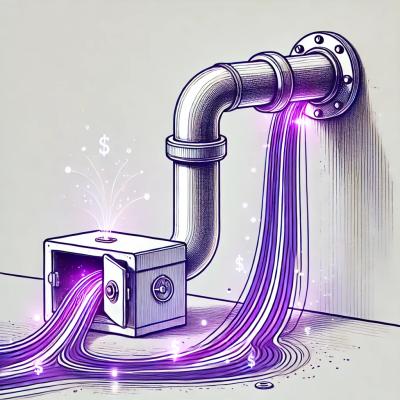
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
react-d3-cloud-modern
Advanced tools
A word cloud react component built with d3-cloud.
Forked from [react-d3-cloud] in order to modernize its dependency on libraries with multiple high-level vulnerabilities.
npm install react-d3-cloud-modern
Simple:
import React from 'react';
import { render } from 'react-dom';
import WordCloud from 'react-d3-cloud-modern';
const data = [
{ text: 'Hey', value: 1000 },
{ text: 'lol', value: 200 },
{ text: 'first impression', value: 800 },
{ text: 'very cool', value: 1000000 },
{ text: 'duck', value: 10 },
];
render(<WordCloud data={data} />, document.getElementById('root'));
More configuration:
import React from 'react';
import { render } from 'react-dom';
import WordCloud from 'react-d3-cloud-modern';
import { scaleOrdinal } from 'd3-scale';
import { schemeCategory10 } from 'd3-scale-chromatic';
const data = [
{ text: 'Hey', value: 1000 },
{ text: 'lol', value: 200 },
{ text: 'first impression', value: 800 },
{ text: 'very cool', value: 1000000 },
{ text: 'duck', value: 10 },
];
const schemeCategory10ScaleOrdinal = scaleOrdinal(schemeCategory10);
render(
<WordCloud
data={data}
width={500}
height={500}
font="Times"
fontStyle="italic"
fontWeight="bold"
fontSize={(word) => Math.log2(word.value) * 5}
spiral="rectangular"
rotate={(word) => word.value % 360}
padding={5}
random={Math.random}
fill={(d, i) => schemeCategory10ScaleOrdinal(i)}
onWordClick={(event, d) => {
console.log(`onWordClick: ${d.text}`);
}}
onWordMouseOver={(event, d) => {
console.log(`onWordMouseOver: ${d.text}`);
}}
onWordMouseOut={(event, d) => {
console.log(`onWordMouseOut: ${d.text}`);
}}
/>,
document.getElementById('root')
);
Please checkout demo
for more detailed props, please refer to below:
name | description | type | required | default |
---|---|---|---|---|
data | The words array | { text: string, value: number }>[] | ✓ | |
width | Width of the layout (px) | number | 700 | |
height | Height of the layout (px) | number | 600 | |
font | The font accessor function, which indicates the font face for each word. A constant may be specified instead of a function. | string | (d) => string | 'serif' | |
fontStyle | The fontStyle accessor function, which indicates the font style for each word. A constant may be specified instead of a function. | string | (d) => string | 'normal' | |
fontWeight | The fontWeight accessor function, which indicates the font weight for each word. A constant may be specified instead of a function. | string | number | (d) => string | number | 'normal' | |
fontSize | The fontSize accessor function, which indicates the numerical font size for each word. | (d) => number | (d) => Math.sqrt(d.value) | |
rotate | The rotate accessor function, which indicates the rotation angle (in degrees) for each word. | (d) => number | () => (~~(Math.random() * 6) - 3) * 30 | |
spiral | The current type of spiral used for positioning words. This can either be one of the two built-in spirals, "archimedean" and "rectangular", or an arbitrary spiral generator can be used | 'archimedean' | 'rectangular' | ([width, height]) => t => [x, y] | 'archimedean' | |
padding | The padding accessor function, which indicates the numerical padding for each word. | number | (d) => number | 1 | |
random | The internal random number generator, used for selecting the initial position of each word, and the clockwise/counterclockwise direction of the spiral for each word. This should return a number in the range [0, 1) . | (d) => number | Math.random | |
fill | The fill accessor function, which indicates the color for each word. | (d, i) => string | (d, i) => schemeCategory10ScaleOrdinal(i) | |
onWordClick | The function will be called when click event is triggered on a word | (event, d) => {} | null | |
onWordMouseOver | The function will be called when mouseover event is triggered on a word | (event, d) => {} | null | |
onWordMouseOut | The function will be called when mouseout event is triggered on a word | (event, d) => {} | null |
To make <WordCloud />
work with Server-Side Rendering (SSR), you need to avoid rendering it on the server:
{
typeof window !== 'undefined' && <WordCloud data={data} />;
}
As of version 0.10.1, <WordCloud />
has been wrapped by React.memo()
and deep equal comparison under the hood to avoid unnecessary re-render. All you need to do is to make your function props deep equal comparable using useCallback()
:
import React, { useCallback } from 'react';
import { render } from 'react-dom';
import WordCloud from 'react-d3-cloud-modern';
import { scaleOrdinal } from 'd3-scale';
import { schemeCategory10 } from 'd3-scale-chromatic';
function App() {
const data = [
{ text: 'Hey', value: 1000 },
{ text: 'lol', value: 200 },
{ text: 'first impression', value: 800 },
{ text: 'very cool', value: 1000000 },
{ text: 'duck', value: 10 },
];
const fontSize = useCallback((word) => Math.log2(word.value) * 5, []);
const rotate = useCallback((word) => word.value % 360, []);
const fill = useCallback((d, i) => scaleOrdinal(schemeCategory10)(i), []);
const onWordClick = useCallback((word) => {
console.log(`onWordClick: ${word}`);
}, []);
const onWordMouseOver = useCallback((word) => {
console.log(`onWordMouseOver: ${word}`);
}, []);
const onWordMouseOut = useCallback((word) => {
console.log(`onWordMouseOut: ${word}`);
}, []);
return (
<WordCloud
data={data}
width={500}
height={500}
font="Times"
fontStyle="italic"
fontWeight="bold"
fontSize={fontSize}
spiral="rectangular"
rotate={rotate}
padding={5}
random={Math.random}
fill={fill}
onWordClick={onWordClick}
onWordMouseOver={onWordMouseOver}
onWordMouseOut={onWordMouseOut}
/>
);
);
npm run build
brew install pkg-config cairo pango libpng jpeg giflib librsvg
npm install
sudo apt-get update
sudo apt-get install libcairo2-dev libjpeg8-dev libpango1.0-dev libgif-dev build-essential g++
npm install
For more details, please check out Installation guides at node-canvas wiki.
npm test
MIT © Yoctol | carlosalvidrez
FAQs
A word cloud component using d3-cloud
The npm package react-d3-cloud-modern receives a total of 15 weekly downloads. As such, react-d3-cloud-modern popularity was classified as not popular.
We found that react-d3-cloud-modern demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.