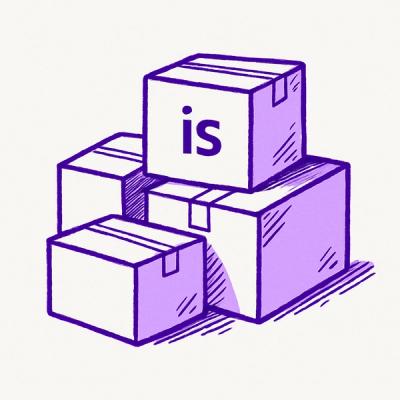
Security News
npm ‘is’ Package Hijacked in Expanding Supply Chain Attack
The ongoing npm phishing campaign escalates as attackers hijack the popular 'is' package, embedding malware in multiple versions.
react-date-picker
Advanced tools
The react-date-picker package is a flexible and customizable date picker component for React applications. It allows users to select dates from a calendar interface and provides various customization options to fit different use cases.
Basic Date Picker
This code demonstrates a basic usage of the react-date-picker component. It initializes a date state and renders a DatePicker component that allows the user to select a date.
import React, { useState } from 'react';
import DatePicker from 'react-date-picker';
function MyApp() {
const [date, setDate] = useState(new Date());
return (
<div>
<DatePicker
onChange={setDate}
value={date}
/>
</div>
);
}
export default MyApp;
Date Range Picker
This code demonstrates how to use react-date-picker to select a date range by using two DatePicker components, one for the start date and one for the end date.
import React, { useState } from 'react';
import DatePicker from 'react-date-picker';
function MyApp() {
const [startDate, setStartDate] = useState(new Date());
const [endDate, setEndDate] = useState(new Date());
return (
<div>
<DatePicker
onChange={setStartDate}
value={startDate}
/>
<DatePicker
onChange={setEndDate}
value={endDate}
/>
</div>
);
}
export default MyApp;
Custom Date Format
This code demonstrates how to customize the date format displayed in the react-date-picker component. The 'format' prop is used to specify the desired date format.
import React, { useState } from 'react';
import DatePicker from 'react-date-picker';
function MyApp() {
const [date, setDate] = useState(new Date());
return (
<div>
<DatePicker
onChange={setDate}
value={date}
format="y-MM-dd"
/>
</div>
);
}
export default MyApp;
Disable Specific Dates
This code demonstrates how to disable specific dates in the react-date-picker component. The 'tileDisabled' prop is used to specify which dates should be disabled.
import React, { useState } from 'react';
import DatePicker from 'react-date-picker';
function MyApp() {
const [date, setDate] = useState(new Date());
const disableDates = [
new Date(2023, 9, 10),
new Date(2023, 9, 15)
];
return (
<div>
<DatePicker
onChange={setDate}
value={date}
tileDisabled={({ date }) => disableDates.some(disabledDate => date.getTime() === disabledDate.getTime())}
/>
</div>
);
}
export default MyApp;
react-datepicker is another popular date picker component for React. It offers a wide range of features including date and time selection, custom date formats, and localization support. Compared to react-date-picker, react-datepicker provides more advanced features such as time selection and inline display.
react-dates is a date picker component developed by Airbnb. It is highly customizable and supports date ranges, single date selection, and internationalization. It is known for its accessibility and mobile-friendly design. Compared to react-date-picker, react-dates offers more comprehensive support for date ranges and better accessibility features.
rc-calendar is a date picker component from the rc-components library. It provides a flexible and customizable calendar interface with support for date and time selection. Compared to react-date-picker, rc-calendar offers more customization options and supports both date and time selection.
A date picker for your React app.
npm install react-date-picker
or yarn add react-date-picker
.import DatePicker from 'react-date-picker'
.<DatePicker />
. Use onChange
prop for getting new values.A minimal demo page can be found in sample
directory.
Online demo is also available!
If you don't need to support legacy browsers and don't need the advanced features this package provides, consider using native date input instead. It's more accessible, adds no extra weight to your bundle, and works better on mobile devices.
<input aria-label="Date" type="date" />
React-Date-Picker will play nicely with React-Time-Picker and React-DateTime-Picker. Check them out!
Your project needs to use React 16.3 or later. If you use an older version of React, please refer to the table below to find a suitable React-Date-Picker version.
React version | Newest compatible React-Date-Picker version |
---|---|
≥16.3 | latest |
≥16.0 | 7.x |
React-Calendar, on which React-Date-Picker relies heavily, uses modern web technologies. That's why it's so fast, lightweight and easy to style. This, however, comes at a cost of supporting only modern browsers.
If you need to support legacy browsers like Internet Explorer 10, you will need to use Intl.js or another Intl polyfill along with React-Date-Picker.
Add React-Date-Picker to your project by executing npm install react-date-picker
or yarn add react-date-picker
.
Here's an example of basic usage:
import { useState } from 'react';
import DatePicker from 'react-date-picker';
type ValuePiece = Date | null;
type Value = ValuePiece | [ValuePiece, ValuePiece];
function MyApp() {
const [value, onChange] = useState<Value>(new Date());
return (
<div>
<DatePicker onChange={onChange} value={value} />
</div>
);
}
If you want to use default React-Date-Picker and React-Calendar styling to build upon it, you can import them by using:
import 'react-date-picker/dist/DatePicker.css';
import 'react-calendar/dist/Calendar.css';
Displays an input field complete with custom inputs, native input, and a calendar.
Prop name | Description | Default value | Example values |
---|---|---|---|
autoFocus | Automatically focuses the input on mount. | n/a | true |
calendarAriaLabel | aria-label for the calendar button. | n/a | "Toggle calendar" |
calendarProps | Props to pass to React-Calendar component. | n/a | See React-Calendar documentation |
calendarIcon | Content of the calendar button. Setting the value explicitly to null will hide the icon. | (default icon) |
|
className | Class name(s) that will be added along with "react-date-picker" to the main React-Date-Picker <div> element. | n/a |
|
clearAriaLabel | aria-label for the clear button. | n/a | "Clear value" |
clearIcon | Content of the clear button. Setting the value explicitly to null will hide the icon. | (default icon) |
|
closeCalendar | Whether to close the calendar on value selection. Note: It's recommended to use shouldCloseCalendar function instead. | true | false |
data-testid | data-testid attribute for the main React-Date-Picker <div> element. | n/a | "date-picker" |
dayAriaLabel | aria-label for the day input. | n/a | "Day" |
dayPlaceholder | placeholder for the day input. | "--" | "dd" |
disableCalendar | When set to true , will remove the calendar and the button toggling its visibility. | false | true |
disabled | Whether the date picker should be disabled. | false | true |
format | Input format based on Unicode Technical Standard #35. Supported values are: y , M , MM , MMM , MMMM , d , dd . Note: When using SSR, setting this prop may help resolving hydration errors caused by locale mismatch between server and client. | n/a | "y-MM-dd" |
id | id attribute for the main React-Date-Picker <div> element. | n/a | "date-picker" |
isOpen | Whether the calendar should be opened. | false | true |
locale | Locale that should be used by the date picker and the calendar. Can be any IETF language tag. Note: When using SSR, setting this prop may help resolving hydration errors caused by locale mismatch between server and client. | Server locale/User's browser settings | "hu-HU" |
maxDate | Maximum date that the user can select. Periods partially overlapped by maxDate will also be selectable, although React-Date-Picker will ensure that no later date is selected. | n/a | Date: new Date() |
maxDetail | The most detailed calendar view that the user shall see. View defined here also becomes the one on which clicking an item in the calendar will select a date and pass it to onChange. Can be "month" , "year" , "decade" or "century" . | "month" | "year" |
minDate | Minimum date that the user can select. Periods partially overlapped by minDate will also be selectable, although React-Date-Picker will ensure that no earlier date is selected. | n/a | Date: new Date() |
monthAriaLabel | aria-label for the month input. | n/a | "Month" |
monthPlaceholder | placeholder for the month input. | "--" | "mm" |
name | Input name. | "date" | "myCustomName" |
nativeInputAriaLabel | aria-label for the native date input. | n/a | "Date" |
onCalendarClose | Function called when the calendar closes. | n/a | () => alert('Calendar closed') |
onCalendarOpen | Function called when the calendar opens. | n/a | () => alert('Calendar opened') |
onChange | Function called when the user picks a valid date. If any of the fields were excluded using custom format , new Date(y, 0, 1, 0, 0, 0) , where y is the current year, is going to serve as a "base". | n/a | (value) => alert('New date is: ', value) |
onFocus | Function called when the user focuses an input. | n/a | (event) => alert('Focused input: ', event.target.name) |
onInvalidChange | Function called when the user picks an invalid date. | n/a | () => alert('Invalid date') |
openCalendarOnFocus | Whether to open the calendar on input focus. Note: It's recommended to use shouldOpenCalendar function instead. | true | false |
portalContainer | Element to render the calendar in using portal. | n/a | document.getElementById('my-div') |
required | Whether date input should be required. | false | true |
returnValue | Which dates shall be passed by the calendar to the onChange function and onClick{Period} functions. Can be "start" , "end" or "range" . The latter will cause an array with start and end values to be passed. | "start" | "range" |
shouldCloseCalendar | Function called before the calendar closes. reason can be "buttonClick" , "escape" , "outsideAction" , or "select" . If it returns false , the calendar will not close. | n/a | ({ reason }) => reason !== 'outsideAction' |
shouldOpenCalendar | Function called before the calendar opens. reason can be "buttonClick" or "focus" . If it returns false , the calendar will not open. | n/a | ({ reason }) => reason !== 'focus' |
showLeadingZeros | Whether leading zeros should be rendered in date inputs. | false | true |
value | Input value. Note that if you pass an array of values, only first value will be fully utilized. | n/a |
|
yearAriaLabel | aria-label for the year input. | n/a | "Year" |
yearPlaceholder | aria-label for the year input. | "----" | "yyyy" |
DatePicker component passes all props to React-Calendar, with the exception of className
(you can use calendarClassName
for that instead). There are tons of customizations you can do! For more information, see Calendar component props.
The MIT License.
| Wojciech Maj |
Thank you to all our sponsors! Become a sponsor and get your image on our README on GitHub.
Thank you to all our backers! Become a backer and get your image on our README on GitHub.
Thank you to all our contributors that helped on this project!
FAQs
A date picker for your React app.
The npm package react-date-picker receives a total of 192,968 weekly downloads. As such, react-date-picker popularity was classified as popular.
We found that react-date-picker demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The ongoing npm phishing campaign escalates as attackers hijack the popular 'is' package, embedding malware in multiple versions.
Security News
A critical flaw in the popular npm form-data package could allow HTTP parameter pollution, affecting millions of projects until patched versions are adopted.
Security News
Bun 1.2.19 introduces isolated installs for smoother monorepo workflows, along with performance boosts, new tooling, and key compatibility fixes.