ("value" in descriptor) descriptor.writable = true; | ||
Object.defineProperty(target, descriptor.key, descriptor); | ||
} | ||
} | ||
function _possibleConstructorReturn(self, call) { if (!self) { throw new ReferenceError("this hasn't been initialised - super() hasn't been called"); } return call && (typeof call === "object" || typeof call === "function") ? call : self; } | ||
function _createClass(Constructor, protoProps, staticProps) { | ||
if (protoProps) _defineProperties(Constructor.prototype, protoProps); | ||
if (staticProps) _defineProperties(Constructor, staticProps); | ||
return Constructor; | ||
} | ||
function _inherits(subClass, superClass) { if (typeof superClass !== "function" && superClass !== null) { throw new TypeError("Super expression must either be null or a function, not " + typeof superClass); } subClass.prototype = Object.create(superClass && superClass.prototype, { constructor: { value: subClass, enumerable: false, writable: true, configurable: true } }); if (superClass) Object.setPrototypeOf ? Object.setPrototypeOf(subClass, superClass) : subClass.__proto__ = superClass; } | ||
function _defineProperty(obj, key, value) { | ||
if (key in obj) { | ||
Object.defineProperty(obj, key, { | ||
value: value, | ||
enumerable: true, | ||
configurable: true, | ||
writable: true | ||
}); | ||
} else { | ||
obj[key] = value; | ||
} | ||
var CountUp = function (_Component) { | ||
return obj; | ||
} | ||
function _inherits(subClass, superClass) { | ||
if (typeof superClass !== "function" && superClass !== null) { | ||
throw new TypeError("Super expression must either be null or a function"); | ||
} | ||
subClass.prototype = Object.create(superClass && superClass.prototype, { | ||
constructor: { | ||
value: subClass, | ||
writable: true, | ||
configurable: true | ||
} | ||
}); | ||
if (superClass) _setPrototypeOf(subClass, superClass); | ||
} | ||
function _getPrototypeOf(o) { | ||
_getPrototypeOf = Object.setPrototypeOf ? Object.getPrototypeOf : function _getPrototypeOf(o) { | ||
return o.__proto__ || Object.getPrototypeOf(o); | ||
}; | ||
return _getPrototypeOf(o); | ||
} | ||
function _setPrototypeOf(o, p) { | ||
_setPrototypeOf = Object.setPrototypeOf || function _setPrototypeOf(o, p) { | ||
o.__proto__ = p; | ||
return o; | ||
}; | ||
return _setPrototypeOf(o, p); | ||
} | ||
function _assertThisInitialized(self) { | ||
if (self === void 0) { | ||
throw new ReferenceError("this hasn't been initialised - super() hasn't been called"); | ||
} | ||
return self; | ||
} | ||
function _possibleConstructorReturn(self, call) { | ||
if (call && (typeof call === "object" || typeof call === "function")) { | ||
return call; | ||
} | ||
return _assertThisInitialized(self); | ||
} | ||
var CountUp = | ||
/*#__PURE__*/ | ||
function (_Component) { | ||
_inherits(CountUp, _Component); | ||
function CountUp() { | ||
var _ref; | ||
var _getPrototypeOf2; | ||
var _temp, _this, _ret; | ||
var _this; | ||
_classCallCheck(this, CountUp); | ||
for (var _len = arguments.length, args = Array(_len), _key = 0; _key < _len; _key++) { | ||
for (var _len = arguments.length, args = new Array(_len), _key = 0; _key < _len; _key++) { | ||
args[_key] = arguments[_key]; | ||
} | ||
return _ret = (_temp = (_this = _possibleConstructorReturn(this, (_ref = CountUp.__proto__ || Object.getPrototypeOf(CountUp)).call.apply(_ref, [this].concat(args))), _this), _this.createInstance = function () { | ||
// Warn when user didn't use containerRef at all | ||
warning(_this.containerRef.current && _this.containerRef.current instanceof HTMLElement, 'Couldn\'t find attached element to hook the CountUp instance into! Try to attach "containerRef" from the render prop to a an HTMLElement, eg. <span ref={containerRef} />.'); | ||
_this = _possibleConstructorReturn(this, (_getPrototypeOf2 = _getPrototypeOf(CountUp)).call.apply(_getPrototypeOf2, [this].concat(args))); | ||
_defineProperty(_assertThisInitialized(_assertThisInitialized(_this)), "createInstance", function () { | ||
if (typeof _this.props.children === 'function') { | ||
// Warn when user didn't use containerRef at all | ||
warning(_this.containerRef.current && _this.containerRef.current instanceof HTMLElement, "Couldn't find attached element to hook the CountUp instance into! Try to attach \"containerRef\" from the render prop to a an HTMLElement, eg. <span ref={containerRef} />."); | ||
} | ||
var _this$props = _this.props, | ||
@@ -50,4 +132,2 @@ decimal = _this$props.decimal, | ||
useEasing = _this$props.useEasing; | ||
return new Count(_this.containerRef.current, start, end, decimals, duration, { | ||
@@ -63,33 +143,51 @@ decimal: decimal, | ||
}); | ||
}, _this.pauseResume = function () { | ||
var _this2 = _this, | ||
reset = _this2.reset, | ||
start = _this2.restart, | ||
update = _this2.update; | ||
}); | ||
_defineProperty(_assertThisInitialized(_assertThisInitialized(_this)), "pauseResume", function () { | ||
var _assertThisInitialize = _assertThisInitialized(_assertThisInitialized(_this)), | ||
reset = _assertThisInitialize.reset, | ||
start = _assertThisInitialize.restart, | ||
update = _assertThisInitialize.update; | ||
var onPauseResume = _this.props.onPauseResume; | ||
_this.instance.pauseResume(); | ||
onPauseResume({ reset: reset, start: start, update: update }); | ||
}, _this.reset = function () { | ||
var _this3 = _this, | ||
pauseResume = _this3.pauseResume, | ||
start = _this3.restart, | ||
update = _this3.update; | ||
onPauseResume({ | ||
reset: reset, | ||
start: start, | ||
update: update | ||
}); | ||
}); | ||
_defineProperty(_assertThisInitialized(_assertThisInitialized(_this)), "reset", function () { | ||
var _assertThisInitialize2 = _assertThisInitialized(_assertThisInitialized(_this)), | ||
pauseResume = _assertThisInitialize2.pauseResume, | ||
start = _assertThisInitialize2.restart, | ||
update = _assertThisInitialize2.update; | ||
var onReset = _this.props.onReset; | ||
_this.instance.reset(); | ||
onReset({ pauseResume: pauseResume, start: start, update: update }); | ||
}, _this.restart = function () { | ||
onReset({ | ||
pauseResume: pauseResume, | ||
start: start, | ||
update: update | ||
}); | ||
}); | ||
_defineProperty(_assertThisInitialized(_assertThisInitialized(_this)), "restart", function () { | ||
_this.reset(); | ||
_this.start(); | ||
}, _this.start = function () { | ||
var _this4 = _this, | ||
pauseResume = _this4.pauseResume, | ||
reset = _this4.reset, | ||
start = _this4.restart, | ||
update = _this4.update; | ||
}); | ||
_defineProperty(_assertThisInitialized(_assertThisInitialized(_this)), "start", function () { | ||
var _assertThisInitialize3 = _assertThisInitialized(_assertThisInitialized(_this)), | ||
pauseResume = _assertThisInitialize3.pauseResume, | ||
reset = _assertThisInitialize3.reset, | ||
start = _assertThisInitialize3.restart, | ||
update = _assertThisInitialize3.update; | ||
var _this$props2 = _this.props, | ||
@@ -102,7 +200,12 @@ delay = _this$props2.delay, | ||
return _this.instance.start(function () { | ||
return onEnd({ pauseResume: pauseResume, reset: reset, start: start, update: update }); | ||
return onEnd({ | ||
pauseResume: pauseResume, | ||
reset: reset, | ||
start: start, | ||
update: update | ||
}); | ||
}); | ||
}; | ||
}; // Delay start if delay prop is properly set | ||
// Delay start if delay prop is properly set | ||
if (delay > 0) { | ||
@@ -114,41 +217,51 @@ setTimeout(run, delay * 1000); | ||
onStart({ pauseResume: pauseResume, reset: reset, update: update }); | ||
}, _this.update = function (newEnd) { | ||
var _this5 = _this, | ||
pauseResume = _this5.pauseResume, | ||
reset = _this5.reset, | ||
start = _this5.restart; | ||
onStart({ | ||
pauseResume: pauseResume, | ||
reset: reset, | ||
update: update | ||
}); | ||
}); | ||
_defineProperty(_assertThisInitialized(_assertThisInitialized(_this)), "update", function (newEnd) { | ||
var _assertThisInitialize4 = _assertThisInitialized(_assertThisInitialized(_this)), | ||
pauseResume = _assertThisInitialize4.pauseResume, | ||
reset = _assertThisInitialize4.reset, | ||
start = _assertThisInitialize4.restart; | ||
var onUpdate = _this.props.onUpdate; | ||
_this.instance.update(newEnd); | ||
onUpdate({ pauseResume: pauseResume, reset: reset, start: start }); | ||
}, _this.containerRef = React__default.createRef(), _temp), _possibleConstructorReturn(_this, _ret); | ||
onUpdate({ | ||
pauseResume: pauseResume, | ||
reset: reset, | ||
start: start | ||
}); | ||
}); | ||
_defineProperty(_assertThisInitialized(_assertThisInitialized(_this)), "containerRef", React__default.createRef()); | ||
return _this; | ||
} | ||
_createClass(CountUp, [{ | ||
key: 'componentDidMount', | ||
key: "componentDidMount", | ||
value: function componentDidMount() { | ||
var _props = this.props, | ||
children = _props.children, | ||
delay = _props.delay; | ||
var _this$props3 = this.props, | ||
children = _this$props3.children, | ||
delay = _this$props3.delay; | ||
this.instance = this.createInstance(); // Don't invoke start if component is used as a render prop | ||
this.instance = this.createInstance(); | ||
if (typeof children === 'function' && delay !== 0) return; // Otherwise just start immediately | ||
// Don't invoke start if component is used as a render prop | ||
if (typeof children === 'function' && delay !== 0) return; | ||
// Otherwise just start immediately | ||
this.start(); | ||
} | ||
}, { | ||
key: 'shouldComponentUpdate', | ||
key: "shouldComponentUpdate", | ||
value: function shouldComponentUpdate(nextProps) { | ||
var hasCertainPropsChanged = this.props.duration !== nextProps.duration || this.props.end !== nextProps.end || this.props.start !== nextProps.start; | ||
return hasCertainPropsChanged || this.props.redraw; | ||
} | ||
}, { | ||
key: 'componentDidUpdate', | ||
key: "componentDidUpdate", | ||
value: function componentDidUpdate(prevProps) { | ||
@@ -161,6 +274,6 @@ // If duration or start has changed, there's no way to update the duration | ||
this.start(); | ||
} | ||
} // Only end value has changed, so reset and and re-animate with the updated | ||
// end value. | ||
// Only end value has changed, so reset and and re-animate with the updated | ||
// end value. | ||
if (this.props.end !== prevProps.end) { | ||
@@ -172,8 +285,8 @@ this.instance.reset(); | ||
}, { | ||
key: 'render', | ||
key: "render", | ||
value: function render() { | ||
var _props2 = this.props, | ||
children = _props2.children, | ||
className = _props2.className, | ||
style = _props2.style; | ||
var _this$props4 = this.props, | ||
children = _this$props4.children, | ||
className = _this$props4.className, | ||
style = _this$props4.style; | ||
var containerRef = this.containerRef, | ||
@@ -185,3 +298,2 @@ pauseResume = this.pauseResume, | ||
if (typeof children === 'function') { | ||
@@ -197,3 +309,7 @@ return children({ | ||
return React__default.createElement('span', { className: className, ref: containerRef, style: style }); | ||
return React__default.createElement("span", { | ||
className: className, | ||
ref: containerRef, | ||
style: style | ||
}); | ||
} | ||
@@ -205,3 +321,3 @@ }]); | ||
CountUp.propTypes = { | ||
_defineProperty(CountUp, "propTypes", { | ||
decimal: PropTypes.string, | ||
@@ -222,4 +338,5 @@ decimals: PropTypes.number, | ||
useEasing: PropTypes.bool | ||
}; | ||
CountUp.defaultProps = { | ||
}); | ||
_defineProperty(CountUp, "defaultProps", { | ||
decimal: '.', | ||
@@ -243,4 +360,4 @@ decimals: 0, | ||
useEasing: true | ||
}; | ||
}); | ||
module.exports = CountUp; |
{ | ||
"name": "react-countup", | ||
"version": "4.0.0-alpha.6", | ||
"version": "4.0.0", | ||
"description": "A React component wrapper around CountUp.js", | ||
@@ -28,4 +28,3 @@ "author": "Glenn Reyes <glenn@glennreyes.com> (https://twitter.com/glnnrys)", | ||
"build": "rollup -c", | ||
"precommit": "lint-staged", | ||
"prepublishOnly": "yarn build", | ||
"prepublish": "yarn build", | ||
"test": "jest" | ||
@@ -39,30 +38,22 @@ }, | ||
"prop-types": "^15.6.2", | ||
"warning": "^4.0.1" | ||
"warning": "^4.0.2" | ||
}, | ||
"devDependencies": { | ||
"babel-core": "^6.26.3", | ||
"babel-eslint": "^7.2.3", | ||
"babel-plugin-transform-class-properties": "^6.24.1", | ||
"babel-preset-env": "^1.7.0", | ||
"babel-preset-react": "^6.24.1", | ||
"eslint": "^4.19.1", | ||
"eslint-config-prettier": "^2.9.0", | ||
"eslint-config-react-app": "^2.1.0", | ||
"eslint-plugin-flowtype": "^2.50.0", | ||
"eslint-plugin-import": "^2.13.0", | ||
"eslint-plugin-jsx-a11y": "^5.1.1", | ||
"eslint-plugin-prettier": "^2.6.2", | ||
"eslint-plugin-react": "^7.10.0", | ||
"husky": "^0.14.3", | ||
"jest": "^23.5.0", | ||
"lint-staged": "^7.2.0", | ||
"prettier": "^1.14.2", | ||
"@babel/core": "^7.1.2", | ||
"@babel/plugin-proposal-class-properties": "^7.1.0", | ||
"@babel/preset-env": "^7.1.0", | ||
"@babel/preset-react": "^7.0.0", | ||
"babel-core": "^7.0.0-bridge.0", | ||
"babel-jest": "^23.6.0", | ||
"husky": "^1.1.2", | ||
"jest": "^23.6.0", | ||
"prettier": "^1.14.3", | ||
"pretty-quick": "^1.8.0", | ||
"raf": "^3.4.0", | ||
"react": "^16.4.1", | ||
"react-dom": "^16.4.1", | ||
"react-test-renderer": "^16.4.2", | ||
"react-testing-library": "^5.0.0", | ||
"rollup": "^0.64.1", | ||
"rollup-plugin-babel": "^3.0.7" | ||
"react": "^16.6.0", | ||
"react-dom": "^16.6.0", | ||
"react-testing-library": "^5.2.3", | ||
"rollup": "^0.66.6", | ||
"rollup-plugin-babel": "^4.0.3" | ||
} | ||
} |
@@ -12,2 +12,6 @@ # [React CountUp](https://react-countup.now.sh) | ||
### Looking for v3.x docs? | ||
Click [here](https://github.com/glennreyes/react-countup/tree/d0002932dac8a274f951e53b1d9b1f4719176147) to get to the previous docs. | ||
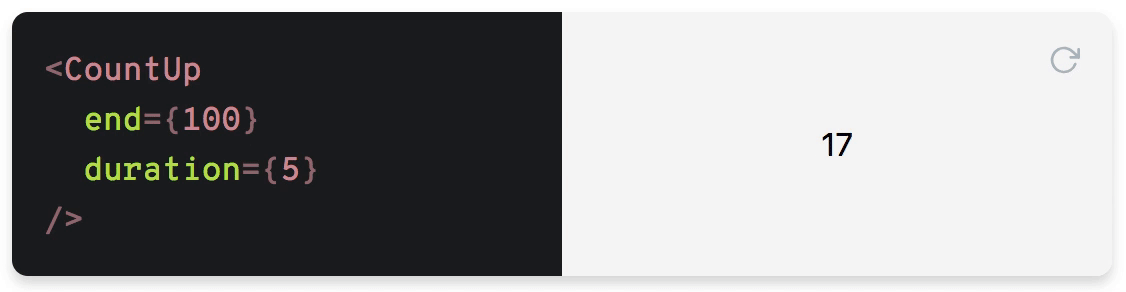 | ||
@@ -124,6 +128,5 @@ | ||
<CountUp start={0} end={100} delay={0}> | ||
{({ countUpRef, start }) => ( | ||
{({ countUpRef }) => ( | ||
<div> | ||
<span ref={countUpRef} /> | ||
<button onClick={start}>Start</button> | ||
</div> | ||
@@ -130,0 +133,0 @@ )} |
@@ -87,8 +87,10 @@ import Count from 'countup.js'; | ||
createInstance = () => { | ||
// Warn when user didn't use containerRef at all | ||
warning( | ||
this.containerRef.current && | ||
this.containerRef.current instanceof HTMLElement, | ||
`Couldn't find attached element to hook the CountUp instance into! Try to attach "containerRef" from the render prop to a an HTMLElement, eg. <span ref={containerRef} />.`, | ||
); | ||
if (typeof this.props.children === 'function') { | ||
// Warn when user didn't use containerRef at all | ||
warning( | ||
this.containerRef.current && | ||
this.containerRef.current instanceof HTMLElement, | ||
`Couldn't find attached element to hook the CountUp instance into! Try to attach "containerRef" from the render prop to a an HTMLElement, eg. <span ref={containerRef} />.`, | ||
); | ||
} | ||
@@ -95,0 +97,0 @@ const { |
No v1
QualityPackage is not semver >=1. This means it is not stable and does not support ^ ranges.
Found 1 instance in 1 package
30203
16
592
1
278
Updatedwarning@^4.0.2