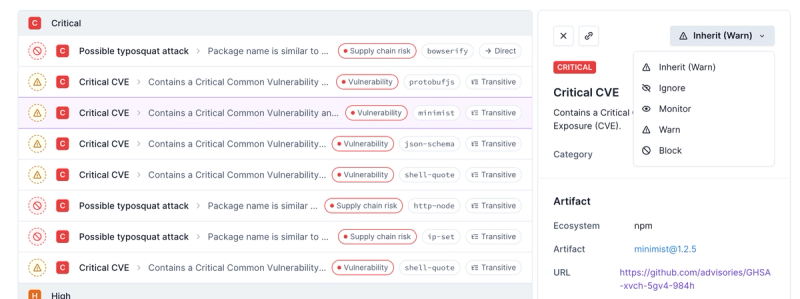
Product
Introducing Enhanced Alert Actions and Triage Functionality
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
react-native-apxor-sdk
Advanced tools
Readme
React Native wrapper for Apxor SDK. Please refer Plugins section to integrate other Apxor plugins.
react-native
version 0.59.0
and lowernpm i react-native-apxor-sdk
react-native link react-native-apxor-sdk
Open up android/app/src/main/java/[...]/MainActivity.java
import com.apxor.reactnativesdk.RNApxorSDKPackage;
to the imports at the top of the filenew RNApxorSDKPackage()
to the list returned by the getPackages()
method@Override
protected List<ReactPackage> getPackages() {
return Arrays.<ReactPackage>asList(
new MainReactPackage(),
...
new RNApxorSDKPackage(), <- ApxorSDK package
...
);
}
Append the following lines to android/settings.gradle
:
include ':react-native-apxor-sdk'
project(':react-native-apxor-sdk').projectDir = new File(rootProject.projectDir, '../node_modules/react-native-apxor-sdk/android')
react-native
version 0.60.0
and higherRun the following commands:
npm i react-native-apxor-sdk
android/build.gradle
maven { url "http://repo.apxor.com/artifactory/list/libs-release-android/" }
maven { url "https://dl.bintray.com/apxor/apxor-android" }
android/app/build.gradle
: implementation 'com.apxor.androidx:apxor-android-sdk-core:2.8.3@aar'
Add the following meta-data
tag inside application
tag of AndroidManifest.xml
file:
<application ...>
...
<meta-data android:name="APXOR_APP_ID" android:value=[REPLACE_YOUR_APXOR_APP_ID_HERE] />
...
</application>
Add following dependencies in the build.gradle
file.
// Add this to track uninstalls and send push notifications from Apxor dashboard
// Note: We don't support firebase-messaging >= 22.0.0 yet
implementation('com.apxor.androidx:apxor-android-sdk-push:1.2.7@aar') {
exclude group: 'com.google.firebase'
}
// Add these for Realtime Actions and Surveys
implementation 'com.apxor.androidx:apxor-android-sdk-qe:1.5.1@aar'
implementation 'com.apxor.androidx:apxor-android-sdk-rtm:1.8.9@aar'
implementation 'com.apxor.androidx:surveys:1.3.6@aar'
// Add this to track application crashes
implementation 'com.apxor.androidx:apxor-android-crash-reporter:1.0.5@aar'
// Helper plugin to create walkthroughs
implementation 'com.apxor.androidx:wysiwyg:1.2.6@aar'
// Add this to log events without attributes at runtime
implementation 'com.apxor.androidx:jit-log:1.0.0@aar'
// Getting a "Could not find" error? Make sure that you've added Apxor's Maven repository to your root-level build.gradle file.
NOTE
If you are using
firebase-messaging >= 22.0.0
, use"com.apxor.androidx:apxor-android-sdk-push-v2"
instead of"com.apxor.androidx:apxor-android-sdk-push"
implementation('com.apxor.androidx:apxor-android-sdk-push-v2:1.2.7@aar') {
exclude group: 'com.google.firebase'
}
Create plugins.json
file in the android/app/src/main/assets/
directory.
Paste the following JSON code in that file:
{
"plugins": [
{
"name": "rtm",
"class": "com.apxor.androidsdk.plugins.realtimeui.ApxorRealtimeUIPlugin"
},
{
"name": "crash",
"class": "com.apxor.androidsdk.plugins.crash.CrashReporterPlugin"
},
{
"name": "push",
"class": "com.apxor.androidsdk.plugins.push.PushPlugin"
},
{
"name": "surveys",
"class": "com.apxor.androidsdk.plugins.survey.SurveyPlugin"
},
{
"name": "wysiwyg",
"class": "com.apxor.androidsdk.plugins.wysiwyg.WYSIWYGPlugin"
},
{
"name": "jitlog",
"class": "com.apxor.androidsdk.plugins.jitlog.ApxorJITLogPlugin"
}
]
}
If you have extended the FirebaseMessagingService
in your application, please use the below code in your extends FirebaseMessagingService
class to receive Push notifications sent from the Apxor dashboard:
if (remoteMessage.getFrom().equals(YOUR_FCM_SENDER_ID)) {
// Your logic goes here
} else {
if (ApxorPushAPI.isApxorNotification(remoteMessage)) {
ApxorPushAPI.handleNotification(remoteMessage, getApplicationContext());
} else {
// Silent or Data push notification which you can send through Apxor dashboard
}
}
To Auto initialize the SDK (Recommended), add the following inside your application
plist file.
Open your application's Info.plist
as source code.
Copy and paste the following piece of code, to create an entry for ApxorSDK:
<key>Apxor</key>
<dict>
<key>Core</key>
<string>{YOUR_APP_ID}</string>
<key>APXSurveyPlugin</key>
<true/>
<key>APXRTAPlugin</key>
<true/>
<key>APXWYSIWYGPlugin</key>
<true/>
</dict>
To add the APXWYSIWYGPlugin, add the following to your application's .podspec
file:
s.dependency 'Apxor-WYSIWYG', '1.0.84'
NOTE
The
APXWYSIWYGPlugin
is only for debug builds, make sure to remove it for production builds.
// Import the SDK as a module
import RNApxorSDK from 'react-native-apxor-sdk'
// Or, import required modules
import { ApxNavigationContainer, logAppEvent } from 'react-native-apxor-sdk'
// Syntax
RNApxorSDK.setUserIdentifier('user_id')
// Example
RNApxorSDK.setUserIdentifier('john@doe.com')
// Syntax
RNApxorSDK.setUserCustomInfo(properties)
RNApxorSDK.setSessionCustomInfo(properties)
// Example
RNApxorSDK.setUserCustomInfo({
city: 'Hyderabad',
age: 12,
})
A wrapper over React Native's View
component to track the View changes in the app.
// Syntax
<ApxorView
tag='<unique_constant_tag>'
{...otherViewProps}
>
// Your code goes here
</ApxorView>
// Syntax
RNApxorSDK.logAppEvent(event_name, properties)
// Example
RNApxorSDK.logAppEvent('ADD_TO_CART', {
userId: 'user@example.com',
value: 1299,
item: 'Sony Head Phone 1201',
})
@react-navigation
for navigation in your app, Apxor SDK automatically tracks screen navigation if you follow the below mentioned steps:Apxor's ApxNavigationContainer
is just a wrapper over NavigationContainer
which internally handles the route navigations (nested route navigations too) and take necessary actions within RNApxorSDK.
To automatically track navigations, use ApxNavigationContainer
and pass the same props you pass to NavigationContainer
like below:
import { ApxNavigationContainer } from 'react-native-apxor-sdk'
import { createStackNavigator } from '@react-navigation/stack'
const Stack = createStackNavigator()
function App() {
return (
<ApxNavigationContainer
theme={yourTheme}
linking={yourLinkingOptions}
fallback={yourFallbackNode}
{...others}
>
<Stack.Navigator initialRouteName='Home'>
<Stack.Screen
name='Home'
component={Home}
/>
<Stack.Screen
name='Details'
component={DetailsScreen}
/>
<Stack.Screen
name='FlatList'
component={FlatListSample}
/>
</Stack.Navigator>
</ApxNavigationContainer>
)
}
// Syntax
RNApxorSDK.logNavigationEvent(screen_name)
// Example
RNApxorSDK.logNavigationEvent('LoginScreen')
// Syntax
RNApxorSDK.trackScreen(tab_name)
// Example
RNApxorSDK.trackScreen('Home')
FAQs
React Native Apxor SDK
The npm package react-native-apxor-sdk receives a total of 893 weekly downloads. As such, react-native-apxor-sdk popularity was classified as not popular.
We found that react-native-apxor-sdk demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
Security News
Polyfill.io has been serving malware for months via its CDN, after the project's open source maintainer sold the service to a company based in China.
Security News
OpenSSF is warning open source maintainers to stay vigilant against reputation farming on GitHub, where users artificially inflate their status by manipulating interactions on closed issues and PRs.