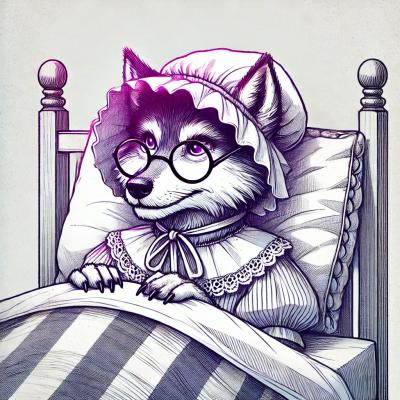
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
react-cropper
Advanced tools
The react-cropper package is a React wrapper for Cropper.js, a JavaScript image cropping library. It allows you to easily integrate image cropping functionality into your React applications.
Basic Image Cropping
This code demonstrates how to set up a basic image cropping component using react-cropper. It includes a reference to the Cropper instance and logs the cropped image data URL to the console.
```jsx
import React, { useRef } from 'react';
import Cropper from 'react-cropper';
import 'cropperjs/dist/cropper.css';
const ImageCropper = () => {
const cropperRef = useRef(null);
const onCrop = () => {
const cropper = cropperRef.current.cropper;
console.log(cropper.getCroppedCanvas().toDataURL());
};
return (
<Cropper
src="https://via.placeholder.com/800x400"
style={{ height: 400, width: '100%' }}
initialAspectRatio={16 / 9}
guides={false}
crop={onCrop}
ref={cropperRef}
/>
);
};
export default ImageCropper;
```
Custom Aspect Ratio
This example shows how to set a custom aspect ratio for the cropping area. In this case, the aspect ratio is set to 4:3.
```jsx
import React, { useRef } from 'react';
import Cropper from 'react-cropper';
import 'cropperjs/dist/cropper.css';
const CustomAspectRatioCropper = () => {
const cropperRef = useRef(null);
const onCrop = () => {
const cropper = cropperRef.current.cropper;
console.log(cropper.getCroppedCanvas().toDataURL());
};
return (
<Cropper
src="https://via.placeholder.com/800x400"
style={{ height: 400, width: '100%' }}
aspectRatio={4 / 3}
guides={false}
crop={onCrop}
ref={cropperRef}
/>
);
};
export default CustomAspectRatioCropper;
```
Cropping with Preview
This example demonstrates how to display a preview of the cropped image. The cropped image data URL is stored in the state and displayed in an <img> element.
```jsx
import React, { useRef, useState } from 'react';
import Cropper from 'react-cropper';
import 'cropperjs/dist/cropper.css';
const CropperWithPreview = () => {
const cropperRef = useRef(null);
const [preview, setPreview] = useState('');
const onCrop = () => {
const cropper = cropperRef.current.cropper;
setPreview(cropper.getCroppedCanvas().toDataURL());
};
return (
<div>
<Cropper
src="https://via.placeholder.com/800x400"
style={{ height: 400, width: '100%' }}
initialAspectRatio={16 / 9}
guides={false}
crop={onCrop}
ref={cropperRef}
/>
<div>
<h3>Preview:</h3>
<img src={preview} alt="Cropped Preview" />
</div>
</div>
);
};
export default CropperWithPreview;
```
react-image-crop is another React component for cropping images. It provides a simple and flexible way to crop images with support for touch devices. Compared to react-cropper, it has a more straightforward API but lacks some advanced features like zooming and rotating.
react-easy-crop is a React component to crop images and videos. It offers a simple and intuitive interface for cropping, with support for touch gestures. It is similar to react-cropper but focuses on ease of use and simplicity, making it a good choice for basic cropping needs.
react-avatar-editor is a React component for cropping and rotating images, particularly useful for profile pictures and avatars. It provides a user-friendly interface for image manipulation. Compared to react-cropper, it is more specialized for avatar editing and includes features like border radius and scale.
Cropperjs as React component
Install via npm
npm install --save react-cropper
You need cropper.css
in your project which is from cropperjs.
Since this project have dependency on cropperjs, it located in /node_modules/react-cropper/node_modules/cropperjs/dist/cropper.css
or node_modules/cropperjs/dist/cropper.css
for npm version 3.0.0
later
ref
has been removed. Use the onInitialized
method to get the cropper
instance.aspectRatio
use initialAspectRatio
.data
, canvasData
and cropBoxData
are directly passed on to cropperjs
and their respective setters are not called as earlier.moveTo
as earlier. Directly use the moveTo
method from the cropper
instance.@types/react-cropper
and provides its own types. Please uninstall/remove @types/react-cropper
as they might 'cause issues.import React, { useRef } from "react";
import Cropper from "react-cropper";
import "cropperjs/dist/cropper.css";
const Demo: React.FC = () => {
const cropperRef = useRef<HTMLImageElement>(null);
const onCrop = () => {
const imageElement: any = cropperRef?.current;
const cropper: any = imageElement?.cropper;
console.log(cropper.getCroppedCanvas().toDataURL());
};
return (
<Cropper
src="https://raw.githubusercontent.com/roadmanfong/react-cropper/master/example/img/child.jpg"
style={{ height: 400, width: "100%" }}
// Cropper.js options
initialAspectRatio={16 / 9}
guides={false}
crop={onCrop}
ref={cropperRef}
/>
);
};
string
null
<Cropper src="http://fengyuanchen.github.io/cropper/images/picture.jpg" />
string
picture
string
null
https://github.com/fengyuanchen/cropperjs#dragmode
https://github.com/fengyuanchen/cropperjs#scalexscalex
https://github.com/fengyuanchen/cropperjs#scalexscaley
https://github.com/fengyuanchen/cropperjs#enable
https://github.com/fengyuanchen/cropperjs#disable
https://github.com/fengyuanchen/cropperjs#zoomto
https://github.com/fengyuanchen/cropperjs#rotateto
Accept all options in the docs as properties.
Use the cropper
instance from onInitialized
to access cropperjs methods
npm run build
npm start
MIT
FAQs
Cropper as React Component
The npm package react-cropper receives a total of 109,109 weekly downloads. As such, react-cropper popularity was classified as popular.
We found that react-cropper demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.