react-image-pan-zoom-rotate
Advanced tools
Comparing version 1.5.0 to 1.6.0
/// <reference types="react" /> | ||
declare type PanViewerProps = { | ||
import PanViewer from './PanViewer'; | ||
declare type ReactPanZoomProps = { | ||
image: string; | ||
@@ -7,3 +8,4 @@ alt?: string; | ||
}; | ||
declare const PanViewer: ({ image, alt, ref }: PanViewerProps) => JSX.Element; | ||
export default PanViewer; | ||
declare const ReactPanZoom: ({ image, alt, ref }: ReactPanZoomProps) => JSX.Element; | ||
export { PanViewer }; | ||
export default ReactPanZoom; |
@@ -1,509 +0,8 @@ | ||
var React = require('react'); | ||
function _inheritsLoose(subClass, superClass) { | ||
subClass.prototype = Object.create(superClass.prototype); | ||
subClass.prototype.constructor = subClass; | ||
'use strict' | ||
_setPrototypeOf(subClass, superClass); | ||
if (process.env.NODE_ENV === 'production') { | ||
module.exports = require('./react-image-pan-zoom-rotate.cjs.production.min.js') | ||
} else { | ||
module.exports = require('./react-image-pan-zoom-rotate.cjs.development.js') | ||
} | ||
function _setPrototypeOf(o, p) { | ||
_setPrototypeOf = Object.setPrototypeOf || function _setPrototypeOf(o, p) { | ||
o.__proto__ = p; | ||
return o; | ||
}; | ||
return _setPrototypeOf(o, p); | ||
} | ||
var ReactPanZoom = /*#__PURE__*/function (_React$PureComponent) { | ||
_inheritsLoose(ReactPanZoom, _React$PureComponent); | ||
function ReactPanZoom() { | ||
var _this; | ||
_this = _React$PureComponent.apply(this, arguments) || this; | ||
_this.getInitialState = function () { | ||
var _this$props = _this.props, | ||
pandx = _this$props.pandx, | ||
pandy = _this$props.pandy, | ||
zoom = _this$props.zoom; | ||
var defaultDragData = { | ||
dx: pandx, | ||
dy: pandy, | ||
x: 0, | ||
y: 0 | ||
}; | ||
return { | ||
comesFromDragging: false, | ||
dragData: defaultDragData, | ||
dragging: false, | ||
matrixData: [zoom, 0, 0, zoom, pandx, pandy], | ||
mouseDown: false | ||
}; | ||
}; | ||
_this.state = _this.getInitialState(); | ||
_this.reset = function () { | ||
var matrixData = [0.4, 0, 0, 0.4, 0, 0]; | ||
_this.setState({ | ||
matrixData: matrixData | ||
}); | ||
if (_this.props.onReset) { | ||
_this.props.onReset(0, 0, 1); | ||
} | ||
}; | ||
_this.onClick = function (e) { | ||
if (_this.state.comesFromDragging) { | ||
return; | ||
} | ||
if (_this.props.onClick) { | ||
_this.props.onClick(e); | ||
} | ||
}; | ||
_this.onTouchStart = function (e) { | ||
var _e$touches$ = e.touches[0], | ||
pageX = _e$touches$.pageX, | ||
pageY = _e$touches$.pageY; | ||
_this.panStart(pageX, pageY, e); | ||
}; | ||
_this.onTouchEnd = function () { | ||
_this.onMouseUp(); | ||
}; | ||
_this.onTouchMove = function (e) { | ||
_this.updateMousePosition(e.touches[0].pageX, e.touches[0].pageY); | ||
}; | ||
_this.onMouseDown = function (e) { | ||
_this.panStart(e.pageX, e.pageY, e); | ||
}; | ||
_this.panStart = function (pageX, pageY, event) { | ||
if (!_this.props.enablePan) { | ||
return; | ||
} | ||
var matrixData = _this.state.matrixData; | ||
var offsetX = matrixData[4]; | ||
var offsetY = matrixData[5]; | ||
var newDragData = { | ||
dx: offsetX, | ||
dy: offsetY, | ||
x: pageX, | ||
y: pageY | ||
}; | ||
_this.setState({ | ||
dragData: newDragData, | ||
mouseDown: true | ||
}); | ||
if (_this.panWrapper) { | ||
_this.panWrapper.style.cursor = 'move'; | ||
} | ||
event.stopPropagation(); | ||
event.nativeEvent.stopImmediatePropagation(); | ||
event.preventDefault(); | ||
}; | ||
_this.onMouseUp = function () { | ||
_this.panEnd(); | ||
}; | ||
_this.panEnd = function () { | ||
_this.setState({ | ||
comesFromDragging: _this.state.dragging, | ||
dragging: false, | ||
mouseDown: false | ||
}); | ||
if (_this.panWrapper) { | ||
_this.panWrapper.style.cursor = ''; | ||
} | ||
if (_this.props.onPan) { | ||
_this.props.onPan(_this.state.matrixData[4], _this.state.matrixData[5]); | ||
} | ||
}; | ||
_this.onMouseMove = function (e) { | ||
_this.updateMousePosition(e.pageX, e.pageY); | ||
}; | ||
_this.onWheel = function (e) { | ||
Math.sign(e.deltaY) < 0 ? _this.props.setZoom((_this.props.zoom || 0) + 0.1) : (_this.props.zoom || 0) > 1 && _this.props.setZoom((_this.props.zoom || 0) - 0.1); | ||
}; | ||
_this.onMouseEnter = function () { | ||
document.addEventListener('wheel', _this.preventDefault, { | ||
passive: false | ||
}); | ||
}; | ||
_this.onMouseLeave = function () { | ||
document.removeEventListener('wheel', _this.preventDefault, false); | ||
}; | ||
_this.updateMousePosition = function (pageX, pageY) { | ||
if (!_this.state.mouseDown) return; | ||
var matrixData = _this.getNewMatrixData(pageX, pageY); | ||
_this.setState({ | ||
dragging: true, | ||
matrixData: matrixData | ||
}); | ||
if (_this.panContainer) { | ||
_this.panContainer.style.transform = "matrix(" + _this.state.matrixData.toString() + ")"; | ||
} | ||
}; | ||
_this.getNewMatrixData = function (x, y) { | ||
var _this$state = _this.state, | ||
dragData = _this$state.dragData, | ||
matrixData = _this$state.matrixData; | ||
var deltaX = dragData.x - x; | ||
var deltaY = dragData.y - y; | ||
matrixData[4] = dragData.dx - deltaX; | ||
matrixData[5] = dragData.dy - deltaY; | ||
return matrixData; | ||
}; | ||
return _this; | ||
} | ||
var _proto = ReactPanZoom.prototype; | ||
_proto.componentWillReceiveProps = function componentWillReceiveProps(nextProps) { | ||
var matrixData = this.state.matrixData; | ||
if (matrixData[0] !== nextProps.zoom) { | ||
var newMatrixData = [].concat(this.state.matrixData); | ||
newMatrixData[0] = nextProps.zoom || newMatrixData[0]; | ||
newMatrixData[3] = nextProps.zoom || newMatrixData[3]; | ||
this.setState({ | ||
matrixData: newMatrixData | ||
}); | ||
} | ||
}; | ||
_proto.render = function render() { | ||
var _this2 = this; | ||
return React.createElement("div", { | ||
className: "pan-container " + (this.props.className || ''), | ||
onMouseDown: this.onMouseDown, | ||
onMouseUp: this.onMouseUp, | ||
onTouchStart: this.onTouchStart, | ||
onTouchMove: this.onTouchMove, | ||
onTouchEnd: this.onTouchEnd, | ||
onMouseMove: this.onMouseMove, | ||
onWheel: this.onWheel, | ||
onMouseEnter: this.onMouseEnter, | ||
onMouseLeave: this.onMouseLeave, | ||
onClick: this.onClick, | ||
style: { | ||
height: this.props.height, | ||
userSelect: 'none', | ||
width: this.props.width | ||
}, | ||
ref: function ref(_ref) { | ||
return _this2.panWrapper = _ref; | ||
} | ||
}, React.createElement("div", { | ||
ref: function ref(_ref2) { | ||
return _ref2 ? _this2.panContainer = _ref2 : null; | ||
}, | ||
style: { | ||
transform: "matrix(" + this.state.matrixData.toString() + ")" | ||
} | ||
}, this.props.children)); | ||
}; | ||
_proto.preventDefault = function preventDefault(e) { | ||
e = e || window.event; | ||
if (e.preventDefault) { | ||
e.preventDefault(); | ||
} | ||
e.returnValue = false; | ||
}; | ||
return ReactPanZoom; | ||
}(React.PureComponent); | ||
ReactPanZoom.defaultProps = { | ||
enablePan: true, | ||
onPan: function onPan() { | ||
return undefined; | ||
}, | ||
onReset: function onReset() { | ||
return undefined; | ||
}, | ||
pandx: 0, | ||
pandy: 0, | ||
zoom: 0, | ||
rotation: 0 | ||
}; | ||
var PanViewer = function PanViewer(_ref) { | ||
var image = _ref.image, | ||
alt = _ref.alt, | ||
ref = _ref.ref; | ||
var _React$useState = React.useState(0), | ||
dx = _React$useState[0], | ||
setDx = _React$useState[1]; | ||
var _React$useState2 = React.useState(0), | ||
dy = _React$useState2[0], | ||
setDy = _React$useState2[1]; | ||
var _React$useState3 = React.useState(1), | ||
zoom = _React$useState3[0], | ||
setZoom = _React$useState3[1]; | ||
var _React$useState4 = React.useState(0), | ||
rotation = _React$useState4[0], | ||
setRotation = _React$useState4[1]; | ||
var _React$useState5 = React.useState(false), | ||
flip = _React$useState5[0], | ||
setFlip = _React$useState5[1]; | ||
var resetAll = function resetAll() { | ||
setDx(0); | ||
setDy(0); | ||
setZoom(1); | ||
setRotation(0); | ||
setFlip(false); | ||
}; | ||
var zoomIn = function zoomIn() { | ||
setZoom(zoom + 0.2); | ||
}; | ||
var zoomOut = function zoomOut() { | ||
if (zoom >= 1) { | ||
setZoom(zoom - 0.2); | ||
} | ||
}; | ||
var rotateLeft = function rotateLeft() { | ||
if (rotation === -3) { | ||
setRotation(0); | ||
} else { | ||
setRotation(rotation - 1); | ||
} | ||
}; | ||
var flipImage = function flipImage() { | ||
setFlip(!flip); | ||
}; | ||
var onPan = function onPan(dx, dy) { | ||
setDx(dx); | ||
setDy(dy); | ||
}; | ||
return React.createElement("div", null, React.createElement("div", { | ||
style: { | ||
position: 'absolute', | ||
right: '10px', | ||
zIndex: 2, | ||
top: 10, | ||
userSelect: 'none', | ||
borderRadius: 2, | ||
background: '#fff', | ||
boxShadow: '0px 2px 6px rgba(53, 67, 93, 0.32)' | ||
} | ||
}, React.createElement("div", { | ||
onClick: zoomIn, | ||
style: { | ||
textAlign: 'center', | ||
cursor: 'pointer', | ||
height: 40, | ||
width: 40, | ||
borderBottom: ' 1px solid #ccc' | ||
} | ||
}, React.createElement("svg", { | ||
style: { | ||
height: '100%', | ||
width: '100%', | ||
padding: 10, | ||
boxSizing: 'border-box' | ||
}, | ||
width: '24', | ||
height: '24', | ||
viewBox: '0 0 24 24', | ||
fill: 'none', | ||
xmlns: 'http://www.w3.org/2000/svg' | ||
}, React.createElement("path", { | ||
d: 'M4 12H20', | ||
stroke: '#4C68C1', | ||
strokeWidth: '2', | ||
strokeLinecap: 'round' | ||
}), React.createElement("path", { | ||
d: 'M12 4L12 20', | ||
stroke: '#4C68C1', | ||
strokeWidth: '2', | ||
strokeLinecap: 'round' | ||
}))), React.createElement("div", { | ||
onClick: zoomOut, | ||
style: { | ||
textAlign: 'center', | ||
cursor: 'pointer', | ||
height: 40, | ||
width: 40, | ||
borderBottom: ' 1px solid #ccc' | ||
} | ||
}, React.createElement("svg", { | ||
style: { | ||
height: '100%', | ||
width: '100%', | ||
padding: 10, | ||
boxSizing: 'border-box' | ||
}, | ||
width: '24', | ||
height: '24', | ||
viewBox: '0 0 24 24', | ||
fill: 'none', | ||
xmlns: 'http://www.w3.org/2000/svg' | ||
}, React.createElement("path", { | ||
d: 'M4 12H20', | ||
stroke: '#4C68C1', | ||
strokeWidth: '2', | ||
strokeLinecap: 'round' | ||
}))), React.createElement("div", { | ||
onClick: rotateLeft, | ||
style: { | ||
textAlign: 'center', | ||
cursor: 'pointer', | ||
height: 40, | ||
width: 40, | ||
borderBottom: ' 1px solid #ccc' | ||
} | ||
}, React.createElement("svg", { | ||
style: { | ||
height: '100%', | ||
width: '100%', | ||
padding: 10, | ||
boxSizing: 'border-box' | ||
}, | ||
width: '24', | ||
height: '24', | ||
viewBox: '0 0 24 24', | ||
fill: 'none', | ||
xmlns: 'http://www.w3.org/2000/svg' | ||
}, React.createElement("path", { | ||
d: 'M14 15L9 20L4 15', | ||
stroke: '#4C68C1', | ||
strokeWidth: '2', | ||
strokeLinecap: 'round', | ||
strokeLinejoin: 'round' | ||
}), React.createElement("path", { | ||
d: 'M20 4H13C10.7909 4 9 5.79086 9 8V20', | ||
stroke: '#4C68C1', | ||
strokeWidth: '2', | ||
strokeLinecap: 'round', | ||
strokeLinejoin: 'round' | ||
}))), React.createElement("div", { | ||
onClick: flipImage, | ||
style: { | ||
textAlign: 'center', | ||
cursor: 'pointer', | ||
height: 40, | ||
width: 40, | ||
borderBottom: ' 1px solid #ccc' | ||
} | ||
}, React.createElement("svg", { | ||
style: { | ||
height: '100%', | ||
width: '100%', | ||
padding: 10, | ||
boxSizing: 'border-box' | ||
}, | ||
width: '24', | ||
height: '24', | ||
viewBox: '0 0 24 24', | ||
fill: 'none', | ||
xmlns: 'http://www.w3.org/2000/svg' | ||
}, React.createElement("path", { | ||
stroke: '#4C68C1', | ||
strokeWidth: '2', | ||
strokeLinecap: 'round', | ||
strokeLinejoin: 'round', | ||
d: 'M9.178,18.799V1.763L0,18.799H9.178z M8.517,18.136h-7.41l7.41-13.752V18.136z' | ||
}), React.createElement("polygon", { | ||
stroke: '#4C68C1', | ||
strokeWidth: '2', | ||
strokeLinecap: 'round', | ||
strokeLinejoin: 'round', | ||
points: '11.385,1.763 11.385,18.799 20.562,18.799 ' | ||
}))), React.createElement("div", { | ||
onClick: resetAll, | ||
style: { | ||
textAlign: 'center', | ||
cursor: 'pointer', | ||
height: 40, | ||
width: 40 | ||
} | ||
}, React.createElement("svg", { | ||
style: { | ||
height: '100%', | ||
width: '100%', | ||
padding: 10, | ||
boxSizing: 'border-box' | ||
}, | ||
width: '24', | ||
height: '24', | ||
viewBox: '0 0 24 24', | ||
fill: 'none', | ||
xmlns: 'http://www.w3.org/2000/svg', | ||
stroke: '#4C68C1', | ||
strokeWidth: 2, | ||
strokeLinecap: 'round', | ||
strokeLinejoin: 'round' | ||
}, React.createElement("path", { | ||
d: 'M15 3h6v6M9 21H3v-6M21 3l-7 7M3 21l7-7' | ||
})))), React.createElement(ReactPanZoom, { | ||
style: { | ||
width: '100%', | ||
height: '100%', | ||
display: 'flex', | ||
justifyContent: 'center', | ||
alignItems: 'center', | ||
zIndex: 1 | ||
}, | ||
zoom: zoom, | ||
setZoom: setZoom, | ||
pandx: dx, | ||
pandy: dy, | ||
onPan: onPan, | ||
rotation: rotation, | ||
key: dx | ||
}, React.createElement("img", { | ||
style: { | ||
transform: "rotate(" + rotation * 90 + "deg) scaleX(" + (flip ? -1 : 1) + ")", | ||
width: '100%' | ||
}, | ||
src: image, | ||
alt: alt, | ||
ref: ref | ||
}))); | ||
}; | ||
module.exports = PanViewer; | ||
//# sourceMappingURL=index.js.map |
@@ -1,8 +0,56 @@ | ||
/// <reference types="react" /> | ||
declare type PanViewerProps = { | ||
image: string; | ||
alt?: string; | ||
ref?: any; | ||
}; | ||
declare const PanViewer: ({ image, alt, ref }: PanViewerProps) => JSX.Element; | ||
export default PanViewer; | ||
import * as React from 'react'; | ||
export interface IDragData { | ||
x: number; | ||
y: number; | ||
dx: number; | ||
dy: number; | ||
} | ||
export interface IReactPanZoomStateType { | ||
dragging: boolean; | ||
mouseDown: boolean; | ||
comesFromDragging: boolean; | ||
dragData: IDragData; | ||
matrixData: number[]; | ||
} | ||
export interface IReactPanZoomProps { | ||
height?: string; | ||
width?: string; | ||
className?: string; | ||
enablePan?: boolean; | ||
reset?: () => void; | ||
zoom?: number; | ||
pandx?: number; | ||
pandy?: number; | ||
rotation?: number; | ||
onPan?: (x: number, y: number) => void; | ||
setZoom: (z: number) => void; | ||
onReset?: (dx: number, dy: number, zoom: number) => void; | ||
onClick?: (e: React.MouseEvent<any>) => void; | ||
style?: {}; | ||
children?: React.ReactNode; | ||
} | ||
export default class ReactPanZoom extends React.PureComponent<IReactPanZoomProps> { | ||
static defaultProps: Partial<IReactPanZoomProps>; | ||
private panWrapper; | ||
private panContainer; | ||
private getInitialState; | ||
state: IReactPanZoomStateType; | ||
UNSAFE_componentWillReceiveProps(nextProps: IReactPanZoomProps): void; | ||
reset: () => void; | ||
onClick: (e: React.MouseEvent<EventTarget>) => void; | ||
onTouchStart: (e: React.TouchEvent<EventTarget>) => void; | ||
onTouchEnd: () => void; | ||
onTouchMove: (e: React.TouchEvent<EventTarget>) => void; | ||
render(): JSX.Element; | ||
private onMouseDown; | ||
private panStart; | ||
private onMouseUp; | ||
private panEnd; | ||
preventDefault(e: any): void; | ||
private onMouseMove; | ||
private onWheel; | ||
private onMouseEnter; | ||
private onMouseLeave; | ||
private updateMousePosition; | ||
private getNewMatrixData; | ||
} |
{ | ||
"name": "react-image-pan-zoom-rotate", | ||
"version": "1.5.0", | ||
"version": "1.6.0", | ||
"description": "React library to give control on image to move zoom and rotate", | ||
@@ -9,4 +9,7 @@ "author": "mgorabbani", | ||
"main": "dist/index.js", | ||
"module": "dist/index.modern.js", | ||
"source": "src/index.tsx", | ||
"typings": "dist/index.d.ts", | ||
"files": [ | ||
"dist", | ||
"src" | ||
], | ||
"engines": { | ||
@@ -22,51 +25,46 @@ "node": ">=10" | ||
"scripts": { | ||
"build": "microbundle-crl --no-compress --format modern,cjs", | ||
"start": "microbundle-crl watch --no-compress --format modern,cjs", | ||
"prepare": "run-s build", | ||
"test": "run-s test:unit test:lint test:build", | ||
"test:build": "run-s build", | ||
"test:lint": "eslint .", | ||
"test:unit": "cross-env CI=1 react-scripts test --env=jsdom", | ||
"test:watch": "react-scripts test --env=jsdom", | ||
"predeploy": "cd example && npm install && npm run build", | ||
"deploy": "gh-pages -d example/build" | ||
"start": "tsdx watch", | ||
"build": "tsdx build", | ||
"test": "tsdx test --passWithNoTests", | ||
"lint": "tsdx lint", | ||
"prepare": "tsdx build", | ||
"size": "size-limit", | ||
"analyze": "size-limit --why" | ||
}, | ||
"peerDependencies": { | ||
"react": "^16.0.0" | ||
"react": ">=16" | ||
}, | ||
"devDependencies": { | ||
"@testing-library/jest-dom": "4.2.4", | ||
"@testing-library/react": "9.5.0", | ||
"@testing-library/user-event": "7.2.1", | ||
"@types/jest": "25.1.4", | ||
"@types/node": "12.12.38", | ||
"@types/react": "16.9.27", | ||
"@types/react-dom": "16.9.7", | ||
"@typescript-eslint/eslint-plugin": "2.26.0", | ||
"@typescript-eslint/parser": "2.26.0", | ||
"microbundle-crl": "0.13.10", | ||
"babel-eslint": "10.0.3", | ||
"cross-env": "7.0.2", | ||
"eslint": "6.8.0", | ||
"eslint-config-prettier": "6.7.0", | ||
"eslint-config-standard": "14.1.0", | ||
"eslint-config-standard-react": "9.2.0", | ||
"eslint-plugin-import": "2.18.2", | ||
"eslint-plugin-node": "11.0.0", | ||
"eslint-plugin-prettier": "3.1.1", | ||
"eslint-plugin-promise": "4.2.1", | ||
"eslint-plugin-react": "7.17.0", | ||
"eslint-plugin-standard": "4.0.1", | ||
"gh-pages": "2.2.0", | ||
"npm-run-all": "4.1.5", | ||
"prettier": "2.0.4", | ||
"react": "16.13.1", | ||
"react-dom": "16.13.1", | ||
"react-scripts": "3.4.1", | ||
"typescript": "3.7.5" | ||
"husky": { | ||
"hooks": { | ||
"pre-commit": "tsdx lint" | ||
} | ||
}, | ||
"files": [ | ||
"dist" | ||
"prettier": { | ||
"printWidth": 80, | ||
"semi": true, | ||
"singleQuote": true, | ||
"trailingComma": "es5" | ||
}, | ||
"size-limit": [ | ||
{ | ||
"path": "dist/mylib.cjs.production.min.js", | ||
"limit": "10 KB" | ||
}, | ||
{ | ||
"path": "dist/mylib.esm.js", | ||
"limit": "10 KB" | ||
} | ||
], | ||
"dependencies": {} | ||
"devDependencies": { | ||
"@size-limit/preset-small-lib": "^7.0.8", | ||
"@types/react": "^18.0.14", | ||
"@types/react-dom": "^18.0.5", | ||
"husky": "^8.0.1", | ||
"react": "^18.2.0", | ||
"react-dom": "^18.2.0", | ||
"size-limit": "^7.0.8", | ||
"tsdx": "^0.14.1", | ||
"tslib": "^2.4.0", | ||
"typescript": "^4.7.4" | ||
} | ||
} |
@@ -10,3 +10,3 @@ # React Image Pan, Zoom & Rotate | ||
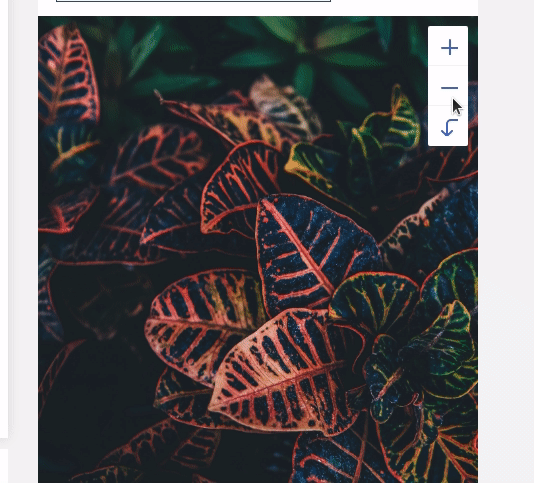 | ||
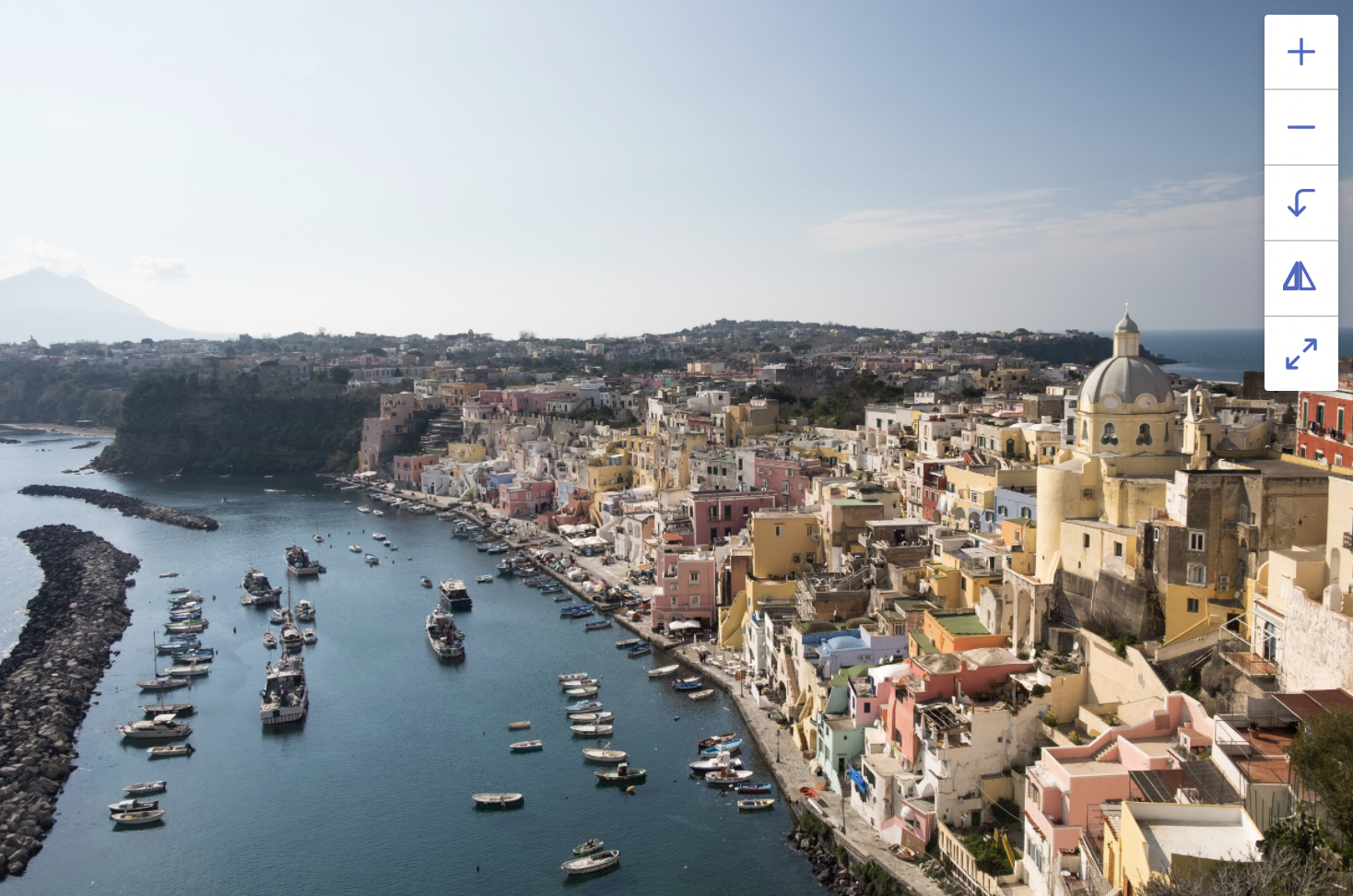 | ||
@@ -22,2 +22,3 @@ [](https://codesandbox.io/s/n1rv671pkj) | ||
- Flip Image | ||
- Reset | ||
@@ -39,4 +40,4 @@ ## Install | ||
```tsx | ||
import React from 'react' | ||
import ReactPanZoom from 'react-image-pan-zoom-rotate' | ||
import React from 'react'; | ||
import ReactPanZoom from 'react-image-pan-zoom-rotate'; | ||
@@ -46,13 +47,19 @@ const App = () => { | ||
<ReactPanZoom | ||
image='https://images.unsplash.com/photo-1551091708-fda32ed3178c' | ||
alt='Image alt text' | ||
image="https://images.unsplash.com/photo-1551091708-fda32ed3178c" | ||
alt="Image alt text" | ||
/> | ||
) | ||
} | ||
); | ||
}; | ||
export default App | ||
export default App; | ||
``` | ||
or Check example folder below file to use your custom UI | ||
``` | ||
ReactPanZoom.tsx | ||
``` | ||
## License | ||
MIT © [mgorabbani](https://github.com/mgorabbani) |
Major refactor
Supply chain riskPackage has recently undergone a major refactor. It may be unstable or indicate significant internal changes. Use caution when updating to versions that include significant changes.
Found 1 instance in 1 package
Environment variable access
Supply chain riskPackage accesses environment variables, which may be a sign of credential stuffing or data theft.
Found 1 instance in 1 package
Minified code
QualityThis package contains minified code. This may be harmless in some cases where minified code is included in packaged libraries, however packages on npm should not minify code.
Found 1 instance in 1 package
123340
10
14
1503
62
2
2
1