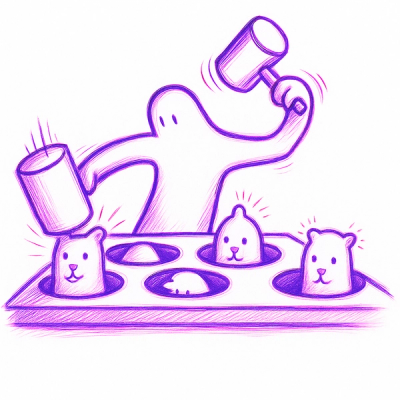
Research
/Security News
Contagious Interview Campaign Escalates With 67 Malicious npm Packages and New Malware Loader
North Korean threat actors deploy 67 malicious npm packages using the newly discovered XORIndex malware loader.
react-dynamodb-helper
Advanced tools
A helper component to interface with dynamodb using the AWS SDK, directly from React
A helper component to interface with dynamodb using the AWS SDK, directly from React
npm install --save react-dynamodb-helper
Then install the dependencies.
npm install --save aws-sdk
You will need to create table(s) in dynamodb according to your data model.
AWS region, secret and access key form the credentials. These are required to use this package. It is crucial that these credentials are given create, retrieve, update and delete permissions in aws for dynamodb.
You can now review the functionality below.
/*
region: aws region
secret: aws secret
key: aws access key
params: query parameters
*/
getData(region, secret, key, params) {}
queryData(region, secret, key, params) {}
scanData(region, secret, key, params) {}
putData(region, secret, key, params) {}
updateData(region, secret, key, params) {}
deleteData(region, secret, key, params) {}
import React, { useEffect } from 'react'
import * as DynamoDB from 'react-dynamodb-helper';
const App = () => {
useEffect(() => {
async function getData() {
var params = {
TableName: "Account_Credentials",
Key : {
"email" : 'hru****@***ies.com',
}
};
// aws_secret and aws_access_key need to have
// dynamodb access
let result = await DynamoDB.getData("aws_region", "aws_secret", "aws_access_key", params)
}
getData();
}, [])
useEffect(() => {
async function updateData() {
var params = {
TableName: "Account_Credentials",
Key : {
"email" : 'hru****@***ies.com',
},
UpdateExpression: "set #otp = :otpVal",
ExpressionAttributeNames: {
"#otp": "otp",
},
ExpressionAttributeValues: {
":otpVal": "1212"
}
};
// aws_secret and aws_access_key need to have
// dynamodb access
let result = await DynamoDB.updateData("aws_region", "aws_secret", "aws_access_key", params)
}
getData();
}, [])
return <div>Hello DynamoDB Helper</div>
}
export default App
Some part of this module is based on the work done by Ninad Thatte & team at MeGo Technologies.
MIT © superflows-dev
FAQs
A helper component to interface with dynamodb using the AWS SDK, directly from React
The npm package react-dynamodb-helper receives a total of 1 weekly downloads. As such, react-dynamodb-helper popularity was classified as not popular.
We found that react-dynamodb-helper demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
North Korean threat actors deploy 67 malicious npm packages using the newly discovered XORIndex malware loader.
Security News
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.