react-native-code-push
Advanced tools
Comparing version 5.2.1 to 5.2.2
@@ -50,2 +50,6 @@ ### Java API Reference (Android) | ||
##### Public Methods | ||
- __setDeploymentKey(String deploymentKey)__ - Sets the deployment key that the app should use when querying for updates. This is a dynamic alternative to setting the deployment key in Codepush constructor/builder and/or specifying a deployment key in JS when calling `checkForUpdate` or `sync`. | ||
##### Static Methods | ||
@@ -52,0 +56,0 @@ |
@@ -54,4 +54,5 @@ ## API Reference | ||
// interrupting the end user | ||
class MyApp extends Component {} | ||
class MyApp extends Component<{}> {} | ||
MyApp = codePush(MyApp); | ||
export default MyApp; | ||
``` | ||
@@ -63,4 +64,5 @@ | ||
// Sync for updates everytime the app resumes. | ||
class MyApp extends Component {} | ||
class MyApp extends Component<{}> {} | ||
MyApp = codePush({ checkFrequency: codePush.CheckFrequency.ON_APP_RESUME, installMode: codePush.InstallMode.ON_NEXT_RESUME })(MyApp); | ||
export default MyApp; | ||
``` | ||
@@ -74,4 +76,5 @@ | ||
// immediately after downloading it | ||
class MyApp extends Component {} | ||
class MyApp extends Component<{}> {} | ||
MyApp = codePush({ updateDialog: true, installMode: codePush.InstallMode.IMMEDIATE })(MyApp); | ||
export default MyApp; | ||
``` | ||
@@ -84,3 +87,3 @@ | ||
// the different stages of the sync process. | ||
class MyApp extends Component { | ||
class MyApp extends Component<{}> { | ||
codePushStatusDidChange(status) { | ||
@@ -111,2 +114,3 @@ switch(status) { | ||
MyApp = codePush(MyApp); | ||
export default MyApp; | ||
``` | ||
@@ -113,0 +117,0 @@ |
### Android | ||
> NOTE | ||
> | ||
> Complete demo configured with "multi-deployment testing" feature is [here](https://github.com/Microsoft/react-native-code-push/files/1314118/rncp1004.zip). | ||
The [Android Gradle plugin](http://google.github.io/android-gradle-dsl/current/index.html) allows you to define custom config settings for each "build type" (e.g. debug, release), which in turn are generated as properties on the `BuildConfig` class that you can reference from your Java code. This mechanism allows you to easily configure your debug builds to use your CodePush staging deployment key and your release builds to use your CodePush production deployment key. | ||
@@ -4,0 +8,0 @@ |
### iOS | ||
> NOTE | ||
> | ||
> Complete demos configured with "multi-deployment testing" feature are [here]: | ||
> * **without using cocoa pods**: [link](https://github.com/Microsoft/react-native-code-push/files/1259957/rncp976.copy.zip) | ||
> * **using cocoa pods**: [link](https://github.com/Microsoft/react-native-code-push/files/1172217/rncp893.copy.zip) | ||
Xcode allows you to define custom build settings for each "configuration" (e.g. debug, release), which can then be referenced as the value of keys within the `Info.plist` file (e.g. the `CodePushDeploymentKey` setting). This mechanism allows you to easily configure your builds to produce binaries, which are configured to synchronize with different CodePush deployments. | ||
@@ -43,4 +49,6 @@ | ||
*NOTE: CocoaPods users may need to run `pod install` before building with their new release configuration.* | ||
*Note: If you encounter the error message `ld: library not found for ...`, please consult [this issue](https://github.com/Microsoft/react-native-code-push/issues/426) for a possible solution.* | ||
Additionally, if you want to give them seperate names and/or icons, you can modify the `Product Bundle Identifier`, `Product Name` and `Asset Catalog App Icon Set Name` build settings, which will allow your staging builds to be distinguishable from release builds when installed on the same device. | ||
Additionally, if you want to give them seperate names and/or icons, you can modify the `Product Bundle Identifier`, `Product Name` and `Asset Catalog App Icon Set Name` build settings, which will allow your staging builds to be distinguishable from release builds when installed on the same device. |
## Android Setup | ||
In order to integrate CodePush into your Android project, perform the following steps: | ||
* [Plugin Installation (Android)](#plugin-installation-android) | ||
* [Plugin Installation (Android - RNPM)](#plugin-installation-android---rnpm) | ||
* [Plugin Installation (Android - Manual)](#plugin-installation-android---manual) | ||
* [Plugin Configuration (Android)](#plugin-configuration-android) | ||
* [For React Native >= v0.29](#for-react-native--v029) | ||
* [For newly created React Native application](#for-newly-created-react-native-application) | ||
* [For existing native application](#for-existing-native-application) | ||
* [For React Native v0.19 - v0.28](#for-react-native-v019---v028) | ||
* [Background React Instances](#background-react-instances) | ||
* [For React Native >= v0.29 (Background React Instances)](#for-react-native--v029-background-react-instances) | ||
* [For React Native v0.19 - v0.28 (Background React Instances)](#for-react-native-v019---v028-background-react-instances) | ||
* [WIX React Native Navigation applications (ver 1.x)](#wix-react-native-navigation-applications) | ||
* [Code Signing setup](#code-signing-setup) | ||
In order to integrate CodePush into your Android project, please perform the following steps: | ||
### Plugin Installation (Android) | ||
@@ -67,4 +81,8 @@ | ||
**For React Native >= v0.29** | ||
#### For React Native >= v0.29 | ||
##### For newly created React Native application | ||
If you are integrating Code Push into React Native application please do the following steps: | ||
Update the `MainApplication.java` file to use CodePush via the following changes: | ||
@@ -111,4 +129,40 @@ | ||
**For React Native v0.19 - v0.28** | ||
##### For existing native application | ||
If you are integrating React Native into existing native application please do the following steps: | ||
Update `MyReactActivity.java` (it could be named differently in your app) file to use CodePush via the following changes: | ||
```java | ||
... | ||
// 1. Import the plugin class. | ||
import com.microsoft.codepush.react.CodePush; | ||
public class MyReactActivity extends Activity { | ||
private ReactRootView mReactRootView; | ||
private ReactInstanceManager mReactInstanceManager; | ||
@Override | ||
protected void onCreate(Bundle savedInstanceState) { | ||
... | ||
mReactInstanceManager = ReactInstanceManager.builder() | ||
// ... | ||
// Add CodePush package | ||
.addPackage(new CodePush("deployment-key-here", getApplicationContext(), BuildConfig.DEBUG)) | ||
// Get the JS Bundle File via Code Push | ||
.setJSBundleFile(CodePush.getJSBundleFile()) | ||
// ... | ||
.build(); | ||
mReactRootView.startReactApplication(mReactInstanceManager, "MyReactNativeApp", null); | ||
setContentView(mReactRootView); | ||
} | ||
... | ||
} | ||
``` | ||
#### For React Native v0.19 - v0.28 | ||
Update the `MainActivity.java` file to use CodePush via the following changes: | ||
@@ -152,3 +206,3 @@ | ||
**For React Native >= v0.29** | ||
##### For React Native >= v0.29 (Background React Instances) | ||
@@ -180,3 +234,3 @@ Update the `MainApplication.java` file to use CodePush via the following changes: | ||
**For React Native v0.19 - v0.28** | ||
##### For React Native v0.19 - v0.28 (Background React Instances) | ||
@@ -201,2 +255,66 @@ Before v0.29, React Native did not provide a `ReactNativeHost` abstraction. If you're launching a background instance, you'll likely have built your own, which should now implement `ReactInstanceHolder`. Once that's done: | ||
#### WIX React Native Navigation applications | ||
If you are using [WIX React Native Navigation **version 1.x**](https://github.com/wix/react-native-navigation) based application, please do the following steps to integrate CodePush: | ||
1. No need to change `MainActivity.java` file, so if you are integrating CodePush to newly created RNN application it might be looking like this: | ||
```java | ||
import com.facebook.react.ReactActivity; | ||
import com.reactnativenavigation.controllers.SplashActivity; | ||
public class MainActivity extends SplashActivity { | ||
} | ||
``` | ||
2. Update the `MainApplication.java` file to use CodePush via the following changes: | ||
```java | ||
// ... | ||
import com.facebook.react.ReactInstanceManager; | ||
// Add CodePush imports | ||
import com.microsoft.codepush.react.ReactInstanceHolder; | ||
import com.microsoft.codepush.react.CodePush; | ||
public class MainApplication extends NavigationApplication implements ReactInstanceHolder { | ||
@Override | ||
public boolean isDebug() { | ||
// Make sure you are using BuildConfig from your own application | ||
return BuildConfig.DEBUG; | ||
} | ||
protected List<ReactPackage> getPackages() { | ||
// Add additional packages you require here | ||
return Arrays.<ReactPackage>asList( | ||
new CodePush("deployment-key-here", getApplicationContext(), BuildConfig.DEBUG) | ||
); | ||
} | ||
@Override | ||
public List<ReactPackage> createAdditionalReactPackages() { | ||
return getPackages(); | ||
} | ||
@Override | ||
public String getJSBundleFile() { | ||
// Override default getJSBundleFile method with the one CodePush is providing | ||
return CodePush.getJSBundleFile(); | ||
} | ||
@Override | ||
public String getJSMainModuleName() { | ||
return "index"; | ||
} | ||
@Override | ||
public ReactInstanceManager getReactInstanceManager() { | ||
// CodePush must be told how to find React Native instance | ||
return getReactNativeHost().getReactInstanceManager(); | ||
} | ||
} | ||
``` | ||
#### Code Signing setup | ||
@@ -244,2 +362,2 @@ | ||
.build() | ||
``` | ||
``` |
{ | ||
"name": "react-native-code-push", | ||
"version": "5.2.1", | ||
"version": "5.2.2", | ||
"description": "React Native plugin for the CodePush service", | ||
@@ -5,0 +5,0 @@ "main": "CodePush.js", |
@@ -70,4 +70,4 @@ [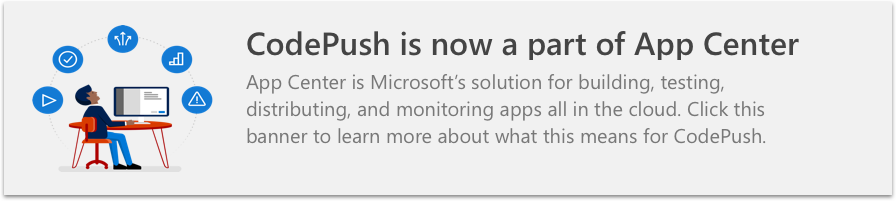](http://microsoft.github.io/code-push/) | ||
| v0.46 | v4.0+ *(RN refactored js bundle loader code)* | | ||
| v0.46-v0.50 | v5.1+ *(RN removed unused registration of JS modules)*| | ||
| v0.51+ | TBD :) We work hard to respond to new RN releases, but they do occasionally break us. We will update this chart with each RN release, so that users can check to see what our "official" support is. | ||
| v0.46-v0.52 | v5.1+ *(RN removed unused registration of JS modules)*| | ||
| v0.53+ | TBD :) We work hard to respond to new RN releases, but they do occasionally break us. We will update this chart with each RN release, so that users can check to see what our "official" support is. | ||
@@ -268,3 +268,3 @@ ### Supported Components | ||
You'll notice that the above steps refer to a "staging build" and "production build" of your app. If your build process already generates distinct binaries per "environment", then you don't need to read any further, since swapping out CodePush deployment keys is just like handling environment-specific config for any other service your app uses (e.g. Facebook). However, if you're looking for examples on how to setup your build process to accommodate this, then refer to the following sections, depending on the platform(s) your app is targeting: | ||
You'll notice that the above steps refer to a "staging build" and "production build" of your app. If your build process already generates distinct binaries per "environment", then you don't need to read any further, since swapping out CodePush deployment keys is just like handling environment-specific config for any other service your app uses (e.g. Facebook). However, if you're looking for examples (**including demo projects**) on how to setup your build process to accommodate this, then refer to the following sections, depending on the platform(s) your app is targeting: | ||
@@ -271,0 +271,0 @@ * [Android](docs/multi-deployment-testing-android.md) |
@@ -42,3 +42,9 @@ var fs = require("fs"); | ||
// 2. Modify jsCodeLocation value assignment | ||
var oldJsCodeLocationAssignmentStatement = appDelegateContents.match(/(jsCodeLocation = .*)/)[1]; | ||
var jsCodeLocations = appDelegateContents.match(/(jsCodeLocation = .*)/); | ||
var oldJsCodeLocationAssignmentStatement; | ||
if (jsCodeLocations) { | ||
oldJsCodeLocationAssignmentStatement = jsCodeLocations[1]; | ||
} else { | ||
console.log('Couldn\'t find jsCodeLocation setting in AppDelegate.'); | ||
} | ||
var newJsCodeLocationAssignmentStatement = "jsCodeLocation = [CodePush bundleURL];"; | ||
@@ -45,0 +51,0 @@ if (~appDelegateContents.indexOf(newJsCodeLocationAssignmentStatement)) { |
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
New author
Supply chain riskA new npm collaborator published a version of the package for the first time. New collaborators are usually benign additions to a project, but do indicate a change to the security surface area of a package.
Found 1 instance in 1 package
1283286
187
3889
3