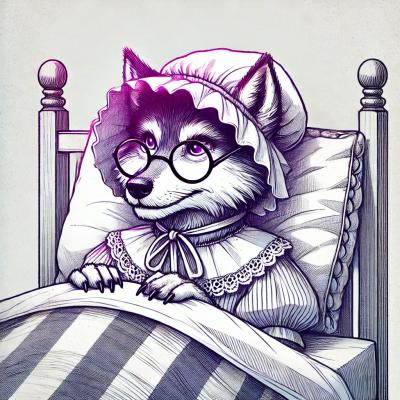
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
react-date-picker
Advanced tools
The react-date-picker package is a flexible and customizable date picker component for React applications. It allows users to select dates from a calendar interface and provides various customization options to fit different use cases.
Basic Date Picker
This code demonstrates a basic usage of the react-date-picker component. It initializes a date state and renders a DatePicker component that allows the user to select a date.
import React, { useState } from 'react';
import DatePicker from 'react-date-picker';
function MyApp() {
const [date, setDate] = useState(new Date());
return (
<div>
<DatePicker
onChange={setDate}
value={date}
/>
</div>
);
}
export default MyApp;
Date Range Picker
This code demonstrates how to use react-date-picker to select a date range by using two DatePicker components, one for the start date and one for the end date.
import React, { useState } from 'react';
import DatePicker from 'react-date-picker';
function MyApp() {
const [startDate, setStartDate] = useState(new Date());
const [endDate, setEndDate] = useState(new Date());
return (
<div>
<DatePicker
onChange={setStartDate}
value={startDate}
/>
<DatePicker
onChange={setEndDate}
value={endDate}
/>
</div>
);
}
export default MyApp;
Custom Date Format
This code demonstrates how to customize the date format displayed in the react-date-picker component. The 'format' prop is used to specify the desired date format.
import React, { useState } from 'react';
import DatePicker from 'react-date-picker';
function MyApp() {
const [date, setDate] = useState(new Date());
return (
<div>
<DatePicker
onChange={setDate}
value={date}
format="y-MM-dd"
/>
</div>
);
}
export default MyApp;
Disable Specific Dates
This code demonstrates how to disable specific dates in the react-date-picker component. The 'tileDisabled' prop is used to specify which dates should be disabled.
import React, { useState } from 'react';
import DatePicker from 'react-date-picker';
function MyApp() {
const [date, setDate] = useState(new Date());
const disableDates = [
new Date(2023, 9, 10),
new Date(2023, 9, 15)
];
return (
<div>
<DatePicker
onChange={setDate}
value={date}
tileDisabled={({ date }) => disableDates.some(disabledDate => date.getTime() === disabledDate.getTime())}
/>
</div>
);
}
export default MyApp;
react-datepicker is another popular date picker component for React. It offers a wide range of features including date and time selection, custom date formats, and localization support. Compared to react-date-picker, react-datepicker provides more advanced features such as time selection and inline display.
react-dates is a date picker component developed by Airbnb. It is highly customizable and supports date ranges, single date selection, and internationalization. It is known for its accessibility and mobile-friendly design. Compared to react-date-picker, react-dates offers more comprehensive support for date ranges and better accessibility features.
rc-calendar is a date picker component from the rc-components library. It provides a flexible and customizable calendar interface with support for date and time selection. Compared to react-date-picker, rc-calendar offers more customization options and supports both date and time selection.
Date picker for React
See demo at jslog.com/react-date-picker
$ npm install react-date-picker
Don't forget to include index.css or index.styl! ( require('react-date-picker/index.css') )
Also you need to have React
included in the page.
The preferred React version for react-date-picker
is >=0.12. The initial version of react-date-picker
worked with React 0.11.2 as well, but I do not intend to continue to support it, in order to be able to focus on advancing the current features and developing other high-quality React components.
var date = '2014-10-10' //or Date.now()
function onChange(moment, dateString){
//...
}
<DatePicker
minDate='2014-04-04'
maxDate='2015-10-10'
date={date}
onChange={onChange}
/>
react-date-picker
uses the awesome moment.js
library ( Big thanks!)
If you don't use npm you can include any of the following:
dist/react-color-picker.js
- the full sources. NOTE: You'll need to include React
separatelydist/react-color-picker.min.js
- minified & optimized version. NOTE: You'll need to include React
separatelydist/react-color-picker.nomoment.js
- the full sources. NOTE: You'll need to include React
AND moment.js
separatelydist/react-color-picker.nomoment.min.js
- minified & optimized version. NOTE: You'll need to include React
AND moment.js
separatelyFAQs
A date picker for your React app.
The npm package react-date-picker receives a total of 148,205 weekly downloads. As such, react-date-picker popularity was classified as popular.
We found that react-date-picker demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.