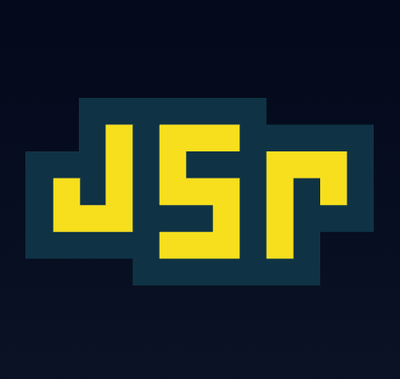
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
react-measure
Advanced tools
The react-measure package is a React component that allows you to measure the dimensions and position of a DOM element. It provides a declarative way to get the size and position of elements, which can be useful for responsive design, animations, and other dynamic UI behaviors.
Measure Dimensions
This feature allows you to measure the width and height of a DOM element. The `Measure` component wraps around the element you want to measure, and the `onResize` callback updates the state with the new dimensions whenever the element is resized.
import React from 'react';
import Measure from 'react-measure';
class MyComponent extends React.Component {
state = {
dimensions: {
width: -1,
height: -1
}
};
render() {
return (
<Measure
bounds
onResize={(contentRect) => {
this.setState({ dimensions: contentRect.bounds });
}}
>
{({ measureRef }) => (
<div ref={measureRef} style={{ width: '100%' }}>
<p>The width of this element is {this.state.dimensions.width}px</p>
<p>The height of this element is {this.state.dimensions.height}px</p>
</div>
)}
</Measure>
);
}
}
export default MyComponent;
Measure Position
This feature allows you to measure the position (top and left) of a DOM element. The `Measure` component wraps around the element you want to measure, and the `onResize` callback updates the state with the new position whenever the element is resized.
import React from 'react';
import Measure from 'react-measure';
class MyComponent extends React.Component {
state = {
position: {
top: -1,
left: -1
}
};
render() {
return (
<Measure
bounds
onResize={(contentRect) => {
this.setState({ position: contentRect.bounds });
}}
>
{({ measureRef }) => (
<div ref={measureRef} style={{ position: 'relative' }}>
<p>The top position of this element is {this.state.position.top}px</p>
<p>The left position of this element is {this.state.position.left}px</p>
</div>
)}
</Measure>
);
}
}
export default MyComponent;
react-resize-detector is a lightweight package that provides a higher-order component and a hook to detect resize events on a DOM element. It is similar to react-measure in that it allows you to measure the dimensions of an element, but it does not provide as many detailed measurements (e.g., position).
react-use-measure is a React hook that allows you to measure the size and position of a DOM element. It is similar to react-measure but uses hooks instead of a component-based API, making it more suitable for modern React applications that prefer hooks.
react-sizeme is a higher-order component that makes it easy to measure the size of a component. It is similar to react-measure in that it provides dimensions, but it is more focused on providing a simple API for measuring size without additional features like position measurement.
Compute measurements of React components. Uses resize-observer-polyfill to detect changes of an element and return the new dimensions.
yarn add react-measure
npm install react-measure --save
<script src="https://unpkg.com/react-measure/dist/react-measure.js"></script>
(UMD library exposed as `Measure`)
Wrap any child component and calculate its client rect.
client
: PropTypes.boolAdds the following to contentRect.client
returned in the child function.
clientTop, clientLeft, clientWidth, and clientHeight.
offset
: PropTypes.boolAdds the following to contentRect.offset
returned in the child function.
offsetTop, offsetLeft, offsetWidth, and offsetHeight.
scroll
: PropTypes.boolAdds the following to contentRect.scroll
returned in the child function.
scrollTop, scrollLeft, scrollWidth, and scrollHeight.
bounds
: PropTypes.boolUses getBoundingClientRect to calculate the element rect and add it to contentRect.bounds
returned in the child function.
margin
: PropTypes.boolUses getComputedStyle to calculate margins and add it to contentRect.margin
returned in the child function.
innerRef
: PropTypes.funcUse this to access the internal component ref
.
onResize
: PropTypes.funcCallback invoked when either element width or height have changed.
children
: PropTypes.funcChildren must be a function. Will receive the following object shape:
measureRef
: must be passed down to your component's ref in order to obtain a proper node to measure
measure
: use to programmatically measure your component, calls the internal measure
method in withContentRect
contentRect
: this will contain any of the following allowed rects from above: client
, offset
, scroll
, bounds
, or margin
. It will also include entry
from the ResizeObserver
when available.
import Measure from 'react-measure'
import classNames from 'classnames'
class ItemToMeasure extends Component {
state = {
dimensions: {
width: -1,
height: -1
}
}
render() {
const { width, height } = this.state.dimensions
const className = classNames(
(width < 400) && 'small-width-modifier'
)
return (
<Measure
bounds
onResize={(contentRect) => {
this.setState({ dimensions: contentRect.bounds })
}}
>
{({ measureRef }) =>
<div ref={measureRef} className={className}>
I can do cool things with my dimensions now :D
{ (height > 250) &&
<div>Render responsive content based on the component size!</div>
}
</div>
}
</Measure>
)
}
}
A higher-order component that provides dimensions to the wrapped component. Accepts types
, which determines what measurements are returned, similar to above. Then returns a function to pass the component you want measured.
Pass an array or single value of either client
, offset
, scroll
, bounds
, or margin
to calculate and receive those measurements as the prop contentRect
in your wrapped component. You can also use the measure
function passed down to programmatically measure your component if you need to. And finally, remember to pass down the measureRef
to the component you want measured.
Passes down the same props as the Measure
child function above, measureRef
, measure
, and contentRect
.
Fun fact, the Measure
component is a thin wrapper around withContentRect
. Just check the source. This means your wrapped component will accept the same props as Measure
does 😊
import { withContentRect } from 'react-measure'
const ItemToMeasure = withContentRect('bounds')(({ measureRef, measure, contentRect }) => (
<div ref={measureRef}>
Some content here
<pre>
{JSON.stringify(contentRect, null, 2)}
</pre>
</div>
))
clone repo
git clone git@github.com:souporserious/react-measure.git
move into folder
cd ~/react-measure
install dependencies
npm install
run dev mode
npm run dev
open your browser and visit: http://localhost:8080/
FAQs
Compute measurements of React components.
We found that react-measure demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.