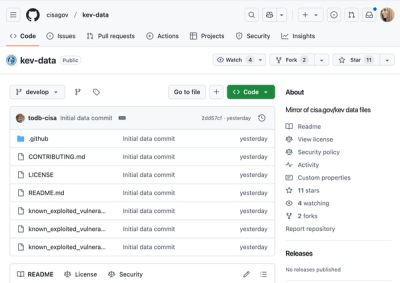
Security News
CISA Brings KEV Data to GitHub
CISA's KEV data is now on GitHub, offering easier access, API integration, commit history tracking, and automated updates for security teams and researchers.
react-multi-select-advanced
Advanced tools
React multi select filter input with advanced features
The React Multi-select Advanced component is a powerful tool that can handle massive data sets without any struggle. It comes with various customizable features that make it very easy to use, so you can tailor it to your specific needs. Now it supports server side search.
Like Beyoncé once said, If you liked it, then you should a put a ⭐ on it. ➽
npm install react-multi-select-advanced
yarn add react-multi-select-advanced
import { useState } from 'react'
// Import component / and types if need it.
import MultiSelectAdvanced, { MultiSelectAdvancedOption } from 'react-multi-select-advanced'
// Mock data
const options = [
{ label: 'Istanbul', value: 'Istanbul' },
{ label: 'Paris', value: 'Paris' },
{ label: 'London', value: 'London' },
{ label: 'Buenos Aires', value: 'Buenos Aires' },
{ label: 'Canberra', value: 'Canberra' },
{ label: 'Havana', value: 'Havana' },
{ label: 'Helsinki', value: 'Helsinki', disabled: true },
{ label: 'Tokyo', value: 'Tokyo' },
{ label: 'Amsterdam', value: 'Amsterdam' },
{ label: 'Moscow', value: 'Moscow' },
{ label: 'Stockholm', value: 'Stockholm', disabled: true },
{ label: 'Singapore', value: 'Singapore' },
{ label: 'Lisbon', value: 'Lisbon' },
{ label: 'Oslo', value: 'Oslo' },
{ label: 'Reykjavík', value: 'Reykjavík' }
]
// Mock pre selected data
const selectedValues = [
{ label: 'London', value: 'London' },
{ label: 'Tokyo', value: 'Tokyo' }
]
// App
function App() {
// Pre-select or not
const [selectedItems, setSelectedItems] = useState(selectedValues as MultiSelectAdvancedOption[])
// Onchange handler
const handleChange = (items: MultiSelectAdvancedOption[]) => setSelectedItems(items)
return (
<div className="App">
<MultiSelectAdvanced options={options} selectedValues={selectedItems} onChange={handleChange} />
</div>
)
}
export default App
import { useState } from 'react'
// Import component / and types if need it.
import MultiSelectAdvanced, { MultiSelectAdvancedOption } from 'react-multi-select-advanced'
// Mock data
const options = [
{ label: 'Istanbul', value: 'Istanbul' },
{ label: 'Paris', value: 'Paris' },
{ label: 'London', value: 'London' },
{ label: 'Buenos Aires', value: 'Buenos Aires' },
{ label: 'Canberra', value: 'Canberra' },
{ label: 'Havana', value: 'Havana' },
{ label: 'Helsinki', value: 'Helsinki', disabled: true },
{ label: 'Tokyo', value: 'Tokyo' },
{ label: 'Amsterdam', value: 'Amsterdam' },
{ label: 'Moscow', value: 'Moscow' },
{ label: 'Stockholm', value: 'Stockholm', disabled: true },
{ label: 'Singapore', value: 'Singapore' },
{ label: 'Lisbon', value: 'Lisbon' },
{ label: 'Oslo', value: 'Oslo' },
{ label: 'Reykjavík', value: 'Reykjavík' }
]
// App
function App() {
// Pre-select or not
const [selectedItems, setSelectedItems] = useState([] as MultiSelectAdvancedOption[])
// Onchange handler
const handleChange = (items: MultiSelectAdvancedOption[]) => setSelectedItems(items)
// Server side search function
const searchOnServer = async (keyword:string) => {
try {
// Before return the data make sure structure is matching with array of MultiSelectAdvancedOption
return await search(keyword)
} catch (error){
return error
}
}
// Mock server response
const search = async (keyword:string) => {
return new Promise(resolve => {
const filteredData = options.cities.filter(city => city.label.toLowerCase().includes(keyword.toLowerCase()))
return setTimeout(() => resolve(filteredData), 500)
})
}
return (
<div className="App">
<MultiSelectAdvanced isServerSide={true} selectedValues={selectedItems} onChange={handleChange} onKeywordChange={keyword => searchOnServer(keyword)} />
</div>
)
}
export default App
Prop | Type | Default | Description |
---|---|---|---|
className | string | Options data. | |
options | array | [] | Options data. |
selectedValues | array | [] | Pre-selected options. |
Input | |||
name | string | auto | input name. |
id | string | auto | input id. |
label | string | Add label to top of input. | |
disabled | boolean | Disables input and all actions. | |
invalid | boolean | If true border color turns to red. | |
inputDelay | number | 1000 | Input delay (ms). |
placeholder | string | Input placeholder. | |
Filter | |||
filterShowLoading | boolean | true | Show/hide loading indicator or component. |
filterLimit | number | Maximum dropdown display limit. | |
filterHighlightKeyword | boolean | false | Highlights matching keyword. Suggested to use with filterLimit because of performance. |
filterOrderByMatchRank | boolean | false | Gives match score if label starts with search keyword. Suggested to use with filterLimit because of performance. |
Selected Items | |||
selectionLimit | number | Limits selected items. | |
hideInputOnSelectionLimit | boolean | false | Hides input when selection limit reached |
selectionMaxVisibleItems | number | Limits selected display items and adds 'x more..' or MoreItemsComponent after items. | |
selectionLabelMaxWidth | number | 100 | Limits max width (px) of display label and wrap with ellipsis. |
selectionShowClear | boolean | false | Shows clear all button after selected items if selected count more than 2. |
selectionShowDeleteButton | boolean | true | Shows remove button inside the selected items. |
Language | |||
languageOverwrite | object | Please see 4. Localization. | |
Custom components | |||
LoadingComponent | JSX.Element | Custom loading indicator. | |
ClearButtonComponent | JSX.Element | Custom clear all button. | |
DeleteButtonComponent | JSX.Element | Custom delete button. | |
MoreItemsComponent | JSX.Element | Custom more items. | |
Callback Type | |||
isServerSide | boolean | false | Enables server side search. |
Callback | |||
onChange | function | Callback function will invoked on selected options are changed. | |
onKeywordChange | function | Callback function to pass keyword and accept data on return. |
Easy to localize component by passing object to prop languageOverwrite
. Default values are as below.
{
selectionLimitReached : 'Max selection limit reached.',
selectionShowClearTitle: 'Clear All',
selectionDeleteTitle: 'Remove',
moreItemsText: '{{count}} more items...'
}
MIT Licensed. Copyright © Lifetoweb 2022.
FAQs
React multi select filter input with advanced features
The npm package react-multi-select-advanced receives a total of 48 weekly downloads. As such, react-multi-select-advanced popularity was classified as not popular.
We found that react-multi-select-advanced demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CISA's KEV data is now on GitHub, offering easier access, API integration, commit history tracking, and automated updates for security teams and researchers.
Security News
Opengrep forks Semgrep to preserve open source SAST in response to controversial licensing changes.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.